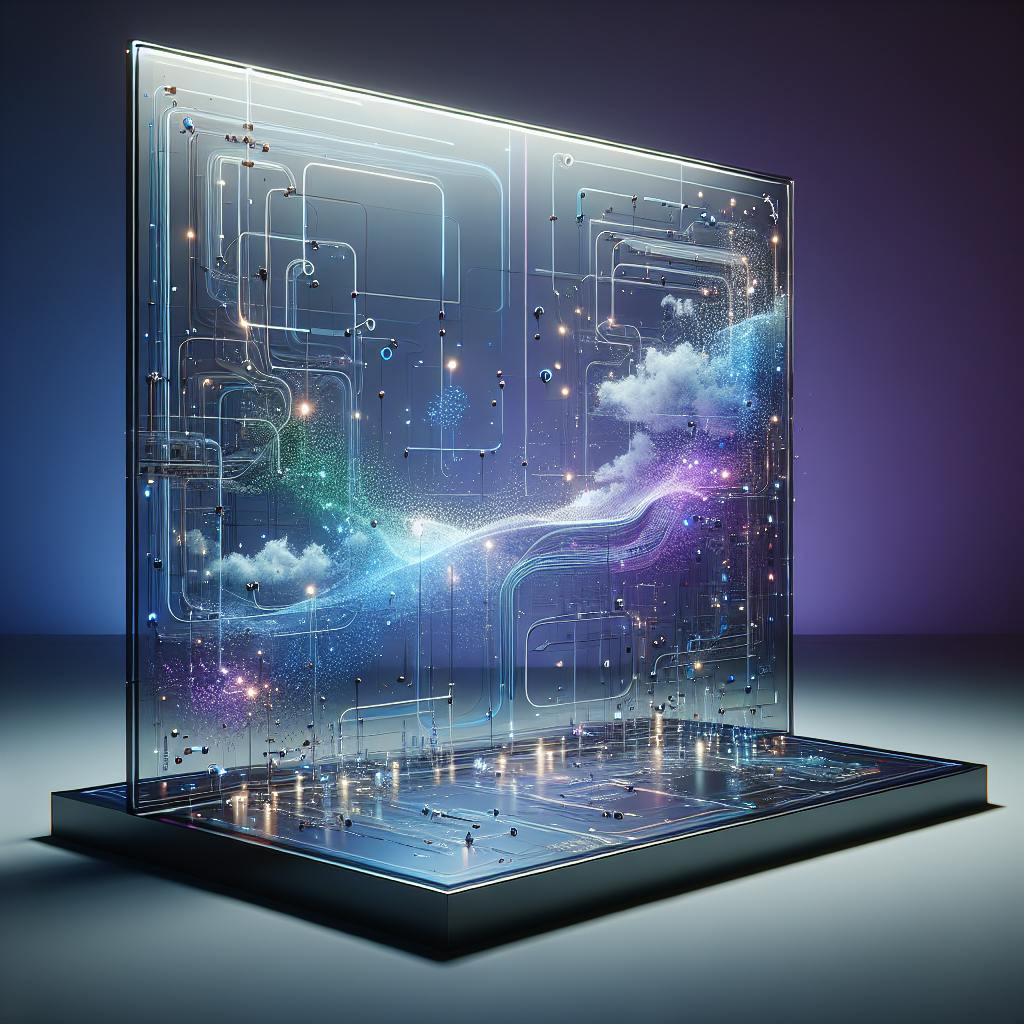
Discover how to integrate gift card APIs for increased sales and customer loyalty, including API selection, coding, testing, and troubleshooting.
Integrating gift card services into your software application offers numerous benefits, including increased sales, improved customer loyalty, and valuable insights into customer behavior. This guide covers the key steps for seamless gift card integration:
Choosing the Right Gift Card API
- Evaluate features like global reach, ease of integration, customization options, security measures, and scalability
- Popular APIs: Google Wallet API, Square Gift Cards API, Reloadly Gift Card API
Integrating the API
- Set up accounts and obtain API keys for authentication
- Configure API endpoints for communication
Coding Gift Card Features
- Purchase and activate gift cards
- Redeem gift cards and check balances
Testing and Deployment
- Test the integration thoroughly using sandbox environments
- Prioritize security and scalability for real-world deployment
Troubleshooting
- Handle API errors like rate limits and authentication issues
- Debug code by using logging, testing in isolation, and checking API documentation
Developer Resources
- Access API documentation for popular gift card APIs
- Join forums and communities for knowledge sharing
By following this guide, you can provide a seamless and rewarding gift card experience for your customers, driving engagement and revenue for your business.
sbb-itb-bfaad5b
Choosing a Gift Card API
When integrating gift card services into your software application, selecting the right gift card API is crucial. With numerous options available, it's essential to evaluate the features, compatibility, and scalability of each API to ensure it aligns with your project requirements.
Evaluating API Features
When evaluating a gift card API, consider the following key features:
Feature | Description |
---|---|
Global Reach | Does the API support gift cards from various countries and regions? |
Ease of Integration | How easily can the API be integrated into your existing system? |
Customization Options | Can the API be customized to fit your brand's identity and design? |
Security Measures | What security features does the API offer to protect against fraud and unauthorized access? |
Scalability | Can the API handle increased traffic and sales volume as your business grows? |
Popular Gift Card APIs
Some popular gift card APIs include:
API | Description |
---|---|
Google Wallet API | Offers a wide range of gift card options and seamless integration with Google's ecosystem. |
Square Gift Cards API | Provides a user-friendly interface and robust reporting features for tracking gift card sales and usage. |
Reloadly Gift Card API | Offers a developer-first approach with flexible integration options and a wide range of gift card brands. |
When selecting a gift card API, consider your project's specific needs and requirements. Evaluate the features, pricing, and scalability of each API to ensure it aligns with your business goals.
By choosing the right gift card API, you can provide your customers with a seamless and rewarding experience, increasing sales and customer loyalty. In the next section, we'll explore the process of integrating a gift card API into your software application.
Integrating a Gift Card API
Integrating a gift card API into your software application is a crucial step in providing a seamless and rewarding experience for your customers. In this section, we'll walk you through the process of integrating a gift card API, from setting up accounts and API keys to configuring API endpoints.
Setting Up Accounts and API Keys
To integrate a gift card API, you'll need to register for a developer account with the gift card service provider. This typically involves creating a username and password, providing some basic information about your company, and agreeing to the terms of service. Once you've registered, you'll be provided with an API key or access token, which you'll use to authenticate your API requests.
Here are the general steps to follow:
1. Register for a developer account: Go to the gift card service provider's website and sign up for a developer account. Fill out the required information, including your company name, email address, and password.
2. Obtain an API key or access token: After registering, you'll be provided with an API key or access token. This is a unique identifier that authenticates your API requests and connects them to your developer account.
3. Store your API key securely: Make sure to store your API key securely, using a secure key management system or environment variables. Never hardcode your API key into your code or share it publicly.
Configuring API Endpoints
Once you have your API key, you'll need to configure your API endpoints to communicate with the gift card service provider's API. This typically involves setting up HTTP requests, specifying the API endpoint URL, and defining the request parameters.
Here are the general steps to follow:
1. Choose the API endpoint: Determine which API endpoint you need to use, based on the gift card service provider's API documentation. For example, you might need to use a specific endpoint for purchasing gift cards, redeeming gift cards, or checking gift card balances.
2. Set up the HTTP request: Use a programming language like Python, Java, or Node.js to set up an HTTP request to the API endpoint. Specify the request method (e.g., GET, POST, PUT, DELETE), the endpoint URL, and any required headers or parameters.
3. Authenticate the request: Include your API key in the request headers or parameters, using the authentication method specified by the gift card service provider. This ensures that your request is authenticated and authorized.
4. Handle the response: Parse the response from the API endpoint, using a JSON parser or XML parser depending on the response format. Extract the relevant information, such as the gift card balance or transaction status.
By following these steps, you can successfully integrate a gift card API into your software application, providing a seamless and rewarding experience for your customers. In the next section, we'll explore the process of coding gift card features, including purchasing and activating gift cards, redeeming and checking balances, and handling errors and exceptions.
Coding Gift Card Features
This section explains how to implement key gift card features using APIs, with examples.
Purchasing and Activating Gift Cards
To purchase and activate gift cards, you need to integrate the gift card API with your application's checkout process. Here's an example using the Medusa JS Client:
medusa.giftCards.create({
amount: 50,
currency: 'USD',
expires_at: '2025-12-31T23:59:59.000Z'
}).then(({ gift_card }) => {
console.log(gift_card.id)
}).catch((e) => {
// handle error
})
This code creates a new gift card with a value of $50, expiring on December 31, 2025. You can then use the gift_card.id
to activate the gift card and make it available for redemption.
Redeeming and Checking Balances
To redeem a gift card or check its balance, you need to use the gift card API to retrieve the gift card details. Here's an example using the Fetch API:
fetch(`${BACKEND_URL}/store/gift-cards/${code}`, {
credentials: "include",
}).then((response) => response.json()).then(({ gift_card }) => {
console.log(gift_card.balance)
}).catch((e) => {
// handle error
})
This code retrieves the gift card details by sending a GET request to the /store/gift-cards/${code}
endpoint, where ${code}
is the gift card code. The response includes the gift card balance, which you can then use to update the user's account or display the balance to the user.
By following these examples, you can implement key gift card features in your application, providing a seamless and rewarding experience for your customers.
Testing and Deploying
Testing the Integration
To ensure your gift card integration works correctly and securely, you need to test it thoroughly. You can use the reference implementation repository, which includes a set of test API requests in a Postman collection. These test requests simulate the API requests that the Toast platform sends to your integration.
Additionally, you can send real requests from the Toast platform using the sandbox testing environment. The Toast integrations team can configure a test restaurant that uses your implementation and a restaurant employee account that you can use to sign into a POS device to simulate gift card transactions.
Testing in the Sandbox Environment
When testing in the sandbox environment, make sure to:
- Verify incoming JWTs with the sandbox public key
- Ensure your implementation is secure and can handle real-world scenarios
Security and Scalability
When deploying your gift card integration, consider the following:
Security
- Follow best practices for secure coding and data handling
- Use secure protocols for data transmission
- Encrypt sensitive data
- Implement access controls
Scalability
- Ensure your integration can handle a high volume of transactions and user activity
- Consider using load balancing, caching, and content delivery networks
- Ensure compliance with relevant industry standards and regulations, such as PCI-DSS for payment card security
By following these testing and deployment strategies, you can ensure that your gift card integration is robust, secure, and scalable, providing a seamless experience for your users.
Troubleshooting Integration Issues
Handling API Errors
When integrating a gift card API, errors can occur due to various reasons. To handle these errors, it's essential to implement robust error handling mechanisms. Here are some strategies to address common API errors:
Error Type | Solution |
---|---|
API Rate Limits | Implement rate limiting on your API requests to avoid hitting the API provider's rate limits. |
Authentication Errors | Verify that your API credentials are correct and up-to-date. Ensure that you're using the correct authentication method. |
Invalid Requests | Validate user input and ensure that the request payload is correct. Use API documentation to understand the required parameters and formats. |
Debugging Code
Debugging is an essential step in identifying and resolving issues within your gift card integration code. Here are some practical debugging tips:
1. Use Logging: Implement logging mechanisms to track API requests, responses, and errors. This helps you identify the source of the issue and debug more efficiently.
2. Test in Isolation: Isolate the problematic code and test it independently to identify the root cause of the issue.
3. Check API Documentation: Refer to the API documentation to ensure that you're using the correct endpoints, parameters, and formats.
By following these troubleshooting strategies, you can efficiently identify and resolve integration issues, ensuring a seamless gift card experience for your users.
Summary and Next Steps
In this guide, we've covered the essential steps for integrating gift card services into your software. From choosing the right API to testing and deploying your integration, we've provided you with practical tips and expert advice.
Key Takeaways
Here's a quick recap of the key points:
- Evaluate API features and select the best API for your needs
- Set up accounts and API keys for secure authentication
- Configure API endpoints for efficient communication
- Code gift card features for purchasing, activating, redeeming, and checking balances
- Test and deploy your integration for scalability and security
- Troubleshoot common integration issues and debug code
Next Steps
As you continue to develop and refine your gift card integration, remember to:
- Stay up-to-date with the latest API documentation and updates
- Monitor and analyze user feedback to identify areas for improvement
- Explore additional resources and tools to enhance your gift card experience
- Prioritize security and scalability to ensure a reliable and efficient integration
By following these guidelines, you'll be well on your way to creating a robust and user-friendly gift card integration that drives engagement and revenue for your business.
Developer Resources
Here, you'll find a curated list of useful resources to support developers in enhancing their integration skills.
API Documentation
API | Resource |
---|---|
Google Wallet | API Docs |
Square Gift Cards | API Docs |
Reloadly Gift Card | API Overview |
Early Access and Forums
Resource | Description |
---|---|
Cardtonic API Early Access | Join the waitlist for early access to Cardtonic's developer API for gift card integration. |
Developer Forums | Participate in online forums and communities to connect with other developers, ask questions, and share knowledge. |
These resources are designed to help you integrate gift card services into your software efficiently and effectively.