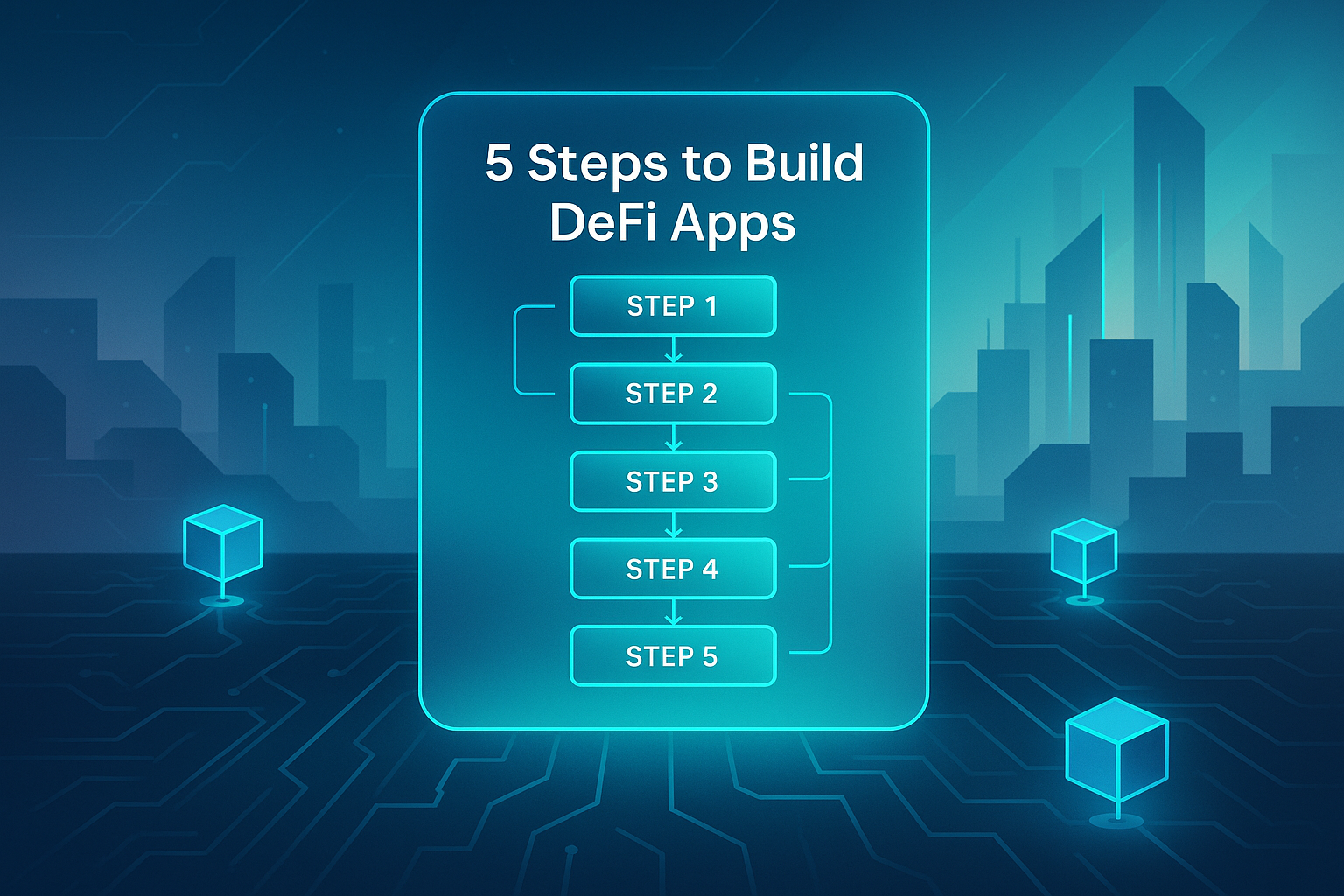
Learn how to create DeFi applications through five essential steps, from choosing a blockchain to launching and maintaining your app.
Want to create a DeFi app but don’t know where to start? Here’s a simple guide to help you get started, from choosing the right blockchain to launching your app. Follow these five steps:
- Choose a Blockchain Platform: Compare Ethereum, Solana, and Binance Smart Chain based on transaction speed, gas fees, and security.
- Plan Your App Structure: Focus on features like wallet integration, lending protocols, and governance systems. Design a user-friendly interface with strong security measures.
- Build Smart Contracts: Write secure and efficient contracts using tools like Solidity, Hardhat, and Truffle. Test thoroughly with audits and bug bounties.
- Connect Frontend and Backend: Use frameworks like React or Vue.js, integrate wallets (e.g., MetaMask), and ensure U.S. formatting standards for currency and dates.
- Launch and Maintain: Conduct security audits, track performance with tools like Tenderly, and monitor user activity to ensure growth.
Quick Comparison of Blockchain Platforms
Platform | Transaction Speed | Gas Fees | Security | Developer Tools |
---|---|---|---|---|
Ethereum | Moderate | High | Strong, established | Extensive resources |
Solana | Fast | Low | Efficient but newer | Focused on scalability |
Binance Smart Chain | Fast | Low | EVM-compatible, versatile | Easy for Ethereum devs |
Build a Full Stack DeFi Application: Code Along
1. Choose a Blockchain Platform
Selecting the right blockchain platform is key to balancing speed, user experience, and cost. Before making a choice, consider specific factors that will directly impact your DeFi project.
Key Factors to Consider
When comparing blockchain platforms, pay attention to these important elements:
Factor | Why It Matters for DeFi |
---|---|
Transaction Speed | Affects how quickly your platform can handle transactions, influencing scalability and user experience. |
Gas Fees | Determines transaction costs, which can impact user adoption and overall affordability. |
Smart Contract Support | Enables automation for complex financial tasks, essential for DeFi functionality. |
Security Features | Protects user assets and builds trust in your platform. |
Developer Tools | Simplifies development and testing, speeding up your project's timeline. |
Popular Platform Choices
Here’s a quick look at what some of the top blockchain platforms bring to the table for DeFi development:
Ethereum
- Known for its established ecosystem, Ethereum provides strong security and a wealth of developer resources. However, transaction fees can rise significantly during network congestion.
Solana
- Solana stands out for its fast transactions and low fees, making it ideal for projects like high-frequency trading. Its focus on scalability and efficiency makes it a solid pick for performance-driven applications.
Binance Smart Chain
- Binance Smart Chain supports Ethereum-based contracts via the Ethereum Virtual Machine (EVM). It offers lower fees and faster block confirmation times, making it a versatile option for various DeFi protocols.
For projects requiring speed and low costs, Solana is a strong contender. If you need proven security and compatibility, Ethereum remains a reliable choice.
2. Plan Your App Structure
Carefully design your DeFi app's structure to ensure smooth functionality and a user-friendly experience. This phase is crucial for defining the core features and setting up the foundation of your app.
Main Features
Focus on integrating the key components that make your DeFi app effective:
Feature Category | Core Components | Priority |
---|---|---|
Asset Management | Wallet integration, token swaps, liquidity pools | High |
Financial Tools | Lending protocols, yield farming, staking mechanisms | High |
User Control | Governance systems, voting mechanisms, proposal creation | Medium |
Risk Management | Oracle integration, automated market makers (AMMs) | High |
Analytics | Portfolio tracking, transaction history, yield calculations | Medium |
For each feature, define specific rules and dependencies to ensure smooth integration.
Interface Design
Follow U.S. standards for formatting to make your app intuitive for users:
- Currency: Use USD format (e.g., $1,000.00).
- Dates: Display in MM/DD/YYYY format.
- Time: Use a 12-hour clock (e.g., 2:30 PM EDT).
- Percentages: Show with two decimal places (e.g., 5.25%).
The interface should be clean and easy to navigate, helping users quickly understand and interact with key features.
Security Measures
Implement strong security protocols to protect your app and its users:
Security Layer | Implementation Details | Purpose |
---|---|---|
Smart Contract Auditing | Use multiple third-party audits | Identify vulnerabilities |
Access Control | Require multi-signature approvals | Secure administrative access |
Rate Limiting | Set transaction caps and cooling periods | Prevent flash loan attacks |
Oracle Security | Integrate Chainlink Price Feeds | Ensure accurate price data |
Emergency Controls | Add circuit breakers and pause mechanisms | Halt attacks in progress |
Combine these measures with automated monitoring systems to detect and respond to anomalies quickly. These security strategies align with earlier decisions about your blockchain platform and smart contract design.
3. Build Smart Contracts
Once your app plan is finalized, the next step is to create smart contracts that are both secure and efficient.
Writing Smart Contracts
Developing smart contracts demands a focus on functionality and security. For Ethereum-based DeFi applications, use Solidity 0.8.19 or later as your starting point. Organize your contracts using established design patterns for clarity and maintainability:
Contract Layer | Purpose | Key Components |
---|---|---|
Core Logic | Main business functionality | Token standards, lending protocols |
Access Control | Permission management | Role-based access controls, admin functions |
Security | Protection mechanisms | Reentrancy guards, basic safeguards |
Integration | External connections | Oracle interfaces, cross-chain bridges |
Modular architecture is essential for future updates and maintenance. Use standardized interfaces like ERC-20 or ERC-721 to ensure compatibility with other systems and improve overall security.
Testing Your Contracts
Thorough testing is critical to ensure your smart contracts function as intended. Use tools like Hardhat or Truffle to perform both unit and integration tests. Focus on:
- Function paths
- Access controls
- Error handling
- State transitions
Don't forget to test external integrations, such as oracle feeds and cross-contract interactions. A multi-step security audit is also vital:
- Automated scans to identify vulnerabilities
- Manual reviews by security professionals
- Formal verification of critical functions
- Bug bounty programs to catch overlooked issues before deployment
These steps will help you ensure a smooth and secure deployment process.
Recommended Development Tools
Choose tools that align with your project's specific needs. Here's a quick guide:
Tool Category | Recommended Options | Primary Use Case |
---|---|---|
Development Environment | Remix IDE, Visual Studio Code | Writing and debugging smart contracts |
Testing Framework | Hardhat, Foundry | Automated testing and deployment |
Security Analysis | Slither, MythX | Identifying vulnerabilities |
Deployment Tools | Truffle, Brownie | Contract deployment and verification |
Monitoring | Tenderly, Etherscan | Runtime analysis and debugging |
Streamline collaboration and deployment by using GitHub and setting up CI/CD pipelines. Additionally, document everything - contract functions, security protocols, deployment steps, and upgrade paths - to ensure clarity and consistency throughout your project.
sbb-itb-bfaad5b
4. Connect Frontend and Backend
Once you've established secure smart contracts and a well-structured app design, the next step is to connect your frontend and backend. This connection ensures your DeFi application runs smoothly and efficiently.
UI Development
For modern DeFi apps, a strong frontend framework is essential to handle real-time blockchain interactions. Popular choices include React and Vue.js, known for their component-based architecture and compatibility with Web3 libraries.
Component Layer | Key Technologies | Functions |
---|---|---|
Frontend Framework | React 18+, Vue.js 3 | Component rendering, state management |
Web3 Integration | ethers.js 6.0, web3.js | Blockchain interaction, contract calls |
State Management | Redux Toolkit, Vuex | Tracking transactions, wallet states |
UI Components | Material-UI, Tailwind CSS | Responsive design, DeFi-specific elements |
When working with smart contract interactions, hooks are a practical way to manage blockchain states. Here's an example:
const useContractInteraction = () => {
const [txStatus, setTxStatus] = useState('idle');
const [balance, setBalance] = useState('0');
// Contract interaction logic
const executeTransaction = async () => {
setTxStatus('pending');
try {
// Contract call implementation
} catch (error) {
setTxStatus('error');
}
};
};
The next step is to establish secure wallet connectivity for handling blockchain transactions.
Wallet Connection
A secure and user-friendly wallet connection is a cornerstone of any DeFi application. While MetaMask is the most commonly used wallet, your app should also support multiple providers using the WalletConnect 2.0 protocol.
Key wallet integration features to include:
- Network detection and switching: Automatically identify the connected network and prompt users to switch if necessary.
- Transaction signing: Guide users through transaction processes with clear and transparent feedback.
- Balance monitoring: Provide real-time updates on token balances and transaction statuses.
Once wallet connectivity is in place, focus on presenting data in a way that aligns with U.S. market conventions.
US Market Standards
To meet U.S. formatting standards for numbers and currency, use the Intl.NumberFormat API. This ensures consistency in how amounts are displayed across your app:
const formatter = new Intl.NumberFormat('en-US', {
style: 'currency',
currency: 'USD',
minimumFractionDigits: 2
});
// Format amount
const amount = formatter.format(1234.56); // "$1,234.56"
This approach ensures your DeFi app meets user expectations while maintaining a professional and polished interface.
5. Launch and Maintain
Launch Steps
Before deploying to the mainnet, ensure a thorough checklist is completed. Conduct comprehensive security audits with trusted providers like CertiK or Trail of Bits to safeguard your project.
Here are the key deployment steps:
- Contract Verification: Submit your smart contracts for verification on platforms like Etherscan to build trust and transparency.
- Gas Optimization: Review and fine-tune your gas usage to improve efficiency.
- Documentation: Create clear and detailed user guides and documentation to assist users and developers.
Once the deployment is successful, the focus should shift to monitoring performance and driving growth.
Performance Tracking
Set up effective monitoring systems to keep an eye on your DeFi app's performance. Use real-time analytics tools for different aspects of your app, such as:
Monitoring Aspect | Tools | Key Metrics |
---|---|---|
Smart Contracts | Tenderly | Gas usage, transaction success rates |
User Activity | Dune Analytics | Active users (daily/monthly), total value locked |
Security | Forta Network | Suspicious transactions, potential threats |
Network Status | The Graph | Chain-specific metrics, indexing performance |
Automated alerts can help you quickly identify and address issues like unusual transaction behavior, contract errors, or liquidity fluctuations.
Growth Management
Post-launch, keep a close eye on performance metrics and user feedback. Regularly update your app to maintain a balance between scalability and security, ensuring a smooth experience for users.
Developer Resources
Explore tools and materials designed to support your DeFi development journey.
daily.dev Tools
Developing DeFi applications means keeping up with the fast-changing world of blockchain technology. daily.dev simplifies this by offering a tailored news feed and access to active developer communities.
The platform’s customized news feed, powered by advanced algorithms, helps you stay updated on topics like smart contract development, blockchain security, and gas optimization. You can adjust your feed to focus on areas such as:
- Smart contract development
- Blockchain security protocols
- Gas optimization techniques
- Frontend integration patterns
For teamwork, the Squads feature (currently in Beta) allows development teams to collaborate in private spaces. These spaces are perfect for sharing resources, solving technical issues, and organizing documentation - key for tackling complex projects like smart contract audits or security reviews. These tools integrate smoothly into your DeFi development workflow.
Learning Materials
daily.dev’s browser extensions make it easy to access curated DeFi resources instantly. With over 1,000,000 developers on the platform, there’s a wealth of shared knowledge, including:
Resource Type | How It Helps DeFi Developers |
---|---|
Tutorials | Step-by-step guides for creating and testing smart contracts |
Security Updates | Alerts on vulnerabilities and recommended practices |
Community Discussions | Peer reviews and shared solutions for common problems |
Development Tools | Insights into new testing frameworks and deployment tools |
The Ask AI feature provides quick answers to technical questions, whether it’s debugging a smart contract or improving gas efficiency. Additionally, daily.dev’s verified company badges create a trusted community by validating developer credentials. With a mix of tutorials, tool comparisons, and product updates, the platform ensures you stay informed and ready to tackle challenges in the fast-moving DeFi space.
These resources make daily.dev a valuable partner for staying ahead in the world of DeFi.
Summary
Main Points
Creating a DeFi application involves five key development stages. Each stage builds on the last to deliver a secure and user-friendly decentralized finance solution:
Development Stage | Key Focus Areas |
---|---|
Platform Selection | Compatibility, scalability, and gas fees |
App Structure | Security setup, user experience, feature focus |
Smart Contracts | Development guidelines, testing, and audits |
Integration | Frontend-backend links, wallet support, and US compliance |
Launch & Growth | Deployment prep, monitoring, and performance tracking |
These steps ensure your DeFi app meets technical needs, user expectations, and US compliance standards.
Future Development
Beyond these initial stages, staying ahead of industry trends is crucial for ongoing success. Continuous learning keeps DeFi applications competitive. daily.dev can be a helpful tool for tracking changes in the industry. Its personalized news feed allows you to stay updated on:
- Smart Contract Updates: Learn about new security measures and optimization techniques.
- Compliance Changes: Keep up with US market regulations.
- Technical Advancements: Follow blockchain platform updates.
With browser extensions and community features, daily.dev simplifies the process of monitoring DeFi trends. Its search tools and shared insights help you navigate changes in the space, ensuring your app stays secure and relevant.
FAQs
What are the main differences between Ethereum, Solana, and Binance Smart Chain for developing DeFi apps?
Ethereum, Solana, and Binance Smart Chain (BSC) each offer unique advantages for DeFi app development, depending on your project needs.
- Ethereum: Known for its robust ecosystem and widespread adoption, Ethereum provides unmatched decentralization and security. However, it often faces high gas fees and slower transaction speeds.
- Solana: Solana is designed for high-speed, low-cost transactions, making it ideal for scalable DeFi applications. It uses a unique consensus mechanism called Proof of History, but its ecosystem is smaller compared to Ethereum.
- Binance Smart Chain: BSC is popular for its affordability and compatibility with Ethereum’s tools (EVM). It offers fast transactions at low costs but is considered less decentralized than Ethereum.
Choosing the right blockchain depends on factors like transaction volume, budget, and the level of decentralization your app requires.
What steps can I take to ensure my DeFi app is secure during development and after launch?
To ensure the security of your DeFi app, focus on these key areas:
- Smart Contract Audits: Conduct thorough audits of your smart contracts using trusted third-party security firms to identify vulnerabilities before deployment.
- Code Reviews: Perform regular peer reviews and testing to catch bugs or potential exploits in your codebase.
- Use Established Frameworks: Build on well-tested and widely adopted blockchain platforms to minimize risks associated with unproven technologies.
- Implement Security Best Practices: Follow industry standards like secure key management, multi-signature wallets, and rate-limiting to protect user funds and data.
- Ongoing Monitoring: Set up systems to monitor your app for unusual activity or potential threats after launch.
By taking these precautions, you can help safeguard your DeFi app and build trust with your users.
What are the best tools and resources for testing and deploying smart contracts in a DeFi app?
When building a DeFi application, testing and deploying smart contracts are critical steps to ensure functionality and security. Popular tools for these tasks include Hardhat and Truffle, which provide robust frameworks for developing, testing, and deploying smart contracts on Ethereum and other blockchains. Ganache is another useful tool for creating a local blockchain environment to simulate transactions during testing.
For deployment, platforms like Remix IDE offer a user-friendly interface to write, compile, and deploy smart contracts directly to a blockchain. Additionally, services such as Infura or Alchemy can help connect your application to the blockchain network efficiently.
Remember to thoroughly test your contracts on a testnet like Ropsten or Goerli before deploying them to the mainnet to avoid potential risks and ensure smooth operation.