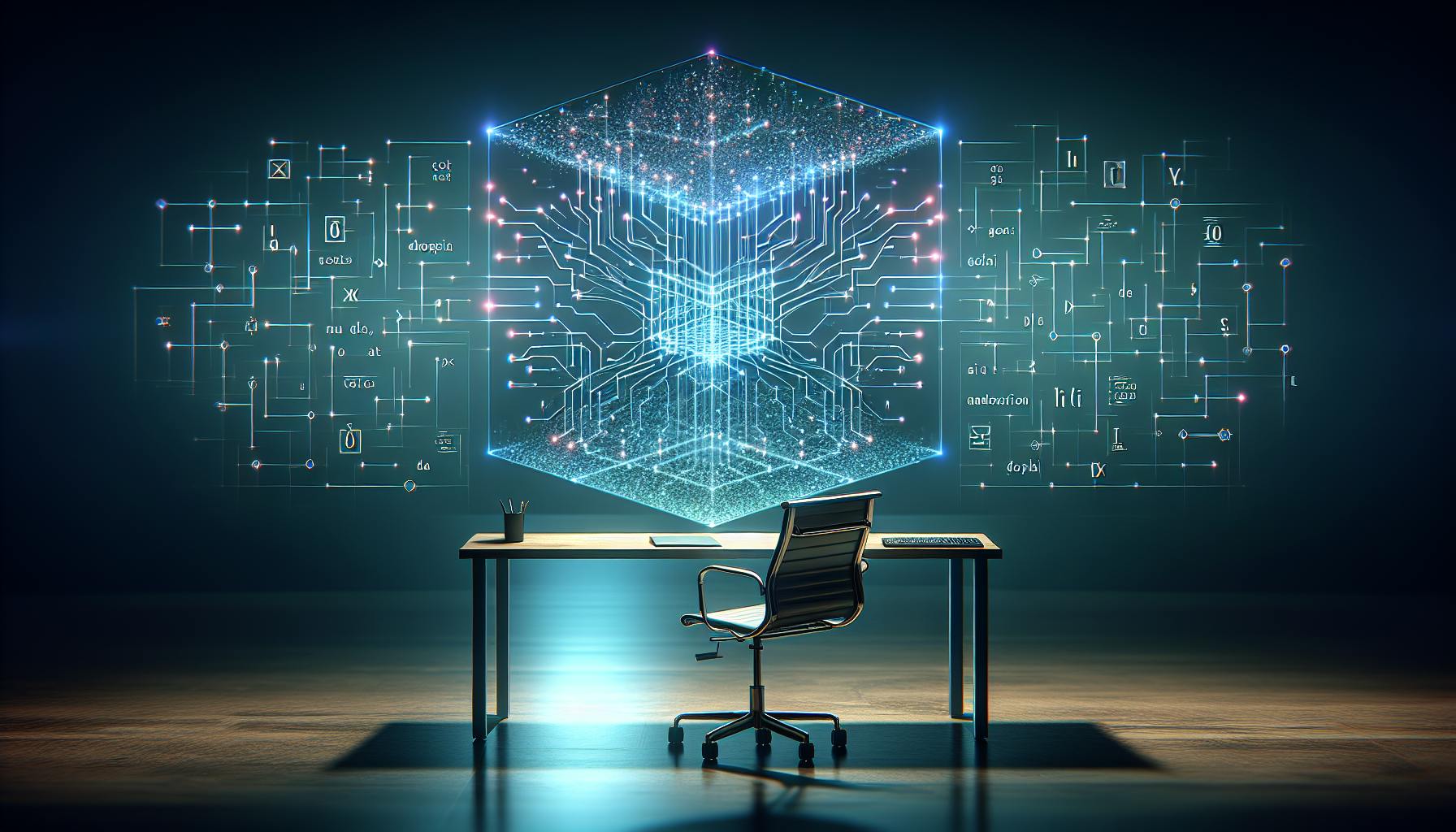
Explore array object essentials for developers, from understanding array objects to advanced techniques and best practices across various programming languages. Master arrays for efficient data management.
Most developers would agree that efficiently managing data is critical, yet often challenging.
Mastering array objects in your code unlocks simpler data manipulation and cleaner architecture patterns...
Understand what array objects are, explore core concepts like indexing and mutation, and apply best practices across JavaScript, Java, PHP, React, C++, and more.
Introduction to Array Objects in Programming
Arrays are one of the most fundamental data structures used across programming languages. As indexed collections of data, they allow developers to store and access related pieces of information efficiently. This makes them essential for managing real-world data in applications.
Understanding Array Objects
At their core, arrays are objects that contain an ordered list of values. The values can be of any data type, like numbers, strings, booleans, objects, and even other arrays. Arrays are created with bracket notation []
and values are separated by commas inside the brackets.
For example, an array holding the numbers 1 through 5 would be defined as:
let numbers = [1, 2, 3, 4, 5];
The index of each value starts at 0 for the first item, then 1 for the second item, and so on. So in the above array, 1
has an index of 0, 2
has an index of 1, etc.
Arrays have built-in properties and methods to manipulate the data they contain, like .length
to get the number of items, .push()
to add items, .pop()
to remove items, and more.
The Role of Arrays in Data Management
Arrays provide an effective way to work with real-world data in applications. For example:
- Storing a list of user contacts or products in a database
- Displaying a set of images in a photo gallery
- Managing a queue or stack data structure for task processing
- Representing colors in an image pixel-by-pixel
By collecting data into arrays, developers can loop through and manipulate values efficiently in bulk. This makes common tasks like searching, sorting, inserting, updating and deleting data much simpler.
Arrays are more flexible and powerful than handling many separate variables. And built-in array methods reduce the need to write manual data processing logic.
Zero-Indexed Collections and Data Types
The index of arrays starts counting at 0 rather than 1. So the first value is accessed as array[0]
, the second as array[1]
, and so on. This zero-based system is standard across programming languages.
Arrays can contain values of any data type - numbers, strings, booleans, objects, and even other arrays. For example:
let mixedData = [5, "Hello", true, {name: "John"}, [1, 2, 3]];
The ability to mix data types and nest arrays enables flexible data modeling for real-world information.
Certain languages also support typed arrays that enforce a single data type for all values. These include typed arrays in JavaScript and array types like Array<int>
in C#.
Real-World Examples of Array Usage
Here are some examples of using arrays in real applications:
- Store a user's purchase history in a shopping cart
- Display search results from a database query
- Manage a playlist of songs in a music app
- Analyze pixel color data in an image editor
- Simulate a deck of cards in a game
- Control turn order for players in a strategy game
No matter the language or project, arrays offer a versatile way to handle real data for all kinds of digital applications and software systems. Understanding them is key for any developer.
What is an array object?
An array object is a data structure that contains a collection of elements accessed using an index. In JavaScript, arrays are high-level list-like objects with useful built-in methods to perform traversal and mutation operations.
Some key characteristics of JavaScript array objects include:
-
Indexed - Array elements are accessed using a zero-based index. For example, the first element is referenced with
[0]
, second with[1]
, and so on. -
Dynamic size - Arrays can grow and shrink in size automatically as elements are added or removed.
-
Fast lookup - Arrays allow fast lookup of elements based on their index. This makes them useful for storing and accessing sequential data.
-
Helper methods - Many useful methods are built-in for common operations like sorting, reversing, mapping, filtering, reducing, etc.
At their most basic level, JavaScript arrays are containers that allow storing a sequence of elements together in memory for easier access. This makes them extremely useful when working with data sets and collections. Understanding arrays is key to effectively managing and manipulating data in JavaScript.
Some common use cases for JavaScript arrays include:
- Storing lists of data like task lists or shopping cart items
- Queue and stack data structures
- Returning multiple values from a function
- Caching values or results
- Data transformations and algorithms
In summary, the array object is a fundamental building block in JavaScript. Mastering arrays allows efficient data access and manipulation for improved coding productivity.
What is an example of an array object?
Arrays are "lists" of related values. Every value in the array is usually of the exact same type and only differentiated by the position in the array. For example, all the quiz scores for a test could be stored in an array with the single variable name: quiz_scores
.
Here is a simple example of an array object in JavaScript:
let quizScores = [80, 90, 95, 100];
This array contains 4 number values representing the scores of 4 students on a quiz.
Some key things to note:
- The array is declared using square brackets
[]
- Each value (element) is separated by a comma
- The position of the value in the array matters
- You can access each value by its index, starting at 0:
quizScores[0]
= 80quizScores[1]
= 90quizScores[2]
= 95quizScores[3]
= 100
So an array allows you to store multiple related values under a single variable name, and easily work with those values by referring to their index position.
This makes arrays very useful for storing and manipulating collections of data in programming. Some other examples of arrays could include:
- A list of student names
- A list of product prices
- A list of scores from multiple test attempts
- A list of tasks to complete
The key benefit is having all related data stored together in an ordered, indexed way for easy access and manipulation.
What is an example of an array like object?
One common example of an array-like object in JavaScript is the arguments
object. The arguments
object is available inside functions and contains all the arguments passed to that function.
For example:
function sum() {
console.log(arguments);
}
sum(1, 2, 3); // { '0': 1, '1': 2, '2': 3 }
The arguments
object has a length
property and its elements can be accessed via bracket notation, similar to arrays. However, it lacks built-in array methods like push()
, pop()
etc.
Another array-like object is NodeList
, which represents a collection of nodes selected from a document. For example, when you query elements with document.querySelectorAll()
, it returns a NodeList.
const divs = document.querySelectorAll('div'); // NodeList
The NodeList
can be iterated over with for...of
loops, has a length
property, and elements can be accessed via index. But it also lacks native array methods.
So in summary - array-like objects behave similar to arrays in some ways, but lack the full functionality. Common examples include arguments
, NodeList
, and HTMLCollection
.
What is array object in Python?
An array in Python is an ordered collection of elements that are accessed via indices. It allows storing multiple values of the same data type in a single variable.
Some key characteristics of Python arrays:
- They have a fixed size that needs to be defined upon creation
- The elements are all required to be of the same data type (e.g. all integers, all strings etc.)
- Elements can be accessed via zero-based indexing using square brackets
- Common operations like appending, inserting, deleting are available
- Useful built-in functions exist for frequent tasks like sorting, reversing, counting elements etc.
Here is a simple example of creating a Python array of integers and accessing the third element:
import array
my_array = array.array('i', [1, 2, 3, 4])
print(my_array[2]) # Prints 3
The array module needs to be imported first. Then we initialize a new integer array with 4 elements. We can print the third element (index 2) to confirm arrays are zero-indexed in Python.
Some use cases where arrays are preferred over lists in Python:
- When memory efficiency is critical since arrays consume less memory
- When working with large data sets for numerical/scientific computing
- When speed is important since arrays are faster than lists for certain operations
So in summary, arrays allow efficient storage and manipulation of homogeneous sequential data in Python. They strike a balance between convenience and performance.
Core Concepts of Array Objects in JavaScript
Delving into the specifics of array objects in JavaScript, examining their properties, methods, and behaviors.
Array Object Properties: Length and Indexing
Arrays in JavaScript are zero-indexed, meaning the first element is accessed via the index 0 rather than 1. The length
property reflects the number of elements contained in the array. We can access array elements using bracket notation and the index, like array[0]
, or use methods like array.push()
and array.pop()
to add and remove elements from the end of an array. The length is dynamically updated as elements are added and removed.
Some key points on array length and indexing:
- Arrays begin indexing at 0 rather than 1
- The
length
property reflects the count of elements - Elements can be accessed via bracket notation using the index
- Useful methods for adding/removing elements are
push()
,pop()
, etc. - Length updates automatically as elements are inserted and deleted
Understanding how indexing and length works is essential for efficiently accessing and manipulating array contents.
Traversal and Mutation Operations in JavaScript Arrays
We can traverse arrays and mutate their contents using a variety of built-in methods:
push()
/pop()
- Add/remove elements from endunshift()
/shift()
- Add/remove elements from beginningsplice()
- Insert/delete elements at a given indexslice()
- Extract a portion of an array as a new arrayindexOf()
/lastIndexOf()
- Find index of element's first/last occurrence
Here is an example showing how we can use these methods to mutate array contents:
let fruits = ['apple', 'banana', 'orange'];
fruits.push('grape'); // ['apple', 'banana', 'orange', 'grape']
fruits.unshift('strawberry'); // ['strawberry', 'apple', 'banana', 'orange', 'grape']
fruits.splice(2, 1); // ['strawberry', 'apple', 'orange', 'grape'] (removed 1 element at index 2)
let citrus = fruits.slice(2); // ['orange', 'grape'] (new array extracted from index 2)
These methods allow flexible approaches to updating arrays and extracting data.
Creating Shallow and Deep Copies of Arrays
When copying arrays, we need to decide whether a shallow or deep copy is required:
- Shallow - Copies object references, so nested objects are not duplicated. Use methods like
slice()
,concat()
, spread syntax. - Deep - Fully independent copy, with all nested objects duplicated. Use
structuredClone()
,JSON.parse(JSON.stringify(array))
.
Here is an example of shallow vs deep copying:
let arr = [{id: 1}];
// Shallow copy
let arrCopy = arr.slice();
// Modify nested object
arr[0].id = 2;
// arrCopy is also changed since objects are passed by reference!
console.log(arrCopy[0].id); // 2
// Deep copy
let deepArrCopy = structuredClone(arr);
// Modify original nested object
arr[0].id = 3;
// deepArrCopy remains unchanged
console.log(deepArrCopy[0].id); // 2
The method used depends on if we want to duplicate nested objects or pass references.
Using Array.prototype Methods in JavaScript
The Array
global object has a variety of methods on its prototype for manipulating arrays:
- Iteration -
forEach()
,map()
,filter()
, etc. - Searching -
indexOf()
,lastIndexOf()
,includes()
- Transformation -
join()
,slice()
,concat()
etc. - Sorting -
reverse()
,sort()
- Other -
toString()
,toLocaleString()
, etc.
We access these methods directly on arrays:
let fruits = ['apple', 'banana', 'orange'];
// Use forEach() to iterate
fruits.forEach(fruit => {
console.log(fruit);
});
// map() to transform elements
let upperCaseFruits = fruits.map(fruit => fruit.toUpperCase());
// indexOf() to search
console.log(fruits.indexOf('banana')); // 1
These built-in methods enable us to work with arrays in a simple yet flexible way. Exploring the full JavaScript array API unlocks additional functionality.
sbb-itb-bfaad5b
Manipulating Array Objects in Java
Array Object Example in Java
Here is a simple example of declaring, initializing, and working with an array in Java:
// Declare an array
int[] numbers;
// Initialize with 10 elements
numbers = new int[10];
// Assign values
numbers[0] = 5;
numbers[1] = 2;
// Print the array length
System.out.println(numbers.length); // Prints 10
// Loop over array
for(int i = 0; i < numbers.length; i++) {
System.out.println(numbers[i]);
}
This demonstrates creating an integer array, assigning values, printing the length property, and looping through to access each element.
Typed Arrays and Data Types in Java
Java arrays have fixed types, unlike JavaScript arrays which are untyped. The type goes after the variable name:
int[] numbers; // array of ints
String[] names; // array of Strings
MyClass[] items; // array of MyClass objects
You cannot store different data types in the same Java array. The array type must match the element type.
Java has typed array classes for primitive types like int[]
and double[]
. For objects, the array type uses the class name like String[]
.
Array Object Operations: Joining and Slicing
Java does not have built-in join or slice methods. But we can implement similar functionality:
Joining:
String[] fruits = {"apple", "banana", "orange"};
String fruitString = String.join(", ", fruits);
// "apple, banana, orange"
Slicing:
int[] numbers = {1, 2, 3, 4, 5};
int[] slice = Arrays.copyOfRange(numbers, 1, 3);
// slice contains [2, 3]
The Arrays
class provides useful utility methods for common array operations.
Loops and Iteration Over Java Arrays
We can loop over arrays in Java with standard loops:
String[] cars = {"Volvo", "BMW", "Ford"};
// for loop
for(int i = 0; i < cars.length; i++) {
System.out.println(cars[i]);
}
// for-each
for(String car : cars) {
System.out.println(car);
}
The for-each style is preferred for easy element access.
While arrays in Java are less flexible than JavaScript, these examples demonstrate their core usage and capabilities. Typed arrays ensure type safety.
PHP and Array Objects: Associative Arrays and More
Understanding Associative Arrays in PHP
Associative arrays in PHP use key-value pairs instead of numeric indexes to store and access data. This provides a more intuitive way to represent real-world data structures.
For example, we can store user data in an associative array like:
$user = [
'name' => 'John',
'email' => 'john@example.com',
'age' => 30
];
We can then easily access the values using the keys, like echo $user['name']; // Prints "John"
.
Some key benefits of associative arrays in PHP include:
- More self-documenting code compared to numeric arrays
- Flexible lookup using any scalar data type for keys
- Ability to iterate over keys and values
- Built-in functions like
array_key_exists()
Overall, associative arrays are extremely useful for managing collections of structured data in PHP applications.
Array Object PHP Functions
PHP provides many built-in functions for working with arrays as first-class objects:
array_push()/array_pop()
- Add/remove elements from the end of an arrayarray_shift()/array_unshift()
- Add/remove elements from the beginning of an arrayarray_slice()
- Extract a slice/subset of an arrayarray_splice()
- Remove/replace elements from an arrayarray_merge()
- Merge one or more arrays togetherarray_map()
- Apply a callback to array elementsarray_filter()
- Filter array elements based on a callback
For example:
$fruits = ['apple', 'banana'];
array_push($fruits, 'orange'); // $fruits is now ['apple', 'banana', 'orange']
$citrus = array_slice($fruits, 1); // $citrus contains ['banana', 'orange']
These functions allow efficient manipulation and transformation of array data structures in PHP.
Object Property Collection with PHP Arrays
We can use associative arrays in PHP to represent and work with object properties.
For example, we can store user data like:
$userProperties = [
'first_name' => 'John',
'last_name' => 'Doe',
'email' => 'john@example.com'
];
And access it like an object:
echo $userProperties['first_name']; // Prints "John"
We can also iterate through the properties:
foreach ($userProperties as $key => $value) {
echo "$key: $value";
}
This technique is useful when working with external APIs that return property collections as arrays.
Overall, associative arrays provide a great way to represent objects in PHP.
Sparse Arrays and Their Management in PHP
Sparse arrays in PHP contain "gaps" - indexes without defined values. For example:
$sparse = [1 => 'first', 3 => 'third']; // Index 2 is missing
PHP efficiently handles sparse arrays without allocating memory for the missing elements. However, adding elements later can be inefficient:
$sparse[] = 'new'; // Adds at end, leaving gap
To fill gaps, explicitly set undefined indexes:
$sparse[2] = 'second'; // Fills gap
When iterating sparse arrays, undefined indexes will be filled with default values.
Overall, explicitly handling gaps in sparse arrays allows optimizing performance and memory usage in PHP.
React and Array Objects: State Management and Rendering
Array objects are a fundamental data structure in JavaScript and are extremely useful in React for managing state and rendering lists of elements. Here's a deep dive into how arrays enable efficient coding practices in React.
Array Object React Example: Rendering Lists
A common use case for arrays in React is to render a list of components dynamically based on state. For example:
const [items, setItems] = useState([]);
return (
<ul>
{items.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
This iterates through the items
array using .map()
and renders a <li>
for each one. Updating the items
array triggers a re-render to display the new list.
State Management with Arrays in React
Managing state with arrays in React requires care to avoid performance issues. For example, mutating the array directly on state causes unnecessary re-renders:
// Anti-pattern!
const addItem = newItem => {
setItems([...items, newItem]);
};
Instead, create a new copy of the array to safely update it:
// Better approach
const addItem = newItem => {
setItems(prevItems => [...prevItems, newItem]);
};
This ensures only changed items re-render for better performance.
Array of Objects React Patterns
An array of objects is a common way to structure state in React. Some useful patterns include:
useReducer
for complex state updates- Caching array reads for fewer renders
- Pagination for long lists using
slice()
- Search/filter using
filter()
These patterns optimize working with array state.
Performance Considerations for Arrays in React
To optimize React performance with arrays:
- Use
React.memo()
on list components - Virtualize long lists with react-window
- Paginate data rather than rendering all at once
- Avoid hidden expensive computations in renders
Careful use of arrays is key for smooth UIs.
Array Objects in C++: Syntax and Performance
Array objects are a fundamental data structure in C++ used to represent collections of elements accessible by index. They provide efficient storage and access of data.
Understanding arrays in C++ requires knowledge of:
- Declaration syntax and initialization
- Accessing elements via index
- Passing arrays to functions
- Multi-dimensional arrays
- Dynamic allocation
- Performance considerations
Array Object Example in C++
Here is an example declaring a simple integer array in C++ with 5 elements:
int myArray[5]; // Declare array of 5 ints
myArray[0] = 1; // Initialize first element
int x = myArray[3]; // Get 4th element
Key things to note:
- Square brackets
[]
declare an array - Elements indexed starting at 0
- Arrays contain contiguous block of memory
Typed Arrays and Buffer Management in C++
C++ allows creating typed arrays that only hold a specific data type like int
or float
. This enables type safety and efficient buffer management.
For example:
// Typed array of floats
float temps[10];
// Array bounds checking in C++
if (index < 0 || index >= temps.size()) {
// Out of bounds error
}
Typed arrays in C++ manage their own memory buffer allocation. The array knows its size and type, enabling bounds checking.
Deep Copy of Array Objects in C++
When assigning or passing C++ arrays, they get shallow copied by default, meaning only the reference gets copied. To avoid unintended data sharing from shallow copies, deep copy the array manually:
int arr1[5] = {1, 2, 3, 4, 5};
// Shallow copy
int arr2 = arr1;
// Deep copy
int arr3[5];
std::copy(arr1, arr1 + 5, arr3);
Now arr1
and arr3
occupy separate memory buffers.
Traversal and Mutation of C++ Arrays
Common ways to traverse and mutate C++ arrays:
- Index-based access: Use bracket notation and index to access elements
- Range-based for loops: Iterate through elements cleanly
- std::sort(): Sort elements of array
- std::transform(): Apply function to each element
Here is an example showcasing some array operations:
int arr[5] = {3, 5, 2, 4, 1};
// Double each element
std::transform(arr, arr + 5, arr,
[](int x) { return 2 * x; });
// Sort the array
std::sort(arr, arr + 5);
// Print elements
for (int i : arr) {
std::cout << i << " "; // 4 2 8 6 10
}
Understanding syntax and performance of arrays in C++ enables efficient data access and manipulation.
Advanced Array Techniques and Best Practices
Exploring more advanced array techniques and best practices for developers to write clean and efficient code.
Array Destructuring and Spread Syntax
The spread syntax (...
) and array destructuring in JavaScript provide concise ways to copy and access array elements.
Destructuring allows directly assigning parts of an array to variables:
let colors = ["red", "green", "blue"];
let [firstColor, secondColor] = colors;
console.log(firstColor); // "red"
console.log(secondColor); // "green"
The spread syntax clones an array or inserts all elements of one array into another:
let colors = ["red", "green", "blue"];
let clonedColors = [...colors];
let combinedColors = ["yellow", ...colors];
These improve readability by avoiding complex array operations like slice()
and splice()
.
Handling Sparse Arrays and Undefined Elements
Sparse arrays contain "holes" where some elements are undefined. This happens when deleting elements or setting them to undefined
.
JavaScript arrays allow sparse elements, but other languages like Java do not.
Strategies for handling sparse arrays:
- Use
.filter()
to remove undefined elements - Use
.map()
and check for undefined before mapping - Use
.find()
to check if an index is defined - Initialize array with
fill()
method to avoid sparseness
Array-Like Objects: NodeList and HTMLCollection
In DOM programming, NodeList
and HTMLCollection
represent lists of elements but lack some Array methods.
Converting them into arrays allows full functionality:
// Get all divs
let divs = document.querySelectorAll("div");
// Convert to array
let divArray = Array.from(divs);
divArray.forEach(div => {
// Can now use array methods
});
Immutable Array Patterns
Mutating arrays can lead to bugs by changing state unexpectedly.
Immutable patterns avoid mutation by:
- Copying arrays before changing, using spread syntax or
slice()
- Using
.concat()
,.filter()
,.map()
etc. which return new arrays - Using libraries like Immer to simplify immutable updates
This ensures operations do not affect the original array.
Conclusion: Mastering Array Objects for Development
Arrays are a fundamental component of programming across languages. As indexed collections storing ordered data, array objects equip developers to efficiently organize, access, and manipulate critical information.
Mastering arrays is key for crafting performant applications and managing complex data relationships. Developers should prioritize learning array methods like map()
, filter()
, and reduce()
to unlock flexible and scalable data workflows.
Best practices like validating array types with Array.isArray()
and making shallow copies to prevent mutation can prevent bugs. Iteration through modern loops like for...of
improves readability.
Overall, arrays facilitate simpler state management, cleaner abstractions, reusable logic, and better testing. Dedicate time to study array theory and memorize key methods.
Arrays power functional programming techniques for transforming data flows. A deep understanding unlocks coding superpowers, enabling developers to ship robust software users love. Start leveling up array skills today!