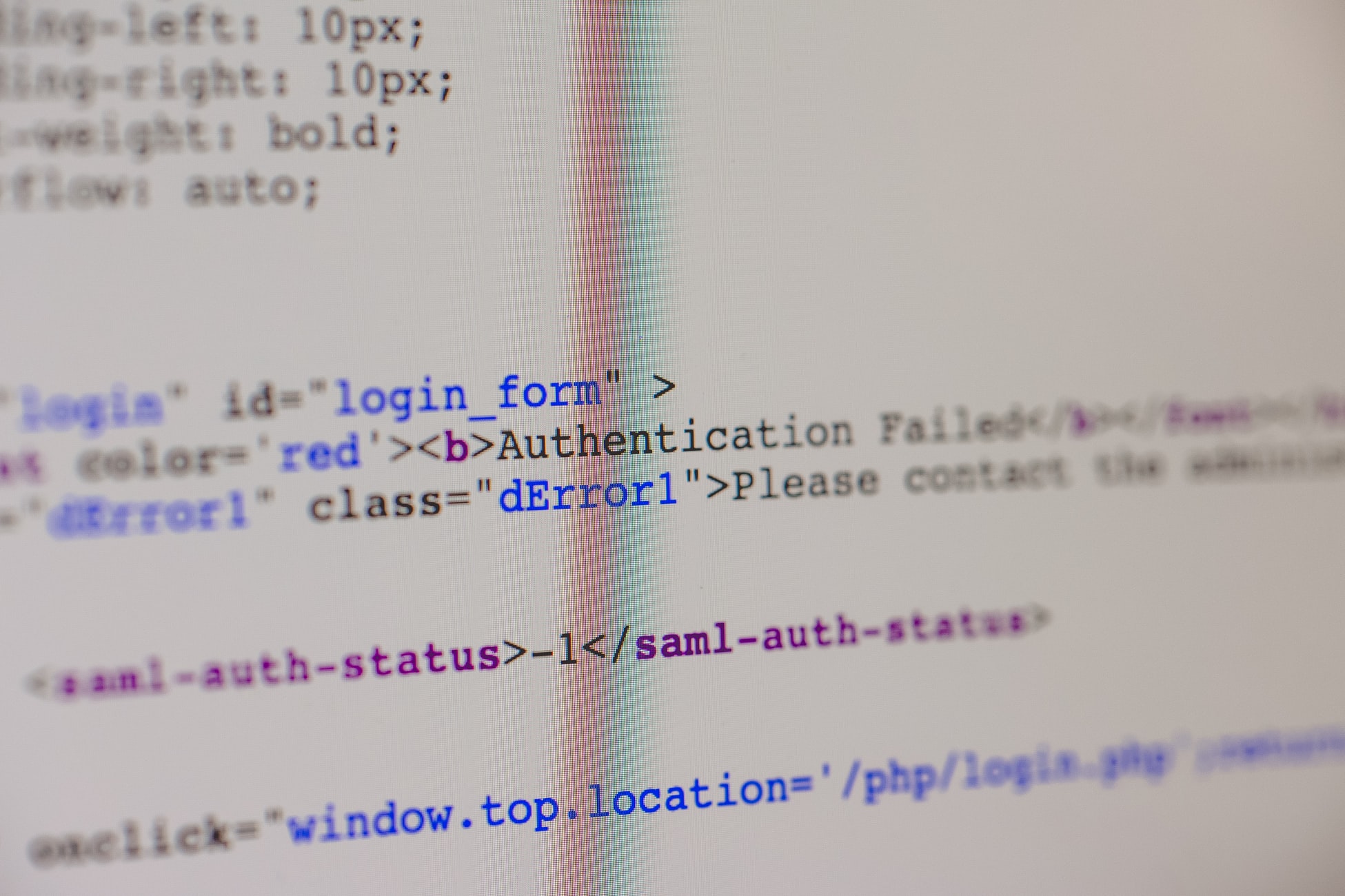
In this post, we will discuss how we can authenticate a Next.js app. Authentication is quite broad and there are different ways to achieve it, but we will talk about the most common methods.
Nextjs
We are in the era of SPAs, JavaScript web frameworks have been on the high rise lately these years. They embody the use of client-side rendering. The components and pages that make up our website are all downloaded and the web content is generated via JavaScript in the browser. This is great because page navigations are smooth and very fast without any server request. But this comes at a great cost when our application becomes large, we have to download the whole bundle and generate the UI content, which really has a great impact on the load time and performance.
Next.js was built to solve this issue. Nextjs is an open-source web framework that lets us build server-rendered and static web applications using React.
Next.js is an open-source React front-end development web framework created by Vercel that enables functionality such as server-side rendering and generating static websites for React-based web applications.
Next.js makes it possible to build a server-rendered React.js app, the content is rendered on the server before being served to the browser. This does away with the client-generated content, anyway Nextjs has an option for us to enable the client-side content generation.
Nextjs has great features that we utilize to build awesome apps:
- dynamic routing
- server rendering
- code splitting
The beautiful thing about Nextjs is that it contains both the frontend code and the server code.
Authenticating Next.js app
Authentication requires the info of a user, and then allows the user access to the application. Authentication is one of the common features of an application, this depends if we want our users authenticated by they can use our app.
We can authenticate a Nextjs app using the set of features it has. What we will do is that when a route in Nextjs is navigated to we will check the user to verify its credentials, if not successful we will redirect the user to a page, if it is successful we proceed to display the page.
In Next.js, routes are declared in the pages folder. Each page has a .js file attached to it. This file exports a component, this component is what Next.js will render when the route is navigated to.
If we have the below in the pages folder:
This will generate the routes:
localhost:3000/home This will render the home/inde.js component.
localhost:3000/home/home will render home/home/js.
The pages/user/[id].js is a dynamic route. The bracket syntax is used to mark that a route is dynamic in Next.js. This allows us to pass named parameters to the component in [id].js. The name in the bracket becomes the parameter name.
The file will map to any routes:
Now, API routes reside in the api folder in the pages folder: pages/api/. These API routes become the endpoints that can be called and Nextjs will respond to them.
Just like in pages, each folder in the api folder maps to an API route except it starts with /api/, for example, pages/api/home.js maps to localhost:3000/api/home.
We can authenticate both a page and an API route. We will see how they are done below.
SSR route protection
Like we said earlier we can protect a route in Nextjs. We want the route to be authenticated on the server and return either a success when authenticated or an error when not authenticated.
SSR routes have an authentication code that is run in the getServerSideProps function. If a getServerSideProps is exported from a page file. That route will be pre-rendered in the server, this means that the content of the page will be generated in the server before it is sent to the client.
Now, the getServerSideProps is called when the page is being pre-rendered. This function returns an object, the property in the object determines the action Nextjs will take in rendering the component. If the returned object contains a props property, Nextjs will proceed to call the corresponding page component and pass the props to the component. So, page components get their props passed to them from the getServerSideProps function. If the object contains a redirect property, this will prompt Nextjs to redirect the route to a specified destination.
Now, the getServerSideProps is a place where we can execute code on the server before a component is loaded. This becomes the ideal place to check for authentication before loading a protected page.
In the above code, we called the getAuthSession to get user session. If there is no user session we know that the user is not authenticated so we return a props object that contains authenticated property set to false. If the user is authenticated we return a props object, we set the user in a user property in the object and also we set the authenticated property set to true. We will receive these props with their values in the ProtectedSSRoute component.
In the ProtectedSSRoute component, we check if the user is authenticated by checking for the authenticated props, then we display the views accordingly.
Server-side redirects
Server-side redirection entails redirecting a current page navigation to another page. Suppose a request for the page at /page/profile is made, this can be re-directed from the server to load page/login instead.
One use cases of server-side redirection is in authentication. When a protected page is accessed by a non-authenticated user, the user is redirected to a login page before the protected hit the browser. It is common in websites these days.
Server-side redirection is an authentication pattern and it is also available in Nextjs. Like we have stated in the above sections, code can be run in the server when a request for a page is made in Nextjs. This is where we can check for user authentication and redirect the user if necessary to another page using the redirect property.
Let’s see an example below:
In the above code, the ProtectedSSRoute component will receive the user information in its props. The getServerSideProps function is defined and also being exported so this page route will be pre-rendered. Inside the getServerSideProps, we call the getAuthSession function to get the user session. If there is no session then we know the user is not authenticated so we return an object that contains a redirect object. This object contains a destination property and a permanent property. This will prompt Nextjs to redirect the route to "login" route.
If the user is authenticated, the returned object will contain a props object, which in turn contains a user with the user value.
This merely demonstrates how we can authenticate a page route in Nextjs and redirect the route from the server if the user is not authenticated. The getServerSideProps made this possible, the authentication and redirection will be done without hitting the browser.
Client-side redirects
Client-side authentication is carried out in the browser. The page component is loaded in the browser when its page route is navigated to. The page component bears the authentication logic that will be executed in the browser unlike authentication in getServerSideProps and getStaticProps that will be execute on the server before the component is loaded.
The user’s authentication details is requested and the component’s UI is not displayed while the details are being fetched. A loading indicator is usually shown to tell the user that some background activity is going on. If the user’s authentication fails then, the user is redirected to a login page. This is usually done with the useRouter hook. If the user’s authentication succeeds the component’s UI is rendered.
Let's see the below component:
The useAuthSession hook is called to get the user session. If there is no user session, the "login" page is loaded. The useSWR data-fetching hook, we used it to fetch the user session from the api/session API, if no user is returned then the Login page is loaded, if a user is found the protected page displays the user.
You see, the authentication check is done from the client-side and the redirection, if the authentication fails is also done in the client.
API protection
What we have learned so far are authentication patterns on page routes. Authentication can also be done on Nextjs API routes.
We may decide that some API routes in our Nextjs need to be only accessed by authenticated users. API routes protection is done similarly with page authentication. We check for the user session data, if found and still valid we let the request to the API route continue, if not we terminate it abruptly and send a "Non authenticated user" error back as the response.
Remember that API routes are in the pages/api folder. An API file for e.g. pages/api/home.js exports an async function:
This function will be executed when the endpoint api/home is called via HTTP.
The req is the incoming message object, an instance of http.IncomingMessage. It holds details about the request to the API, for example, the URL, the HTTP method, the params, body, etc.
The res is the response message object, an instance of http.ServerResponse contains functions to help us send back a response to the user.
So to protect the API endpoint we do this:
See here, this API endpoint returns home data to the user, but since it is a protected endpoint, we first check for the user session. If there is no session found or it is invalid, we return a "Non-authenticated user." message to the user with a 403 status.
If we find a valid user session we proceed to get the home data and send it to the user.
So, that's an API route authentication in Nextjs nicely done.
Conclusion
We learned about different authentication patterns in Nextjs, we started by introducing Nextjs and went on to learn about implementing authentication in a Nextjs app.
We saw how to protect a page from the server-side, and how we can protect a page and also redirect un-authenticated both on the server-side and on the client-side. Lastly, we learned how we can authenticate an API route.
Several authentication providers can help us add a layer of security to our protected routes: