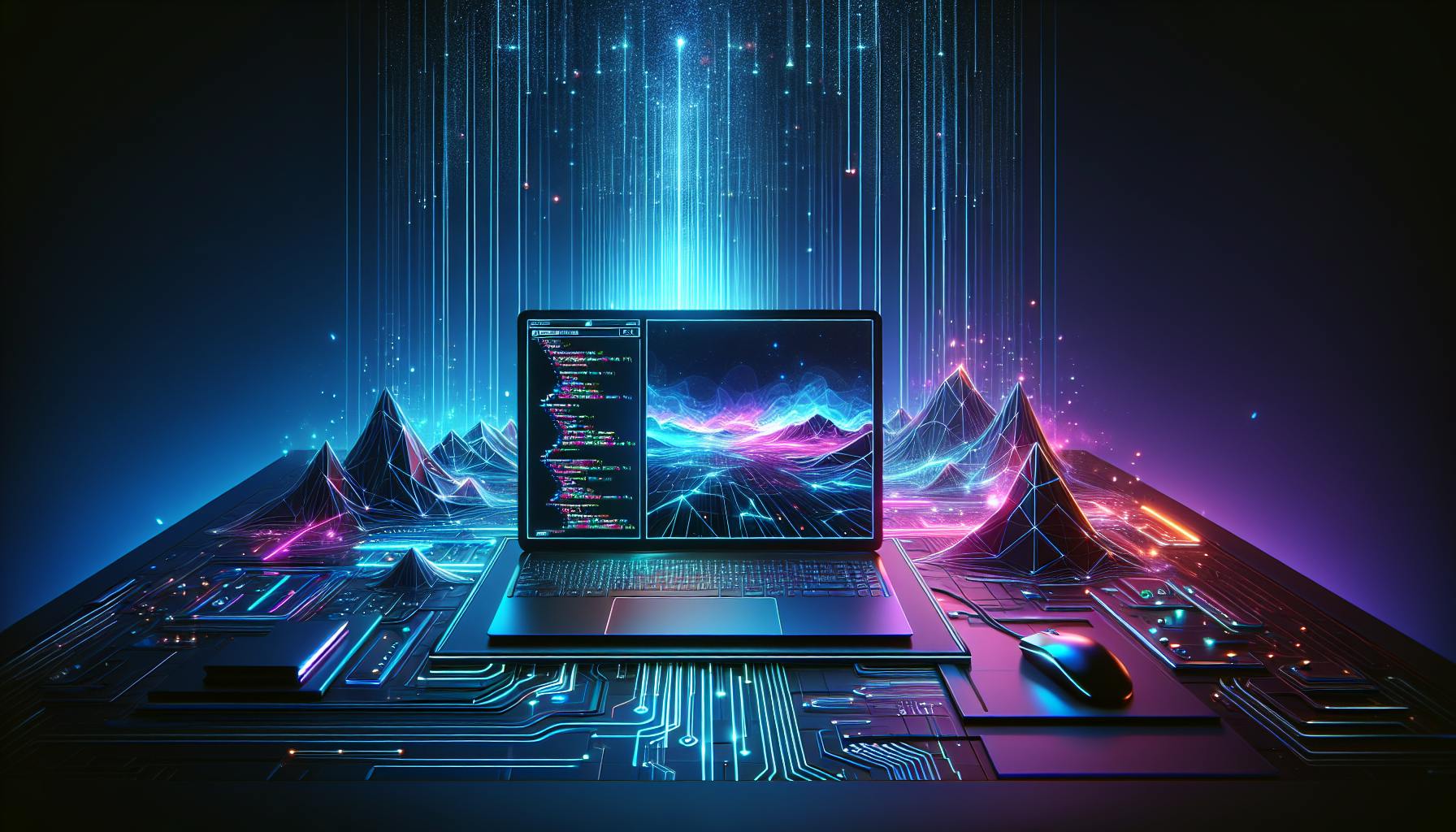
Learn the basics of Bootstrap CSS for developers, including key benefits, core concepts, setup process, and customization options. Get started with HTML, CSS, and JavaScript to enhance your web development skills.
Bootstrap CSS is a toolkit for developers to create responsive and mobile-first websites easily. Here's a quick overview:
- Bootstrap is free and open-source, offering ready-made components and utilities that adjust to different screen sizes.
- Key benefits include ease of designing responsive sites, a variety of pre-made designs, and customization options through Sass.
- Prerequisites involve basic knowledge of HTML, CSS, and a bit of JavaScript.
- Core concepts cover the grid system for layout design, responsive features for mobile compatibility, and ready-to-use components for UI elements.
- Getting started with Bootstrap involves downloading it, using a CDN, or installing it via NPM, followed by incorporating its CSS and JS files into your project.
- Customizing Bootstrap can be done by overriding default styles, utilizing Sass for deeper customizations, or using its jQuery plugins for added interactivity.
This guide aims to equip you with the basics of Bootstrap CSS, highlighting its benefits, setup process, core concepts, and customization options.
HTML
HTML is the backbone of any website. You need to know about:
- Basic stuff like titles, paragraphs, lists, and how to make forms.
- Special tags that help organize your site, like ones for headers, navigation, sections, and footers.
- How to use class and id attributes to style things.
- Setting up your page with divs and spans to keep everything in order.
Understanding HTML helps you set up the content that Bootstrap will make look good.
CSS
To get the most out of Bootstrap, you need to know some CSS, which is what makes websites look nice. You should understand:
- How to pick out parts of your page and make them look a certain way.
- Basics like colors, fonts, space around things, and how to lay things out.
- The rules of how styles are applied and how to make your styles win when there's a conflict.
- How to make your site look good on phones and tablets, not just computers.
Knowing CSS means you can tweak Bootstrap's styles to fit your site perfectly.
JavaScript
While you can use Bootstrap without being a JavaScript whiz, knowing a bit about it helps a lot. This includes:
- How to find and change parts of your page with code.
- Making things happen when users click, hover, or fill out forms.
- How to write simple functions.
- Understanding basic coding stuff like variables.
A lot of Bootstrap's cool features need JavaScript, so knowing the basics lets you use everything it offers.
With a good grasp of HTML, CSS, and a bit of JavaScript, you'll be ready to use Bootstrap to make your website look awesome. The guides and documentation expect you to know these things, so they're important to have under your belt.
Getting Started with Bootstrap
What is Bootstrap?
Bootstrap is a tool that helps you make websites and web apps that look good on any device. It's like a big box of building blocks for the web. Here's what you should know:
- It was made by some folks at Twitter and shared with the world in 2011.
- Lots of big and small sites use it because it's pretty handy.
- It's made with HTML, CSS, and JavaScript.
- It includes a bunch of ready-made pieces like buttons and menus that automatically adjust to screen size thanks to its grid system.
- You can change how it looks with some coding skills using Sass.
- It's free to grab and use for your projects.
Basically, Bootstrap makes it easier and faster to create websites that work well on phones, tablets, and computers.
Why Use Bootstrap?
Using Bootstrap has its perks:
- It saves time - You don't have to start from scratch when you want to add common elements like buttons or menus. Bootstrap has them ready to use.
- Mobile-friendly - Its design adjusts to fit screens of any size, so your site will look great on any device.
- Consistent look - It helps keep the design of your site looking uniform.
- You can tweak it - With some coding, you can change the colors, sizes, and more to fit your style.
- Lots of people use it - This means it's easy to find help online, and it gets regular updates.
For making websites quickly, Bootstrap is a great tool because it cuts down on the amount of code you have to write yourself.
Installing Bootstrap
There are a couple of ways to start using Bootstrap:
- Download it - Grab the pre-made CSS and JavaScript from the Bootstrap website and add it to your project. This is great if you want everything stored on your computer.
- Use a CDN - This is a link you add to your site that loads Bootstrap from the internet. It's quick and easy, but you need an internet connection for it to work.
- NPM package - If you're using Node.js, you can install Bootstrap as a dependency, which is handy for more complex projects.
The best way to start depends on your project. For quick tests, using a CDN is super fast. For bigger projects, downloading Bootstrap gives you more control.
Here's how to set it up:
- Visit getbootstrap.com and hit "Download".
- Put the Bootstrap files into your project folder.
- Link the CSS file in your webpage's
<head>
and the JavaScript file right before the</body>
tag. - Look at Bootstrap's website for examples on how to use its components.
And that's it! You're now ready to use Bootstrap to make your website.
Core Concepts of Bootstrap
Bootstrap gives developers the tools they need to make websites that work well on phones and computers without a lot of hassle. Let's break down some of the main ideas you need to know to get the most out of Bootstrap.
Grid System
The grid system is a way to divide your webpage into 12 flexible columns. This makes it super easy to arrange your content in a neat and responsive way.
Here's what you need to know:
- Containers help keep your content centered and neat.
- Rows group columns together and stop content from spilling out.
- Bootstrap has 5 sizes (or breakpoints) for different devices: xs, sm, md, lg, xl.
- You can control column width, like making a column take up 4 spaces on a small screen with
.col-sm-4
. - This system is great for making your site look good on any device.
Responsive Design
Bootstrap uses special rules (media queries) and helper classes to make your site adjust:
- Content can resize or move around on the page automatically.
- You can show or hide elements depending on the screen size.
- Adjust things like spacing and font size easily.
- Use responsive containers to keep everything looking right.
This means your site will look great, no matter what device someone is using.
Bootstrap Components
Bootstrap comes with a bunch of ready-made parts, like:
- Nav bars
- Buttons and forms
- Tables and lists
- Alerts and status bars
- Cards and small info boxes
- Slideshows
These components are already designed to look good and work well on any device, which saves you a lot of time. You can also change them to fit your style better.
Building a Simple Webpage with Bootstrap
Bootstrap is like a toolkit for building websites that look great on phones, tablets, and computers without a lot of fuss. It comes with a bunch of shortcuts and pre-made parts that save you time. Let's go through how to make a simple webpage using Bootstrap.
Set Up the HTML Template
First, we need a basic webpage setup that includes Bootstrap's style and script files. Here's a simple template:
<!doctype html>
<html lang="en">
<head>
<!-- Important bits for mobile and Bootstrap -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<!-- Bootstrap's CSS -->
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css" crossorigin="anonymous">
<title>My Bootstrap Site</title>
</head>
<body>
<!-- Where our page content will go -->
<!-- Bootstrap's JavaScript -->
<script src="https://code.jquery.com/jquery-3.5.1.slim.min.js" crossorigin="anonymous"></script>
<script src="https://cdn.jsdelivr.net/npm/popper.js@1.16.0/dist/umd/popper.min.js" crossorigin="anonymous"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/js/bootstrap.min.js" crossorigin="anonymous"></script>
</body>
</html>
This code sets up a basic page and includes Bootstrap so we can start using it.
Add a Navigation Bar
Next, we can add a navigation bar at the top of our page using Bootstrap's navbar
component:
<nav class="navbar navbar-expand-lg navbar-light bg-light">
<a class="navbar-brand" href="#">My Site</a>
<button class="navbar-toggler" type="button">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse">
<ul class="navbar-nav mr-auto">
<li class="nav-item active">
<a class="nav-link" href="#">Home</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">About</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Contact</a>
</li>
</ul>
</div>
</nav>
This code makes a responsive header with a menu that works on mobile devices too.
Structure Page Layout
Finally, let's organize the main part of our page using Bootstrap's grid system. Here's how you might set up a section with a main area and a sidebar:
<main class="container">
<header class="jumbotron">
<h1>Welcome!</h1>
<p>This is my page built with Bootstrap.</p>
</header>
<section class="row">
<div class="col-md-8">
<!-- Place for blog posts -->
</div>
<aside class="col-md-4">
<!-- Sidebar content goes here -->
</aside>
</section>
This setup uses Bootstrap's grid to create a main content area and a sidebar, making sure everything looks good on any screen size.
sbb-itb-bfaad5b
Customizing and Extending Bootstrap
Override Default Styles
To change how Bootstrap looks, you can add your own CSS file after Bootstrap's. Just target the parts you want to change. For instance:
.navbar {
background-color: #333;
}
.btn-primary {
color: #fff;
background-color: #007bff;
border-color: #007bff;
}
This way, you can tweak colors, fonts, and sizes but keep Bootstrap's layout working smoothly.
Utilize Sass Source Files
If you want to dive deeper into customizing Bootstrap, use its Sass files. This means you can adjust things like colors or fonts before you even start your project. For example:
$body-bg: #333;
$primary: #007bff;
When you compile these files with your changes, Bootstrap will match your unique style.
Bootstrap Theming
Bootstrap lets you make your own themes using Sass. You can set your own color schemes like this:
$theme-colors: (
"primary": #333
);
This changes the main color to #333. It's a powerful way to make Bootstrap fit your style.
jQuery Plugins
Bootstrap uses jQuery for extra features like pop-ups or sliders. For example:
$('#myModal').modal(options)
This code makes a pop-up window work. Bootstrap has lots of these plugins to make your site more interactive.
Conclusion
Bootstrap is a super handy tool for making websites quickly that look good on any screen, like phones or computers. If you know the basics of HTML, CSS, and a little JavaScript, you can start using Bootstrap to make your work easier.
Key Takeaways
- Bootstrap saves you a lot of time because you don't have to write much CSS to make your site look good and work on mobile.
- It has things like the grid system and ready-to-use components that make putting together websites really easy.
- If you know how to use Sass and jQuery, you can change how Bootstrap looks and add cool features to your site.
- Learning Bootstrap takes some time, but it's worth it because it makes building websites faster and helps avoid problems with how your site looks in different browsers.
In short, Bootstrap makes web development faster and easier. It lets you focus on making your site awesome instead of worrying about layout and design problems. Keep exploring Bootstrap's guides and community tips to get even better at using it. The skills you learn will help you a lot in making all kinds of web projects.
Related Questions
Do I need to learn CSS if I know Bootstrap?
Yes, knowing CSS is important even if you use Bootstrap. Bootstrap uses CSS to make web development faster by offering ready-made designs. But to use Bootstrap well, you need to understand the basics of CSS first.
Can you use CSS and Bootstrap together?
Absolutely, you can mix your own CSS with Bootstrap. It’s like adding your personal touch to the Bootstrap foundation. Just make sure your custom CSS comes after Bootstrap’s in the webpage code, so your styles apply correctly.
Do you need to write CSS with Bootstrap?
Even with Bootstrap, you’ll find yourself writing some CSS. It’s essential for tweaking the designs to fit your project perfectly. Knowing things like the box model helps when you’re setting up layouts with Bootstrap’s grid.
What is Bootstrap CSS in programming?
Bootstrap is a toolkit that helps make web development easier. It offers a bunch of design templates for common web elements like forms and buttons, using HTML and CSS. It also has optional JavaScript features. Using Bootstrap can speed up making websites look good.