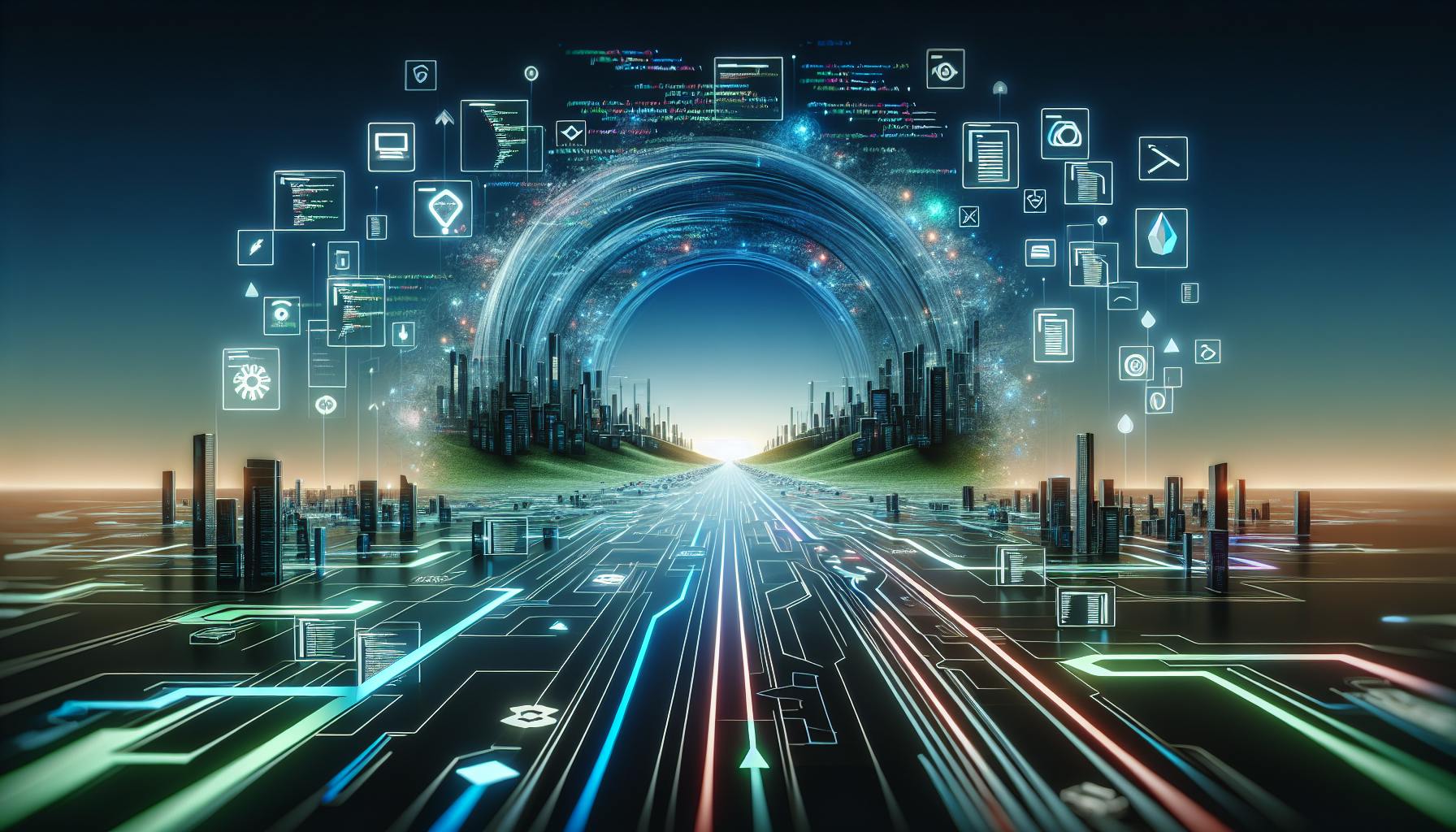
Learn about client-side rendering (CSR) in Angular, its key features, performance, SEO considerations, and optimizations. Explore Angular framework, architecture, SSR vs. CSR, and optimization strategies.
Client-side rendering (CSR) in Angular is all about making your web pages dynamic and fast, directly in your browser. Here's what you need to know:
- Angular and CSR: Angular excels at CSR, creating interactive pages that update without full page reloads.
- Key Features: Includes components, directives, services, dependency injection, and change detection for seamless updates.
- Performance: CSR might start slower but offers a more app-like, responsive experience afterward.
- SEO and Loading: Initial load times and SEO might need extra attention with CSR.
- Optimizations: Techniques like lazy loading, compression, and server-side rendering (SSR) integration can enhance performance.
In simple terms, Angular uses components and directives to dynamically update your webpage in the browser, making things faster and more interactive after the initial load. While there are challenges like SEO and the first page load speed, with the right strategies, such as optimization and possibly mixing in some SSR, you can create fast, SEO-friendly Angular apps.
Understanding Angular Framework and Architecture
Angular is a popular tool for making websites that can change and update without having to reload the page. It's all about doing the heavy lifting on the user's browser instead of on a server far away. Let's break down how Angular makes this possible and what's under its hood.
Exploring Core Capabilities
Angular has some key features that help with updating websites on the fly:
- Components - These are like LEGO blocks for your website, letting you reuse parts of the interface easily.
- Directives - Think of these as magic spells that can change how things look or act in your website without needing new HTML.
- Services - These are helpers that do specific jobs, like getting data, so you don't have to write the same code over and over.
- Dependency Injection - This is a fancy way of giving your components what they need to work, making them easy to swap out or update.
- Change Detection - Angular keeps an eye on what data has changed and automatically updates the website to match.
- Routing - This lets users move around different parts of your website without the whole page needing to reload.
These features let Angular handle updates and changes right in the browser, making websites feel quick and responsive.
Architecture and Project Anatomy
An Angular project is set up in a specific way, with important files and folders:
- package.json - Lists all the tools and packages your project needs.
- angular.json - The main settings file for your project.
- main.ts - The starting point that gets your app going.
- index.html - The basic HTML page that the browser loads first.
- Components - The parts of your site, like headers, footers, and buttons.
- Services - The bits that do specific jobs, like fetching data.
- Modules - These help organize your code, especially for routing and grouping related parts together.
All these pieces work together so that when someone uses your site, Angular can update pages and move between them smoothly without having to ask the server for a whole new page each time. This is what makes Angular great for building fast, modern web apps.
Client-Side Rendering vs. Server-Side Rendering
Performance and User Experience
When a website uses client-side rendering, it means the browser does the work of showing the website. This is great for making websites that update quickly and let you move around smoothly without waiting for pages to reload. But, the first time you visit the site, it might take a bit longer to load everything.
Server-side rendering, on the other hand, does most of the work on the server. This can make the first page you visit load faster because it's already prepared. But if you go to other pages, it might feel slower because it needs to load each new page fully.
So, in simple terms:
- CSR: Might start slow, but then it gets quicker and feels more like an app.
- SSR: Quick start, but moving around the site might be slower. Less smooth.
SEO and Initial Page Load Times
With client-side rendering, there used to be worries about search engines not finding the site easily. But now, search engines are better at understanding these sites. Still, server-side rendering might have an edge for being noticed by search engines right away.
Also, server-side rendering can make the first page you visit load really fast because it's ready to go. But, client-side rendering can also be fast at the start if it's set up well.
So, when deciding what to use:
- CSR can be okay for search engines but might need some extra work. It can be quick at the start if you use smart tricks.
- SSR is great for getting noticed by search engines and for fast loading at the beginning.
Both ways can be made to feel faster with some smart setup, like breaking up the code, squishing it down, and remembering parts of the site so they load quicker next time. The best choice depends on what you want your website to do and who will be using it.
Angular's Client-Side Rendering Process
Angular makes web apps that update without making you wait for the page to reload. Let's break down how Angular does this:
Building Blocks
Angular has some main parts that help it show things on your screen without having to ask the server every time:
- Components - Think of these as the pieces of a puzzle. They're parts of the webpage that Angular can show and update on its own.
- Directives - These are like instructions that tell the webpage how to change or behave.
- Data Binding - This keeps the information on the page fresh without manual updates.
- Services - These are like helpers for doing specific jobs, like getting data, so everything doesn't get too cluttered.
- Dependency Injection - This is a way for Angular to give components the tools they need.
All these parts work together so Angular can make updates directly in your browser.
Lifecycle Hooks
Angular uses special moments called lifecycle hooks to manage what's happening on the page:
- ngOnInit - This is when Angular sets up a component. It's a good time to grab any data you need.
- ngOnDestroy - This happens right before Angular removes a component from the page.
- ngOnChanges - This is for when the information that a component uses changes.
By using these hooks, developers can make sure the right things happen at the right time, like grabbing data or cleaning up. This helps keep the webpage feeling quick and responsive.
In short, Angular uses a mix of components, instructions, and special moments to make web pages that can change on the fly, without having to load everything again. This makes for a smoother experience as you're using the site.
sbb-itb-bfaad5b
Configuring and Optimizing Angular for CSR
Project Setup and Tooling
When you start an Angular project, setting it up right is key for making things run smoothly. Here's what to do:
- Use Angular CLI to create your project. This tool sets things up with good defaults, like making sure your code is ready to run fast.
- Make sure your tools for building the app, like Webpack, are set to make your code as small and fast as possible. This means getting rid of code you don't use and making files smaller so they load quickly.
- Get your testing setup ready early with tools like Karma and Jasmine. This helps you catch problems before they get big.
- When you're ready to share your app with the world, turn on the settings that make your app run faster and turn off anything meant for checking your code.
Keeping your code neat and using Angular the way it's meant to be used will help avoid slow-downs.
Lazy Loading and Other Optimization Strategies
Here are some ways to make your Angular app run faster:
- Lazy load - This means only loading parts of your app when they're needed, which helps your app start faster.
- Ahead-of-Time Compilation - This gets your app ready to run faster by doing some work ahead of time.
- Compression - Make your app's files smaller so they can be downloaded quicker.
- Use trackBy - This helps Angular keep track of items in a list, so it doesn't have to redo work.
- Optimize Change Detection - Change how Angular checks for updates to make it faster.
- Virtual Scrolling - This only shows items in a list that you can see, making scrolling smoother.
- Caching - Save some data so your app doesn't have to ask for it again, making things load faster.
- SSR - For really important content, you can make it ready on the server so users see it right away.
Keep looking for ways to make your app faster, like checking if you're using too much code and finding parts that are slow.
Conclusion
Getting the Hang of Client Side Rendering in Angular
Client side rendering (CSR) is a big deal when you're building websites or apps with Angular. It's about making pages come to life in your browser, which makes things feel quick and smooth.
Here's a quick review of what we talked about with CSR in Angular:
- Angular is really into client side rendering, which means it builds pages using pieces called components and templates.
- The good stuff includes faster updates without waiting for the server, and keeping the work of making pages and the server stuff separate.
- The not-so-good includes slower first-time page loads and a bit of a headache for getting noticed by Google unless you put in extra effort.
- Important bits to know are components, directives (which are like instructions for your page), data binding (keeping info up-to-date), services (helpers for tasks), and dependency injection (a fancy way of giving components what they need).
- Special moments in the process, like
ngOnInit
, let you do things at just the right time. - Making things run faster can involve lazy loading (loading parts only when needed), making your code ready ahead of time, squishing your files to make them smaller, and a few other tricks.
- Sometimes, mixing CSR with server-side rendering (SSR) can give you the best of both worlds for certain pages.
To get really good at CSR:
- Understand how Angular gets from asking for a page to showing it to you, including those special moments.
- Set up your apps to run as well as possible, starting with the tools Angular gives you.
- Use smart ways to speed things up, like remembering parts of your page so they load faster next time.
- Think about using SSR for parts of your site that need to be super quick or easy for Google to find.
Getting client side rendering right is great for making your site or app fast and easy to use. Knowing how Angular handles making pages can help you build better projects.
As Angular gets better, so will the way it does CSR. We've just touched on the basics here - there's always more to learn and try out!
Related Questions
How does client-side rendering work in Angular?
In simple terms, Angular apps run in your web browser. They load pages when you do something, like click a link. If you need pages to load before you open the app, you use something called Angular Universal.
What is client-side rendering for dummies?
Think of Client-Side Rendering (CSR) like this:
- Initial Request: You go to a website by typing its address or clicking a link. Your browser asks the website's server to send the content.
- Minimal Data: The server sends back a simple HTML page and some JavaScript files. This HTML doesn't show much yet; it's just the groundwork.
How does SSR work in Angular?
Server-Side Rendering (SSR) mixes the best parts of making pages load in your browser (dynamic and interactive) with the quick load times and SEO perks of having pages ready on the server.
How does rendering work in Angular?
Here's a simple breakdown of Server-side rendering (SSR) in Angular:
- Server Renders Pages: Pages are made ready on the server, so you get the full page with all its content right away.
- Browser Takes Over: After the page loads in your browser, Angular starts up the app using the page's existing content.