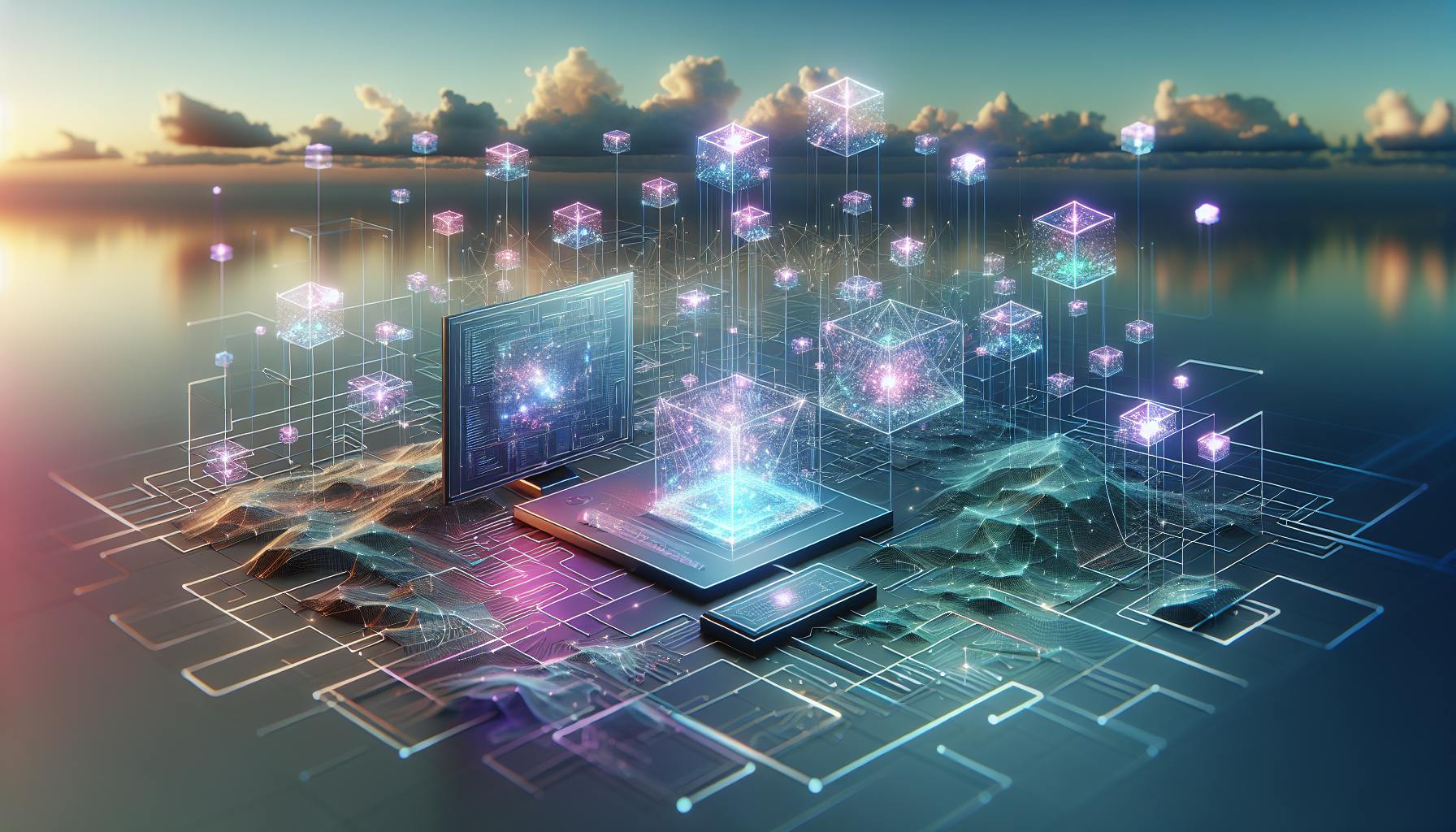
Explore 5 engaging coding challenges that cater to all levels, from beginners to experienced programmers. Sharpen your coding skills and have fun with these puzzles!
Looking to have fun while coding? Dive into these 5 engaging coding challenges that cater to all levels, from beginners to more experienced programmers. These problems not only sharpen your coding skills but also let you enjoy the process. Here's a quick peek:
- The Magic Equation Challenge: Discover patterns in number series.
- Self-Replicating Program: Create a program that shows its own code.
- Perfect Tic-Tac-Toe: Develop an unbeatable tic-tac-toe AI.
- Topological Sort Puzzle: Sort tasks based on their dependencies.
- The Nim Game Script: Code a strategy game with an unbeatable AI.
These challenges cover a range of topics, including pattern recognition, creating self-replicating code, game development, sorting algorithms, and strategic thinking. Whether you're preparing for job interviews, learning new programming languages, or just looking for a fun coding experience, these problems offer a great way to learn, think critically, and connect with other programmers.
List of Fun Coding Challenges
1. The Magic Equation Challenge
This challenge is about finding a pattern in a series of numbers. Your job is to write a simple program that can figure out what number comes next in the series.
For example, take a look at this series:
2, 4, 8, 16, 32, 64, 128...
The pattern here is pretty straightforward. Each number is just the one before it multiplied by 2. So, if your program gets 128, it should say the next number is 256.
This challenge helps you learn about:
- Spotting patterns
- Basic math in programming
- Using loops (to create the series)
- Writing functions (to keep your code neat)
You could make this more interesting by trying out different series, like the Fibonacci sequence or factorials. The main thing is to figure out the basic rule or formula that makes the series work.
This is a good starting point for beginners because it's about turning patterns you see in the world into code. You could even add a feature where users can make their own series. Try it out and see what cool patterns you can come up with!
2. Self-Replicating Program
Imagine writing a program that can create a copy of itself! This challenge is all about making a simple program that, when run, shows its own code.
Here's why it's a cool puzzle:
- It makes you think about how a program can look at and use its own code.
- It's a mix of being creative and logical at the same time - you need to figure out a smart way to show the code.
- When it works, it's like you've made a very basic form of life that can copy itself!
You can try different methods:
- Open and read the program file: Just open the file your code is in and show what's inside.
-
Use a string variable: Create a variable like
myCode
and set it to a string that is your code, then showmyCode
. - Put the code in the print command: Make a print command that includes special codes to show the quotes and code characters.
The main things to get right are making sure the code looks right with spaces, special characters for quotes, and making sure the code that gets shown can run and make a copy again.
It's a challenge many programmers try, but it's always fun to do it yourself. Getting your program to copy itself feels awesome!
This challenge is good for learning about files, text, variables, special text characters, and showing text - which is great for beginners. But even if you're more experienced, you'll like the thinking and creativity part. Give it a go!
3. Perfect Tic-Tac-Toe
Everyone knows tic-tac-toe, right? But imagine creating a computer program that can play tic-tac-toe so well, it never loses. This challenge is not just fun but also a cool way to sharpen your problem-solving skills!
Why this is a neat project:
- You already know how to play tic-tac-toe, which means you understand what your program needs to do.
- Even though the game is simple, figuring out how to make a computer win or tie every time can be tricky.
- When you get it right, it's super satisfying!
To build this unbeatable tic-tac-toe program, consider:
- How to show the game board and moves in code. Using a list or a grid works well for this.
- Identifying all the ways to win the game and how your program can spot them.
- Thinking ahead about the player's possible moves and how your program can block or beat them.
- Creating a set of rules for your program to follow so it always ends up winning or tying.
You'll learn about:
- Using lists or grids to set up game spaces.
- Going through the game board to check for wins.
- Algorithms like Minimax that help your program think ahead about future moves.
- Writing code that keeps an eye out for what the opponent might do next.
Making a tic-tac-toe game that can't be beaten is a fun way to dive into some smart coding strategies. The trick is to tackle it one step at a time, thinking about all the possible moves and outcomes. Nail it, and you'll feel like a coding genius!
4. Topological Sort Puzzle
This challenge is all about organizing things in a straight line, especially when you have a bunch of tasks that depend on each other. Imagine you have tasks A, B, and C, where A needs to happen before B, and B before C. Your job is to arrange them from start to finish without messing up the order.
Here's a simple example:
A -> B
B -> C
A -> C
A good way to line them up would be:
A, B, C
Because A comes first, then B, and C last.
Why this is a cool challenge:
- It's like solving a puzzle where you have to figure out the right order of things.
- You get to learn about how to organize tasks and the rules for ordering them.
- It's satisfying to get everything in the right order.
How to tackle it:
- Think of the tasks as points on a graph and the dependencies (which comes before which) as arrows between them.
- Use a method to mark tasks as done as you go through them.
- List the tasks in the order you finished them.
Things to watch out for:
- Loops in the tasks where there's no clear start or end.
- Deciding which task to do next when you have options.
What you'll learn:
- How to use graphs to solve problems.
- The basics of sorting tasks in an order.
- How to deal with tricky situations where the order isn't clear.
This topological sort challenge is great for both new and experienced coders. It's a fun way to learn about organizing tasks and dealing with complex problems. Plus, it's a skill that's super useful in real life, like when scheduling projects or analyzing financial data.
5. The Nim Game Script
The Nim game is a classic brain teaser perfect for beginners. Here's the gist of it:
Two players face off with several piles of objects (like sticks or coins) between them. Each turn, a player can remove as many objects as they wish, but only from one pile. The one who has to take the last object loses.
This challenge is cool because:
- You can easily picture the game and use simple arrays to code it.
- Figuring out the strategy to always win involves some neat math logic.
- You get to create an AI player that can't be beaten!
Here's how to start coding it:
- Represent the piles with arrays.
- Create a function that lets players remove objects from a pile.
- Develop an AI function that uses the winning strategy for Nim.
- Switch turns between the player and the AI.
- Check if any objects are left after each turn. If not, the game ends!
What's great about this project:
- You'll practice using arrays and changing their values.
- You'll learn how to make functions that handle different parts of the game.
- You'll challenge yourself by programming an AI that always knows the best move.
- You'll work on managing turns between two players.
Coding the Nim game is a fantastic way to dip your toes into game development and AI. It's not just about making a game; it's about creating a smart opponent that follows a clear strategy to win. Try it out, and enjoy crafting a game where strategy is key!
sbb-itb-bfaad5b
Conclusion
These five coding challenges are a great way for coders at all levels to get better and have fun at the same time. By working on these puzzles, whether on your own or with others, you can:
- Get better at the basics: Challenges like the Magic Equation help you practice spotting patterns, doing math, using loops, and writing functions.
- Think outside the box: Trying to make a program that can copy itself pushes you to think in new ways about how code works.
- Use logic: Building a Tic-Tac-Toe game that never loses makes you think hard about what moves to make and when.
- Keep things in order: Sorting tasks with the Topological sort challenge teaches you how to line things up in a way that makes sense, using graphs.
- Try new things: Making a Nim game gets you into game theory and how to program a smart computer opponent.
Coding for fun also lets you meet other people who like coding. You can work together, help each other out, and make new friends. It's a great way to share ideas, get advice, and build connections.
In the end, these coding challenges are about learning more and getting better in a fun, friendly way. Whether you're getting ready for job interviews, wanting to learn new programming languages, or just coding for the joy of it, these challenges have something for everyone. It's all about exploring, being creative, and making friends along the way.
Related Questions
What is fun about coding?
Coding is like solving a puzzle. It feels great to see your ideas work out right in front of you. The fun parts about coding include:
- Seeing your creations work
- Figuring out solutions to tricky problems
- Being creative and making new things
- Working with others on cool projects
How do I start coding for fun?
If you're new and want to enjoy coding, try these steps:
- Learn the basics well
- Play coding games and tackle fun challenges
- Find someone experienced who can help and encourage you
- Don't forget to take breaks to keep your mind fresh
- Ask for feedback to get better
- Set small goals for yourself and celebrate when you reach them
The most important thing is to have fun and not put too much pressure on yourself.
What are some of the difficulties with codes?
Coding can be tough sometimes. Here are a few challenges you might face:
- Figuring out where to start
- Understanding complex ideas
- Finding and fixing mistakes in your code
- Doubting your skills
- Staying motivated when things get tough
- Keeping up with new tools and languages
The best way to deal with these challenges is to be patient, ask for help, and believe in yourself. Tackle one small piece at a time.
Why are coding problems so hard?
Coding is about telling computers exactly what to do, which is different from how we usually communicate. It can be hard because:
- There are strict rules for writing code
- It requires a lot of abstract thinking
- You have to keep track of many details
- Finding and fixing errors can be tricky
- You need to use math and algorithms in smart ways
But, with time and effort, you can get better and start solving more complex problems. Stay curious and enjoy the journey.