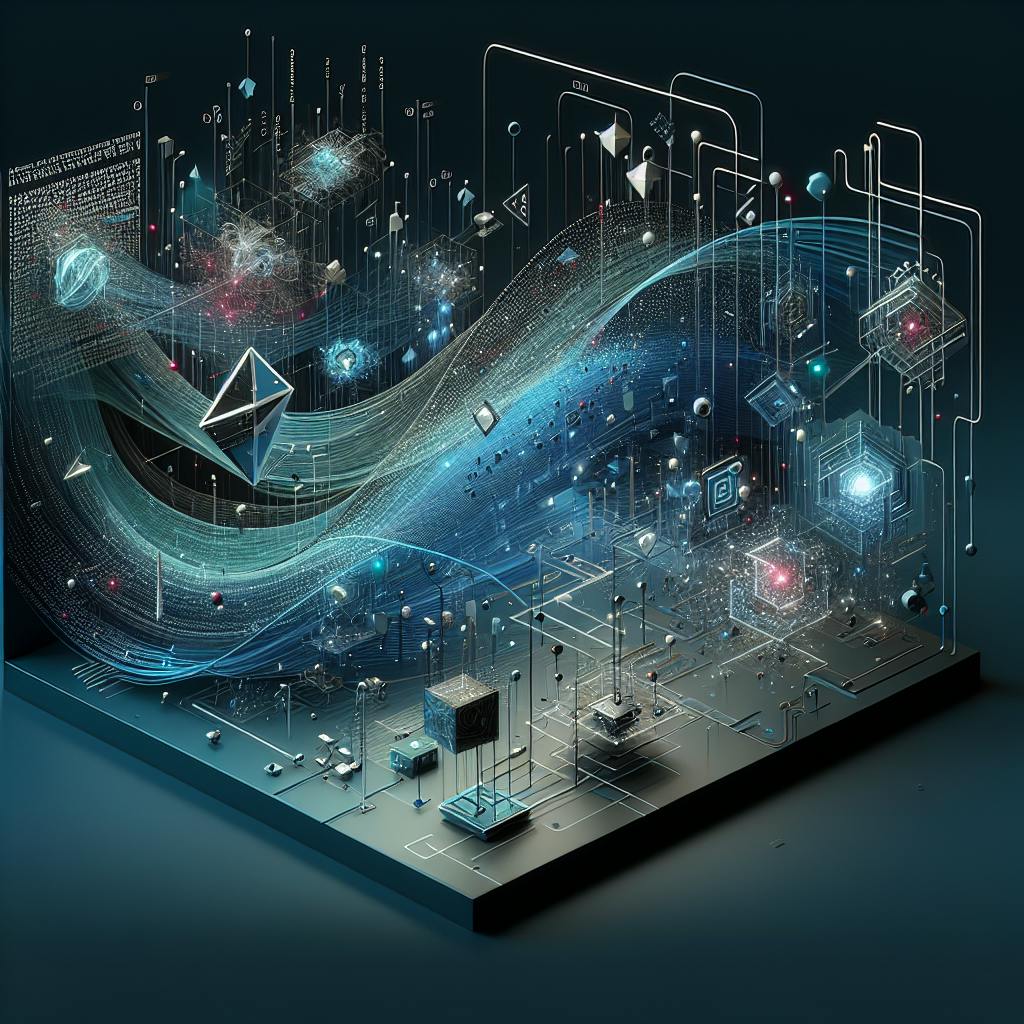
Explore the complex world of networked physics in multiplayer games, tackling challenges like latency, collisions, and syncing issues.
Networked physics in multiplayer games is tough. Here's what you need to know:
- It's about making physics work smoothly across devices and servers
- Main challenges: latency, sync issues, collisions, and scaling
- Most game engines aren't built for this
Key points:
- Start with networked physics from day one
- Use client prediction and server checks
- Balance server and client-side handling
- Implement lag compensation techniques
- Optimize data transfer and compression
Quick tips:
- Use fixed timesteps for consistency
- Stick to simple collision shapes
- Let the server make final physics decisions
- Test often on different devices
Remember: Networked physics is complex. Keep learning and experimenting as the field evolves.
Challenge | Solution |
---|---|
Latency | Client prediction, server checks |
Collisions | Server authority for key actions |
Sync issues | Interpolation, fixed intervals |
Scaling | Optimize data, client prediction |
Related video from YouTube
Main Challenges in Networked Physics
Networked physics in multiplayer games is tricky. Here's why:
Latency Issues
Latency can ruin fast-paced games. How do we fix it?
- Use client prediction
- Let the server check client actions
Many racing games use two physics engines:
- One synced with the server
- Another for client-side predictions
This helps smooth things out. As one dev said:
"The user would see an averaged position between the current and previous predicted positions. This prevents too much jitter."
Collision Headaches
Collisions can mess up physics sync between server and clients.
Server or client handling? It's a trade-off:
Where | Good | Bad |
---|---|---|
Server | Secure, consistent | Slower |
Client | Fast | Cheating risk |
Most games use both. Server authority for key stuff, client for quick reactions.
Syncing Game States
Keeping everyone on the same page is tough, especially with lag.
How to align physics across clients?
- Use interpolation to smooth movement
- Update at fixed intervals
Don't use "lockstep" for fast games. It's too slow.
Handling Lots of Players
More players = more physics problems.
To keep things smooth:
- Cut down on network data
- Lean on client prediction
- Split players into smaller groups
Complex Topics
Let's tackle some tricky issues in networked physics.
1. Stopping Cheaters
Cheating ruins the fun. Here's how to keep things fair:
- Server controls shared objects
- Clients send commands through player objects
- Use server-side checks
Unity developer Graithen says:
"Send input events and do the rest on the server. It's secure and gives good user feedback."
Here's how:
1. Set up a trigger volume for interactive objects
2. When a player hits "E", send an activation command
3. Replicate across all clients
This keeps things secure and responsive.
2. Complex Object Interactions
Managing lots of interactive objects? Try this:
- Use "Object Oriented" coding
- Create a master blueprint (e.g., BP_Light)
- Make child classes that inherit from it
This helps manage different items without repeating code. Think: a master "Switch" blueprint controlling doors, lights, and pickups.
3. Advanced Lag Fixes
Lag can make or break a game. Here are some fixes:
Lag Compensation
This lets the server see each client's view by:
- Keeping object position history
- Knowing client delay
- Using past raycasting
Set it up:
- Add
HitboxRoot
to your GameObject's top node - Keep dynamic objects separate from
Hitbox
nodes
This helps hit static objects while avoiding issues with moving ones.
Time Rewinding
For ultra-precise games, use sub-tick accuracy:
- Server knows exact object positions between ticks
- Can determine hits even with tiny movements
Here's a lag-compensated raycast in C#:
Runner.LagCompensation.RaycastAll(transform.position, transform.forward, rayLength, player: Object.InputAuthority, _hits, layerMask, clearHits: true);
sbb-itb-bfaad5b
Fixing Common Problems
Reducing Jerky Movement
Jerky movement can ruin a player's experience. Here's how to smooth things out:
- Turn on interpolation for rigidbodies near the camera. This cuts down on visual jitter.
- Match your Fixed Timestep to your rendering frame rate. For 60Hz, set it to 1/60.
- Use client-side prediction for immediate feedback. But watch out - the server might disagree.
- When correcting positions, don't snap. Blend between positions instead.
A Unity forum user noted:
"Both approaches work well. If I wasn't such a perfectionist, I probably wouldn't notice it's a tiny bit jerky compared to 'position += movement * delta' which creates the smoothest movement."
Keeping Physics Consistent Across Devices
For fair gameplay, physics need to be consistent. Here's how:
- Use a fixed timestep for physics on all devices.
- Stick to basic collision shapes like spheres and boxes.
- Let the server make final physics decisions.
- Use lag compensation techniques like time rewinding.
Here's a C# example of a lag-compensated raycast:
Runner.LagCompensation.RaycastAll(transform.position, transform.forward, rayLength, player: Object.InputAuthority, _hits, layerMask, clearHits: true);
- Test your game on different devices often.
Tips and Performance Improvement
Reducing Network Data Use
Want to cut down on bandwidth in physics-heavy games? Here's how:
- Only send what's needed. In a MOBA, send the hero's destination, not their constant position.
- Use fixed timesteps to keep clients in sync.
- Make your simulation deterministic. It's easier to replicate and needs less data.
- Avoid chain reactions. They're hard to sync and fix.
- Compress your data packets. Less data = less bandwidth.
Finding Performance Issues
Spot those pesky networked physics bottlenecks:
- Unity's Physics Debugger is your friend. Use it to find collider issues.
- Keep an eye on physics objects. Too many active rigid bodies? That's a red flag.
- Test with lag. Use a simulator to create terrible network conditions.
- Tweak your Fixed Timestep. It's a balancing act between speed and accuracy.
- Watch out for complex colliders. They're performance hogs. Try using simpler shapes instead.
"Networking is about looking at the things that you NEED to network. This is the key to planning and building efficient networking." - Glenn Fiedler, Network Physics Expert
Wrap-up
Networked physics in game dev? It's tough. Here's the rundown:
Latency is a pain. Devs use tricks like client-side prediction to smooth things out.
Collisions need careful syncing across networks. Otherwise, things get messy.
Syncing game states is crucial. Deterministic sims help, but they're not always doable.
Lots of players = server and bandwidth strain. Gotta optimize that data transfer.
How to tackle this stuff?
- Use fixed timesteps and deterministic sims when you can
- Compress those data packets
- Unity's Physics Debugger is your friend
- Test with fake lag early on
Glenn Fiedler, a network physics guru, says:
"Networking is about looking at the things that you NEED to network. This is the key to planning and building efficient networking."
Keep learning and tweaking. This field's always changing.
Want to go deeper? Look into:
- Advanced lag compensation
- Machine learning in physics sims
- VR/AR impacts on networked physics
The networked physics world keeps evolving. Stay curious, keep experimenting.