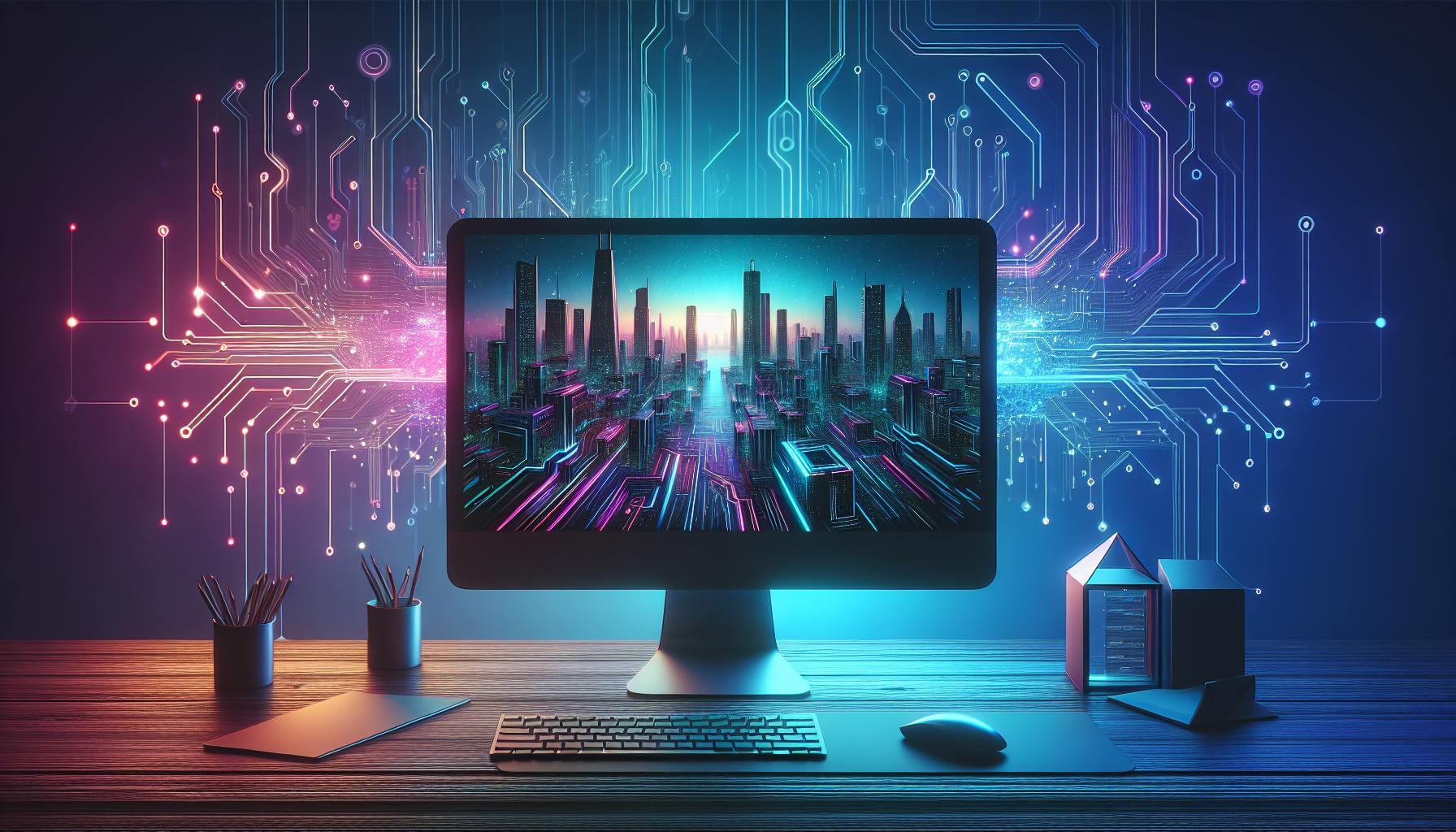
Discover how Next.js simplifies the process of creating fast, scalable developer news platforms. Learn about setting up your environment, integrating news APIs, customizing user experience, optimizing performance, enhancing SEO, and deployment tips.
Next.js is a powerful tool built on React that simplifies the process of developing fast, scalable websites, making it an ideal choice for creating developer news platforms. Here's a quick overview of what you'll learn:
- Understanding Next.js: Discover how Next.js uses React, server-side rendering, auto code splitting, and simplified routing to enhance web development.
- Setting Up Your Next.js Environment: Steps to install Node.js, create a Next.js app, and add necessary libraries.
- Integrating News APIs: How to pull in news stories using APIs like NewsAPI.org, and display them in your Next.js app.
- Customizing User Experience: Implement user profiles and personalized news feeds with tools like NextAuth.js.
- Optimizing Performance: Techniques such as server-side rendering, incremental static regeneration, and code splitting to improve your app's efficiency.
- Enhancing SEO: Strategies to make your Next.js app more visible in search results, including leveraging SSR and implementing metadata best practices.
- Deployment and Scaling: Tips for deploying your app with Vercel and scaling with AWS Lambda.
By following this guide, you'll learn how to leverage Next.js for creating a developer news platform that is fast, SEO-friendly, and scalable.
Core Concepts
- React Framework Builds: Since Next.js uses React, you can use all the cool features and parts of React to build your site.
- Server-Side Rendering: This means that your web pages are prepared on the server first. This helps your site load faster and look better in search results.
- Auto Code Splitting: Next.js automatically divides your code into smaller pieces, so your site loads quicker.
- Simplified Routing: It's really easy to link different pages together because of how Next.js organizes files.
- Plugin Ecosystem: You can add extra features to your site easily, like better analytics or SEO tools.
Benefits for News Apps
Next.js is great for news apps because:
- SEO Optimization: Having your pages ready on the server means search engines can find your content more easily.
- Performance: The smart way it handles code and pages means your site runs fast.
- Scalability: As more people visit your site, Next.js can handle the growth smoothly.
- Rapid Development: Quick updates and easy page linking mean you can build your site faster.
With Next.js, making a news site is less complicated. It loads fast, grows with your audience, and makes sure people can find your content easily. Plus, using React means you can organize your site into parts that are easier to manage. In short, Next.js takes away a lot of the hard work in building a news app.
Setting Up Your Next.js Environment
To start making a Next.js app for developer news, you first need Node.js on your computer. Node lets you run JavaScript code outside the internet browser.
Here's how to get your Next.js development environment ready:
- Install Node.js Visit nodejs.org and download the LTS version that works with your computer. This also gives you npm, which is a tool for installing Next.js and other helpful libraries.
-
Create a Next App
Open your terminal (the command line tool) and type:
This command creates a new Next project in a folder namednpx create-next-app my-dev-news
my-dev-news
. -
Install Additional Libraries
Open the
package.json
file in your new project folder. Here, you can add other libraries you might need, like:
After adding them, run"dependencies": { "next": "latest", "react": "^18.2.0", "react-dom": "^18.2.0", "axios": "^1.2.1" }
npm install
to download these libraries. - Set Up Version Control Start a git repository to keep track of your changes. After your initial commit, create a GitHub repository and push your code there. This helps you monitor your project's progress.
With these steps, you're ready to begin working on your Next.js app! Next, you might want to look into adding custom serverless functions for fetching data or setting up user login features.
Integrating News APIs
Adding news APIs to your Next.js app is a smart way to get a lot of different news stories for developers. These APIs let you easily bring in news from many sources, keeping your app filled with the latest updates.
Here are a couple of news APIs you might want to use:
- NewsAPI.org - This one gives you news headlines and stories from more than 30,000 sources. It also lets you find news that fits what you're looking for by using filters.
- ContextualWeb Unsplash - This service offers high-quality pictures that match your topics, making your articles look better.
Here's a simple way to get news from NewsAPI using Axios in your Next.js app:
export async function getServerSideProps() {
const apiResponse = await axios.get(
`https://newsapi.org/v2/top-headlines?category=technology&apiKey=${API_KEY}`
);
return {
props: {
articles: apiResponse.data
}
}
}
This code gets news data and makes it ready for your app. It does this before the page shows up to users, which makes your app faster and better for them.
For getting data right when users are on the site, you can use a tool called SWR. It's great for fetching data and makes sure your app always has the latest news without you having to do much.
import useSWR from 'swr';
function NewsList() {
const { data } = useSWR('/api/news', fetch);
if (data) {
return (
<div>
{data.articles.map((article) => (
<NewsArticle key={article.url} article={article} />
))}
</div>
);
}
return <LoadingSpinner />;
}
With SWR, your app automatically updates when there's new data, so users always see the latest news.
By using these APIs and tools, your Next.js app can show fresh, relevant news to developers. It's not too hard to set up, and it adds a lot of value to your app.
Customizing User Experience
Making a news app work for each person means changing it based on what they like or want. Next.js helps you do just that, making sure everyone gets news that interests them.
Implementing User Profiles
Letting users log in makes the app more personal. You can use something called NextAuth.js to make logging in easy. Here's a simple way to let people sign in with their Google account:
import NextAuth from "next-auth"
import GoogleProvider from "next-auth/providers/google"
export default NextAuth({
providers: [
GoogleProvider({
clientId: process.env.GOOGLE_ID,
clientSecret: process.env.GOOGLE_SECRET
})
]
})
You can keep track of what users like, such as their favorite topics or where theyโre from, in a database:
const userSchema = new Schema({
name: String,
topics: [String],
locations: [String],
sources: [String]
});
module.exports = mongoose.model('User', userSchema);
Then, use this info to show them news they're interested in.
Building Personalized News Feed
Once you know what users like, you can use Next.js to show them news that matches their interests:
export default async function handler(req, res) {
const user = await User.findById(req.userId);
const response = await fetch(
`https://newsapi.org/v2/top-headlines?categories=${user.topics.join(',')}&country=${user.locations[0]}&apiKey=${API_KEY}`
);
res.status(200).json(response.data);
}
This code looks for news that fits the user's chosen topics and place.
You can then put this news into a feed made just for them:
export default function Feed({ articles }) {
return (
<div>
{articles.map(article => (
<ArticleCard article={article} />
))}
</div>
)
}
export async function getServerSideProps(context) {
const articles = await fetchPersonalizedArticles(context.userId);
return {
props: {
articles
}
}
}
Next.js makes sure this feed is ready to go before the user even sees it, making everything run smoothly.
By setting up profiles and knowing what users like, Nextjs makes sure everyone gets news that's just right for them.
Optimizing Performance
Next.js has some great tools to make sure your news app works fast and smoothly. Let's talk about how you can use Next.js to make your app quicker and more efficient.
Server-Side Rendering
Next.js can prepare your web pages on the server before they even get to the user. This means when someone visits your page, they see the whole page right away, without waiting. It's like having your coffee ready as soon as you wake up - super quick.
Here's a simple way to do it:
// Fetch data in getServerSideProps
export async function getServerSideProps() {
const response = await fetch(`https://api.example.com/data`);
const data = await response.json();
return {
props: {
data
}
}
}
// Pass fetched data to page as props
export default function Page({ data }) {
return <div>{data}</div>
}
This method is really good for news apps because it makes pages load super fast.
Incremental Static Regeneration
For pages that don't change much, Next.js can update them every so often (like every hour) with new stuff. This means:
- Your pages load super quick
- Your content stays fresh without needing to update all the time
Set it up like this:
export async function getStaticProps() {
return {
props: {
data: []
},
revalidate: 3600 // update page every hour
}
}
This way, your news stays up-to-date without slowing down your site.
Image Optimization
Next.js has a special way to handle images that makes sure they load fast and look good. It can:
- Load images as they're needed
- Adjust image size for different screens
- Keep images ready to go quickly
For news sites with lots of pictures, this means your pages won't get bogged down by big images.
import Image from 'next/image'
export default function Article({imgUrl}) {
return (
<Image
src={imgUrl}
width={600}
height={400}
/>
)
}
Code Splitting
Next.js automatically breaks up your code into smaller pieces that only load when needed. For news apps with lots of different pages or parts, this means your site can load just the bits it needs, without waiting for everything at once. It's like only opening the apps you need on your phone, so it runs faster.
You don't have to do anything special for this - Next.js does it all for you!
By using these tips, you can make your news app really quick and enjoyable for your readers.
sbb-itb-bfaad5b
Enhancing SEO
Next.js is really good at making sure search engines like Google can easily find and understand your website. This is mostly because it can create your pages beforehand on the server. Let's go over some simple ways you can use Next.js to help your site show up better in search results.
Leveraging SSR for Better Crawlers
SSR stands for server-side rendering. This means Next.js prepares your web pages on a server before anyone even visits your site. This is great for SEO because:
- Faster indexing - Search engines can see and understand your pages quicker.
- Better rankings - Your site might show up higher in search results.
- Improved sharing - When people share your content on social media, it looks better.
Next.js does a lot of the heavy lifting for you, making your content ready for search engines.
Implementing Metadata Best Practices
Metadata is like a mini-advertisement for your web page that search engines read. Here are some simple rules:
- Unique page titles - Make sure each page has a special title. Keep it short.
- Meta descriptions - Write a short summary for each page. This shows up in search results.
- Open Graph tags - These help your pages look good when shared on social media.
- JSON-LD markup - This is code that helps search engines understand your content better.
You can add this information in a special file in Next.js like this:
export default class Document extends NextDocument {
render() {
return (
<Html>
<Head>
<title>Page Title</title>
<meta name="description" content="Page description" />
<meta property="og:title" content="Shared title" />
<script type="application/ld+json">
// Structured data
</script>
</Head>
<body>
...
</body>
</Html>
)
}
}
Adding Sitemaps
A sitemap is a map of your website that helps search engines find all your pages. You can make Next.js automatically create one for you:
// pages/sitemap.xml.js
export const getServerSideProps = async ({ res }) => {
const pages = await getAllPages() // method to fetch pages
const sitemap = createSitemap(pages)
res.setHeader('Content-Type', 'text/xml')
res.write(sitemap)
res.end()
return { props: {} }
}
This way, search engines can easily see all your pages.
Optimizing for Social Sharing
Adding Open Graph metadata makes your content look better on social media:
<Head>
<meta property="og:title" content="Post Title" />
<meta property="og:image" content={postImage} />
<meta property="og:description" content={excerpt} />
</Head>
And don't forget about Twitter Cards for even better sharing on Twitter:
<meta name="twitter:card" content="summary_large_image" />
Leveraging Friendly URLs
Next.js lets you make easy-to-read URLs that help people find your content:
pages/blog/[slug].js
// => yoursite.com/blog/nextjs-seo-guide
By following these tips, you can help your site get noticed more by search engines and people alike.
Deployment and Scaling
Putting your Next.js app online is pretty straightforward with Vercel, a platform made just for Next.js apps. Vercel makes sure your app runs smoothly and can handle more visitors when needed.
Deploying on Vercel
To get your app online with Vercel:
- Upload your Next.js app to GitHub or directly to Vercel
- Vercel will figure out it's a Next.js app and set things up for you
- You can tweak settings and add environment variables if you need to
- Vercel gives you a fast network, secure connections, and smart features without extra work
Once your app is up, Vercel takes care of:
- Updating your app whenever you make changes
- Letting you use your own web addresses
- Keeping your secrets safe but accessible to your app
- Making sure your site loads quickly everywhere
Vercel is all about making the process easy from start to finish.
Scaling on AWS Lambda
For bigger projects, you can use AWS Lambda to make sure your Next.js app can grow:
- Make your app and API routes ready for Lambda
- Split your app into different parts that can grow on their own
- Set up a way for internet requests to reach the right parts of your app
- Use shared code to keep things efficient
- Use fast storage or databases to keep things speedy
This setup lets each part of your app grow without affecting the others. You can also use other tools to make sure your app stays fast and responsive.
Testing is super important - start checking how well your app handles lots of users early on. Keep testing and making improvements as you go.
In short, Vercel is great for getting your Next.js app online quickly, and AWS Lambda helps you make sure it can grow. Remember to test and tweak your app to keep it running smoothly as more people use it.
Case Studies
Here are some examples of Next.js developer news apps and platforms:
DevNews Daily
DevNews Daily is a Next.js app that gives developers a personal news update every day.
Platform:
- Built with Next.js
- Uses Vercel for putting it online
- MongoDB for storing data
- NewsAPI to get news articles
Features:
- Sign-in and user profiles
- Pick your favorite topics and news sources
- Daily email with news
- No ads
- Works well on phones and computers
- Good for showing up in search results
Feedback:
"I like getting my developer news in a simple way every morning. It helps me start my day right."
Upcode
Upcode is a place for developers to talk, learn with tutorials, ask questions, and read news.
Platform:
- Next.js for the front end
- Backend with Express + Prisma
- Uses PostgreSQL for data
- Hosted with AWS
Features:
- Log in with social media accounts
- Profiles for developers
- News feeds you can customize
- Leave comments on articles
- Dark mode for easy reading
- Video lessons
Feedback:
"The news feed that's just for me makes sure I don't miss out on important JavaScript news."
DevHerald
DevHerald is a site that brings together news for developers, made with Next.js.
Platform:
- Next.js for building the site
- Backend with Node.js
- MongoDB for storing data
- Runs on DigitalOcean
Features:
- Gathers news from many places
- Search through articles
- Save articles to Pocket
- Get alerts for new news
- Looks and works great on both computers and phones
Feedback:
"DevHerald lets me keep up with developer news the way I like, focusing on what I'm interested in."
Conclusion
Next.js makes creating a website for developer news a lot easier and improves the experience for both the people making the site and the ones using it.
Here's why Next.js is helpful:
- It has a feature called server-side rendering that makes websites load faster.
- Setting up links between pages is simple.
- It automatically breaks up the code so that the website doesn't take too long to load.
- There are many add-ons available to make your site do more things.
- Putting your site online is straightforward with a service called Vercel.
For people making websites, Next.js takes away a lot of the hard work. You don't have to worry as much about the technical side of things like setting up servers or making sure pages link correctly.
For visitors, websites built with Next.js load quickly and moving around the site feels smooth. There are also neat ways to make the site show you more of what you like.
We wanted to show you that using Next.js makes it easier to build news sites that developers will want to use. It takes care of the tough stuff so you can focus on creating great content and features.
Whether you're starting with a small project or thinking big, Next.js helps you get the news out to your audience without a lot of hassle. It's also built to handle more work as your site grows.
In short, Next.js makes things simpler for everyone involved in developer news. It helps you quickly try out new ideas while making sure the site performs well, which is really important for websites that update their content often.