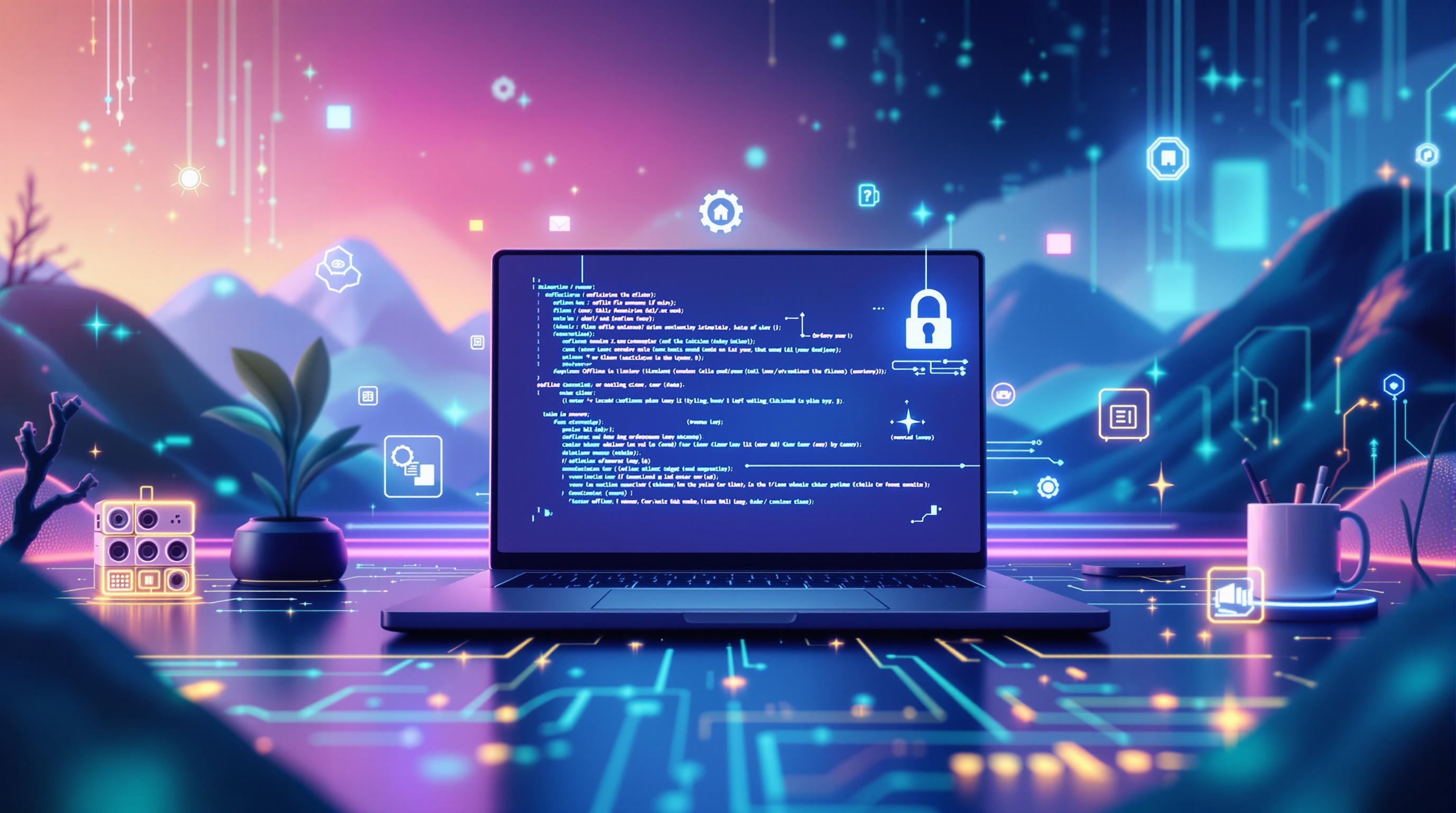
Learn how to implement offline file sync in apps to enhance user experience, boost retention, and manage data efficiently.
Key takeaways:
- Offline sync is crucial for modern apps
- It keeps users happy, protects data, and improves app speed
- 70% of users expect apps to work offline
- Apps with offline features have up to 3x higher user retention
How to implement offline sync:
- Choose the right storage: IndexedDB, Web Storage, or Cache API
- Use Service Workers for background sync
- Handle data conflicts with strategies like Last Writer Wins or Manual Intervention
- Optimize data transfer with delta sync and compression
- Implement smart caching and efficient data formats
Real-world examples:
- Evernote: Create and edit notes offline
- Spotify: Play music offline
- Google Maps: Navigate offline
Remember: "Local-first software: You own your data, in spite of the cloud." - Martin Kleppmann
This guide covers everything from setting up local storage to fixing sync conflicts and optimizing performance. Let's dive in and make your apps work anywhere, anytime.
Related video from YouTube
Offline Sync Basics
Offline file sync lets users work without an internet connection. Let's explore the key ideas behind this tech.
How Sync Works
Sync keeps data consistent across devices. There are two main ways to do this:
1. One-way synchronization
Data moves from source to target. The target doesn't change or send feedback. It's great for keeping an exact or partial copy of source data.
2. Two-way synchronization
Data flows both ways. Systems exchange info with each other. It's flexible but can get tricky when conflicts pop up.
One-way sync is simple and has perks:
- Keeps data clean
- Makes data management easier
- Boosts security
- Costs less to set up
Here's a real example: When an Applicant Tracking System (ATS) marks a candidate as hired, it automatically adds their info to the Human Resource Information System (HRIS). This speeds up onboarding.
Two-way sync is more complex but flexible. It's used when devices need to share and update info back and forth. Think of syncing files between your desktop and laptop - you always have the latest version on both.
Handling Data Conflicts
Two-way sync can lead to conflicts when the same data changes in different places between syncs. Here's how to deal with that:
- Last Writer Wins (LWW): The newest change wins.
- Timestamp Ordering: Apply changes based on when they happened.
- Manual/User Intervention: Let the user decide.
- Merge/Patch: Try to combine changes from different sources.
- Predefined Rules: Solve conflicts using specific criteria or business logic.
Mobterest Studio says:
"The choice of conflict resolution strategy depends on the nature of the data, the specific requirements of the application, and the desired user experience."
Tips for handling conflicts:
- Use Change Data Capture (CDC) to sync only what's changed. This reduces system load.
- Test your sync processes often to make sure they work right.
- If users need to solve conflicts manually, make the interface clear and easy to use.
For example, FileCloud Sync creates a separate "Conflict_" file when it can't merge changes automatically. This keeps both versions so users can pick which changes to keep.
Setting Up Local Storage
Picking the right local storage method is key for offline-capable apps. Let's look at your options and how to structure data for fast syncing.
Storage Method Options
You've got three main choices for local storage:
1. IndexedDB
Great for lots of structured data. You can run complex queries and use indexing. Perfect if your app needs to handle big datasets offline.
2. Web Storage
This includes localStorage and sessionStorage. It's simple key-value storage. Easy to use, but limited (usually 5-10MB). Best for small data amounts or app settings.
3. Cache API
Made for caching network requests and responses. It's great for storing things like HTML, CSS, and JavaScript files for offline use.
Here's a quick comparison:
Storage Method | Capacity | Use Case | Persistence |
---|---|---|---|
IndexedDB | Large (depends on device) | Complex, structured data | Until cleared |
Web Storage | Usually 5-10MB | Simple key-value pairs | localStorage: stays, sessionStorage: session only |
Cache API | Large (depends on device) | Network responses, file caching | Until cleared or expires |
Pick based on what your app needs. Building a complex offline-first PWA? IndexedDB might be your best bet.
"Building robust offline capabilities, efficient caching, and reliable data synchronization is crucial for enhancing the user experience in modern Android applications." - Amar Kumar
Data Structure Planning
After picking your storage method, plan your data structure. A good structure makes syncing faster and more efficient.
Some tips:
- Keep it flat: Don't nest data too deep. Flat data syncs and queries easier.
- Use unique IDs: Give each data item a unique ID. This helps track changes and fix conflicts during sync.
- Separate different data: Store different data types in separate stores or tables. This makes queries faster and partial syncs easier.
- Store only what you need: Only keep essential info locally. This uses less storage and speeds up syncing.
- Use versioning: Keep track of data versions to help fix conflicts during sync.
For example, don't store whole user profiles locally. Just store user IDs and key info, and fetch full profiles when needed.
Make sure your data structure fits your storage method. IndexedDB, for instance, works well with object stores that match your app's data models.
sbb-itb-bfaad5b
Building Sync Features
Adding offline sync to your app? It's a game-changer for user experience. Let's break down how to set it up using service workers and manage sync tasks like a pro.
Using Service Workers
Service workers are your app's secret weapon for offline features. They sit between your app, the browser, and the network. Here's how to get them up and running:
1. Register the Service Worker
First things first, register your service worker. Pop this code into your main JavaScript file:
if ("serviceWorker" in navigator) {
navigator.serviceWorker.register("/sw.js")
.then(registration => {
console.log("Service worker registered:", registration);
})
.catch(error => {
console.error("Service worker registration failed:", error);
});
}
2. Install and Cache Resources
Now, in your sw.js
file, set up an install event to cache your important stuff:
const cacheName = "my-offline-cache-v1";
const filesToCache = [
"/index.html",
"/styles.css",
"/app.js"
];
self.addEventListener("install", event => {
event.waitUntil(
caches.open(cacheName)
.then(cache => cache.addAll(filesToCache))
);
});
3. Handle Fetch Events
Catch those network requests to serve cached content when offline:
self.addEventListener("fetch", event => {
event.respondWith(
fetch(event.request).catch(() => caches.match(event.request))
);
});
Managing Sync Tasks
Background sync is your friend for handling offline actions. Here's how to make it work:
1. Register a Sync Event
When you need to sync data, register a sync event like this:
navigator.serviceWorker.ready.then(registration => {
registration.sync.register("sync-data")
.then(() => console.log("Sync registered"))
.catch(error => console.error("Sync registration failed:", error));
});
2. Handle the Sync Event
In your service worker, listen for the sync event:
self.addEventListener("sync", event => {
if (event.tag === "sync-data") {
event.waitUntil(syncData());
}
});
async function syncData() {
// Perform sync operations here
console.log("Data synced");
}
3. Error Handling and Retries
Don't let sync failures bring you down. Implement some solid error handling and retry logic:
async function syncData() {
const MAX_RETRIES = 3;
let retries = 0;
while (retries < MAX_RETRIES) {
try {
// Attempt sync
await performSync();
console.log("Sync successful");
return;
} catch (error) {
console.error("Sync failed:", error);
retries++;
await new Promise(resolve => setTimeout(resolve, 1000 * retries));
}
}
console.error("Sync failed after max retries");
}
With these features in place, you're setting up your users for a smooth offline experience. But don't forget to test! Throw different network conditions at your app to make sure your sync features hold up.
"Service Workers are quite powerful as they can take control over network requests, modify them, serve custom responses retrieved from the cache, or synthesize responses completely." - MDN Web Docs
Real-world success story? Look at Twitter Lite. They've nailed this approach, letting users read tweets and write messages even when offline. Once the connection's back, everything syncs up with the server without a hitch.
Fixing Sync Conflicts
Building offline-capable apps? You'll run into sync conflicts. Let's dive into some practical ways to handle these tricky situations and keep your data in check across devices.
Conflict Fix Methods
Picking the right way to resolve conflicts is key. Here are some solid approaches:
1. Server-First
This method puts the server's version on top. It's simple, but watch out - you might lose important client changes.
2. Client-First
Here, the client's version wins. Great for apps where local changes matter most, but be careful not to overwrite crucial server updates.
3. Custom Fixes
Want more control? Build your own resolution logic. This lets you handle conflicts based on specific data types or business rules.
Here's a cool trick: sync actions, not values. Some advanced systems use this to cut down on conflicts. Check it out:
let customStrategy = {
resolve: (base, server, client, operationName) => {
let resolvedData;
switch (operationName) {
case "updateUser":
delete client.socialKey
resolvedData = Object.assign(base, server, client)
break
case "updateRole":
client.role = "none"
resolvedData = Object.assign(base, server, client)
break
default:
resolvedData = Object.assign(base, server, client)
}
return resolvedData
}
}
This strategy lets you handle conflicts differently for each operation. It's like having a Swiss Army knife for data syncing.
Data Type Sync Rules
Different data types need different sync rules. Here's how to handle some common ones:
Timestamps: Always add a timestamp to your data. It's like a digital time stamp that helps you track when changes happen.
Financial Data: Be extra careful with money stuff. Users could overspend if they're buying things on multiple devices without syncing. To avoid this, set up a transaction log system.
"You'd essentially be doing this. You are balancing all transactions... between all devices." - Unity Discussions Contributor
Text Files: When syncing text files, try using a diff algorithm to merge changes when you can. If that's not possible, create conflict files to keep all versions safe.
FileCloud Sync handles text file conflicts in a smart way. If it can't merge changes automatically, it creates a separate "Conflict_" file:
filename(Conflict_other_date).txt // for shared folders
filename(Conflict_teamname_date).txt // for team folders
This way, no data gets lost, and users can sort out conflicts later.
Complex Data Structures: For trickier data, think about using a Conflict-free Replicated Data Type (CRDT). These are special data structures that can be copied across multiple computers in a network. They can be updated independently and still end up in a consistent state, without needing complex consensus protocols.
Making Sync Better
Let's dive into some practical ways to boost your app's performance and user experience by optimizing offline file sync. We'll cover how to save data, cut down on bandwidth use, and fine-tune sync timing.
Save Data and Bandwidth
Smart data management is key for smooth offline sync. Here's how to trim data transfer and save bandwidth:
1. Delta Sync
Why sync entire files when you can just transfer the changes? It's like sending a quick text update instead of retelling the whole story. AppSheet's Delta sync, for example, only syncs data that's been updated since the last fetch.
2. Compression
Squeezing data before transfer is like vacuum-packing your luggage - it takes up less space. Google's Brotli compression can shrink data up to 26% more than gzip. That means faster transfers and less bandwidth gobbled up.
3. Smart Caching
Think of caching like keeping a snack in your pocket - it's there when you need it, no trip to the store required. Here's a simple example:
async function fetchData() {
const cachedData = localStorage.getItem('apiData');
if (cachedData) {
console.log('Using cached data');
return JSON.parse(cachedData);
}
const response = await fetch('https://api.example.com/data');
const data = await response.json();
localStorage.setItem('apiData', JSON.stringify(data));
return data;
}
This function checks for cached data before making an API call. It's like checking your pocket before heading to the store.
4. Efficient Data Formats
Using structured data formats like SQLite or Realm is like organizing your closet - it makes finding things faster and reduces clutter. For web apps, IndexedDB is great for storing lots of structured data efficiently.
Sync Timing and Storage
When and how you sync can make a big difference:
1. Background Syncing
Use background services or WorkManager for Android apps to sync without bugging the user. It's like having your phone update apps while you sleep - things just get done.
2. Adaptive Sync Intervals
Adjust how often you sync based on network conditions and data importance. CrashPlan, for instance, lets users set different bandwidth limits for when their PC is idle versus in use.
3. Smart Token Handling
When using Sync SDKs, handle tokens wisely. Set a Time-to-Live (TTL) of a few hours (max 24) for tokens to clear out expired Sync objects on time. Storing tokens in temporary storage can speed up app start-up.
4. Data Partitioning
Split your data into must-haves and nice-to-haves. Sync the critical stuff more often, and leave the rest for off-peak hours or Wi-Fi time.
5. Network-Aware Syncing
Tweak your sync strategy based on network quality. For example, robocopy.exe has a neat trick to manage bandwidth during file transfers:
robocopy source destination /ipg:1000
This command adds a 1-second breather between packets, easing the network load during busy hours.
Conclusion
Let's recap what we've learned about offline file sync for developers in 2024:
Offline sync isn't optional anymore. It's a must-have for great user experience. Here's why:
- 70% of users expect apps to work offline
- Apps with offline features have up to 3x higher user retention
- Offline functionality can boost user engagement by up to 45%
Real-world examples show how it's done:
- Evernote: Create and edit notes offline, sync when online
- Spotify: Play music offline
- Google Maps: Navigate offline
Key points for implementing offline sync:
1. Pick the right storage
Choose between IndexedDB, Web Storage, or Cache API based on what your app needs.
2. Transfer data efficiently
Use delta sync and compression to save bandwidth and speed up syncing.
3. Handle conflicts
Have a solid plan for resolving conflicts to keep data consistent across devices.
4. Focus on core features
Make sure the most important parts of your app work offline.
Martin Kleppmann, a top software engineer, says:
"Local-first software: You own your data, in spite of the cloud."
Keep this in mind when building offline sync.
The future is offline-first. By mastering these techniques now, you're not just fixing today's problems. You're setting up your apps for a world where working offline is normal, not special.