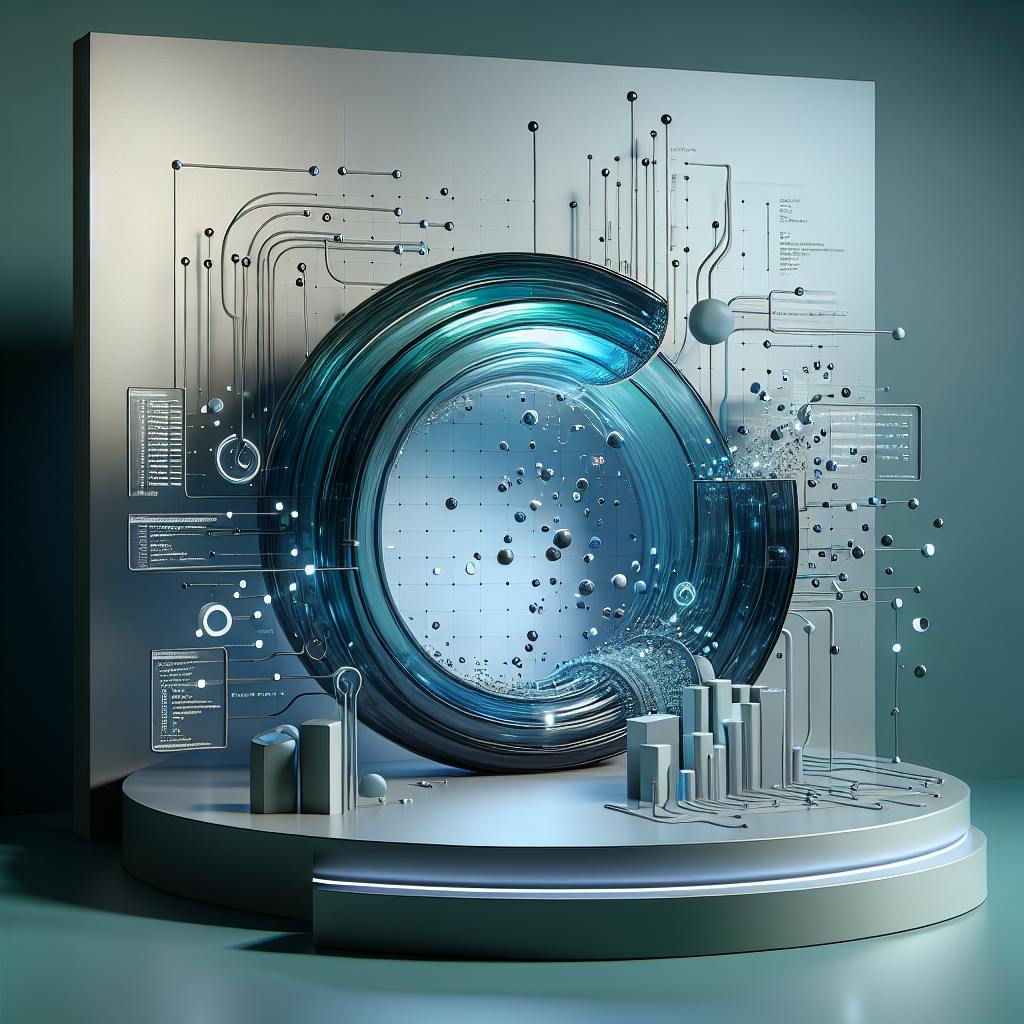
Discover how Scikit-Learn pipelines streamline machine learning workflows, ensuring consistency, reducing errors, and enhancing model performance.
Scikit-Learn pipelines streamline machine learning workflows by combining data preprocessing and model training into a single, cohesive process. Here's what you need to know:
- Pipelines bundle multiple transformers and an estimator into one object
- They ensure consistent data transformations across training and testing
- Pipelines reduce code repetition and minimize errors
- They work seamlessly with Scikit-Learn's cross-validation and hyperparameter tuning tools
Key benefits:
- Simplify complex ML workflows
- Improve code organization and maintainability
- Prevent data leakage during model evaluation
- Enable easy model sharing and reproducibility
Quick comparison of pipeline types:
Type | Description | Best For |
---|---|---|
Simple | Chain basic steps | Straightforward workflows |
Feature Union | Apply multiple transformers to same data | Complex feature engineering |
Column Transformer | Apply different transformations to different columns | Mixed data types |
Pipelines are essential for building robust, efficient, and reproducible machine learning models. By mastering Scikit-Learn pipelines, you'll streamline your ML projects and boost your productivity.
Related video from YouTube
Basics of Scikit-Learn pipelines
Scikit-Learn pipelines are like assembly lines for your machine learning projects. They string together multiple steps of data processing and model training into one smooth workflow.
Key parts of pipelines
Pipelines have two main ingredients:
- Transformers: These handle data prep. They learn patterns from training data and apply those patterns to new data.
- Estimators: These are your actual ML models. They train on data and make predictions.
Here's a simple pipeline example:
from sklearn.pipeline import Pipeline
from sklearn.preprocessing import StandardScaler
from sklearn.svm import SVC
pipe = Pipeline([
('scaler', StandardScaler()),
('svc', SVC())
])
In this case, StandardScaler
is our transformer, and SVC
(Support Vector Classifier) is our estimator.
Different pipeline types
Pipelines come in various flavors:
- Simple pipelines: These chain together basic steps, like the example above.
- Feature Union pipelines: These apply multiple transformers to the same data and combine the results.
- Column Transformer pipelines: These apply different transformations to different columns in your dataset.
Let's look at a more complex example using ColumnTransformer
:
from sklearn.compose import ColumnTransformer
from sklearn.preprocessing import OneHotEncoder, StandardScaler
from sklearn.impute import SimpleImputer
preprocessor = ColumnTransformer(
transformers=[
('num', StandardScaler(), ['age', 'income']),
('cat', OneHotEncoder(), ['gender', 'occupation'])
])
full_pipeline = Pipeline([
('preprocessor', preprocessor),
('classifier', RandomForestClassifier())
])
This pipeline handles both numerical and categorical data, scaling numbers and encoding categories before feeding everything into a Random Forest model.
Pipelines shine when you're dealing with messy, real-world data. They keep your preprocessing steps organized and make sure you apply the same steps to both your training and test data.
Plus, they play nice with Scikit-Learn's cross-validation and hyperparameter tuning tools. This means you can optimize your entire workflow - from data cleaning to model training - all at once.
Creating pipelines
Now that we understand the basics, let's dive into building pipelines with Scikit-Learn. We'll start simple and work our way up to more complex setups.
Basic pipeline setup
Setting up a basic pipeline is straightforward. Here's how:
- Import the necessary modules
- Define your transformers and estimators
- Create the pipeline object
Let's look at a simple example:
from sklearn.pipeline import Pipeline
from sklearn.preprocessing import StandardScaler
from sklearn.linear_model import LogisticRegression
pipe = Pipeline([
('scaler', StandardScaler()),
('classifier', LogisticRegression())
])
This pipeline scales the data and then applies logistic regression. It's that simple!
Adding data prep and model training
Real-world data often needs more preprocessing. Let's build a pipeline for the California housing dataset:
from sklearn.compose import ColumnTransformer
from sklearn.impute import SimpleImputer
from sklearn.preprocessing import StandardScaler, OneHotEncoder
from sklearn.ensemble import RandomForestRegressor
numeric_features = ['MedInc', 'HouseAge', 'AveRooms', 'AveBedrms', 'Population', 'AveOccup']
categorical_features = ['Ocean_Proximity']
numeric_transformer = Pipeline([
('imputer', SimpleImputer(strategy='median')),
('scaler', StandardScaler())
])
categorical_transformer = Pipeline([
('imputer', SimpleImputer(strategy='constant', fill_value='missing')),
('onehot', OneHotEncoder(handle_unknown='ignore'))
])
preprocessor = ColumnTransformer([
('num', numeric_transformer, numeric_features),
('cat', categorical_transformer, categorical_features)
])
pipe = Pipeline([
('preprocessor', preprocessor),
('regressor', RandomForestRegressor())
])
This pipeline handles both numeric and categorical data, imputes missing values, scales numeric features, and one-hot encodes categorical features before feeding everything into a Random Forest regressor.
More complex pipeline setups
For more advanced scenarios, you might need nested pipelines or custom transformers. Here's an example using a custom transformer:
from sklearn.base import BaseEstimator, TransformerMixin
class OutletTypeEncoder(BaseEstimator, TransformerMixin):
def fit(self, X, y=None):
return self
def transform(self, X):
X_ = X.copy()
X_['is_supermarket'] = X_['Outlet_Type'].isin(['Supermarket Type1', 'Supermarket Type2', 'Supermarket Type3'])
return X_
pipe = Pipeline([
('outlet_encoder', OutletTypeEncoder()),
('preprocessor', preprocessor),
('regressor', RandomForestRegressor())
])
This pipeline includes a custom transformer that creates a new binary feature based on the outlet type, followed by our previous preprocessor and regressor steps.
Improving pipelines
Once you've set up your Scikit-Learn pipeline, it's time to make it work better. Let's look at two key ways to boost your pipeline's performance: fine-tuning parameters and testing with cross-validation.
Fine-tuning parameters
To get the most out of your pipeline, you need to adjust its settings. This is where GridSearchCV
and RandomizedSearchCV
come in handy.
GridSearchCV
checks every possible combo of parameters you give it. It's thorough but can be slow. Here's how to use it:
from sklearn.model_selection import GridSearchCV
from sklearn.ensemble import RandomForestClassifier
pipe = Pipeline([
('preprocessor', preprocessor),
('classifier', RandomForestClassifier())
])
param_grid = {
'classifier__n_estimators': [100, 200, 300],
'classifier__max_depth': [5, 10, 15]
}
grid_search = GridSearchCV(pipe, param_grid, cv=5)
grid_search.fit(X_train, y_train)
print("Best params:", grid_search.best_params_)
print("Best score:", grid_search.best_score_)
RandomizedSearchCV
is faster. It picks random combos to test instead of trying them all. Use it like this:
from sklearn.model_selection import RandomizedSearchCV
from scipy.stats import randint
param_dist = {
'classifier__n_estimators': randint(100, 500),
'classifier__max_depth': randint(5, 20)
}
random_search = RandomizedSearchCV(pipe, param_dist, n_iter=100, cv=5)
random_search.fit(X_train, y_train)
Testing with cross-validation
Cross-validation helps you check how well your pipeline works on different parts of your data. It's a good way to spot overfitting.
Here's a simple way to do 5-fold cross-validation:
from sklearn.model_selection import cross_val_score
scores = cross_val_score(pipe, X, y, cv=5)
print("Cross-validation scores:", scores)
print("Mean score:", scores.mean())
For a more detailed look, use cross_validate
:
from sklearn.model_selection import cross_validate
cv_results = cross_validate(pipe, X, y, cv=5,
scoring=['accuracy', 'precision', 'recall'])
print("Mean accuracy:", cv_results['test_accuracy'].mean())
print("Mean precision:", cv_results['test_precision'].mean())
print("Mean recall:", cv_results['test_recall'].mean())
sbb-itb-bfaad5b
Understanding pipeline results
After running your Scikit-Learn pipeline, you need to know how to read and show what it did. Let's break this down into two key parts.
Reading the results
To see what your pipeline did, you can use the named_steps
attribute. This shows you all the steps in your pipeline:
print(pipe.named_steps)
For a clearer view, try printing the pipeline to an HTML file:
from sklearn.utils import estimator_html_repr
with open('pipeline.html', 'w') as f:
f.write(estimator_html_repr(pipe))
This gives you a neat, clickable diagram of your pipeline steps.
To check how well your model works, look at its performance metrics. For example, if you used cross_validate
, you might see something like this:
print(f"Accuracy: {cv_results['test_accuracy'].mean():.2f}")
print(f"Precision: {cv_results['test_precision'].mean():.2f}")
print(f"Recall: {cv_results['test_recall'].mean():.2f}")
Showing feature importance
Knowing which features matter most can help you understand your model better. Here's how to get feature importance for different types of models:
For tree-based models (like Random Forest):
importances = pipe.named_steps['classifier'].feature_importances_
feature_names = pipe.named_steps['preprocessor'].get_feature_names_out()
for name, importance in zip(feature_names, importances):
print(f"{name}: {importance:.4f}")
For linear models (like Logistic Regression):
coef = pipe.named_steps['classifier'].coef_[0]
feature_names = pipe.named_steps['preprocessor'].get_feature_names_out()
for name, c in zip(feature_names, coef):
print(f"{name}: {c:.4f}")
To make this info easier to read, put it in a table:
Feature | Importance |
---|---|
age | 0.2345 |
income | 0.1678 |
gender | 0.0987 |
Remember, these numbers show how much each feature affects the model's decisions. Higher numbers mean the feature is more important.
Tips for using pipelines
Scikit-Learn pipelines can be tricky, but with the right approach, you can fix errors and speed things up. Let's look at some practical tips.
Fixing errors
Common pipeline problems often stem from data issues or mismatched steps. Here's how to tackle them:
1. Check data types
Make sure your data types match what each step expects. For example:
from sklearn.preprocessing import StandardScaler
from sklearn.pipeline import Pipeline
# Create a pipeline with standard scaling
standard_scaler = StandardScaler()
preprocess_pipeline = Pipeline([
('scale', standard_scaler)
])
If your data isn't numeric, this pipeline will fail. Always check your data types before feeding them into the pipeline.
2. Use custom transformers for debugging
Create a custom transformer to print out data at different stages:
from sklearn.base import TransformerMixin, BaseEstimator
import pandas as pd
class Debugger(BaseEstimator, TransformerMixin):
def transform(self, data):
print("Shape of Pre-processed Data:", data.shape)
print(pd.DataFrame(data).head())
return data
def fit(self, data, y=None, **fit_params):
return self
Add this to your pipeline to spot issues early:
pipeline = Pipeline([
('debug1', Debugger()),
('scale', StandardScaler()),
('debug2', Debugger()),
# ... other steps
])
3. Handle missing data
Missing data can break your pipeline. Use imputation techniques:
from sklearn.impute import SimpleImputer
pipeline = Pipeline([
('imputer', SimpleImputer(strategy='mean')),
# ... other steps
])
Making pipelines run faster
Speed up your pipelines with these tips:
1. Use parallel processing
The n_jobs
parameter can speed up certain steps:
from sklearn.preprocessing import StandardScaler
from sklearn.compose import ColumnTransformer
preprocessor = ColumnTransformer(
transformers=[
('num', StandardScaler(), ['age', 'income']),
# ... other transformers
],
n_jobs=-1 # Use all available processors
)
2. Feature selection
Remove irrelevant features to cut down processing time:
from sklearn.feature_selection import SelectKBest
pipeline = Pipeline([
('feature_selection', SelectKBest(k=10)),
# ... other steps
])
3. Efficient hyperparameter tuning
Use RandomizedSearchCV
instead of GridSearchCV
for faster tuning:
from sklearn.model_selection import RandomizedSearchCV
param_dist = {
'n_estimators': [100, 200, 300],
'max_depth': [5, 10, 15]
}
random_search = RandomizedSearchCV(
estimator,
param_distributions=param_dist,
n_iter=10,
cv=5,
n_jobs=-1
)
Advanced pipeline methods
Let's dive into some advanced ways to customize and extend Scikit-Learn pipelines.
Making custom pipeline parts
Sometimes, you need to create special components for specific jobs in your pipeline. Here's how:
1. Create a custom transformer
To make a custom transformer, inherit from BaseEstimator
and TransformerMixin
:
from sklearn.base import BaseEstimator, TransformerMixin
class CustomTransformer(BaseEstimator, TransformerMixin):
def __init__(self, column_name, multiplier=2):
self.column_name = column_name
self.multiplier = multiplier
def fit(self, X, y=None):
return self
def transform(self, X):
X_transformed = X.copy()
if pd.api.types.is_numeric_dtype(X_transformed[self.column_name]):
X_transformed[self.column_name] *= self.multiplier
else:
X_transformed[self.column_name] = X_transformed[self.column_name].apply(lambda x: str(x).capitalize())
return X_transformed
This transformer multiplies numeric columns by a given value or capitalizes string columns.
2. Use the custom transformer in a pipeline
Now, add your custom transformer to a pipeline:
from sklearn.pipeline import Pipeline
from sklearn.preprocessing import StandardScaler
from sklearn.ensemble import RandomForestClassifier
pipeline = Pipeline([
('custom', CustomTransformer('age', multiplier=3)),
('scaler', StandardScaler()),
('rf', RandomForestClassifier())
])
3. Create task-specific transformers
For more complex tasks, you can create specialized transformers. Here's an example of an age imputer:
class AgeImputer(BaseEstimator, TransformerMixin):
def __init__(self, max_age):
self.max_age = max_age
def fit(self, X, y=None):
self.mean_age = round(X['Age'].mean())
return self
def transform(self, X):
X.loc[(X['age'] > self.max_age) | (X['age'] < 0), "age"] = self.mean_age
return X
Use it in a pipeline like this:
pipe = Pipeline([
('age_imputer', AgeImputer(max_age=100)),
('imputer', SimpleImputer()),
('scaler', StandardScaler()),
('rf', RandomForestClassifier())
])
Saving and reusing pipelines
Saving pipelines for later use is key for consistent data processing. Here's how:
1. Save the pipeline
Use Python's built-in pickle
module to save your pipeline:
import pickle
# Fit your pipeline
pipeline.fit(X_train, y_train)
# Save the pipeline
with open('my_pipeline.pkl', 'wb') as file:
pickle.dump(pipeline, file)
2. Load and use the saved pipeline
To use your saved pipeline:
# Load the pipeline
with open('my_pipeline.pkl', 'rb') as file:
loaded_pipeline = pickle.load(file)
# Use the loaded pipeline
predictions = loaded_pipeline.predict(X_test)
By saving your pipeline, you ensure that all data preparation steps are applied consistently to new data.
Conclusion
Scikit-Learn pipelines are game-changers for machine learning workflows. They're not just a nice-to-have; they're essential tools for anyone serious about building efficient, maintainable ML models.
Here's why pipelines matter:
- Streamlined workflows: Pipelines combine data preprocessing and model training into a single, cohesive process. This means less code duplication and fewer chances for errors.
- Consistency is key: By using pipelines, you ensure that the same transformations are applied to both training and test data. This prevents inconsistencies that can wreck your model's performance.
- Time-saver: Pipelines automate repetitive tasks, freeing you up to focus on the more creative aspects of ML development.
- Reproducibility: With pipelines, it's easier to recreate your results and share your work with others.
But don't just take my word for it. Let's look at some real-world impact:
"Pipelines aren't just a 'nice-to-have.' They're the backbone of robust Machine Learning systems."
This quote sums it up perfectly. Pipelines are the unsung heroes of ML, working behind the scenes to make everything run smoothly.
Remember:
- Master pipelines to boost your productivity
- Use them to keep your projects organized and error-free
- Leverage pipelines for consistent data transformations
By embracing Scikit-Learn pipelines, you're setting yourself up for success in the world of machine learning. They're your ticket to cleaner code, more reliable models, and a smoother development process overall.
So, what are you waiting for? Start building your pipelines today and watch your ML projects take off!
FAQs
What are two advantages of using sklearn pipelines?
Sklearn pipelines offer two main advantages:
- Encapsulation: Pipelines bundle all preprocessing and modeling steps into a single object. This makes your code cleaner and easier to manage.
- Reduced Code Repetition: You don't have to repeat preprocessing steps when trying different models. This saves time and reduces errors.
Let's break these down:
Encapsulation
Pipelines wrap up all your data processing and model training into one neat package. It's like having a Swiss Army knife for machine learning. Instead of juggling multiple tools, you have everything in one place.
Reduced Code Repetition
Imagine you're testing 5 different models. Without pipelines, you'd have to preprocess your data 5 times. With pipelines, you do it once. It's a huge time-saver.
Here's a quick comparison:
Without Pipelines | With Pipelines |
---|---|
Repeat preprocessing for each model | Preprocess once |
More code to maintain | Less code to maintain |
Higher chance of errors | Lower chance of errors |
Harder to share and reproduce | Easier to share and reproduce |
Pipelines aren't just a convenienceโthey're a best practice. They help you build more robust, maintainable machine learning workflows.