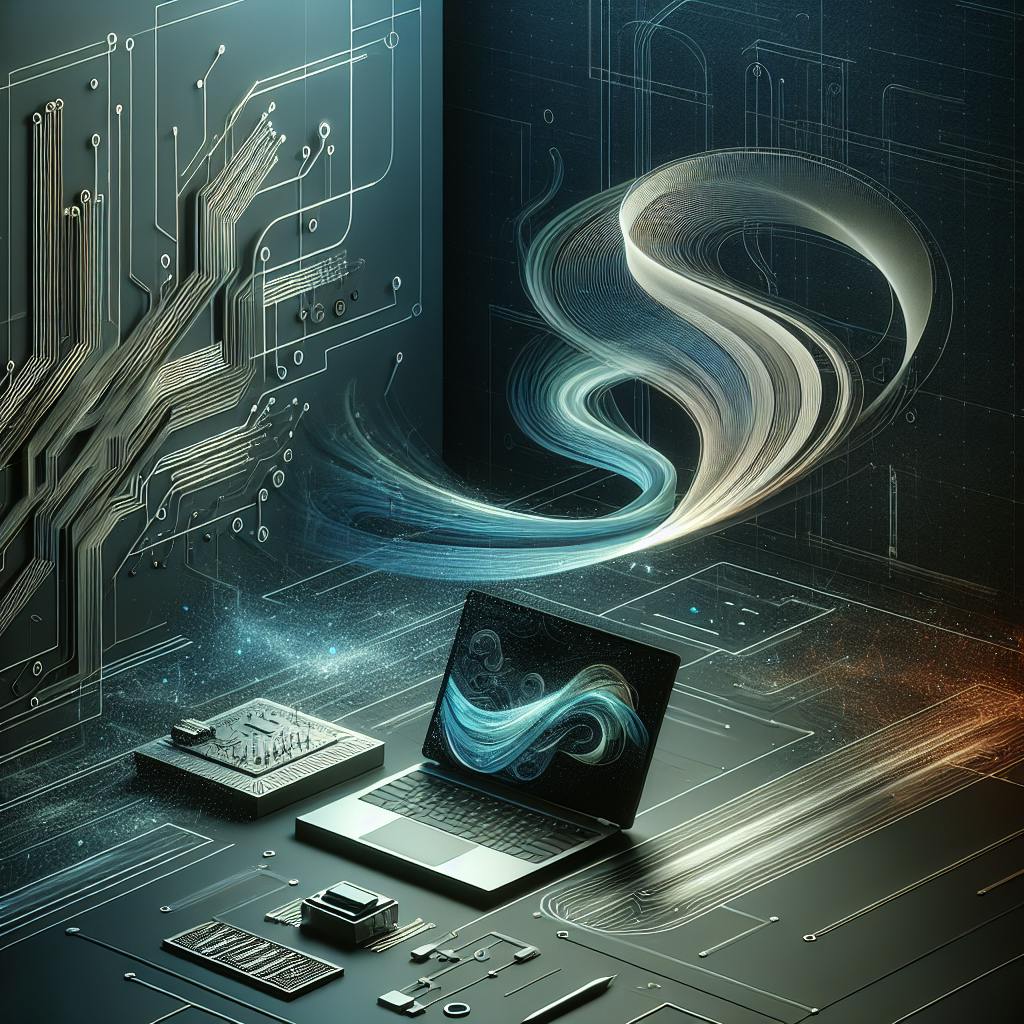
Learn the top 10 best practices for using build tools effectively in software development. Improve efficiency, reduce errors, and create a streamlined development process.
Here are the 10 key best practices for using build tools effectively:
- Choose the right build tool for your project
- Integrate with version control systems
- Automate build processes
- Optimize build speed
- Implement continuous integration
- Manage dependencies properly
- Set up comprehensive testing
- Use build configurations/profiles
- Implement logging and monitoring
- Keep build scripts clean and maintainable
Following these practices helps developers:
- Improve efficiency
- Reduce manual errors
- Create a more streamlined development process
Practice | Benefit |
---|---|
Automation | Saves time, reduces errors |
Integration | Improves collaboration |
Optimization | Faster builds, quicker feedback |
Testing | Catches bugs early |
Monitoring | Identifies issues quickly |
Implementing these best practices leads to more reliable, efficient software development for projects of any size.
Related video from YouTube
1. Choose the Right Build Tool
Picking the best build tool for your project is a key step in software development. Here's how to make a good choice:
What to Think About
- Project Needs: Look at how big and complex your project is. Small projects might work well with simple tools like Apache Ant. Bigger projects with many parts might need stronger tools like Gradle or Maven.
- Team Skills: Think about what tools your team knows how to use. A tool that's easy to learn and has good support can help your team work better.
- Customization: Some tools, like NPM and Gradle, let you add lots of extras to fit your needs. Others have a more fixed way of working.
Speed and Help
Check how fast the build tool works, especially if you're switching from another tool. Sometimes, you can make your current tool work better instead of changing to a new one. Also, make sure the tool you pick has a good community or company behind it to help when you have problems.
Team Agreement
It's important that your whole team agrees on the build tool. When everyone likes the tool, it's easier to work together and get things done faster.
Factor | Why It's Important |
---|---|
Project Size | Matches tool to project needs |
Team Knowledge | Easier adoption and use |
Customization Options | Fits specific workflow needs |
Performance | Affects development speed |
Support Available | Helps solve problems quickly |
Team Agreement | Improves teamwork and efficiency |
2. Implement Version Control Integration
Connecting your build tools with version control systems (VCS) like Git helps teams work better together and makes building software easier. Here's why it's important and how to do it well.
Why Use Version Control
VCS tools help track changes in your code over time. They let many people work on the same project without messing up each other's work. When you connect your build tool to a VCS, you make sure every build uses the latest code. This keeps your work consistent and reliable.
Benefits of Connecting Build Tools and VCS
Benefit | Description |
---|---|
Automatic Builds | Builds start on their own when code changes |
Easy to Track Changes | See who changed what and why |
Better Branch Management | Test new features separately before adding them to the main code |
How to Connect Build Tools and VCS
- Pick the Right Tools: Make sure your build tool works with your VCS. Many build tools like Jenkins and Travis CI work well with common VCS platforms.
- Set Up Automatic Triggers: Use webhooks to start builds when code changes. This keeps your builds up-to-date.
- Choose When to Build: Decide what should start a build, like when someone asks to add new code. This helps use your resources well.
3. Automate Build Processes
Making build processes automatic helps make software development more reliable and faster. By doing this, developers can make many parts of building software happen on their own, like turning code into a program, testing it, packaging it, and putting it where it needs to go. This cuts down on mistakes people might make and keeps things the same across different setups.
Main Steps to Make Builds Automatic
1. Pick Good Tools: Choose tools that fit what your project needs. Some popular ones are Jenkins, Gradle, and Maven. Each works well for different kinds of projects and programming languages.
2. Set Up a Central System: Having one main place to handle builds makes it easier to manage and share the final software. This helps a lot when teams work on software for different devices or systems.
3. Add Automatic Testing: Make tests run on their own as part of the build. This helps find problems early, before they become big issues.
4. Use Alerts: Set up a way to tell team members when builds are done or if there are problems. This helps everyone fix issues quickly.
5. Work with Version Control: Connect your build system to tools like Git that keep track of code changes. This way, new code gets built and tested right away.
Step | Why It's Important |
---|---|
Pick Good Tools | Matches tools to what your project needs |
Set Up a Central System | Makes managing and sharing builds easier |
Add Automatic Testing | Finds problems early |
Use Alerts | Helps team fix issues fast |
Work with Version Control | Keeps builds up-to-date with latest code |
4. Make Builds Faster
Making builds faster helps teams work better and get things done quicker. Here's how to speed up your builds:
1. Run Tests at the Same Time
Instead of running tests one after another, run many at once. This saves a lot of time. Also, try to run only the tests that check the parts of code you changed.
2. Fix Build Scripts
Look at your build scripts often. Remove steps you don't need and update old commands. Good scripts make builds faster and easier to fix.
3. Use Strong Computers
The computers you use for builds matter. Better computers with fast parts can make builds much quicker. If you use cloud services, pick stronger options to save time.
4. Watch How Builds Work
Use tools to see how your builds are doing. These tools show you where builds are slow. Check build reports often to find ways to make them faster.
Way to Speed Up Builds | How It Helps |
---|---|
Run tests at once | Saves time by doing many things together |
Fix build scripts | Removes slow parts and makes builds smoother |
Use strong computers | Makes everything run faster |
Watch how builds work | Helps find and fix slow parts |
5. Implement Continuous Integration
Continuous Integration (CI) helps teams work better together and catch problems early. Here's how to set up CI:
1. Use One Main Code Storage
Keep all your code in one place. This makes it easier to:
- Work together
- Track changes
- Go back to old versions if needed
2. Make Builds Happen on Their Own
Set up your system to build code automatically when someone makes changes. This:
- Finds problems quickly
- Keeps your code ready to use
3. Make Builds Fast
Quick builds are important. Aim for builds that finish in a few minutes. To speed up builds:
- Break them into smaller parts
- Run tests at the same time
- Save parts that haven't changed
4. Add Tests to Builds
Make sure your builds run tests on their own. This:
- Catches problems early
- Saves time on manual testing
- Makes sure new changes don't break old code
5. Let People Know What's Happening
Tell your team when builds are done or if there are problems. Use tools that send messages right away. This helps:
- Fix issues quickly
- Keep everyone in the loop
CI Practice | Why It's Good |
---|---|
One code storage | Makes teamwork easier |
Automatic builds | Finds issues fast |
Fast builds | Encourages frequent updates |
Built-in tests | Catches bugs early |
Quick updates | Helps fix problems fast |
sbb-itb-bfaad5b
6. Manage Dependencies Effectively
Managing dependencies well is key for developers, especially when working on big projects that use many libraries. Here's how to do it:
Use a Tool to Handle Dependencies
Tools like Maven or Gradle make it easier to manage dependencies. They let you:
- List all your project's dependencies in one file
- Get the right versions of libraries automatically
- Update libraries easily
Set Clear Rules
Make rules for how your team handles dependencies. This includes:
- Choosing specific versions of libraries to use
- Updating libraries regularly to fix security issues
- Using as few dependencies as possible
Check for Security Problems
Look for security issues in your dependencies often. Use tools that can find known problems in your libraries. This helps you fix issues before they cause trouble.
Fix Conflicts Quickly
When libraries don't work well together, it can cause problems. To avoid this:
- Use version numbers that make sense
- Update or downgrade libraries when needed
- Write down what changes you make
Remove What You Don't Use
Check your project and take out any dependencies you're not using. This:
- Makes your project simpler
- Reduces the chance of security issues
- Makes your project build faster
Tip | Why It's Good |
---|---|
Use a dependency tool | Makes managing libraries easier |
Set clear rules | Keeps everyone on the same page |
Check for security issues | Stops problems before they start |
Fix conflicts quickly | Keeps your project running smoothly |
Remove unused dependencies | Makes your project simpler and safer |
7. Implement Proper Testing
Good testing helps make sure your software works well. Here's how to do it:
Use Automatic Tests
Make tests run on their own as part of your build process. This helps find problems quickly without people having to do it by hand. Add different kinds of tests like:
- Unit tests (check small parts)
- Integration tests (check how parts work together)
- Functional tests (check if it does what it should)
Run Many Tests at Once
If you can, run multiple tests at the same time. This makes testing faster, especially for big projects. If your computers can't do this, think about using cloud services to help.
Keep Test Areas Clean
Use a fresh, separate place for each test. This stops old tests from messing up new ones. Using containers can help make sure each test starts the same way.
Start with Quick Tests
Do fast, simple tests first before doing longer, more complex ones. This lets you know about problems sooner. Quick tests look at basic things, while longer tests check harder stuff.
Check and Update Tests Often
Look at your tests regularly to make sure they still work for your current code. As you add new features or change old ones, update your tests too. This helps keep your tests useful.
Testing Practice | What It Does | Why It's Good |
---|---|---|
Automatic Tests | Runs tests without people | Finds problems fast |
Parallel Testing | Runs many tests at once | Makes testing quicker |
Clean Test Areas | Uses fresh space for each test | Stops old tests from causing issues |
Quick Tests First | Does simple checks before complex ones | Gives fast feedback |
Regular Updates | Keeps tests current with code changes | Ensures tests stay useful |
8. Use Build Configurations
Build configurations help developers make their builds work for different settings, like development, testing, and production. By using profiles, you can change parts of your build process to fit what each setting needs. This makes your builds more flexible and efficient.
Why Use Build Configurations
Reason | Explanation |
---|---|
Fit Different Settings | Change settings based on where you're using the build |
Work on Many Systems | Makes it easier to use the same build on different computers |
Use Tools When Needed | Turn on special tools only when you need them |
Change Values Easily | Set different values for each setting without changing the main code |
How to Use Build Configurations Well
1. Make Clear Profiles: Write down what each profile is for in your build files. This helps your team know when to use each one.
2. Check Your Profiles: Always test your configurations to make sure they work right. This stops problems when you use them in different places.
3. Keep Them Up to Date: Look at your build configurations often. Fix them when your project needs change or when you start using new tools.
9. Set Up Good Logging and Watching
Good logging and watching help keep your build process working well. They let developers see what's happening, find slow parts, and fix errors quickly. This makes build tools more reliable and helps teams work better together.
Why Logging and Watching Are Good
Benefit | Description |
---|---|
Find Errors | Logs show what happened during builds, making it easy to spot problems |
See How Well It Works | Watching build times and computer use helps find slow parts |
Keep Old Information | Logs let teams see changes over time and understand how code changes affect builds |
How to Set Up Good Logging
- Use Organized Logs: Make logs in a format like JSON instead of plain text. This makes it easier to search and study logs with computers.
- Use Different Log Levels: Use levels like DEBUG, INFO, WARN, and ERROR to sort messages. This helps developers focus on what's important.
- Use One Place for Logs: Think about using systems that put all logs in one place (like ELK Stack or Splunk). This gives a clear view of the whole build process and makes watching easier.
Tools for Watching
Using tools to watch your builds can make them work better. Tools like Prometheus or Grafana can keep track of important things like:
What to Watch | Why It's Important |
---|---|
Build Times | Shows if builds are getting faster or slower |
Success Rates | Tells you how often builds work correctly |
Computer Use | Helps you see if you need more powerful computers |
10. Keep Build Scripts Clean and Easy to Manage
Making build scripts easy to read and update helps teams work better. Good scripts make it easier to fix problems and change things when needed. Here's how to do it:
Use Clear Names
Give your scripts and variables names that show what they do. This helps people understand the scripts quickly. For example:
Good Names | Bad Names |
---|---|
buildJava.sh |
script1.sh |
runTests.py |
do_stuff.py |
Break Scripts into Small Parts
Split your build scripts into smaller pieces that do one job each. This makes it easier to:
- Use parts of the script again
- Find and fix problems
- Test each part on its own
Add Comments and Notes
Write comments in your scripts to explain tricky parts. Also, keep a separate file that tells how the whole build process works. This helps new team members understand the scripts.
Use Version Control
Keep your build scripts in a system like Git. This lets you:
- See what changes were made
- Go back to old versions if needed
- Work with others on the same scripts
Check and Fix Regularly
Look at your build scripts often to make sure they still work well. As your project changes, your scripts might need to change too. Regular checks help keep your scripts working right.
Why Check Scripts | How Often |
---|---|
Remove old code | Every few months |
Make sure they work with new tools | When you update tools |
Fix slow parts | If builds get slower |
Add new features | When project needs change |
Conclusion
Using build tools well is key for making good software quickly. The ten tips in this guide help developers make their build process better. These tips cover everything from picking the right tool to keeping build scripts neat.
Always Try to Get Better
Software development keeps changing. It's important to:
- Look at your build process often
- Make it better when you can
- Try new tools that might help
Work Together and Talk
Teams work best when they share what they know. This means:
- Talking about what works well
- Sharing problems they've had
- Finding new ways to fix issues together
Keep an Eye on Things
It's important to watch how your builds are doing. This helps you:
- See if builds are working well
- Find slow parts
- Fix problems quickly
What to Watch | Why It's Important |
---|---|
How long builds take | Shows if builds are getting faster or slower |
How often builds work | Tells you if your process is reliable |
Computer use | Helps you know if you need better computers |
FAQs
What are the main jobs a Build Tool should do?
Build tools help make software development easier by doing many tasks automatically. Here are the key jobs a good build tool should do:
Job | What it does |
---|---|
Turn code into programs | Changes the code developers write into something computers can run |
Run tests | Checks if the code works correctly |
Make guides | Creates instructions on how to use the software |
Get the software ready to use | Puts all the parts of the software together |
Handle outside code | Makes sure the software has all the extra bits it needs to work |
Work with other tools | Helps the software get from the developers to the users quickly |
These jobs help teams work better together and make fewer mistakes when building software.
Why are these jobs important?
Having a build tool do these jobs helps in several ways:
- Saves time by doing repetitive tasks automatically
- Reduces mistakes that can happen when people do these jobs by hand
- Makes it easier for team members to work together
- Helps get new versions of the software out faster