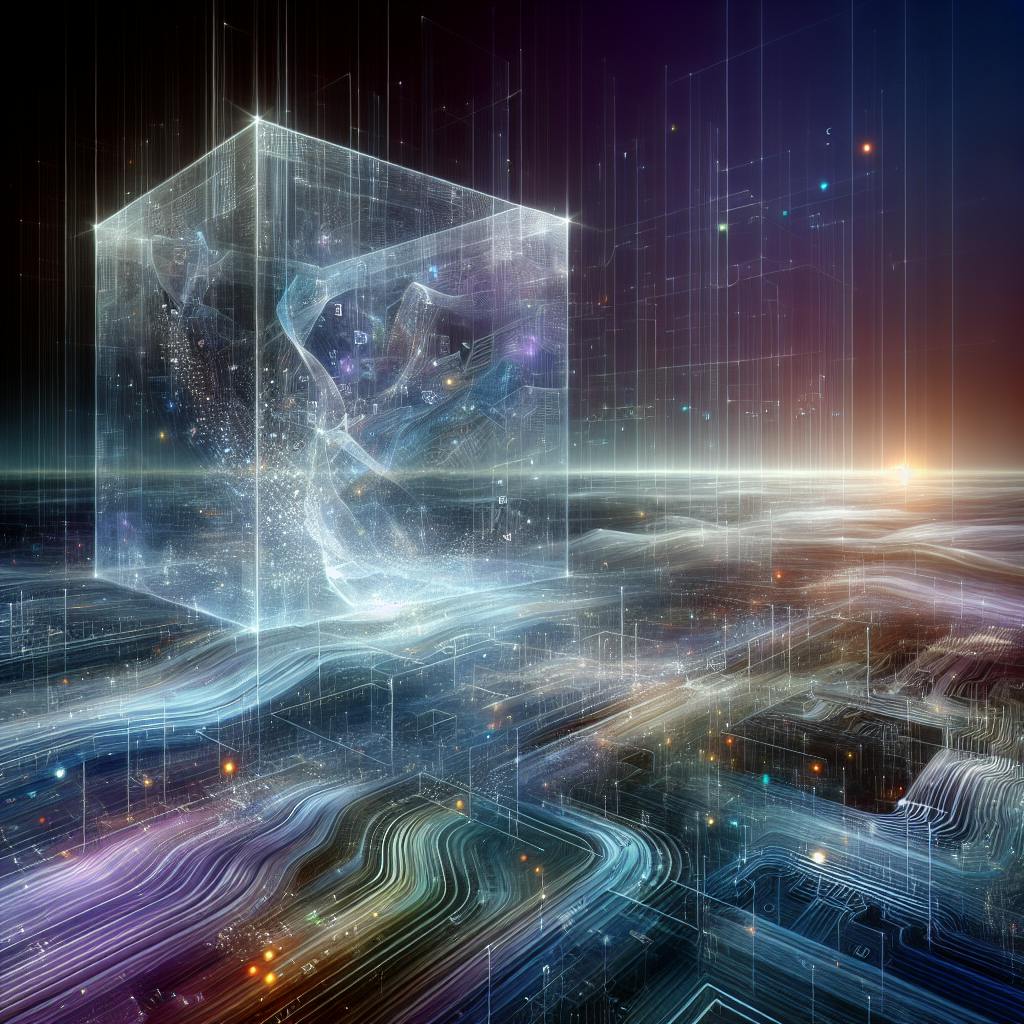
Explore the essentials of Haskell programming with this guide on HaskellWiki. Learn installation, basics, functions, and more for effective coding in Haskell.
Haskell is a statically typed and purely functional programming language that uses pure functions, immutability, and recursion to write code. HaskellWiki is a comprehensive online resource that provides in-depth documentation, tutorials, and guides for learning Haskell.
Related video from YouTube
Getting Started with Haskell
-
Install the Haskell Platform
Operating System Installation Steps Windows Download the installer from www.haskell.org/platform/ and follow the instructions. Mac OS X Run brew install haskell-stack
in Homebrew.Linux Use your distribution's package manager (e.g., sudo apt-get install haskell-platform
on Ubuntu). -
Use GHCi (Interactive Tool)
-
Open a terminal and type
ghci
to launch the GHCi interpreter. -
Enter Haskell expressions and see the results.
-
Haskell Basics
Arithmetic Operations
Operator | Operation |
---|---|
+ |
Addition |
- |
Subtraction |
* |
Multiplication |
/ |
Division |
Joining Strings
-
Use the
++
operator to concatenate strings. -
Use the
concat
function to join a list of strings.
Functions
-
Functions are defined using pattern matching.
-
Functions can be partially applied (curried).
Console Input and Output
-
Use
putStrLn
to print to the console. -
Use
getLine
to get user input.
Data Types and Structures
-
Haskell has a powerful type inference system.
-
Common data structures include lists and tuples.
-
Numeric types:
Int
,Integer
,Float
,Double
.
Defining and Using Functions
-
Use pattern matching to define multiple cases for a function.
-
Add type signatures to specify the types of function arguments and return values.
Key Syntax Features
-
Use
let
expressions to define local variables or functions. -
Use
case
expressions for pattern matching.
Using Haskell Libraries
-
The Prelude library provides essential functions and data types.
-
Import external libraries using the
import
statement.
Next Steps
-
Explore Haskell resources like HaskellWiki, Haskell.org, and "Learn You a Haskell for Great Good!"
-
Practice with exercises and projects on platforms like Exercism and CodinGame.
-
Join the Haskell community on forums, mailing lists, and IRC channels.
sbb-itb-bfaad5b
Setting Up Your Haskell Environment
To start learning Haskell, you need to set up your environment. This section will guide you through installing Haskell and setting up your development environment.
Install the Haskell Platform
The Haskell Platform is a package that includes the Glasgow Haskell Compiler (GHC), the Haskell interpreter GHCi, and essential libraries. Here's how to install it:
Operating System | Installation Steps |
---|---|
Windows | Download the Haskell Platform installer from www.haskell.org/platform/ and follow the installation instructions. |
Mac OS X | Install the Haskell Platform using Homebrew by running brew install haskell-stack . |
Linux | Install the Haskell Platform using your distribution's package manager. For example, on Ubuntu, run sudo apt-get install haskell-platform . |
Using GHCi, the Interactive Tool
GHCi is an interactive Haskell environment that allows you to execute Haskell code snippets. To access GHCi, open a terminal or command prompt and type ghci
. This will launch the GHCi interpreter, where you can enter Haskell expressions and see the results.
Here's an example of how to use GHCi:
$ ghci
GHCi, version 8.10.4: https://www.haskell.org/ghc/ :? for help
Loading package ghc-prim... linking... done.
Loading package integer-gmp... linking... done.
Loading package base... linking... done.
Prelude>
In the GHCi prompt, you can enter Haskell expressions, such as 2 + 2
, and see the results:
Prelude> 2 + 2
4
GHCi is a valuable tool for learning Haskell, as it allows you to experiment with the language and see immediate results.
Haskell Basics: Syntax and Operations
Arithmetic in Haskell
Haskell supports basic arithmetic operations like addition, subtraction, multiplication, and division. You can perform these operations using the following operators:
Operator | Operation |
---|---|
+ |
Addition |
- |
Subtraction |
* |
Multiplication |
/ |
Division |
Here are some examples of arithmetic operations in Haskell:
Prelude> 2 + 3
5
Prelude> 5 - 2
3
Prelude> 4 * 5
20
Prelude> 10 / 2
5
Note that the /
operator performs floating-point division, which returns a decimal result. If you want to perform integer division, use the div
function:
Prelude> 10 `div` 2
5
Joining Strings in Haskell
In Haskell, you can join strings using the ++
operator. This operator concatenates two strings together. Here's an example:
Prelude> "Hello, " ++ "world!"
"Hello, world!"
You can also use the concat
function to join a list of strings together:
Prelude> concat ["Hello, ", "world!"]
"Hello, world!"
Using Functions in Haskell
In Haskell, functions are a fundamental concept. You can define a function to perform a specific task. Here's an example of a simple function that takes two integers and returns their sum:
add :: Int -> Int -> Int
add x y = x + y
You can apply this function to two integers like this:
Prelude> add 2 3
5
Note that Haskell functions can also be curried, which means they can be partially applied. For example, you can apply the add
function to a single integer, which returns a new function that takes another integer as an argument:
Prelude> let addTwo = add 2
Prelude> addTwo 3
5
In this example, addTwo
is a new function that takes an integer as an argument and returns the result of adding 2 to that integer.
Console Input and Output
In this section, we'll explore how to handle console input and output in Haskell, which are essential for interactive programs.
Printing to the Console
To print to the console, you can use the putStrLn
function, which takes a string as an argument. Here's an example:
main :: IO ()
main = do
putStrLn "Running Main!"
putStrLn "How Exciting!"
When you run this code, it will print "Running Main!" and "How Exciting!" to the console.
You can also use the print
function, which is similar to putStrLn
, but it adds a newline character at the end of the string.
main :: IO ()
main = do
print "Running Main!"
print "How Exciting!"
Getting User Input
To get user input from the console, you can use the getLine
function, which returns a string. Here's an example:
main :: IO ()
main = do
putStrLn "Enter Your Name!"
name <- getLine
let message = "Hello, " ++ name ++ "!"
putStrLn message
When you run this code, it will prompt the user to enter their name, and then print a greeting message with their name.
Indentation in Haskell Code
In Haskell, indentation is important, especially when it comes to block structures like do
blocks. You need to indent your code correctly to define the scope of the block.
Here's an example:
main :: IO ()
main = do
putStrLn "Enter Your Name!"
name <- getLine
let message = "Hello, " ++ name ++ "!"
putStrLn message
In this example, the putStrLn
and let
statements are indented under the do
block, which defines their scope. If you don't indent your code correctly, you'll get a syntax error.
Remember to always use consistent indentation in your Haskell code to avoid errors and make your code more readable.
Data Types and Structures
In Haskell, you need to understand the type system and data structures to write efficient code. This section covers the basics of Haskell's type system and how to work with common data structures like lists and tuples.
Type Inference and Declarations
Haskell has a powerful type inference system, which means you don't always need to specify the types of variables. However, in some cases, you need to provide type declarations to avoid ambiguity or ensure type safety.
For example, consider the following code:
add x y = x + y
In this case, the type of add
is inferred to be Num a => a -> a -> a
, which means that add
takes two arguments of the same numeric type and returns a result of the same type.
However, in some cases, you may need to provide an explicit type declaration to avoid ambiguity. For example:
add :: Int -> Int -> Int
add x y = x + y
In this case, we've explicitly declared the type of add
to be Int -> Int -> Int
, which means that add
takes two Int
arguments and returns an Int
result.
Using Lists and Tuples
Lists and tuples are common data structures in Haskell. A list is a collection of values of the same type, while a tuple is a collection of values of different types.
Here's an example of a list:
numbers :: [Int]
numbers = [1, 2, 3, 4, 5]
And here's an example of a tuple:
person :: (String, Int)
person = ("John", 30)
You can use pattern matching to extract elements from lists and tuples. For example:
head :: [a] -> a
head (x:_) = x
firstName :: (String, Int) -> String
firstName (name, _) = name
Numeric Types in Haskell
Haskell provides several numeric types, including Int
, Integer
, Float
, and Double
. Each type has its own range and precision.
Here's a brief overview of each type:
Type | Description |
---|---|
Int |
A 32-bit or 64-bit integer type, depending on the platform. |
Integer |
An arbitrary-precision integer type, which can represent very large integers. |
Float |
A single-precision floating-point type, which has a limited range and precision. |
Double |
A double-precision floating-point type, which has a wider range and higher precision than Float . |
You can use numeric literals to create values of these types. For example:
x :: Int
x = 42
y :: Float
y = 3.14
z :: Double
z = 2.71828
Note that you can also use type conversions to convert between numeric types. For example:
x :: Int
x = 42
y :: Float
y = fromIntegral x
In this case, we've used the fromIntegral
function to convert the Int
value x
to a Float
value y
.
Defining and Using Functions
In Haskell, functions are a fundamental concept. This section covers the basics of defining and using functions in Haskell.
Pattern Matching in Functions
Pattern matching is a technique in Haskell that allows you to specify multiple alternatives for how to handle a piece of data. In the context of functions, pattern matching enables you to define multiple cases for how to handle different inputs.
For example, consider the following function that calculates the factorial of a given number:
factorial :: Integer -> Integer
factorial 0 = 1
factorial n = n * factorial (n - 1)
In this example, we've defined two cases for the factorial
function: one for when the input is 0, and another for when the input is any other integer. The first case returns 1, while the second case recursively calls the factorial
function with the input decremented by 1.
Adding Type Signatures
Type signatures are annotations that specify the type of a function. In Haskell, type signatures are optional, but they can help catch type errors at compile-time and make your code more readable.
For example, consider the following function that takes two integers and returns their sum:
add :: Int -> Int -> Int
add x y = x + y
In this example, we've added a type signature to the add
function, specifying that it takes two Int
arguments and returns an Int
value. This helps the compiler ensure that the function is used correctly and prevents type errors at runtime.
By using pattern matching and type signatures, you can write more robust and maintainable functions in Haskell. In the next section, we'll explore more advanced features of Haskell functions.
Key Haskell Syntax Features
Using 'let' and 'case'
Haskell's syntax features are designed to make your code more expressive and readable. Two essential syntax features in Haskell are the let
and case
expressions.
The 'let' Expression
The let
expression is used to define local variables or functions within a scope. It allows you to assign a value to a name, making it available for use within the current scope.
Here's an example:
let x = 5
in x + 3
In this example, x
is defined as 5
and then used in the expression x + 3
.
The 'case' Expression
The case
expression is used for pattern matching, which enables you to specify multiple alternatives for handling a piece of data. It consists of an expression followed by multiple alternatives, each with a pattern and a corresponding action.
Here's an example:
case x of
0 -> "zero"
1 -> "one"
_ -> "other"
In this example, the case
expression evaluates the value of x
and returns a string based on the pattern matched.
Combining 'let' and 'case'
You can combine let
and case
expressions to define and use variables and functions in Haskell. Here's an example:
let factorial n =
case n of
0 -> 1
_ -> n * factorial (n - 1)
in factorial 5
In this example, the let
expression defines a factorial
function, which uses a case
expression to calculate the factorial of a given number.
By mastering the use of let
and case
expressions, you can write more efficient and readable Haskell code.
Using Haskell Libraries
Haskell libraries are a powerful tool for extending the capabilities of the Haskell language. They provide a way to reuse code, simplify complex tasks, and leverage the work of other developers.
The Prelude Library
The Prelude library is Haskell's default environment, and it provides a set of essential functions and data types that are used frequently in Haskell programming. The Prelude library is implicitly imported by default, so you don't need to explicitly import it in your code.
Here are a few examples of functions in the Prelude library:
Function | Description |
---|---|
+ |
Addition |
* |
Multiplication |
head |
Returns the first element of a list |
tail |
Returns the rest of the list, excluding the first element |
length |
Returns the length of a list |
Importing External Libraries
In addition to the Prelude library, Haskell has a vast collection of external libraries that can be imported into your code. These libraries provide specialized functionality for tasks such as data analysis, web development, and more.
To import an external library, you can use the import
statement followed by the name of the library. For example:
import Data.List
Once you've imported a library, you can use its functions and data types in your code. For example, the Data.List
library provides a sort
function that can be used to sort a list of elements:
import Data.List
myList = [3, 1, 2, 4]
sortedList = sort myList
By using Haskell libraries, you can write more efficient and effective code, and take advantage of the work of other developers in the Haskell community.
Next Steps for Learning Haskell
Now that you've learned the basics of Haskell, it's time to take your skills to the next level. Here are some next steps to help you continue your Haskell journey:
Explore Haskell Resources
Here are some resources to help you learn Haskell:
Resource | Description |
---|---|
HaskellWiki | A comprehensive wiki dedicated to Haskell, featuring tutorials, guides, and documentation. |
Haskell.org | The official Haskell website, providing resources, documentation, and community forums. |
Learn You a Haskell for Great Good! | A popular online book that teaches Haskell through interactive exercises and examples. |
Practice with Exercises and Projects
Practice is key to mastering Haskell. Here are some resources to help you practice:
Resource | Description |
---|---|
Exercism | A platform offering a series of exercises to help you improve your Haskell skills. |
CodinGame | A coding game platform that allows you to practice Haskell by solving puzzles and challenges. |
Haskell for all | A blog featuring real-world Haskell tutorials and projects. |
Join the Haskell Community
Connecting with the Haskell community can help you stay motivated and learn from others. Here are a few ways to get involved:
Resource | Description |
---|---|
Haskell subreddit | A community-driven forum for discussing Haskell and getting help with problems. |
Haskell mailing lists | Subscribe to mailing lists dedicated to Haskell, such as the Haskell-Cafe list. |
IRC channels | Join Haskell IRC channels, such as #haskell on Freenode, to connect with other Haskell enthusiasts. |
By following these next steps, you'll be well on your way to becoming proficient in Haskell. Remember to practice regularly, explore different resources, and connect with the Haskell community to stay motivated and inspired.
FAQs
How to start programming in Haskell?
To get started with Haskell, follow these steps:
-
Install Haskell: Download and install the Haskell Platform from the official website.
-
Open GHCi: Open a terminal and type
ghci
to start the GHC interpreter. -
Write your first Haskell program: Start with simple arithmetic operations, string concatenation, or function calls.
Resources to learn Haskell:
Resource | Description |
---|---|
HaskellWiki | A comprehensive wiki for Haskell tutorials, guides, and documentation. |
Haskell.org | The official Haskell website with resources, documentation, and community forums. |
"Learn You a Haskell for Great Good!" | An online book that teaches Haskell through interactive exercises and examples. |
Practice and join the community:
Resource | Description |
---|---|
Exercism | A platform offering exercises to improve your Haskell skills. |
CodinGame | A coding game platform to practice Haskell by solving puzzles and challenges. |
Haskell subreddit | A community-driven forum for discussing Haskell and getting help with problems. |
Remember to practice regularly, explore different resources, and connect with the Haskell community to stay motivated and inspired.