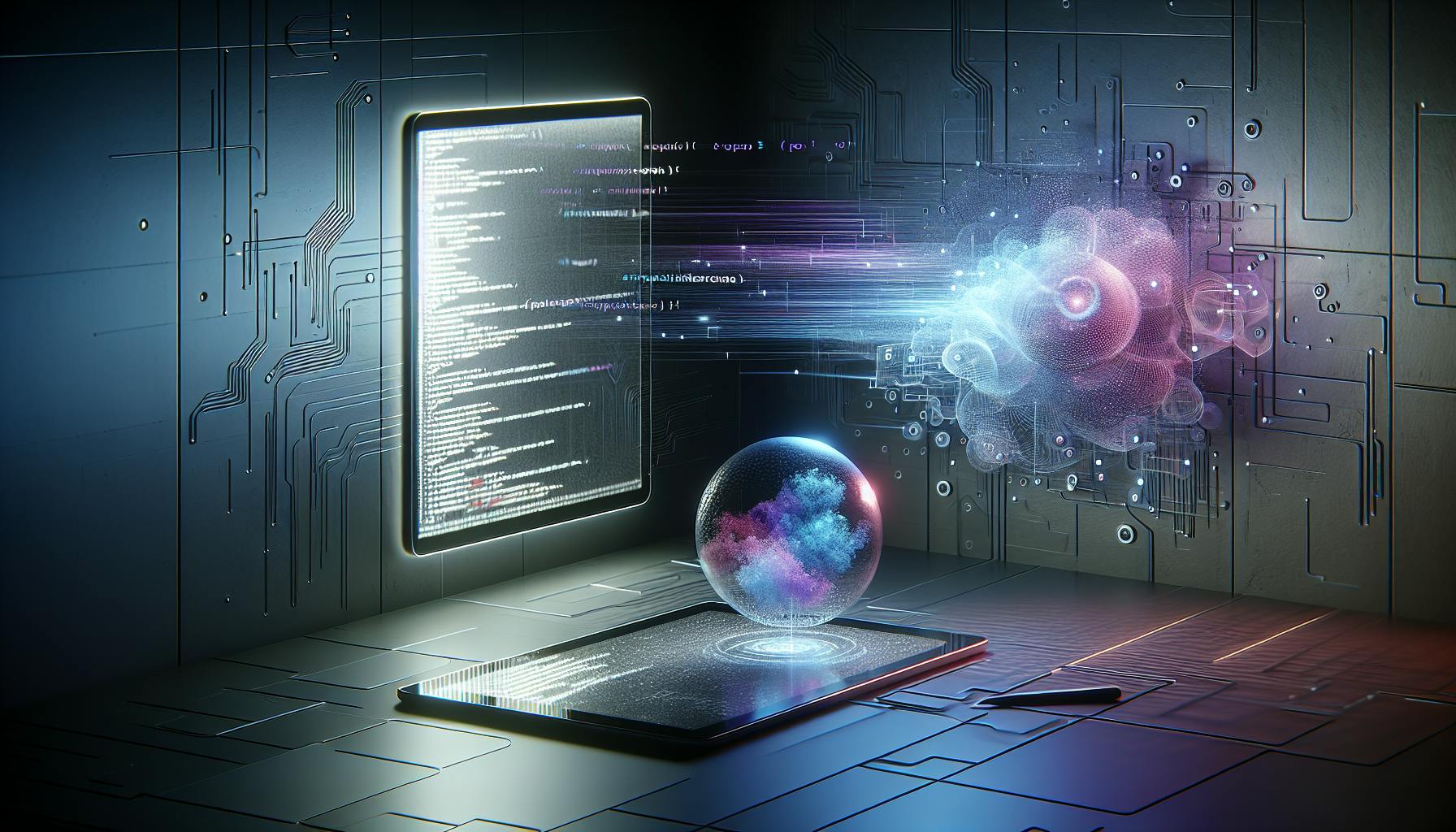
Learn about the `pop()` method in JavaScript, its usefulness for array manipulation, and how to customize it for specific needs. Explore practical applications and deep dive into its syntax and parameters.
Understanding the pop()
method in JavaScript is crucial for effective array manipulation. This method allows you to remove the last item from an array and receive it as a return value. If the array is empty, pop()
returns undefined
. Here's a quick rundown:
- What
pop()
Does: Removes and returns the last item of an array. - When to Use: Ideal for managing lists, undoing actions, or processing items in LIFO order.
- Comparison with Other Methods: Unlike
shift()
which removes the first item,pop()
targets the end. To add items,push()
appends them to the array. - Practical Applications: From simplifying chores in a to-do list to managing dynamic playlists and undo stacks.
- Customization: You can create a custom
pop()
function to handle specific needs, add callbacks, or implement undo functionality.
By mastering pop()
, you enhance your ability to manipulate arrays efficiently, tailor functionality to your needs, and understand fundamental JavaScript array operations.
Defining Arrays
You can put almost anything on your list - fruits, numbers, or even other smaller lists. To make one, you just list your items between square brackets like this:
let fruits = ['apple', 'banana', 'orange'];
This makes a list called fruits
with three items. If you want to see what the first item is, you just count starting from 0:
let firstFruit = fruits[0]; // 'apple'
A handy thing about lists is they tell you how many items they have, which makes it easier to keep track of everything.
Importance of Manipulation Methods
JavaScript gives you tools to change your lists easily. One handy tool is pop()
, which takes off the last item and shows it to you:
let removedFruit = fruits.pop();
console.log(removedFruit); // 'orange'
console.log(fruits); // ['apple', 'banana']
It's like crossing the last item off your shopping list. There are other tools too, like push()
to add items to the end, or shift()
and unshift()
to add and remove items from the start.
Knowing these tools helps you organize and update your lists without a hassle.
Deep Dive: The Pop Method
Syntax and Parameters
The pop() method is super simple to use because it doesn't need any extra information from you. Here's how you write it:
array.pop()
In this line, array
is just the name of the list you're working with.
Here's what you should remember about pop():
- It's a tool that all lists (arrays) can use
- It doesn't need any extra details from you
- It changes the original list by taking off the last item
How Pop Works
When you use pop(), it does three things: takes off the last item of a list, makes the list one item shorter, and then gives you the item it removed.
For example:
let fruits = ['apple', 'banana', 'orange']
let removedFruit = fruits.pop()
console.log(removedFruit) // 'orange'
console.log(fruits) // ['apple', 'banana']
Here, pop() took 'orange' out of the fruits list and gave it to us. The list then went from having 3 items to 2.
If you try pop() on an empty list, it won't have anything to give back, so you get undefined:
let empty = []
let removed = empty.pop()
console.log(removed) // undefined
Pop vs. Other Methods
Pop() is just one way to change a list. There are others like:
- push(): Puts items at the end of a list
- shift(): Takes off the first item, not the last
- unshift(): Puts items at the start, not the end
The main thing about pop() is it always takes off the last item.
Here's how these methods compare:
let nums = [1, 2, 3]
nums.pop() // Takes off 3 - Gives back 3
nums.push(4) // Puts 4 at the end - Tells you the new size
nums.shift() // Takes off 1 - Gives back 1
nums.unshift(5) // Puts 5 at the start - Tells you the new size
So, pop() is a handy way to take off and find out about the last item in a list.
Practical Applications
Simplifying Array Manipulation
The pop()
method makes working with lists of items, like a to-do list, a lot easier. Imagine you have a list of chores:
let todos = ['Dishes', 'Laundry', 'Vacuum', 'Mop'];
When you finish the last chore on the list, you can use pop()
to take it off:
let completedTask = todos.pop();
console.log(todos);
// ['Dishes', 'Laundry', 'Vacuum']
This way, the chore you just finished is removed from the list and you can even show a message saying it's done. It's much easier than trying to figure out how many chores are left and then removing the last one manually.
pop()
is great for when you need to take off and look at the last item in a list without much trouble.
Advanced Use Cases
The pop()
method is perfect for certain advanced uses because it works by removing the last item you added:
LIFO Stacks
pop()
fits perfectly for a LIFO (Last In First Out) stack, which is like a pile where you take off the top item first. This is handy for actions like undoing the last step in a process:
let undoStack = [];
function doAction(action) {
undoStack.push(action);
// Do action
if (tooManyActions) {
undoStack.pop(); // Undo last action
}
}
Processing Queues
pop()
can work with queues if you use it alongside shift()
, which takes off the first item added:
let printQueue = [];
function addToQueue(doc) {
printQueue.push(doc);
}
function printNext() {
let next = printQueue.shift();
print(next);
if (printQueue.length > 0) {
setTimeout(printNext, 500)
}
}
printNext();
Dynamic Playlists
pop()
is also good for managing playlists by removing songs after they play:
let playlist = ['song1', 'song2', 'song3'];
function playNext() {
let next = playlist.shift();
play(next);
if (playlist.length > 0) {
setTimeout(playNext, 5000);
} else {
console.log('Playlist complete!');
}
}
playNext();
In short, pop()
is really useful for a bunch of advanced programming needs because it lets you easily remove the last item you added.
Beyond the Basics
Creating a Custom Pop Method
Making your own version of the pop()
method is a great way to really understand how it works. Here are some reasons you might want to do this:
- To get a better grasp of how array manipulation methods work
- To add your own rules or handle errors in a specific way
- To make it work better for certain tasks
- To make sure it works in older web browsers
Here's a simple guide to making a basic pop()
method:
- Check if the array has any items by looking at
array.length
- If it's empty, return
undefined
- Save the last item in the array in a variable by using
array.length - 1
- Remove the last item by decreasing
array.length
by 1 - Give back the last item you saved
This is a pretty straightforward way to recreate the pop()
method.
Customizing Your Polyfill
Once you've made your own pop()
method, you can add more features to it:
Callback Function
You can have it run a special function every time you use pop()
:
function pop(array, callback) {
// pop logic
if (callback) {
callback(removedElement);
}
return removedElement;
}
Undo
Keep track of items you've removed so you can put them back if you need to:
let removedElements = [];
function pop(array) {
let element = array[array.length - 1];
removedElements.push(element);
// pop logic
return element;
}
function undoPop() {
array.push(removedElements.pop());
}
Error Handling
Make sure to check for mistakes, like if someone tries to pop()
something that's not an array:
function pop(array) {
if (!Array.isArray(array)) {
throw new Error('Expected an array');
}
// pop logic
}
By adding these features, you can make your pop()
method do even more!
sbb-itb-bfaad5b
Mastering Pop
Pro Tips
Before you use pop()
, it's a good idea to check if there's anything in the array. You can do this by seeing if array.length
is more than 0. This way, you won't run into issues trying to remove something from an empty array:
let fruits = [];
if (fruits.length > 0) {
let lastFruit = fruits.pop();
} else {
console.log('No fruits left!');
}
You can also mix pop()
with other methods like push()
and unshift()
to rearrange items:
let firstFruit = fruits.shift();
fruits.push(firstFruit); // Moves first item to end
Performance Considerations
Using pop()
is usually quicker than shift()
because of the way they change arrays.
pop()
just makes the array shorter, while shift()
has to move everything down one spot. So, if you're working with a lot of items, pop()
might be the faster choice.
Here's a quick test showing pop()
is more than twice as fast as shift()
:
pop x 7,161,249 ops/sec ยฑ0.94% (88 runs sampled)
shift x 3,111,765 ops/sec ยฑ1.04% (86 runs sampled)
But, this might vary depending on the JavaScript engine you're using and your specific code. If speed matters for what you're doing, try both and see which works better.
Overall, pop()
and push()
are your go-to for quick adding and removing items in an array.
Frequently Asked Questions
What is the difference between pop() and shift()?
The big difference is where they remove an item from in your list (or array). pop()
takes away the last item, and shift()
removes the first one. Here's a quick example to show you:
let fruits = ['apple', 'banana', 'orange'];
let last = fruits.pop(); // This takes away 'orange'
console.log(last); // Shows 'orange'
let first = fruits.shift(); // This takes away 'apple'
console.log(first); // Shows 'apple'
To sum it up:
pop()
gets rid of and shows you the last itemshift()
gets rid of and shows you the first item
How do I remove an element from an array by index?
If you want to remove a specific item using its position number (index), you can use splice()
. Just tell it where to start:
let fruits = ['apple', 'banana', 'orange'];
fruits.splice(1, 1); // This removes 'banana' at position 1
The splice()
method needs to know how many items to take out, so splice(index, 1)
means remove one item at the given spot.
What happens if I pop() an empty array?
If your list is empty and you try to use pop()
, it won't be able to remove anything, so it gives you undefined
. The list stays the same, empty.
For instance:
let fruits = [];
let last = fruits.pop();
console.log(last); // Shows undefined
console.log(fruits); // Shows [] (still empty)
This means pop()
can't take anything out of an empty list, so it returns undefined
, and the list doesn't change.
Conclusion
The pop()
method is really handy when you're working with lists in JavaScript. It helps you by taking off the last item from a list and telling you what it was. This is super useful for making lists shorter, undoing the last action, or going through items one by one.
Here are some quick points to remember about pop()
:
- It takes away the last item of a list and shows it to you, making the list one item shorter.
- If the list is empty and you try to use
pop()
, it won't do anything and will just sayundefined
. - It's like the
pop
action for a stack of items, where you always take from the top. - When you use it with other methods like
push()
orshift()
, you can do a lot of cool things with your lists. - It's usually faster than
shift()
because it doesn't have to move all the items around.
Making your own version of pop()
can be really useful too:
- It helps you understand how lists work better.
- You can make it do specific things you want, like checking for errors or running a special function when you use
pop()
. - You can even make it possible to undo a
pop()
if you change your mind. - It's a good way to make sure your code works even on older websites that might not support the latest JavaScript.
Getting to know simple methods like pop()
can make a big difference in how you work with JavaScript. It's part of getting better at handling data and making your programs do what you want.
If you're curious and want to learn more, here are some ideas:
- Check out other list methods like
slice()
,splice()
, orconcat()
. - Look into libraries that help with list stuff, like Lodash.
- Learn about different ways to organize data, like stacks, queues, or linked lists.
- Try making your own methods and playing around with creating new types of data.
Learning JavaScript is a journey, but knowing the basics, like how pop()
works, sets you up for success!
Related Questions
How does pop function work in JavaScript?
The pop()
method in JavaScript takes off the last item from an array and gives it back to you. If there's nothing in the array, it gives back undefined
because there's nothing to remove. This makes the array shorter by one item.
What does pop() do?
pop()
is a way to remove the last item from an array and then show you what that item was. If the array doesn't have any items, it will return undefined
. It's important to know that using pop()
changes the array by making it smaller.
What is push and pop in JavaScript?
In JavaScript, push()
and pop()
are two ways to work with arrays. push()
adds an item to the end of an array, while pop()
removes the last item. These methods are handy for managing what's in your arrays.
How to pop object from array in JavaScript?
To remove an item from somewhere in the middle of an array, pop()
and shift()
might not help because they only remove the last and first item, respectively. To get rid of an item that's not at the beginning or end, you'd need a different approach.