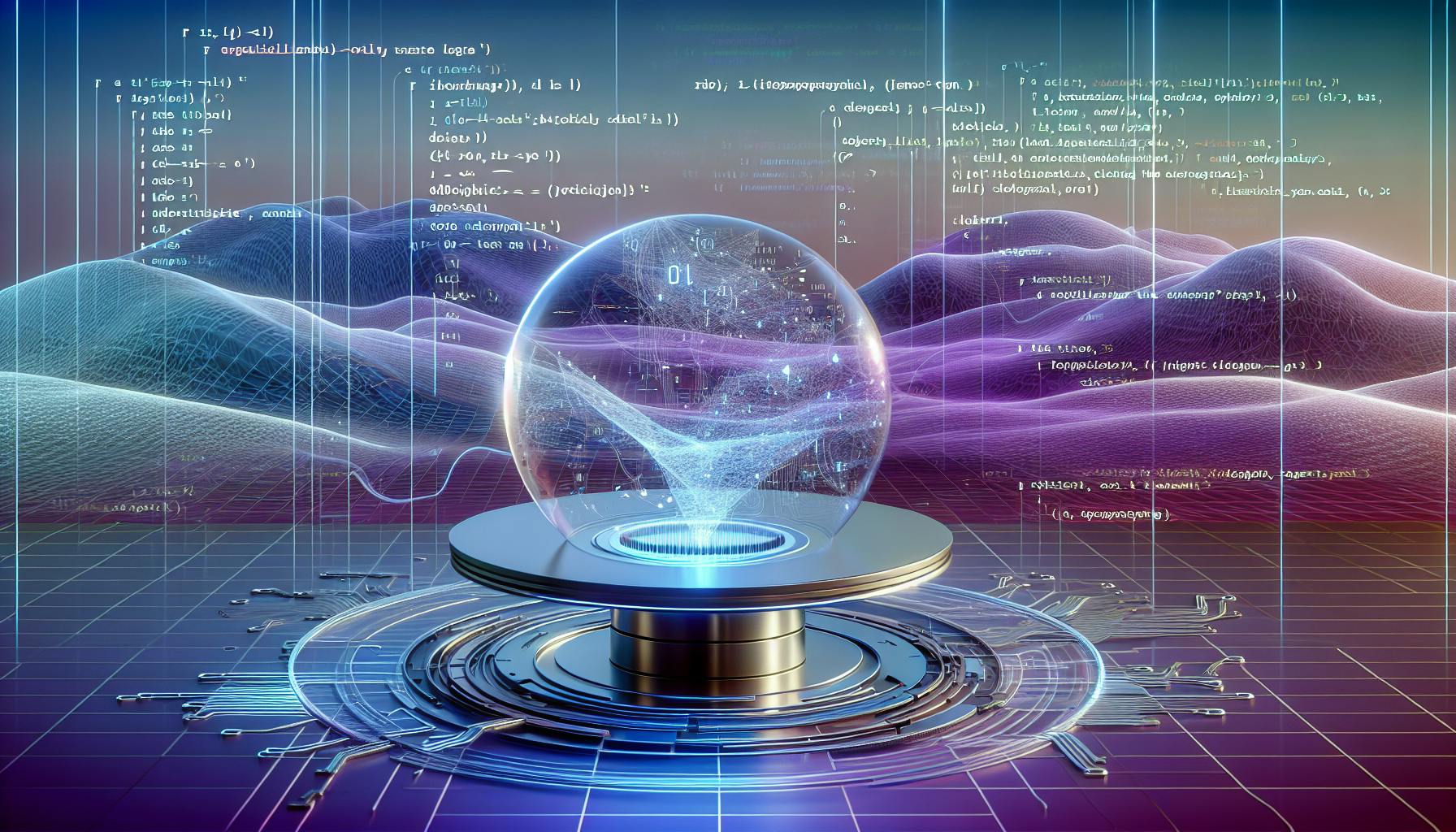
Explore the key principles of designing and creating a programming language, from purpose and readability to efficiency and extensibility. Learn about compilers, core design principles, and practical steps for developing your own language.
Creating your own programming language is a fascinating journey that involves careful consideration of several key principles to ensure it meets the needs of its users effectively. Whether you're designing a language to tackle specific types of projects or aiming for general use, understanding the core elements is crucial. Here's a quick overview:
- Purpose: Identify the main problem your language aims to solve and its target audience.
- Readability and Expressibility: Ensure the language is easy to understand and expressive enough to allow programmers to convey ideas clearly.
- Efficiency and Scalability: Optimize for performance and ensure it can handle large-scale projects.
- Human-Centric Design: Focus on making the programming experience enjoyable and intuitive.
- Extensibility: Allow for growth and community contributions to keep the language evolving.
This guide will dive deeper into these principles, offering insights into the basics of programming languages, the role of compilers, and practical steps to take when creating your own language.
What is a Programming Language?
Think of a programming language as a way to chat with computers. It's how developers tell a computer what to do. The computer then turns this into actions it can understand and carry out.
Here are some basics:
-
Syntax - These are the rules on how to write your code. For example, some languages want you to put a semicolon (;) at the end of each instruction.
-
Semantics - This is about what the bits of your code actually do. It's the meaning behind the commands.
-
Variables - These are like boxes where you can store information. You can put things in, take things out, or check what's inside.
-
Data types - This tells you what kind of data you can put in your variables, like numbers or text.
-
Expressions and operators - These are the tools to do math or compare things in your code.
-
Control flow statements - These are instructions that decide what happens next, like making a choice or repeating actions.
-
Comments - These are notes for people reading the code. The computer ignores them, but they're super helpful for humans.
Overall, programming languages make it possible for humans to write instructions that computers can follow. The way a language is designed affects how easy it is to use.
The Role of Compilers
Compilers are like translators. They take the code you write in a human-friendly way and turn it into something the computer can understand and do.
Here's what they do:
-
They change your code into a more basic form that the computer can work with directly.
-
Before translating, they check your code to make sure there are no mistakes. This helps find problems early.
-
They make the code run faster and more efficiently.
-
Some languages that need compilers are C, C++, and Rust. Other languages, like JavaScript and Python, work differently and don't always need them.
-
The simpler your programming language is, the easier it is to make a compiler for it.
So, compilers help turn your ideas into actions a computer can perform. When you create your own programming language, thinking about how to make a good compiler is important.
Core Design Principles
When you set out to create your own programming language, it's really important to first think about what you want it to do and who will use it. Ask yourself what problems it will solve and how it will make coding easier or better for people.
1. Purpose and Problem Solving
- Think about what you want your language to be great at. Is it for all kinds of programming, or just for certain areas like making websites or doing science stuff?
- Look at what's annoying or hard in other languages and try to do it better in yours. This could be making errors easier to find, working on many different devices, or making programs run faster.
- Decide where your language can run. Will it work on computers, phones, or web browsers?
- Keep in mind who will use your language. Is it for pros, students, or just for fun? Make sure it fits their needs.
Knowing what you want your language to do helps make sure every part of it works towards that goal.
2. Readability and Expressibility
Making code easy to read and write is super important. You want people to easily get what the code does and be able to put their ideas into code without a headache.
Some tips include:
- Use familiar ways of writing code so it feels natural.
- Name things clearly so everyone knows what they do.
- Use spaces and lines to make code neat and easy to follow.
- Let people add notes and explanations in their code.
- Make it simple to use parts of code again or to use tools made by others.
- Give clear errors that help find and fix problems.
Keeping code clean and predictable makes it friendlier for everyone.
3. Efficiency and Scalability
You want your language to not only run fast but also be able to handle big projects as they grow.
- Choosing between a compiler or an interpreter can affect speed.
- The way you organize data and solve problems can save memory and make things quicker.
- Making your language good at doing many things at once or working across many computers helps it scale up.
- Being strict about what kind of data can be used can catch mistakes early but might limit flexibility.
- How your language deals with unused data can make coding easier.
Thinking about these things from the start is better than trying to fix them later.
4. Human-Centric Design
Remember, real people will use your language, so make it as easy as possible for them.
- Use abstraction to keep things simple.
- Break big problems into smaller pieces.
- Hide complicated stuff behind simpler interfaces.
- Make it easy to reuse code.
- Provide tools for seeing how code works and finding bugs.
- Make sure your language is forgiving when people make mistakes.
Focusing on making coding a pleasant experience will make people want to use your language.
5. Extensibility and Community Building
Allowing your language to grow and improve over time is key.
- Make it easy to add new features or tools.
- Work well with other software.
- Have a library of ready-to-use code for common tasks.
- Let people modify your language without breaking it.
Building a community around your language helps it get better and supports the people using it. Sharing ideas and working together makes everyone's experience better.
With clear goals, a focus on making coding easier, and support from a community, your language has a great chance of success.
Practical Steps to Creating Your Programming Language
Define the Grammar
The first thing you need to do is set up the rules for how code in your language should look. This is called the grammar. It includes:
- What symbols and words can be used.
- How to put those symbols together to make something the computer understands.
- Rules for doing math and setting up conditions.
- How to declare variables.
- The way to write if statements and loops.
- How to handle errors.
Tools like ANTLR can help with this. Your goal is to make these rules clear, so they're easy to follow and don't cause confusion. Look for guides on how to create programming language grammar for more help.
Develop the Compiler
Once you have your rules set, you need to make a compiler. This is the tool that turns the code written in your language into something a computer can run. The main parts include:
- Lexer - Reads the code and breaks it down into pieces.
- Parser - Makes sure the code fits the grammar and organizes it in a tree structure.
- Semantic analyzer - Adds extra info like data types.
- Intermediate code generator - Turns the organized code into a simpler form.
- Optimizers - Makes the code run faster.
- Code generator - Turns the optimized code into machine code the computer can use.
You can find step-by-step guides on building a compiler. Using existing tools can make this easier. The main aim is to translate your language's code into machine code efficiently.
Test and Iterate
Now, test your compiler:
- Try it with correct code to see if it works as expected.
- Use wrong code to check if it finds errors.
- Check how fast it runs and how much memory it uses.
- Get feedback from people who try it.
You'll find bugs, but that's normal. Use the feedback to make your language better. Keep improving it based on what users say. Testing and updating are key to making a programming language that people like using.
sbb-itb-bfaad5b
Conclusion
Making your own programming language is a big project. It's all about thinking through how your language should work and making it easy for people to use. Keeping the people who will use your language in mind will help you create something that really helps them.
Here are some key points to remember as you start this project:
- Start with the basics. First, make a simple version of your language that works well with just the basic features. Try it out, see what people think, and then slowly add more to it.
- Keep it simple. Always look for ways to make things easier for the people writing code. Using simple ideas to hide complicated stuff is very important.
- Write good guides. Spend time on tutorials, how-tos, and examples. Making it easy for people to start using your language is crucial.
- Share your work early. When you share what you're making, you get help and ideas from others. Working together often leads to better results.
- Make it enjoyable to use. Think about small details like clear error messages, smart suggestions, and easy-to-use designs. These things make people trust and like your language more.
- Plan for the future. Think about how your language can handle more work over time. Make sure it can do many things at once and work with other software easily. Adding new features should also be straightforward.
Creating a programming language is a journey that will challenge and inspire you. The goal is to make a new tool that helps developers. See this as a chance to explore and be creative. And most importantly, enjoy the process!
Related Questions
What are the principles of language design?
When creating a programming language, think about these important ideas:
- Simplicity: The language should be straightforward. This makes it easier to use and less likely to have mistakes.
- Security: Having safety features like checking for errors can protect users.
- Fast translation: A compiler can make your language work faster than an interpreter.
- Efficient object code: Using smart ways to make the final computer-readable code run faster is good.
- Readability: It's important that the code tells clearly what it does. Using names that make sense and organizing code well helps a lot.
Remember, keeping things simple is often best.
How to design your own programming language?
Here's a basic plan:
- Decide what you want your language to do and who it's for.
- Create rules for how to write code in it.
- Make tools that check and organize code.
- Give meaning to your code structures.
- Add features for managing data.
- Build a compiler or interpreter.
- Help users learn your language with guides.
- Listen to user feedback to make your language better.
Start with easy stuff and improve as you go.
What are the principles of programming languages?
These principles help shape a good programming language:
- Keeping things simple and consistent.
- Having essential tools like if statements and loops.
- Choosing data types that make coding flexible.
- Making sure code is easy to read and understand.
- Handling errors smartly.
- Being clear about what each part of the code does.
These ideas help make languages that are powerful yet easy to use.
What is the design of a programming language?
Designing a programming language includes:
- Setting up rules for writing code.
- Deciding what each part of the code means.
- Picking the right types of data.
- Choosing how to move through the code.
- Figuring out how to deal with mistakes.
- Making sure the code is easy to read.
- Adding features to help with coding big projects.
- Creating tools to help check and run code.
The goal is to make a language that lets people express their ideas clearly and works well.