
In this post, I want to share my insights about how I built a small service called Langauge (it's not a typo, thank you Grammarly) with the Injex Framework and Injex Express Plugin.
With Langauge, you add a colorful badge of gauges displaying the programming languages you used in a particular GitHub repository. You can add it to your markdown files or your website, share it as an image on social media and even print it and put it on the wall! ๐
The Motivation
Every GitHub repository has a colorful language bar at the right panel of its home page. For example, here is the language bar of Quickey, another open-source project of mine.
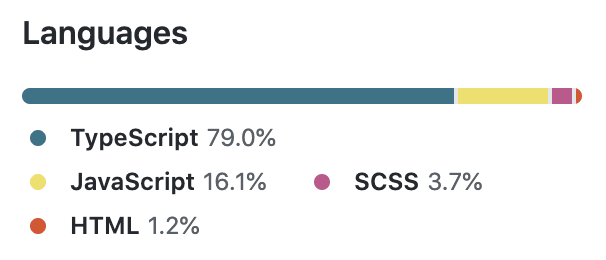
So why bother and create Langauge, you ask? Well, because I want to show off my skills in some other places, of course :)
Let's take Quickly for another example, Quickey is a module that can be installed via NPM. Please go and check it out for a second...
Welcome back! Have you noticed something is missing there? Right! It's the colorful language bar we saw earlier! It's a GitHub feature, so we can't see it on the project's NPM page nor any other place outside the repository's home page.
So here's ladies and gentlemen, the motivation!
Roadmap
So, with that motivation in mind, let's meet the tools we're going to use to create this colorful ballet.
Data
To display these gauges, we need a way to fetch the number of bytes partitioned by a given GitHub repository's programming languages. This is the easy part since we are dealing with GitHub repositories here. The GitHub API is the place to look at, and guess what? GitHub already thought about us and has a great resource just for that. Fetching repository bytes, partitioned by its programming languages, is easy as sending a GET request to https://api.github.com/repos/quickey/quickey/languages.
The response is:
Colors
Colors are the smiles of nature โ Leigh Hunt
Each language in GitHub's repository language bar has a unique color. For example, JavaScript's color is lightish yellow (#ECE066), CSS's is dark purple (#503F7A). Can you see the problem here? How many programming languages and technologies do you know? Moreover, how many there are? My guess is it's too much. Again with the help of GitHub, quick research, and I found this repository called Linguist.
Linguist's source code includes a YAML file with all the languages and technologies ever known to GitHub with some metadata on each of them, including a unique color! I created a simple script, so it's easy to fetch that YAML, convert it to JSON, and save it as a module within my source code.
The Gauge
Now that we have the data and the colors, we can go and create our gauges!
A few weeks ago, I started playing with the Sketch App. One thing I like about Sketch is the ability to create vector shapes and export them as SVG.
Opening Sketch, creating some vectors, adding some text, and after 10 minutes, I had this nice gauge!

After exporting this gauge to SVG and cleaning it a little bit, I ended up with the following code:
From static SVG to dynamic PNG
I like SVG because it stands for Scalable Vector Graphics, which means I can take the gauge SVG, resize it to huge dimensions, and the quality remains the same as in its original size. Another thing is that SVG is made of pure and readable XML. Just like in HTML, each shape or label is created with a markup element.
The idea is to take this SVG markup and change it so that I can set the language, percentage, color, and gauge rotation dynamically. After that, I need to take this SVG and convert it into an image format like PNG.
Since I'm using JavaScript and NodeJS, a quick search in the NPM registry and I found Sharp, a library that takes an SVG as an input and converts it into various image formats and sizes.

Connecting the dots
After we met the tools we're going to use for developing the Langauge service, Let's explore the edges and see how we can put together these vertices.
Choosing the right web framework
As I said earlier in this post, I use NodeJS as the backend for the Langauge service. I used to work with Express as a web framework. Still, I felt that something is missing. This is why I created Injex, a dependency-injection framework for TypeScript applications.
Injex includes a dependency-injection IoC container powered by a plugin system, so you can use Injex plugins or, if you like, create your own plugins.
The Injex Express Plugin makes express application development look and feel more elegant.
Our service has only one endpoint, the one that gets a repository owner and name and responds with a colorful gauges image of the programming languages used on the repository. I won't go over the entire source code in this post, and you can go and read it by yourself. Instead, I'll cover the service domain parts.
The request model
Each request to Langauge can be customized with options like disable colors, set the number of columns, and more. I'm going to use TypeScript interfaces to describe the request model.
Controller
The controller handles each incoming GET request to /:owner/:repo. The render method receives the express Request and Response arguments and passes the model to the manager to render the image.
We define the LangaugeController class as an Injex Controller by using the @controller() decorator. The render method is defined as a @get() handler for the /:owner/:repo route with the RequestValidationMiddleware as the request validator middleware. If the validator fails, an error returns to the client.
We then invoke the generate method of the language manager with the request model, and the result is sent to the client as an image.
The generate method receives the git owner and repo with the rest of the model options as arguments. In line 3, we take the renderer creator from a dictionary of creators, each renderer creator in the rendererCreators dictionary is indexed by the type option. Line 5 fetches the repository languages from the GitHub API, as we saw earlier in the roadmap section. We then use the threshold option to filter out any language usage percentage below this value. In line 15, the render method is invoked and returns a bitmap buffer, which then returned from the generate method.
SVG Template
Before we can render, we need a template for the SVG to change it dynamically and compile it with different data. I'm using Handlebars for this task. Handlebars provide the power necessary to let you build semantic templates effectively with no frustration. So I took the SVG generated from Sketch as we saw at the roadmap, and converted it into this Handlebars template:
As you can learn from this template file, we are going to compile it with this schema:
Wrapping everything up
Now let's take a look at the renderer's code to see how it takes our handlebars template and converts it into an image of colorful gauges.
Upon creation, the renderer receives the original options from the request model, the total bytes for each language, the object key is the language name, and the value is the number of bytes from the total bytes.
First, I need to take this object and convert it into an array of languages with more properties like the color and the percentage of total bytes. The code is straight forward. I'm using Lodash reduce to convert the JSON object to an array:
Now that I have the array of languages with colors and percentages, I can compile the handlebars template.
I need to hydrate each one of the languages with translateX, translateY, and rotation properties. The hydrateRendererLanguages method calculates the position and rotation of each gauge in the SVG.
As you can see, I'm using the Lodash chunk function to create a matrix of columns and rows based on the columns option (line 3). The default value is the number of languages, so we get only one row if there is no value for columns.
In lines 7 and 9, I'm iterating the matrix to calculate the translations. Remember the gauge I created in Sketch? Its needle points to the north at 0ยฐ. I need to translate it so that 0% = -135ยฐ and 100% = 135ยฐ, so the rotation value is calculated in line 15. The translations of X and Y are pretty simple, and both are calculated in lines 16 and 17, respectively.
Let's get back to the renderer. Now that we have the hydrated languages array, we need to compile the template and send it to Sharp to do the rest.
The results
To see the final result, go to https://badge.langauge.io/:OWNER/:REPO to see your project Langauge badge!
For example, here is the Langauge badge for Injex:
https://badge.langauge.io/uditalias/injex
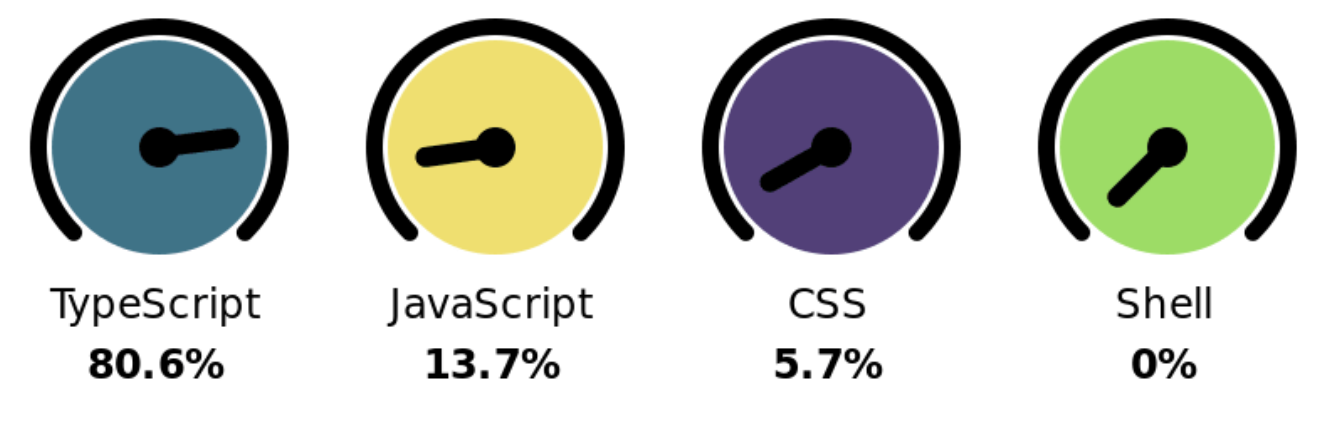
Summary
So, to sum it up, we just saw how to combine the data from GitHub API, the colors from Linguist (an open-source project by GitHub), minimal Sketch skills, and the Sharp library to create a colorful badge of gauges. We then saw how to build a server with the Injex Framework to manage the application modules and dependencies.
I hope you enjoyed the way I built this service. I suggest you go and check out the source code repository. I'll appreciate your feedback about the service and be grateful for any contribution.
For convenience, you can test a live working version of this service in this Codesendbox:
Happy Coding!