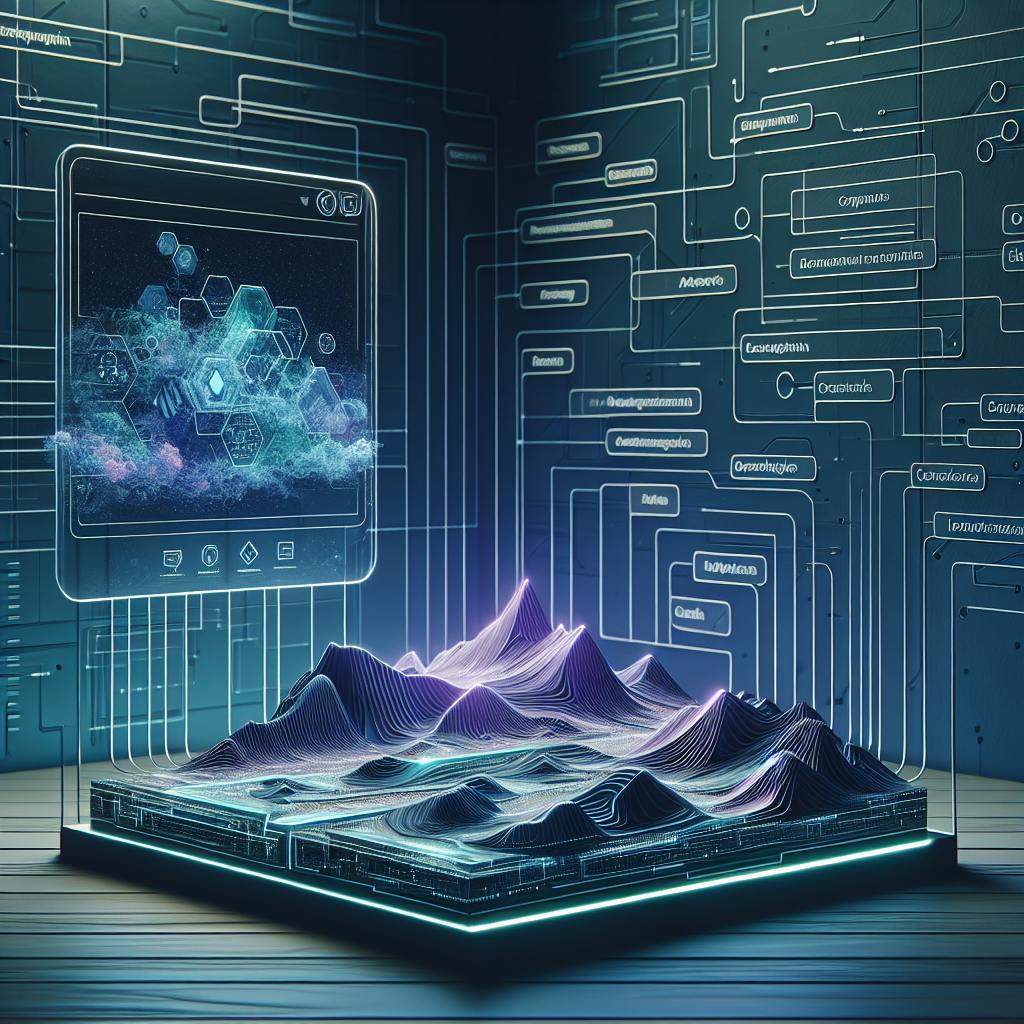
Discover essential best practices for building scalable Node.js microservices in 2024, from modular design to performance optimization.
Want to build scalable, efficient Node.js microservices? Here's what you need to know:
- Break your app into small, focused modules
- Use containers (Docker) and orchestration (Kubernetes)
- Implement service discovery and API gateways
- Secure communication with encryption and authentication
- Give each service its own database
- Add circuit breakers to prevent cascading failures
- Use asynchronous communication (events and message queues)
- Set up centralized logging and monitoring
- Automate testing at all levels
- Optimize performance with caching and load balancing
Quick Comparison:
Practice | Key Benefit | Tool Example |
---|---|---|
Modular design | Easier updates | - |
Containerization | Consistent environments | Docker |
Service discovery | Easy scaling | Consul |
Secure communication | Data protection | JWT, OAuth2 |
Database per service | Independent data | MongoDB |
Circuit breakers | Fault tolerance | Opossum |
Async communication | System resilience | RabbitMQ |
Centralized logging | Faster debugging | Winston |
Automated testing | Catch bugs early | Jest |
Performance optimization | Faster response times | Redis |
These practices help build robust, scalable Node.js microservices. Companies like Netflix, Uber, and Amazon use these techniques to handle millions of users daily.
Related video from YouTube
1. Build for Growth
Think of Node.js microservices as Lego blocks for your app. Here's how to make them strong:
Break into Modules
Split your app into small, focused parts. Each part should do one thing well.
Netflix did this. They broke their big app into 700+ microservices. The result?
- Faster bug fixes
- Easier feature updates
- Millions of users, no crashes
Make Services Stateless
Stateless services don't remember past requests. This makes scaling a breeze.
Why it works:
Benefit | How |
---|---|
Easy scaling | Just add servers |
No data loss | Data isn't tied to one server |
Better speed | Less work per server |
Amazon's shop uses this. It's how they handle crazy Black Friday sales without breaking.
Spread the Load
Don't overwork one server. Use load balancers to share tasks.
Node.js shines here. It can juggle lots of connections at once.
Uber uses Node.js for millions of daily rides. They spread the work across many servers to keep things smooth.
"Node.js lets us handle many connections with little overhead on one process." - Matt Ranney, ex-Chief Systems Architect, Uber
2. Find Services Easily
In a microservices setup, services need to find each other fast. Here's how:
Use Service Lists
Think of a central service list as a phone book for your microservices. It includes:
- Service names
- Locations (URLs or IPs)
- Service functions
When one service needs another, it checks this list.
Update Service Info Live
Services change. They move, scale up, or go offline. Your list needs to keep up.
Use tools that update service info in real-time. This way, services always know where to find each other.
Here's how it works:
- New service starts: "I'm here!"
- Other services can find it right away
- If it stops, it's removed from the list
This keeps everything running smoothly as your system grows.
Compare Service Finding Tools
Several tools can help with service discovery. Here's a quick look at three popular options:
Tool | Key Features | Best For |
---|---|---|
Consul | Health checks, Multi-datacenter support | Large, complex systems |
etcd | Simple key-value store, Fast | Smaller setups, Kubernetes |
Zookeeper | Strong consistency, Used by Hadoop | Big data applications |
Pick the tool that fits your needs. Consider:
- System size
- Service change frequency
- Team experience
3. Keep Service Talk Safe
In Node.js microservices, secure communication is crucial. Here's how to do it:
Use Strong Encryption
SSL/TLS is a must. It's like a secret code for your data:
- Stops eavesdropping
- Prevents data tampering
- Verifies sender identity
To set it up:
- Get SSL/TLS certificates
- Use HTTPS for all service communication
- Keep certificates updated
Check Who's Talking
Encryption isn't enough. You need to verify identities. Enter JWTs and OAuth2:
Tool | Function | Advantage |
---|---|---|
JWT | Service ID | Quick verification |
OAuth2 | Login management | Wide compatibility |
Set Up an API Gateway
Think of an API Gateway as your security guard. It:
- Monitors incoming traffic
- Blocks suspicious requests
- Controls service access
Adam Gola, TSH's QA Engineer, says:
"Enforce HTTPS across your app. Encrypt sensitive info ASAP, and decrypt it as late as possible. Never send it in plain text."
An API Gateway might seem like extra work, but it's worth it. It's easier to manage one entry point than securing each service individually.
4. Use Containers
Containers are a big deal for Node.js microservices. They pack your code and its environment together, so it runs the same everywhere.
Docker for Services
Docker's the top choice for containerizing Node.js microservices. It bundles your app and what it needs into one neat package.
Here's a simple Dockerfile for a Node.js app:
FROM node:14-alpine
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 3000
CMD ["npm", "start"]
This does a few things:
- Uses a small Node.js image
- Sets up where to work
- Installs what's needed
- Copies your code
- Opens a port
- Starts your app
Manage with Kubernetes
Kubernetes takes container management up a notch. It's like a boss for your containerized team.
Kubernetes can:
- Add or remove containers as needed
- Spread traffic around
- Restart containers that fail
Fun fact: 88% of companies use Kubernetes to manage their containers.
Why Containers Help
Containers fix common microservices issues:
Problem | How Containers Help |
---|---|
"Works on my machine" | Same setup everywhere |
Scaling troubles | Easy to copy and spread out |
Resource fights | Keeps processes separate |
Deployment pain | Makes it simple and repeatable |
Docker and Kubernetes make a great team for Node.js microservices. They keep your services movable, growable, and easy to handle.
5. Watch and Record Well
Monitoring your Node.js microservices is crucial. Here's how to do it right:
Collect Logs in One Place
Scattered logs? Nightmare. Centralize them. It's faster, easier, and less headache-inducing.
Why? Quicker bug fixes, easier pattern spotting, and less time wasted.
Use Winston for log gathering:
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
format: winston.format.json(),
defaultMeta: { service: 'user-service' },
transports: [
new winston.transports.File({ filename: 'error.log', level: 'error' }),
new winston.transports.File({ filename: 'combined.log' }),
],
});
This sets up Winston to log errors and other info separately.
Track Requests Across Services
Need to follow requests between services? Use a unique ID for each.
Zipkin's great for this. Here's how to add it to Express:
const express = require('express');
const { Tracer } = require('zipkin');
const zipkinMiddleware = require('zipkin-instrumentation-express').expressMiddleware;
const tracer = new Tracer({ /* config */ });
const app = express();
app.use(zipkinMiddleware({ tracer }));
This middleware adds tracing to your Express app. Spot issues faster.
Use Tools to Check Speed
Slow services? App killer. Watch performance in real-time.
Try Prometheus and Grafana. Here's a basic Prometheus setup:
const express = require('express');
const promClient = require('prom-client');
const app = express();
const collectDefaultMetrics = promClient.collectDefaultMetrics;
collectDefaultMetrics({ timeout: 5000 });
app.get('/metrics', (req, res) => {
res.set('Content-Type', promClient.register.contentType);
res.end(promClient.register.metrics());
});
This creates a /metrics
endpoint for Prometheus to scrape data from.
sbb-itb-bfaad5b
6. Give Each Service Its Own Database
In Node.js microservices, data management is crucial. Here's the lowdown:
Separate Data, Happy Services
Each microservice needs its own database. It's like giving each kid their own room - no fighting over toys.
Here's how to hook up a MongoDB database to your Node.js microservice:
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost/userService', {
useNewUrlParser: true,
useUnifiedTopology: true
});
const db = mongoose.connection;
db.on('error', console.error.bind(console, 'connection error:'));
db.once('open', () => console.log('Connected to UserService database'));
Choose Your Database Wisely
Different services, different needs. Pick a database that fits:
Service Type | Database Choice | Why? |
---|---|---|
User Profiles | MongoDB | Flexible for varied user data |
Product Catalog | PostgreSQL | Keeps inventory consistent |
Logging | Elasticsearch | Quick log searches |
Caching | Redis | Speedy in-memory access |
Keeping Data in Sync
Separate databases can make data consistency tricky. Here's how to handle it:
1. Saga pattern: Break big operations into smaller, reversible steps.
2. Event-driven updates: One service changes data, then shouts it out to others.
Check out this event emission example:
const EventEmitter = require('events');
class OrderService extends EventEmitter {
createOrder(order) {
// Order creation logic
this.emit('orderCreated', order);
}
}
const orderService = new OrderService();
orderService.on('orderCreated', (order) => {
// Update inventory, notify shipping, etc.
});
This lets other services react to changes without direct database access.
"Data consistency between distributed data stores can be a real headache. It requires a whole new way of thinking about app design." - Dilfuruz Kizilpinar, microservices guru
7. Add Circuit Breakers
Circuit breakers are safety switches for your microservices. They stop problems from spreading and keep your system running.
How They Work
Circuit breakers watch your service calls. If too many fail, they "open" and stop sending requests to the problematic service. Instead, they return a preset response or error.
They have three states:
State | Description | Action |
---|---|---|
Closed | All good | Requests flow |
Open | Service failing | Requests blocked |
Half-Open | Testing recovery | Limited requests |
When open, the circuit waits before trying again. This gives the service time to recover.
Tools for Node.js
For Node.js, you've got options:
- Opossum: Easy to use.
- Hystrix: From Netflix, now community-run.
- Resilience4j: Lightweight fault tolerance.
Here's Opossum in action:
const circuitBreaker = require('opossum');
const axios = require('axios');
const breaker = new circuitBreaker(axios.get, {
timeout: 3000,
errorThresholdPercentage: 50,
resetTimeout: 30000
});
breaker.fire('http://api.example.com/data')
.then(console.log)
.catch(console.error);
"Circuit breakers are a must-have for microservices. They're the difference between a hiccup and a system meltdown." - Martin Fowler, Software Architect
Circuit breakers are key for robust microservices. They prevent cascading failures and keep your system resilient.
8. Talk Without Waiting
In microservices, speed is king. Let's see how to make your services chat without slowing each other down.
Use Events to Share Info
Events are like gossip for services. Here's the scoop:
- A service does something cool
- It shouts about it
- Other services listen for juicy news
- They act when they're good and ready
This keeps everyone moving. In Node.js, you can use EventEmitter:
const EventEmitter = require('events');
class OrderService extends EventEmitter {
placeOrder(order) {
// Do the thing
this.emit('orderPlaced', order);
}
}
const orderService = new OrderService();
orderService.on('orderPlaced', (order) => {
console.log('Got a new order:', order);
});
orderService.placeOrder({ id: 123, item: 'Book' });
Set Up Message Queues
For bigger setups, try message queues like RabbitMQ. They're like a post office for your services.
Here's a taste using RabbitMQ with Node.js:
const amqp = require('amqplib');
async function sendMessage() {
const connection = await amqp.connect('amqp://localhost');
const channel = await connection.createChannel();
const queue = 'tasks';
const message = 'New task';
await channel.assertQueue(queue);
channel.sendToQueue(queue, Buffer.from(message));
console.log('Sent:', message);
setTimeout(() => connection.close(), 500);
}
sendMessage();
This lets services drop off messages and keep moving.
Why Not Waiting Rocks
Not waiting makes your system:
- Faster: No twiddling thumbs
- Tougher: One service down? No problem
- Flexible: Change stuff without breaking everything
Approach | Pros | Cons |
---|---|---|
Direct calls | Easy setup | Services get clingy |
Events | Quick, independent | Can be a pain to debug |
Message queues | Solid, scalable | More moving parts |
9. Test Automatically
Testing microservices isn't easy. But with smart tactics, you can catch bugs early. Here's how to test your Node.js microservices effectively:
Test Small Parts
Start with unit testing. It's like checking each ingredient before cooking.
Here's a quick Jest example:
const sum = (a, b) => a + b;
test('adds 1 + 2 to equal 3', () => {
expect(sum(1, 2)).toBe(3);
});
Keep tests small and focused. They'll run faster and be easier to fix.
Test Service Interactions
Next, check if your services play well together. This is integration testing.
For a user service and order service, you might do:
test('user can place an order', async () => {
const user = await userService.create({ name: 'Alice' });
const order = await orderService.create({ userId: user.id, item: 'Book' });
expect(order.userId).toBe(user.id);
});
This ensures your services work as a team.
Automate Your Testing
Set up automatic testing. It'll catch issues fast.
Quick steps:
- Use a CI/CD tool (Jenkins, GitHub Actions)
- Run tests on every code push
- Only deploy if tests pass
Here's how often companies typically run tests:
Test Type | Frequency |
---|---|
Unit | Every push |
Integration | Daily |
End-to-end | Weekly |
The goal? Catch bugs early when they're cheaper to fix.
"At Netflix, we run over 150,000 tests per day in our build pipeline. It lets us deploy confidently multiple times daily", says a Netflix engineer.
10. Make Services Fast
Speed matters for Node.js microservices. Here's how to boost it:
Cache Smart
Caching cuts slow database queries and API calls. It's like keeping your go-to tools close by.
Try in-memory caching:
const cache = require('memory-cache');
function getData(key) {
const cachedData = cache.get(key);
if (cachedData) return cachedData;
const data = fetchFromDatabase();
cache.put(key, data, 60000); // 1 minute cache
return data;
}
For bigger apps, Redis is your friend. It's fast and works across servers.
"Caching slashed our API call time from 2 seconds to 95 milliseconds", says a PaymentsOS engineer.
Balance the Load
Don't overwork one server. Spread tasks out.
Use NGINX as a reverse proxy. It's your traffic cop for servers.
NGINX perks:
Feature | Benefit |
---|---|
Load balancing | Spreads requests |
Caching | Stores common responses |
SSL termination | Handles HTTPS |
"NGINX nearly doubled our speed from 900 to 1600 requests per second", reports an NGINX, Inc. case study.
Optimize Node.js
Tweak Node.js for extra oomph:
- Set heap limits with
--max-old-space-size
- Enable DNS caching
- Keep connections alive in request.js
For heavy lifting, try worker threads:
const { Worker } = require('worker_threads');
function runWorker(data) {
return new Promise((resolve, reject) => {
const worker = new Worker('./worker.js', { workerData: data });
worker.on('message', resolve);
worker.on('error', reject);
});
}
This keeps your main thread free to handle other tasks.
Wrap-up
Node.js microservices are a game-changer for building scalable apps. Here's the lowdown:
- Split your app into small, focused services
- Give each service its own database
- Use API gateways and strong encryption
- Embrace Docker and Kubernetes
- Set up centralized logging and monitoring
- Automate testing at all levels
The future? It's looking good:
Trend | Impact |
---|---|
AI integration | Smarter apps |
Serverless | Less infrastructure hassle |
WebSockets | Better real-time features |
TypeScript | Cleaner code |
Big players like Uber, Netflix, and Amazon are already on board. What's next?
- Fancier orchestration tools
- AI and machine learning integration
- Beefed-up security and resilience
"We're always learning and tweaking our approach", says a Netflix senior engineer.
The microservices journey isn't over. It's just getting started.