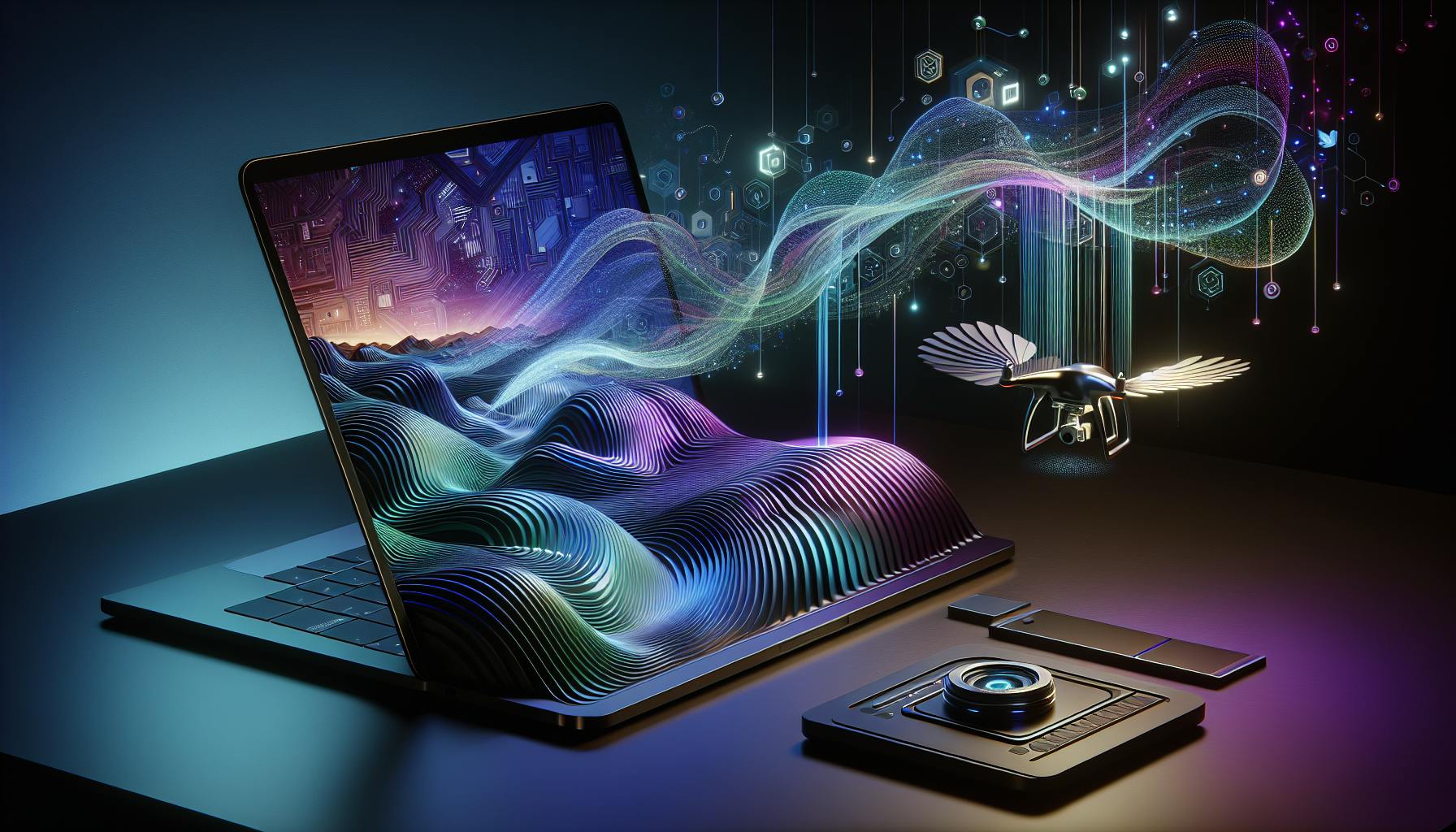
Boost your software development performance with these tips on optimizing code, database interactions, security, testing, workflow, and continuous learning.
Looking to give your software development a speed boost? Here's a straightforward guide to making your code run faster and your development process more efficient:
- Simplify Your Code: Keep it clean and reuse functions to minimize work.
- Use the Right Tools: Pick the best data storage and access tools for your needs.
- Asynchronous Programming: Run tasks simultaneously to improve speed.
- Efficient Algorithms: Choose algorithms that scale well with increasing data.
- Caching: Remember frequently used data to avoid reprocessing.
- Database Optimization: Implement indexes, simplify queries, and use connection pooling for faster database interactions.
- Security: Balance strong protection with performance by selectively applying encryption and using efficient protocols.
- Automated Testing: Regularly test for functionality and performance issues.
- Workflow Optimization: Use keyboard shortcuts, automate repetitive tasks, and streamline your toolchain for a smoother work process.
- Continuous Learning: Keep your skills sharp by exploring new languages, contributing to open source, and staying current with industry trends.
By focusing on these key areas, you can enhance both your coding efficiency and the overall performance of your applications.
Harness the Power of Tools and Resources
As developers, you have a bunch of tools and resources that can help you figure out where your code might be slow and how to make it faster. Here's how you can use some of these tools to improve your code's speed.
Profiling Tools Pinpoint Problem Areas
Profiling tools are like detectives for your code. They can show you where your code is slow or using too much memory. For instance:
- VisualVM can show you how much memory your code is using and where it might be getting stuck.
- Chrome DevTools can help you see where your web page's JavaScript might be slow.
- cProfile is great for Python code, showing you which functions are taking the longest.
Using these tools helps you know exactly where to make your code faster.
Linting Catches Inefficiencies
Linters are like your code's spellcheck. They look for problems like:
- Variables you don't use that are just taking up space
- Steps in your code that you don't need
- Bad habits that make your code slow
Fixing these issues can make your code run smoother.
Algorithm Visualization Builds Intuition
Watching how algorithms work through animations can help you understand which ones are faster or more efficient. Websites like Visualgo.net make it easier to get how different algorithms and data structures work.
Collaboration Fuels Innovation
Working with others, especially in the open source community, lets you share ideas and improve your code together. It's a great way to learn new tricks and make your code better.
Continuous Improvement Mindset
Always looking for ways to make your code better is key. Regularly checking your code's speed and making small improvements can lead to big gains over time.
Using these tools and keeping an open mind about improving your code can really help make it run faster. It's all about finding issues, fixing them, learning new things, working together, and getting better bit by bit.
Master the Art of Database Optimization
Making your database work better is crucial for fast applications. Here's how to make your database interactions smoother:
Implement Indexes for Faster Lookups
Put indexes on columns you often use for searching or combining data. This can make your searches much quicker. Look at slow searches to figure out which indexes will help most.
Tune Your Queries
Make your searches simple and only ask for the data you really need. Try to avoid complex joins if you can and don't make the database repeat tasks.
Connection Pooling
Instead of opening a new connection for every request, reuse existing ones. This cuts down on extra work and makes things faster. Look for libraries or database features that help with this.
Denormalize Data When Needed
Sometimes, making copies of data or grouping information together can make reading data faster because you don't need as many joins. But remember, this might make writing data slower and use more space.
Partition Tables
Splitting big tables into smaller parts based on time or ID range means you only have to look through a small piece of data. This can make searches and upkeep quicker.
Use Caching
Keep data you use a lot in memory so you don't have to ask the database for it every time. Tools like Memcached and Redis are good for this.
Monitor and Tune
Watch how your database is doing, keep an eye on slow searches, and see how performance changes. Adjust settings like how much memory to use and how many tasks can run at once based on what you find.
By setting up the right indexes, simplifying your searches, managing connections well, using caching, splitting up big tables, and keeping an eye on things, you can make your database access quicker for faster applications.
Prioritize Security Without Compromising Performance
When we build apps, we want them to be quick and also keep our users' information safe. But sometimes, adding security can slow things down. Here are some ways to keep things fast and secure at the same time:
Encrypt Data Judiciously
Encryption is a way to keep data safe, but it can make things slower. Only encrypt really important info like passwords. Use TLS/SSL for moving data around safely. Sometimes, only certain parts of the data need this extra protection.
Use Lightweight Security Protocols
JWT tokens for logging in are quicker than sessions. OAuth 2.0 lets people access stuff securely and works better for a lot of users than sessions. These options are lighter and faster.
Implement Rate Limiting
Rate limiting helps stop hackers by limiting how often they can try to break in, without stopping regular users. This means setting limits on how many times someone can try to log in or send wrong requests.
Assess Security Needs
Not all information is equally sensitive. Decide what needs the most protection and focus on that. Avoid using heavy security for everything.
Cache Securely
Caching makes apps faster by not redoing tasks. But, it needs to be done safely to avoid risks. Make sure the cached data is safe to use again.
Monitor Efficiently
Keeping an eye on what's happening is key for both security and keeping things running smoothly. But too much tracking can slow things down. Only keep track of the really important stuff.
Automate Testing
Automatic tests help find security problems quickly, without slowing down development. Test the parts of your code that handle logins and data protection to make sure they're strong and fast.
By focusing on the most critical security needs and choosing smarter, lighter options, you can keep your apps fast and safe.
Continuous Testing and Monitoring
Testing and keeping an eye on your software all the time is key to making sure it works really well. Here's how you can do it:
Implement Automated Testing
- Set up tests that run on their own for different parts of your software to spot problems early.
- Use the approach of writing tests first (TDD) to help plan your work and find issues fast.
- Make sure to automatically check for security risks regularly.
Monitor in Real-Time
- Use tools like Kibana, Grafana, or Splunk to make sense of important data in a visual way.
- Keep track of important info from your app, the tech it runs on, and how it's doing business-wise.
- Set up alerts for when things aren't going as expected.
- This helps you find and fix issues quickly.
Conduct Load Testing
- Check how your app handles a lot of users at once.
- Find out where it struggles before your users do.
- Make sure your app can grow and handle more work over time.
Prioritize Issues
- Use the data you're tracking to figure out what to fix first.
- Focus on the big problems that are causing the most trouble.
- Fixing these major issues is most important.
Keeping your software tested and monitored all the time helps it perform better and stay reliable. Setting up automatic tests, watching over your app's data, testing how it handles lots of work, and fixing the biggest problems are all important steps.
sbb-itb-bfaad5b
Workflow Optimization for Developers
Making your work process better can really help you do more in less time. Here are some easy ways to do it:
Use Keyboard Shortcuts
Learning shortcuts on your keyboard for your coding tools can save a lot of time. This means doing things quicker without using the mouse. Some common shortcuts help with completing code, finding and replacing text, and organizing your code neatly.
Automate Repetitive Tasks
Don't waste time on the same boring tasks. Use tools or write scripts that do these jobs for you. This could be anything from fixing your code format, running tests, to setting up your projects.
Streamline Your Toolchain
Using too many tools can slow you down. Pick tools that work well together and make your job easier. For example:
- Have your code editor show errors as you type
- Use automated systems to help test and deliver your work
- Make sure your tools connect easily with services on the cloud
Organize Your Workspace
A messy work area can distract you. Keep your digital space clean by:
- Closing programs or tabs you don't need
- Using software to manage your open windows
- Organizing your editor so you can see your code, terminal, and other tools easily
- Keeping your most used files and folders easy to access
Take Breaks and Switch Contexts
Sticking to one task for too long can tire you out. Remember to:
- Step away and take short breaks
- Move between different tasks
- Mix up tasks that need a lot of focus with easier ones
Keeping your tools, workspace, and schedule in check is key to doing your best work. What other tips do you have for making your work process better?
Continuous Improvement of Technical Skills
Keeping your coding skills sharp and up-to-date is key in the fast-moving tech world. Here's how you can keep getting better at coding:
Learn a New Language or Framework
- Pick a new programming language or framework that catches your eye or solves a problem you're facing.
- Begin with small steps by following tutorials and creating basic projects.
- Getting the hang of the basics makes learning more languages easier down the line.
Work on Open Source Projects
- Contributing to open source projects lets you dive into big, complex pieces of code.
- By fixing bugs and adding new features, you'll improve your skills and gain confidence.
- You'll also get helpful feedback from experienced developers when they review your code.
Try Different Development Roles
- Try your hand at different parts of development, like front-end, back-end, or testing.
- This helps you find out what you need to learn more about.
- It also turns you into a versatile developer who understands how the whole app works.
Stay Up-To-Date on Industry Trends
- Keep up with new trends by reading blogs, forums, and newsletters related to web development, software development, or cloud computing.
- Test out the latest tools and tech in your own projects.
- Whenever you can, go to meetups and conferences.
Undertake Certifications
- Getting certified shows you know your stuff in certain tech areas.
- The study process itself teaches you a lot about real-world problems.
- Certifications can help you move into new areas or get ahead in your career.
Practice Effective Learning Techniques
- Set clear goals to focus your learning.
- The best way to learn is by doing, so work on labs and projects.
- Use tools like flashcards and repetition to help remember what you learn.
Develop a Growth Mindset
- See tough times as chances to get better through hard work.
- Don't be scared of failing - it's just a step in the learning process.
- Believe that you can always improve with time and effort.
By committing to regularly updating your skills, you'll be able to keep up with changes in the industry and open up new job opportunities. Regular practice and trying out different learning methods will make a big difference over time.
Boosting Developer Productivity
Making developers more productive is key to getting good software done without wasting time. Here are some straightforward ways to do that:
Automate Repetitive Tasks
Use tools to handle boring tasks like checking code, running tests, and putting software out there automatically. This lets developers spend more time on creating code.
Standardize Processes
Agree on how to do things like writing code, using Git, and testing. This makes life easier because there's no arguing about the small stuff.
Prioritize Focus Time
Make sure developers have big chunks of time to code without interruptions. Jumping between tasks too much can slow things down and mess with the quality of work.
Promote Knowledge Sharing
Encourage working together on coding, reviewing each other's work, and talking about design to learn from each other. Knowing more helps the whole team do better.
Choose Appropriate Tools
Give developers the right tools for their specific jobs instead of making everyone use the same thing. The right tool can make a job much easier.
Gather Feedback
Regularly ask if there are things making work hard and fix them quickly. Don't let small problems turn into big ones.
Enable Work-Life Balance
Make sure developers aren't working too much and getting burnt out. Rested developers do better work. Taking care of oneself should be part of the job.
By focusing on these areas, we can help developers do their best work more easily. What other tips do you think are important?
Conclusion
Making your development work better and faster doesn't have to be complicated. Here's a quick rundown on how to do it:
- Clean coding practices - Keep your code simple, use the right tools for handling data, do tasks at the same time when you can, check how fast your code runs, and use caching to avoid redoing work. This helps your code run smoother.
- Utilizing profiling tools - Tools like VisualVM and Chrome DevTools can show you where your code is slow. This lets you fix those spots to speed things up.
- Database optimization - Make your database searches fast by using indexes, simplifying your searches, reusing connections, and keeping data organized. This makes your database work faster.
- Security prioritization - Protect important data without making everything slower by choosing smart security methods, limiting how often users can try to log in, and making sure your monitoring doesn't slow things down.
- Continuous testing and monitoring - Use automated tests to find problems early, keep an eye on how your system is doing in real-time, test how it handles lots of users, and fix the big issues first.
- Workflow optimization - Learn keyboard shortcuts, automate boring tasks, keep your tools simple, and organize your workspace. Taking short breaks helps too.
- Technical skill development - Try learning a new programming language, work on open source projects, explore different parts of development like web development or cloud computing, and keep up with new trends.
- Productivity enhancement - Automate tasks that you have to do over and over, agree on how to do common tasks, block off time to focus, share knowledge with your team, pick the right tools for the job, listen to feedback, and make sure to balance work with rest.
By focusing on these areas, you'll be able to make your coding work better and do it more efficiently. It's all about making small changes that add up over time.
Related Questions
How do you increase performance from your code?
To make your code run faster, you can:
- Choose smart ways to solve problems with algorithms and data structures that can handle more work easily
- Check your code to find slow spots
- Keep your code neat and organized so it's easier to handle
- Use ready-made code libraries that are built for speed
- Be smart about how you use memory, try to reuse things instead of making new ones all the time
- Always keep an eye on how fast your code is with tests and monitoring tools
How can I speed up my development team?
To get your team moving faster:
- Figure out what's most important and do that first
- Break tasks into smaller parts so more can be done at the same time
- Make repetitive tasks automatic, like testing and putting your software out there
- Keep everyone in the loop with quick meetings and clear instructions
- Think about getting help from outside for some tasks
- Cut down on interruptions so your team can focus on coding
What is the best way to increase software development productivity?
To help your developers do more, faster:
- Make boring tasks automatic
- Use tools and frameworks that make building software quicker
- Agree on how to do things so everyone's on the same page
- Work together on code and check each other's work
- Make sure there's time to focus without being disturbed
- Always look for ways to do better, based on what users say and your own reviews
How can an IDE improve a programmers productivity?
IDEs help programmers work faster by:
- Helping with code as you write, and finding information quickly
- Setting up projects and configurations automatically
- Working smoothly with Git for version control
- Making it easier to find and fix problems in your code
- Helping organize and improve your code structure
- Keeping everything you need in one place so you don't have to switch between tools