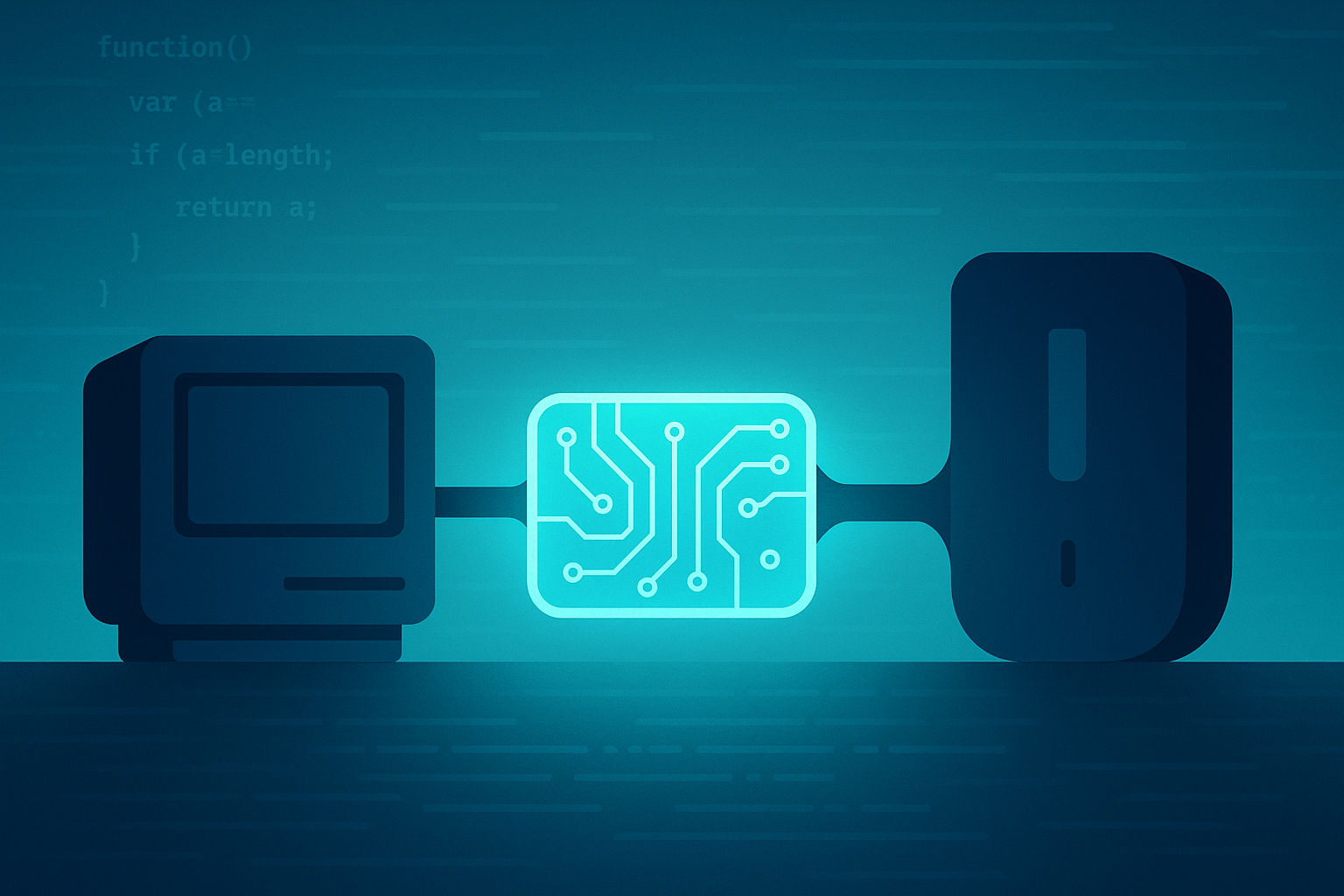
Learn how the Adapter Pattern in Java bridges incompatible interfaces, allowing seamless integration of legacy systems without altering original code.
The Adapter Pattern in Java connects incompatible interfaces, acting as a translator between systems. It’s useful for integrating legacy systems or third-party libraries without altering their original code. Here’s what you need to know:
- What It Does: Bridges different interfaces to work together seamlessly.
- When to Use It: Ideal for legacy system integration, third-party library compatibility, or reusing code when interfaces don’t align.
- How It Works: Includes three components:
- Target Interface: Defines the methods expected by the client.
- Adaptee: The existing class with an incompatible interface.
- Adapter Class: Translates between the Target Interface and Adaptee.
Example: A legacy payment system uses makePayment()
, but your app expects processPayment()
. An adapter bridges this gap, ensuring compatibility.
Key Benefits:
- Simplifies integration of old and new systems.
- Keeps original code untouched, reducing risks.
- Promotes clean, maintainable design.
Limitations:
- Adds an extra layer of complexity.
- Requires updates if interfaces evolve.
The Adapter Pattern Explained and Implemented in Java ...
Main Elements of the Adapter Pattern
The Adapter Pattern relies on three key components to ensure different interfaces can work together seamlessly.
The Target Interface
The Target Interface acts as the standard that client code interacts with. Think of it as the "plug" your application connects to. It defines the methods the client code can call, creating a consistent interaction point regardless of the underlying system.
For instance, in a payment processing system, the Target Interface might look like this:
public interface PaymentProcessor {
void processPayment(double amount);
boolean verifyTransaction(String transactionId);
}
This interface outlines the expected behavior, ensuring client code operates independently of specific payment system implementations.
The Original Class (Adaptee)
The Adaptee represents the existing class you need to integrate but doesn't match the Target Interface. This could be a legacy system, a third-party library, or any class with an incompatible design.
Here's an example of a legacy payment system:
public class LegacyPaymentSystem {
public void makePayment(float value) {
// Legacy payment logic
}
public int checkPaymentStatus(long paymentId) {
// Legacy verification logic
return 1;
}
}
The method names and parameter types here differ from those in the Target Interface, making direct integration impossible.
The Adapter Class
The Adapter class bridges the gap between the Target Interface and the Adaptee. It implements the Target Interface while internally working with the Adaptee's methods. This class handles the necessary translations to make the two systems compatible.
Here's an example of an adapter connecting the two payment systems:
public class PaymentSystemAdapter implements PaymentProcessor {
private LegacyPaymentSystem legacySystem;
public PaymentSystemAdapter(LegacyPaymentSystem system) {
this.legacySystem = system;
}
@Override
public void processPayment(double amount) {
// Convert double to float and call the legacy method
legacySystem.makePayment((float)amount);
}
@Override
public boolean verifyTransaction(String transactionId) {
// Convert String to long and map status codes
int status = legacySystem.checkPaymentStatus(Long.parseLong(transactionId));
return status == 1;
}
}
The Adapter handles tasks like:
- Translating method names
- Converting data types
- Adjusting parameters
- Transforming return values
Java Implementation Guide
Here's how to implement the Adapter Pattern in Java step by step:
1. Defining the Target Interface
Start by creating a clear and concise interface for the target functionality:
public interface PaymentProcessor {
void processPayment(double amount);
boolean verifyTransaction(String transactionId);
TransactionStatus getTransactionDetails(String transactionId);
}
public enum TransactionStatus {
COMPLETED,
PENDING,
FAILED
}
2. Creating the Original Class
Next, define the existing class (Adaptee) that has a different interface:
public class LegacyPaymentSystem {
private static final String API_VERSION = "1.2.3";
public void makePayment(float value, String currency) {
System.out.println("Processing $" + value + " payment via legacy system");
// Logic for legacy payment processing
}
public int checkPaymentStatus(long paymentId) {
// Status codes: 1 = success, 0 = pending, -1 = failed
return determineStatus(paymentId);
}
public Map<String, Object> getPaymentInfo(long paymentId) {
Map<String, Object> details = new HashMap<>();
// Populate payment details
return details;
}
private int determineStatus(long paymentId) {
// Internal logic to determine status
return 1;
}
}
3. Implementing the Adapter
Create an adapter class to bridge the gap between the target interface and the Adaptee:
public class ModernPaymentAdapter implements PaymentProcessor {
private final LegacyPaymentSystem legacySystem;
private static final String DEFAULT_CURRENCY = "USD";
public ModernPaymentAdapter(LegacyPaymentSystem system) {
this.legacySystem = system;
}
@Override
public void processPayment(double amount) {
legacySystem.makePayment((float) amount, DEFAULT_CURRENCY);
}
@Override
public boolean verifyTransaction(String transactionId) {
int status = legacySystem.checkPaymentStatus(Long.parseLong(transactionId));
return status == 1;
}
@Override
public TransactionStatus getTransactionDetails(String transactionId) {
int status = legacySystem.checkPaymentStatus(Long.parseLong(transactionId));
return mapToTransactionStatus(status);
}
private TransactionStatus mapToTransactionStatus(int legacyStatus) {
switch (legacyStatus) {
case 1: return TransactionStatus.COMPLETED;
case 0: return TransactionStatus.PENDING;
default: return TransactionStatus.FAILED;
}
}
}
4. Writing the Client Code
Finally, use the adapter in your client code to interact with the legacy system through the unified interface:
public class PaymentClient {
private final PaymentProcessor paymentProcessor;
public PaymentClient(PaymentProcessor processor) {
this.paymentProcessor = processor;
}
public void executePayment(double amount, String transactionId) {
try {
paymentProcessor.processPayment(amount);
if (paymentProcessor.verifyTransaction(transactionId)) {
TransactionStatus status =
paymentProcessor.getTransactionDetails(transactionId);
System.out.println("Transaction status: " + status);
}
} catch (Exception e) {
System.err.println("Payment processing failed: " + e.getMessage());
}
}
}
// Usage example
public class Main {
public static void main(String[] args) {
LegacyPaymentSystem legacySystem = new LegacyPaymentSystem();
PaymentProcessor adapter = new ModernPaymentAdapter(legacySystem);
PaymentClient client = new PaymentClient(adapter);
client.executePayment(99.99, "12345");
}
}
This approach ensures smooth type conversions, proper error handling, and a clear mapping of statuses. The use of dependency injection through the PaymentProcessor
interface also keeps the design flexible and testable.
sbb-itb-bfaad5b
Benefits and Limitations
The Adapter Pattern offers a range of advantages for Java developers but also comes with some challenges. Here's a quick breakdown of its key strengths and drawbacks in software development.
Pros and Cons Overview
Aspect | Advantages | Challenges |
---|---|---|
Code Integration | Helps integrate incompatible interfaces; Allows legacy code to work with newer systems | Adds an extra layer of abstraction, increasing complexity; May require multiple adapters |
Maintenance | Keeps changes isolated to adapter classes; Makes system updates easier | Needs updates if interfaces evolve; Adds extra code to maintain |
Testing | Simplifies unit testing by separating interfaces; Supports mock object creation | Both adapter and adaptee need testing; Expands test coverage requirements |
Performance | Low runtime overhead for simple use cases; Works efficiently in most scenarios | Can introduce slight performance issues; Using multiple adapters may increase memory usage |
Development | Encourages cleaner code by separating concerns; Improves code reusability | Requires careful initial setup; May be harder for new developers to grasp |
These points help developers weigh the trade-offs when deciding to use the Adapter Pattern.
This pattern is especially useful in enterprise-level Java applications where older systems need to work with modern components. Acting as a bridge, it allows gradual updates without disrupting existing systems. It also ensures that client code and legacy systems remain separate, lowering the chances of bugs during updates.
Usage Examples and Tips
Implementation Examples
The Adapter Pattern is a practical way to connect systems that don't naturally work together. For instance, imagine a financial transaction system where a legacy payment processor needs to work with a modern payment gateway. By using an adapter, you can seamlessly connect the old and new components.
Here’s how this might look in code:
// Legacy payment processor
public class LegacyPaymentProcessor {
public void processPaymentOld(String accountId, double amount) {
// Legacy payment logic
}
}
// Modern payment interface
public interface ModernPaymentGateway {
void processPayment(PaymentDetails details);
}
// Adapter implementation
public class PaymentProcessorAdapter implements ModernPaymentGateway {
private LegacyPaymentProcessor legacyProcessor;
public PaymentProcessorAdapter(LegacyPaymentProcessor legacyProcessor) {
this.legacyProcessor = legacyProcessor;
}
public void processPayment(PaymentDetails details) {
legacyProcessor.processPaymentOld(
details.getAccountId(),
details.getAmount()
);
}
}
Implementation Guidelines
Follow these tips to design better adapters:
-
Keep Interfaces Focused
Design adapter interfaces to be as simple and specific as possible. Splitting large interfaces into smaller ones can make your code easier to maintain and reduce dependencies. -
Handle Errors Gracefully
Include exception handling to translate errors from legacy systems into a format that modern components can understand. For example:public class RobustAdapter implements NewInterface { private LegacySystem legacy; public void newMethod() { try { legacy.oldMethod(); } catch (LegacyException e) { logger.error("Legacy system error: " + e.getMessage()); throw new ModernException("Operation failed", e); } } }
-
Test Thoroughly
Ensure your adapter works as expected by testing for interface compliance, proper error handling, performance, and smooth integration with other systems.
Learning with daily.dev
Want to stay sharp on the Adapter Pattern? Check out daily.dev for curated developer news and discussions. It’s a great resource for exploring best practices and real-world examples of this pattern.
Summary
The Adapter Pattern in Java helps connect incompatible interfaces. This guide covered how to use it for linking legacy systems with modern code.
Here are the key components:
- Target Interface: Defines how the client interacts with the system.
- Adaptee: The original class with functionality that needs adapting.
- Adapter Class: Bridges the gap by implementing the target interface.
To apply this pattern effectively:
- Focus on a single responsibility - converting one interface to another.
- Handle errors properly when crossing system boundaries.
- Test thoroughly, including edge cases, to ensure reliability.
Using adapters allows for clean, maintainable code that connects different interfaces without changing the original functionality. It’s a practical way to integrate older systems into newer ones.
FAQs
How does the Adapter Pattern help connect legacy systems with modern applications?
The Adapter Pattern acts as a bridge between incompatible interfaces, enabling legacy systems to work seamlessly with modern applications. By creating an adapter class, you can translate the existing interface of a legacy system into one that matches the requirements of a new application, without altering the original code.
This approach is particularly useful when integrating older systems that cannot be modified but still need to interact with newer software. It ensures compatibility while reducing the risk and cost of overhauling legacy systems, making it a practical solution for maintaining and modernizing existing infrastructure.
What are some best practices for creating an Adapter class in Java to ensure it is efficient and easy to maintain?
When designing an Adapter class in Java, follow these best practices to ensure maintainability and efficiency:
- Keep it simple: Focus on translating the interface of one class to another without adding unnecessary complexity.
- Favor composition over inheritance: Use composition to wrap the adaptee class, as it provides more flexibility and reduces tight coupling.
- Adhere to SOLID principles: Ensure the adapter conforms to the Single Responsibility Principle by limiting its role to bridging incompatible interfaces.
- Write clear documentation: Explain the purpose and behavior of the adapter, especially when working in collaborative environments.
By following these guidelines, you can create adapters that are both efficient and easy to maintain in the long term.
How does the Adapter Pattern impact application performance, and how can potential issues be addressed?
The Adapter Pattern can introduce a slight performance overhead due to the additional layer of abstraction it creates. This is because the adapter acts as an intermediary, translating requests between incompatible interfaces, which can marginally increase processing time in performance-critical applications.
To minimize potential drawbacks, consider the following steps:
- Use the Adapter Pattern only when necessary: Avoid overusing it in scenarios where simpler design solutions can achieve the same result.
- Optimize the adapter implementation: Ensure that the adapter logic is efficient and avoids unnecessary computations.
- Profile your application: Regularly test and monitor performance to identify any bottlenecks caused by the adapter and address them promptly.
While the Adapter Pattern is a powerful tool for increasing flexibility and reusability, mindful implementation can help maintain optimal application performance.