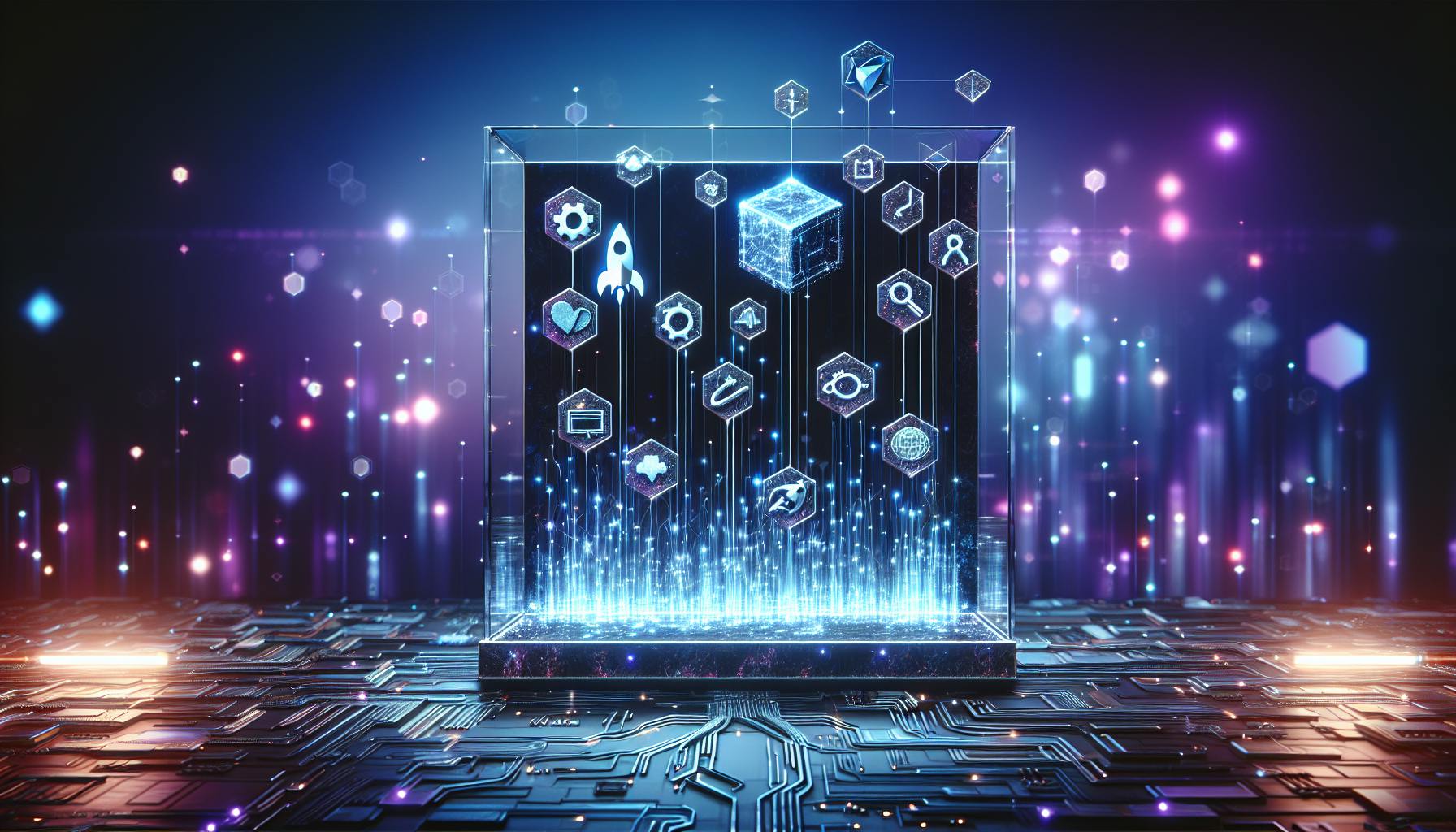
Learn how to automate Chrome extension development efficiently. Explore tools, techniques, and steps to streamline the process, from testing to publishing.
Automating the development of Chrome extensions can significantly streamline the process, making it faster, more efficient, and less prone to errors. Hereโs a straightforward rundown of what this article covers:
- Quick and easy updates with scripts for building and releasing new versions.
- Enhanced testing ensures extensions work perfectly across various platforms.
- Cross-browser functionality simplifies developing extensions for Chrome, Firefox, and more.
- Reduced mistakes thanks to automated repetitive tasks and thorough testing.
Tools like Puppeteer, Playwright, and specific browser settings play a crucial role in automation, alongside essential skills in JavaScript, using the Web extensions API, and setting up automatic build and deployment pipelines. From automating your build process and testing to debugging techniques and eventually publishing your extension, this guide walks you through each step to automate Chrome extension development efficiently.
Key Takeaways:
- Utilize GitHub Actions for automating builds, testing, and releases.
- Employ JavaScript Build Tools like Gulp and Grunt for live reloading and optimization.
- Implement Automated Testing with Puppeteer or Selenium for end-to-end validation.
- Apply Debugging Techniques using Chrome's built-in tools and logging for troubleshooting.
- Follow steps for Publishing Extensions on the Chrome Web Store, ensuring your extension is available to users.
By embracing automation, developers can focus more on innovation and less on the repetitive aspects of extension development.
Overview of Components
Chrome extensions are small programs that add extra features to your browser. They're made up of several important parts:
The manifest.json file is like the extension's ID card. It tells Chrome what the extension is called, what version it is, what permissions it needs, and which scripts to run. It's the starting point for any extension.
Background scripts are like the behind-the-scenes workers. They run without you seeing them, doing things like sending requests and waiting for the browser to do something.
Content scripts are the parts that mess with the web pages you visit. They can add new JavaScript or change the page's look with CSS to do things like block ads or highlight text.
Popup pages pop up when you click the extension's icon. They're little web pages where the extension can show you stuff or let you do things.
Options pages are where you can change how the extension works to suit your needs. They're also made with HTML and JavaScript.
Extension Lifecycle
When you first add an extension to Chrome, it reads the manifest and starts up any background scripts. From then on, it waits for stuff to happen, like you opening a new tab or clicking its icon.
Whenever you go to a new page, the content scripts get to work, changing the page if that's what they're supposed to do. Clicking the extension icon brings up the popup page, and you can get to the options page through a menu.
Extensions can talk to each other, too. For example, a script on a webpage might send a message to a background script to save some data.
If you remove the extension, it stops all its scripts and takes away its permissions. However, some data it saved might stick around unless you delete it yourself.
So, in short, Chrome extensions use different scripts and pages to make browsing better or more personalized. The manifest file is like the blueprint that tells Chrome how to put everything together.
Setting Up the Environment
To start making your Chrome extension automatically, you need a few key tools and some know-how:
Code Editor
A good code editor like Visual Studio Code is important. You want it to have:
- A place to type commands directly
- A way to find and fix errors easily
- Support for adding extra features
- Options to change its look and shortcuts
This helps you write, check, and send off your code quicker.
Node.js
Node.js is needed for running many of the scripts that make things automatic. Download the Current or LTS version.
Testing Frameworks
Tools like Jest or Mocha are super helpful. They allow you to:
- Automatically check your code for mistakes when you make changes
- Pretend to be in a browser to see if things work right
- Test features without having to do it by hand
Getting good at writing tests is a must.
Browser Automation Tools
Puppeteer and Playwright are great for testing in browsers like Chrome or Firefox without having to do everything yourself. They can:
- Open browsers when needed
- Act like they're on a mobile device
- Use pages as if they're a real person
- Take pictures of pages
- Check how fast things load
This means less boring manual testing for you.
Skills
Knowing your way around JavaScript is key. You should learn about:
- Writing code that can wait for things to happen
- How to use the Web extensions API
- How to test small parts of your code
- Using Git for version control
- Setting up automatic build and deployment pipelines
With these tools and skills, you can make the process of building, testing, and releasing your Chrome extension much smoother. It's worth the effort for the time and headaches you'll save.
Automating Development Tasks
Making the same steps over and over again when developing Chrome extensions can be a drag. Let's look at how to make these tasks run by themselves, saving time and avoiding mistakes.
GitHub Actions Workflows
GitHub Actions is a tool that lets us automate stuff like:
-
Building - Whenever you make changes and push them to the
main
branch, you can have a setup that automatically gets everything ready, checks for errors, packages your extension, and makes sure your manifest file is correct. -
Testing - You can set up a way to automatically install your extension, open a browser with Puppeteer, and run tests whenever you push changes or make a pull request. You can even test on different browsers like Chrome, Firefox, and Edge.
-
Releasing - When you're ready to release a new version of your extension on the Chrome Web Store, you can automate the process. This can include uploading your extension, writing up the store listing, and sending it off for review as soon as you make a GitHub release.
The cool part is you don't have to do these tasks by hand, everything runs the same way every time, and you find out quickly if something's wrong. You can even use ready-made workflows from GitHub Marketplace to get going quicker.
JavaScript Build Tools
Tools like Gulp and Grunt help with the day-to-day work:
-
Live Reloading - Automatically updates your browser when you make changes, using a plugin called browsersync.
-
SASS/CSS - Makes your stylesheets look good, adds browser-specific prefixes, and makes the files smaller so they load faster.
-
Bundling - Puts all your scripts together with Webpack, and changes newer JavaScript into a form that works in all browsers with Babel.
-
Linting - Checks your code for mistakes automatically every time you save, using ESLint.
-
Optimization - Makes images smaller, and compresses your JavaScript and CSS to make your extension faster.
-
Scaffolding - Lets you quickly set up new parts of your extension.
By automating these boring tasks, developers can spend more time creating cool stuff. You can find ready-to-use setups in the tool's community to make things even easier.
In short, GitHub Actions takes care of testing, building, and releasing your extension, while build tools make working on your extension faster and less of a hassle. Together, they let you focus on improving your extension without getting bogged down in repetitive tasks.
Automated Testing
End-to-End Testing
End-to-end testing is like a thorough check-up for Chrome extensions. It means setting up the extension in a browser and then pretending to be a user to see if everything works right. Here are some tools that help with that:
- Puppeteer - This is a tool that lets you control Chrome without actually opening it. You can do things like click buttons, fill out forms, and take pictures of the webpage. It's great for making sure your extension works without having to do it all by hand.
- Selenium - This tool can control not just Chrome, but also Firefox and Edge. It's used to check how your extension works in different browsers.
When you're testing, remember to:
- Set things up, do the test, then check if you got what you expected. This keeps your tests easy to follow.
- Wait for parts of the page to load before trying to do anything. This helps avoid errors where the test fails just because something was slow to appear.
- Split your tests into smaller parts that each look at one thing. This makes it easier to fix problems when they pop up.
- Use the browser without showing the actual window (headless mode) to make tests run faster.
- Automatically run these tests every time you change your code. This way, you catch problems early.
Headless Testing
Testing without showing the browser window speeds things up and uses less computer power. Here's how to do it:
-
Start Chrome with special settings so it doesn't open up the window.
-
Use a consistent ID for your extension so the computer doesn't get confused when installing it.
-
Restart the browser in a special mode to clear everything out between tests.
-
Make sure the browser starts the same way every time to keep your tests consistent.
-
Set the browser to pretend it's a bigger screen for testing how things look on different devices.
Testing like this gets you quick feedback and makes sure your extension works well, even without seeing it on your screen.
sbb-itb-bfaad5b
Debugging Techniques
Finding and fixing bugs in Chrome extensions can be a bit complex because there are many parts working together. Here's how to make it easier:
Use Debugging Tools
Chrome has some built-in tools that are super helpful for finding problems. You can:
- Stop the code and look at what's happening at that moment.
- Choose specific places in the code to pause and see what's going on.
- Watch for network requests to see if there are issues with data coming in or out.
- Check for slow performance or JavaScript mistakes.
Don't forget to check both the background and content script parts.
Log Everything
Using console.log()
to write out what's happening in the code can really help. This way, you can track where things might be going wrong by looking at the steps taken, values of variables, and any errors.
Test in Isolation
Try testing different parts of your extension on their own to find where the issue is:
- Turn off other extensions to make sure they're not causing problems.
- Load your extension without installing it to quickly test changes.
- Split your code into smaller parts and test them one by one.
Simulate Different Environments
Sometimes, problems only show up for some users. Make sure to:
- Test using both Chrome and Firefox with tools like Puppeteer or Playwright.
- Pretend you're on a phone.
- Clear out storage and cookies between tests.
- Fake server responses.
- Use different user profiles.
Automate Testing
Setting up tests that automatically run every time you make a change can help catch bugs early. Use tools like Jest for testing smaller parts of your code.
Use Flags
Turning on certain Chrome settings can give you more tools to find bugs:
#enable-devtools-experiments
gives you extra tools in Chrome's DevTools.#extensions-on-chrome-urls
lets your extension work on Chrome's internal pages.
Ask for Help
If you're stuck, sometimes just asking another developer can help. They might see something you missed.
By following these steps, you can systematically find and fix bugs in your Chrome extension. It's all about testing thoroughly and breaking down your code to tackle issues more effectively.
Publishing Extensions
Getting your extension into the Chrome Web Store lets people find, install, and use what you've made. Here's how to do it step by step.
Enabling Developer Mode
First off, you need to pay $5 to get set up as a Chrome Developer. This is a one-time thing that lets you use some extra tools and publish your extensions.
- Head over to the Chrome Web Store developer dashboard and hit Get Started.
- Agree to the Developer Agreement and handle the $5 fee.
Now, you're all set to upload extensions and take care of them after they're up.
Packaging the Extension
Before you send your extension off to the store, you'll want to:
- Make sure you've squashed any bugs and that everything's working right.
- Double-check your manifest file to make sure all the details are correct.
- Give your extension a unique ID to keep things smooth.
- Zip up your source files into a
.zip
file.
This gets your extension ready to go.
Submitting to the Store
To get your extension out there:
- Go back to the Chrome Developer Dashboard and click Add new item.
- Choose your
.zip
file and upload it. - Fill in the blanks with your extension's name, a description, some pictures, and pick a category. The more helpful and clear your info is, the better.
- Send it off for review. This part checks if your extension follows the rules and usually takes between 1-7 days.
Once it passes, your extension will show up in the Chrome Web Store for everyone to find and install!
Updating Existing Extensions
When you've got a new version ready:
- Change the version number in your manifest file.
- Package up the new version and upload it through the dashboard.
- New updates typically get checked within hours, so your users can start using the latest version sooner.
By sticking to these steps, you can get your Chrome extension out to the world through the Chrome Web Store. Make sure everything's set up right to get through the review smoothly and provide a great experience for your users.
Conclusion
Making the process of creating Chrome extensions automatic can really help save time and make sure everything works well. By using tools like GitHub Actions, JavaScript tools, and testing tools for browsers, developers can:
- Speed things up by automatically handling updates, testing, and releasing.
- Make things more reliable with a lot of tests in different situations.
- Improve how fast things run by optimizing the build process and using live reloading.
- Make finding and fixing problems easier with logging, testing one thing at a time, and trying out different scenarios.
- Make getting your extension out there smoother by making sure it meets the Chrome Web Store's needs.
In short, automation lets developers spend more time making cool new features instead of doing the same tasks over and over. The time saved means they can try out new ideas, while automatic checks make sure the extension works right for everyone.
By using the steps we talked about, developers can set up a way to take care of a lot of the hard work on their own. This means they can focus on making better and more interesting extensions. As more developers use these automation tools, we're going to see even cooler and more useful extensions come out!
Related Questions
How do I automate Chrome extensions?
To automate tasks in Chrome extensions, you can use a tool called Selenium IDE. Here's a simple way to do it:
- Add the Selenium IDE extension to your browser.
- Open Selenium IDE.
- Start recording a test by doing things in your browser as you normally would.
- If needed, tweak the test a bit to make sure it does exactly what you want.
- Save your test.
- Run the test to watch it do the actions automatically.
- If you want, you can turn the test into a script for more advanced use.
Selenium IDE makes it easy to create and run tests without deep coding knowledge.
What programming language do Chrome extensions use?
Chrome extensions use web tech like HTML, CSS, and JavaScript. JavaScript is the key language for adding functionality to extensions.
Can I develop a Chrome extension?
Yes, if you're familiar with HTML, CSS, and JavaScript, you can definitely create your own Chrome extension. The available APIs let you interact with browser events and data.
What is the best automation extension for Chrome?
One of the top tools for automating tests in Chrome is the Selenium IDE extension. It's great for recording, tweaking, and running tests easily. Plus, you can export these tests for use in more complex testing setups.