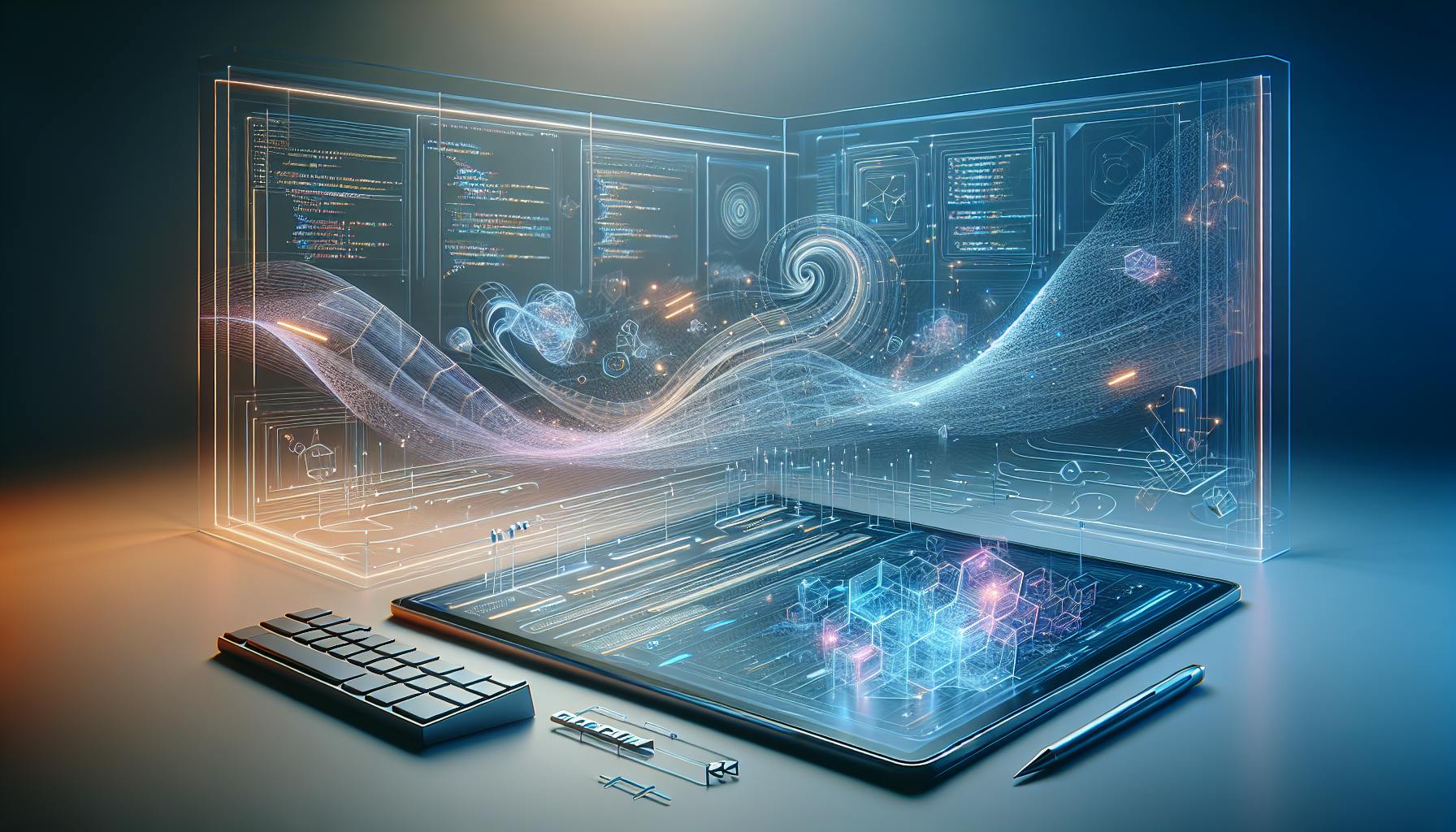
A comprehensive guide to competitive programming for beginners, covering essential concepts, learning resources, practice strategies, participating in contests, and advancing to the next level.
Diving into competitive programming can seem daunting at first, but with the right approach, it's an enriching journey that sharpens your coding skills, prepares you for tech job interviews, and connects you with a global community of coders. Here's a quick guide to get you started:
- Why Competitive Programming? It's not just about coding fast; it's about solving problems smartly within constraints, improving analytical skills, and staying updated with coding trends.
- Getting Started: Choose a beginner-friendly programming language like Python or Java for their simplicity and supportive communities.
- Essential Concepts: Master data structures, algorithms, and complexity analysis to solve coding puzzles effectively.
- Practice Platforms: Start with websites like Codeforces, CodeChef, and LeetCode, which offer problems sorted by difficulty.
- Learning Resources: Utilize books, online courses, and platforms like GeeksforGeeks for deep dives into specific topics.
- Contest Participation: Engage in various contests to test your skills, speed, and adaptability.
- Staying Motivated: Regular practice, participating in contests, and learning from solutions are key to progress.
- Advancing Further: Once comfortable, explore more complex topics and aim for prestigious contests to challenge yourself further.
Whether you're a complete beginner or looking to refine your skills, this guide lays out the steps to embark on your competitive programming journey.
What is Competitive Programming?
Competitive programming is like a timed puzzle game for coders. You're given problems to solve, but you have to do it quickly, without using too much computer memory, and your solution has to work perfectly every time. Here's what you usually need to watch out for:
- Time limits - You've got only a few seconds to come up with a solution. This means your code has to be fast.
- Memory limits - Your solution can't be a memory hog. You need to figure out how to do more with less.
- Correctness - Your code must solve the problem correctly for all possible scenarios, even the tricky ones.
- Task requirements - Sometimes, there are extra rules, like how long your code can be or which programming languages you can use.
The main goal is to create solutions that are quick, light on memory, and correct. This helps you become a better programmer.
Brief History and Evolution
Competitive programming started with college contests in the 1970s. Thanks to the internet, now anyone from anywhere can join in.
Here's a quick timeline:
- 1970 - The first college contest happened.
- 1977 - A big annual contest for colleges started.
- 1998 - Topcoder started, making contests available to everyone, not just students.
- 2000s - More platforms like CodeChef and HackerRank popped up.
- Today - Millions of people around the world take part in these contests every year.
The internet made it easy for anyone interested in coding to try these challenges. Now, there's a huge community of people who enjoy solving coding puzzles and getting better at programming.
Chapter 2: Who Should Get Into Competitive Programming?
Intended Audience
Competitive programming is a great fit for students and professionals in tech who want to sharpen their thinking and get ready for coding interviews. Here's who will find it especially useful:
- University students in computer science or similar areas. It's a practical way to apply what you learn in class.
- Software engineers who want to stay sharp or prepare for job interviews focusing on algorithms and data structures.
- Aspiring programmers aiming to enter the tech world. It's a solid way to build coding skills that employers look for.
- Math lovers who enjoy solving puzzles and challenges. The problems in competitive programming are right up their alley.
- Anyone looking to get better at thinking on their feet. It's all about solving problems quickly and logically.
Useful Skills and Traits
Some skills and qualities can help you do well in programming competitions:
- Persistence: Keeping at it, even when the problems are tough. Being ready to try again and again.
- Analytical skills: Being able to take a big problem, break it into smaller parts, and figure out the best solution.
- Learning mindset: Always looking to learn from mistakes and improve. Checking out how others solve problems can be a big help.
- Logic and reasoning: Using logic to work through problems step by step. Spotting patterns and connections is key.
- Efficiency: Finding answers quickly within the time limit. Knowing which data structures and algorithms to use can save a lot of time.
- Attention to detail: Making sure you understand the problem fully, including all the small details. Catching those tricky bits that are easy to miss.
With some practice and effort, competitive programming can help anyone get better at these skills.
Chapter 3: Choosing the Right Programming Language
Comparison of Languages
Language | Pros | Cons |
---|---|---|
C++ | - Fast execution speed - Great for data structures and algorithms |
- Hard for beginners |
Java | - Works on many devices - Good for organizing code |
- Not as fast as C++ |
Python | - Simple to learn - Lots of helpers |
- Slower than the rest |
When you're starting in competitive programming, picking the right language matters. Here's a quick look at the top options:
C++ is a favorite in programming competitions because it's fast and has built-in support for the complex tools you'll need. But, it can be pretty tough for newbies.
Java can be used on various devices and has features that help keep your code neat. The downside? It's a bit slower than C++.
Python is the easiest to learn and has a big community ready to help. The trade-off is that it's not as fast, making it less ideal for time-sensitive problems.
Recommendation for Beginners
If you're new to competitive programming, starting with Python or Java might be best. Their simpler syntax makes them more approachable than C++.
As you get more comfortable with programming basics and start to Solve Problems using Data Structures and Algorithms, you might want to switch to C++ for its speed advantage in contests like Codeforces, Codechef, or Topcoder.
But starting with Python or Java is great because they have lots of learning resources and supportive communities. This can make your introduction to competitive programming smoother. Later on, you can always move on to C++ when you're ready for a challenge.
Chapter 4: Essential Concepts to Master
Let's dive into some key ideas and tools you need to get good at competitive programming. These basics will help you solve those tricky coding puzzles more effectively.
Data Structures
Think of data structures as different ways to keep your data organized so you can use it more efficiently. Here's a quick rundown of some you should know about:
- Arrays - They're like shelves where you store items of the same kind. You can quickly grab any item you need.
- Linked Lists - Imagine a treasure hunt where each clue leads to the next. Adding or removing clues is easy.
- Stacks - Picture a stack of plates. You can only take the top plate off or put a new one on top.
- Queues - It's like standing in line. The first person in line is the first one to get served.
- Trees - Think of it as a family tree but for data. Each branch can have up to two children.
- Graphs - A web of connected points. It's great for showing how things are connected.
- Tries - A special kind of tree used for searching words quickly, like in a dictionary.
Algorithms
Algorithms are the steps you follow to solve a problem. Knowing the right one can make solving puzzles much easier.
- Sorting - It's like organizing your bookshelf in order. There are different ways, like quicksort or mergesort.
- Searching - Trying to find a book on your shelf. Binary search helps you find it faster.
- Dynamic Programming - Breaking a big problem into smaller, easier ones. It helps you find the best solution faster.
- Graph Algorithms - Finding the best route on a map. Dijkstra's algorithm is one way to find the shortest path.
Analysis of Complexity
This is about figuring out how fast your solution is and how much memory it uses. Here's what you need to know:
- Big O - Tells you the worst-case scenario, like how long the longest search could take.
- Big Omega - Shows you the best case, like the shortest time to find a book.
- Big Theta - Gives an average idea, like how long it usually takes to find your book.
Understanding these concepts helps you make smart choices about how to solve problems, especially in competitive programming contests like Codeforces or Topcoder. It's all about finding the right balance between speed and using less memory.
Chapter 5: Setting Up Your Coding Environment
Choosing an IDE/Editor
When you start with competitive programming, picking the right tool to write your code in can really help. Here are some good options:
- Visual Studio Code - A favorite for many because it's free and can be made even better with add-ons. It's great for coding contests.
- Sublime Text - A simple and quick editor that makes your code look nice and is easy to tweak.
- Vim/Neovim - These are for those who like using keyboard shortcuts and want to customize their coding space. They're a bit more advanced.
- CLion - Perfect for C++ coding with built-in features to check and fix your code. There's a free version for students.
- PyCharm - A smart tool for Python with helpful features for fixing and running your code. There's a free version too.
Visual Studio Code is a good middle ground for ease of use and making it your own. Sublime Text is another good choice if you want something more straightforward.
Browser Extensions
Some browser add-ons can make coding easier:
- Codeforces Keyboard Shortcuts - Lets you do common tasks on Codeforces with keyboard shortcuts.
- Code Notes - You can save code snippets for later, which is handy for looking back at how you solved something.
- Enhancer for Codeforces - Makes the Codeforces website easier to use with extra features.
- Dark Reader - Turns websites dark, so they're easier on your eyes.
These add-ons can help you work faster and keep things organized right in your browser.
Using Online IDEs
Platforms like Codeforces offer their own places to code online:
Pros:
- Easy to use with access to problems.
- No need to set anything up on your computer.
- They take care of sending in your solutions.
Cons:
- You might not get to use the tools or languages you like.
- You have to work within the platform's limits.
- Might not have all the features of standalone tools.
Online IDEs are a quick way to jump into coding problems. But as you get more into competitive programming, you might prefer setting up your own coding space for more flexibility.
Try both and see what works for you. With some practice, you'll find the setup that lets you code the best.
Chapter 6: Starting with Competitive Programming
Getting into competitive programming might look tough at first, but with a clear plan, you can get going easily. Here's a simple guide to help beginners dive into competitive programming:
Choose a Platform to Practice
To start, pick a website where you can find coding problems sorted by how hard they are. This way, you can slowly challenge yourself more as you get better. Some good places for beginners include:
- Codeforces - Here, problems are grouped into levels and rated by how tough they are. It's a great place to practice algorithms.
- CodeChef - You'll find lots of questions sorted by topics and difficulty levels.
- LeetCode - This site ranks problems from Easy to Hard. It's also a favorite for getting ready for job interviews.
These websites help you easily find problems that match your skill level and let you code right in your browser.
Read Problems Carefully
Take your time to really understand each problem before you start coding:
- Go over the problem description a few times, picturing what it's asking.
- Make sure you know what all the important words and rules mean.
- Think about different examples and special cases you'll need to consider.
- Figure out what you need to put into the function and what it should give back.
Jumping straight into coding without this step often means solving the wrong problem!
Start Solving!
- Try solving a few problems every day in the language you're learning. Begin with the easier ones.
- Go back to problems you've solved before after a while to see if you can make your solutions better.
- Join in on short contests to get used to coding quickly.
It's okay if it's hard at first. Competitive programming gets easier with practice. Just keep at it, and you'll gradually get better.
Chapter 7: Learning Resources and Platforms
Useful Books
Here are some great books to help you get better at competitive programming:
- Competitive Programming 3 by Steven Halim and Felix Halim - This book covers important topics like data structures, algorithms, and math concepts you'll need to know.
- Guide to Competitive Programming by Antti Laaksonen - It's a good book for learning how to code efficiently and solve problems.
- Competitive Programmer's Handbook by Antti Laaksonen - Offers a wide range of tips and advice on competitive programming.
- Pearls of Functional Algorithm Design by Richard Bird - This book teaches you how to use functional programming to design algorithms.
- Algorithm Design Manual by Steven Skiena - Covers lots of different algorithms and how to analyze them.
For specific topics, these books are helpful:
- Introduction to Algorithms by Cormen et al. - A well-known textbook for learning algorithms.
- Algorithms by Dasgupta et al. - Focuses on understanding algorithms at a high level.
- Computational Geometry by Mark de Berg - Good for learning how to solve geometry problems.
Online Courses
Here are some online courses that can help you improve your competitive programming skills:
- Divide and Conquer, Sorting and Searching, and Randomized Algorithms from Stanford University on Coursera - Great for learning about different ways to design algorithms.
- Data Structures and Algorithms Specialization on Coursera - A set of courses from UC San Diego that covers the basics.
- Competitive Programmer's Core Skills on Educative - Focuses on the skills you need for programming competitions.
- Grokking the Coding Interview: Patterns for Coding Questions on Educative - Helps you get ready for job interviews by practicing common question patterns.
Websites and Online Judges
These websites have lots of resources and problems to practice with:
- GeeksforGeeks - A good place for learning about data structures and algorithms.
- LeetCode - Has interview questions from companies that you can practice coding online.
- HackerRank - Offers a big collection of challenges sorted by difficulty.
- Codeforces - A popular site for joining programming contests.
- AtCoder - A Japanese site with regular contests and lots of problems to solve.
- Topcoder - Hosts competitive programming events and has tutorials.
- CodeChef - An Indian site with contests and many algorithmic problems to work on.
sbb-itb-bfaad5b
Chapter 8: Practice Strategies and Techniques
Practice Consistently
Make it a habit to work on competitive programming problems every day. Tackling different kinds of problems regularly and going back to ones you've done before can really sharpen your skills.
- Try to solve 3-5 problems each day, slowly moving to harder ones.
- Look at problems you solved a while back to see if you can find a better solution now.
- Have small contests with friends and talk about how each of you approached the problems.
Keeping at it regularly is the best way to get good at competitive programming.
Attempt All Contest Problems
When you're in a contest, try to work on every problem, not just the ones that seem easy. This helps you learn more and improve your coding skills.
- Make sure to read all the problem statements before you decide to skip any.
- Quickly decide which problems are easy, medium, or hard.
- Start with the easy ones, then move on to the tougher ones.
- After the contest, go over all the problems, even the ones you didn't solve.
Trying different kinds of problems can make you better at competitive programming.
Review Solutions Thoroughly
Looking at solutions, especially for problems you couldn't solve, is a great way to learn new ways to tackle problems in the future.
- Check out the best solutions even for problems you did solve. You might find a smarter way to do it.
- If you didn't solve a problem, really understand the solution before you try to code it.
- Figure out the main idea that makes the solution work.
- Group problems by the kind of thinking they need so you can review them later.
Going through solutions carefully can help you get better at competitive programming.
Chapter 9: Participating in Contests
Benefits of Contests
Joining contests is a great way to get better at competitive programming. Here's why they're helpful:
- Speed: They teach you to code fast and efficiently because you're racing against the clock.
- Testing skills: Contests give you a chance to see how good you are compared to others. It's a great way to see how much you've learned.
- Exposure to problems: In contests, you'll face a wide variety of challenges. This helps you learn new things and get better at solving problems.
Types of Contests
Contests come in different shapes and sizes:
- Short contests: These are quick, lasting only about 1-2 hours with a few problems to solve. They check how fast and accurately you can code.
- Long contests: These take longer, about 5-6 hours, and have more difficult questions. They're good for testing your focus and ability to solve tough problems over time.
- Marathon matches: These are the ultimate test, going on for days and including many challenging problems. They need a lot of skill and patience to get through.
Contest Preparation
Getting ready for a contest is important. Here are some tips:
- Revise concepts: Go over important topics like data structures, algorithms, and how to analyze problems. This will help you during the contest.
- Analyze past problems: Look at questions from previous contests to get an idea of what to expect.
- Outline approaches: Think about how you'll tackle different types of problems quickly.
- Practice adequately: Solve lots of practice problems under timed conditions to get used to the pressure.
With good preparation, contests can really help you improve your competitive programming skills.
Chapter 10: Overcoming Challenges
Getting Stuck on Problems
It's normal to hit a roadblock when you're working on coding problems. Before you rush to find the answer, try these steps first:
- Read the question again carefully to make sure you didn't miss anything important.
- Look for small clues in the problem tags or editorials that hint at what you need to know, without giving away the whole answer.
- If you're really stuck, take a short break. Sometimes, stepping away for a bit helps you see things more clearly when you come back.
- Work through the problem with pen and paper using examples. This can help you understand it better.
- Talk about the problem with friends or online. Explaining what you're thinking can help spot where you might be going wrong.
Trying these steps helps you get better at figuring things out on your own, bit by bit.
Feeling Overwhelmed
Sometimes, competitive programming can feel like too much. If you're feeling swamped, remember these tips:
- Practice a little bit every day instead of trying to do a lot all at once. Building your skills slowly is easier to keep up.
- Don't forget to take breaks. If you're feeling worn out, it's okay to step away and come back later.
- Break big problems down into smaller parts. This makes them seem less scary.
- Celebrate the small victories. Focus on what you're learning and getting better at, not just the problems you solve.
Keeping a steady pace and focusing on gradual improvement can help keep you from getting burned out.
Staying Motivated
Keeping up your enthusiasm for competitive programming is key. Here are some ways to stay motivated:
- Make sure you really understand the basics before moving on to harder stuff. Knowing the foundation well makes everything else easier.
- Keep track of your progress by going back to problems that used to be tough for you. Seeing how far you've come can be a big boost.
- Pick contests that are right for your level. Challenges that feel just right can be more fun.
- Join online coding communities. Talking with others who are learning too can give you new ideas and keep you going.
Taking it step by step and focusing on learning and growing, rather than comparing yourself to others, can help keep you motivated.
Chapter 11: Advancing to Next Level
Filling Knowledge Gaps
As you get more into competitive programming, you might notice some areas where you're not as strong. Here's how to get better:
- Look back at problems you've worked on. If there are concepts you found tough or had to look up a lot, those are areas you need to work on.
- Pick up a good book or an online course on algorithms and data structures. Pay extra attention to the parts you find hard.
- Practice coding up the data structures and algorithms yourself. This is a great way to make sure you really understand them.
- Try using tools that show you visually how complex data structures work. Seeing them in action can help you understand them better.
Spending time to improve your basic computer science knowledge will help you solve harder problems later.
Exploring New Topics
Once you're comfortable with the basics, it's time to learn about more complex stuff:
- Segment Trees - These help you work with ranges of numbers in an array more efficiently, especially when you have to make a lot of updates or ask a lot of questions about those ranges.
- Fenwick Trees (Binary Indexed Trees) - Similar to segment trees, these are good for when you need to update numbers in an array and ask about the sum of a range of numbers quickly.
- Dynamic Programming - This technique lets you solve tricky problems by breaking them down into smaller problems and remembering the solutions to those smaller problems.
- String Algorithms - These are special tricks for dealing with strings, like finding patterns or matching strings efficiently.
Learning these topics will let you tackle a wider range of problems.
Targeting Prestigious Contests
When you feel ready, try entering some of the big contests:
- Google Code Jam - A huge contest with tough challenges. Doing well can get you noticed by Google.
- Facebook Hacker Cup - An annual contest with lots of problem-solving. Good performance might get you an interview at Facebook.
- ACM-ICPC - A team contest where you work with others to solve as many problems as you can in 5 hours. It's a big deal in the coding world.
Joining these contests is a great way to see how you stack up against top coders from all over, and it can even help your career.
Conclusion
Starting competitive programming might look tough, but it's definitely something you can get the hang of if you approach it the right way. Here's a simple breakdown to help you get started and keep improving.
Starting Out
When you're just beginning with competitive programming:
- Choose Python or Java at first. They're easier to learn, so you can focus on solving problems instead of getting stuck on complicated code.
- Try websites like Codeforces where problems are sorted by how hard they are. This lets you start easy and gradually take on tougher challenges.
- Always read the problem descriptions carefully to make sure you understand what's being asked before you dive into coding.
Learning Effectively
To get better over time:
- Work on a few new problems every day and revisit old ones to help remember what you've learned.
- Look at solutions for problems you've solved to see if there's a smarter way to do them.
- Keep notes on new things you learn, like different ways to organize data or steps to solve problems.
Building Consistency
To make competitive programming a regular part of your life:
- Code regularly, not just once in a while.
- Join contests to practice under pressure and see how well you're doing.
- Identify your weak spots and focus on getting better in those areas through practice.
Advancing Skills
To move up to more challenging stuff:
- Start learning about tougher topics like dynamic programming, handling strings, and using special trees.
- Try out big contests like Google Code Jam to see how you stack up against others.
- Think about joining a group or club where you can learn from others who are also into competitive programming.
With steady practice and focusing on areas where you need to improve, you'll start finding those tricky coding problems a lot more manageable. Set small goals for yourself, keep track of your progress, and celebrate the wins along the way. Before you know it, you'll be tackling those challenges with confidence.
Related Questions
Which competitive coding platform is best for beginners?
For those just starting with competitive programming, here are some top sites:
- Codeforces - It sorts problems by how hard they are, so you can slowly take on more challenging ones. Great for learning about algorithms.
- CodeChef - Offers lots of practice problems sorted by topic and difficulty. It's a good place to get to grips with different coding concepts.
- LeetCode - Has problems ranked from easy to hard. It's especially good if you're preparing for job interviews.
- HackerRank - Offers a wide range of coding challenges at different levels.
- Topcoder - Not only does it host contests, but it also provides tutorials on competitive programming.
These websites are great for finding problems that match your current skill level.
Can a beginner do competitive programming?
Absolutely, beginners can dive into competitive programming. Start with these steps:
- Pick up a basic programming language like C++, Java, or Python.
- Get comfortable breaking down problems into smaller parts you can handle.
- Work on combining solutions for small problems into bigger ones.
- Tackle practice problems on sites that offer a range of difficulties.
With regular practice, beginners can improve their skills and gain confidence in competitive programming. Look for contests that fit your level to help you grow.
What is the best language for competitive programming for beginners?
Python is often recommended for beginners because:
- It's straightforward to learn, which lets you focus more on problem-solving.
- There's a lot of helpful resources and a supportive community.
- While it's a bit slower, it's a good place to start learning how to develop solutions.
Java and C++ are also common choices but might be a bit harder to learn at first. Python is great for starting out because it lets you concentrate on learning how to solve problems.
Where to start with competitive programming?
If you're new to this, here's a simple plan:
- Pick a programming language to learn, like Python, Java, or C++.
- Begin practicing on websites like CodeChef or Codeforces.
- Learn about data structures and algorithms.
- Start with easy practice problems.
- Try out some short contests to get used to coding quickly.
- Look at how others solved problems you couldn't.
Starting with problems that match your skill and gradually increasing the difficulty is a good strategy. Keep practicing, and you'll get better over time.