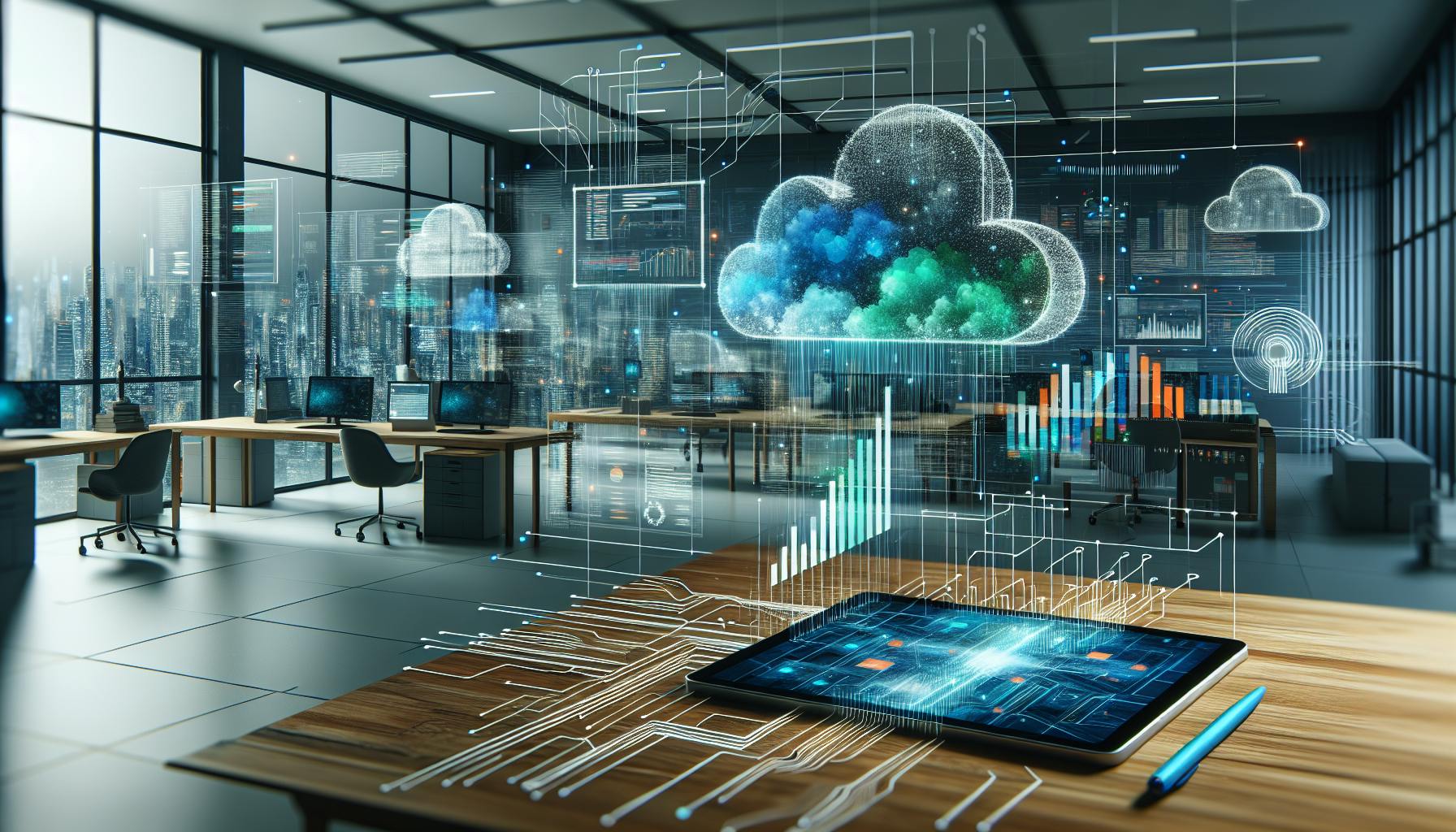
Learn how to effectively profile cloud app performance to reduce costs, enhance user experience, and troubleshoot issues before they escalate.
Cloud app performance profiling helps you:
- Find what slows down your app
- Cut cloud costs
- Improve user experience
- Catch issues before they grow
Here's what you need to know:
-
Focus on key metrics:
- CPU use
- Memory use
- Network speed
- Disk speed
-
Set up profiling:
- Choose tools for your language and cloud
- Enable profiling APIs
- Add profiling code to your app
-
Run regular checks:
- Test with each code update
- Monitor live for critical apps
-
Fix issues:
- Optimize code
- Scale your app
- Redesign inefficient parts
-
Make it routine:
- Add to your build process
- Set up alerts
- Track normal performance
Tool | Best For | Key Feature |
---|---|---|
Datadog | Full-stack | Real-time dashboards |
Pyroscope | Continuous | Leak detection |
Amazon CodeGuru | AWS apps | AI-powered baselines |
Google Cloud Profiler | Multi-cloud | Flame graphs |
Pick a tool that fits your setup, language, and budget.
Related video from YouTube
What You Need to Know First
Let's cover the essentials before we jump into cloud app performance profiling.
Cloud Basics
Cloud apps run on shared resources. This means other factors can impact your app's performance:
- How resources are allocated
- Network delays
- Storage speed
- Other apps using the same infrastructure
Knowing these helps you spot potential slowdowns.
Key Performance Measures
Focus on these core metrics when profiling your cloud app:
Metric | What It Is | Why It's Important |
---|---|---|
CPU Use | % of compute power used | Shows if your app is maxing out the processor |
Memory Use | RAM your app uses | Spots memory leaks or inefficient code |
Network Speed | How fast data moves | Affects user experience and app responsiveness |
Disk Speed | How fast data is read/written | Impacts data processing and retrieval times |
Tools and Permissions
To start profiling, you'll need:
1. Access rights: Make sure you can monitor and change your cloud resources.
2. Monitoring tools: Use your cloud provider's built-in services (like Amazon CloudWatch or Azure Monitor).
3. Profiling software: Pick tools that fit your app's language and framework:
4. Data storage: Set up a system to store and analyze your profiling data.
Setting Up for Profiling
Let's get your cloud app ready for performance profiling.
Picking Tools
Choose tools that fit your app's language and cloud platform:
Language | Tools |
---|---|
Go | GCP Cloud Profiler |
Java | Azure App Insights Profiler, GCP Cloud Profiler |
Node.js | GCP Cloud Profiler |
Python | GCP Cloud Profiler |
.NET | Azure App Insights Profiler |
Cloud Platform Setup
1. Enable profiling API
GCP:
gcloud services enable cloudprofiler.googleapis.com
Azure: Turn on App Insights in your App Service settings.
2. Set access rights
Make sure you can monitor and change cloud resources.
3. Data storage
GCP keeps data for 30 days. For longer, set up a system to save profiles.
Adding Profiling to Your App
1. Install profiler
Python on GCP:
pip3 install google-cloud-profiler
.NET on Azure: It's already there in App Service.
2. Start profiling
Python on GCP:
import googlecloudprofiler
def main():
googlecloudprofiler.start(
service='your-service-name',
service_version='v1',
project_id='your-project-id'
)
# Your app code here
.NET on Azure: No extra code needed if App Insights is on.
3. Check it's working
Run your app and look for messages about profile creation and uploads.
Types of Performance Checks
When profiling cloud apps, focus on CPU, memory, network, and disk. Here's a breakdown:
CPU Use
CPU usage is your app's "brain" at work. High CPU? Your app slows down.
To check:
- Install a VM monitoring agent
- Use cloud platform tools (like GCP's Monitoring Agent)
- Spot CPU-hungry processes
Quick GCP setup:
curl -sSO https://dl.google.com/cloudagents/add-monitoring-agent-repo.sh
sudo bash add-monitoring-agent-repo.sh
sudo apt-get update
sudo apt-get install stackdriver-agent
Memory Use
Memory checks catch leaks and crashes. How to monitor:
- Use cloud platform tools
- Watch for growing memory use
- Set high-use alerts
Network Speed
Slow networks = slow apps. Check by:
- Measuring app-to-dependency latency
- Tracking data transfer rates
- Finding network bottlenecks
Disk Speed
Disk I/O can bog down apps. Monitor with:
- Linux tools like
iostat
- Cloud platform disk metrics
Azure's helpful disk metrics:
Metric | Measures |
---|---|
Data Disk IOPS Consumed Percentage | Data disk IOPS limit usage |
OS Disk Bandwidth Consumed Percentage | OS disk bandwidth usage |
VM Cached IOPS Consumed Percentage | VM cached IOPS usage |
Regular checks give you a full performance picture. Spot and fix issues before users notice.
Gathering and Understanding Data
Let's dive into how to collect and interpret profiling data to boost your cloud app's performance.
How Often to Check
Your app's needs and dev cycle dictate profiling frequency. Here's a general guide:
- Run automated tests with each code commit
- Do monthly performance tests or with big updates
- For critical apps, monitor 24/7
Pyroscope offers round-the-clock monitoring, catching issues that might slip through less frequent checks.
Live vs. Test Environments
Both live and test profiling have their perks:
Environment | Pros | Cons |
---|---|---|
Live | Real data, Actual load | User impact risk, Less control |
Test | Safe experiments, Controlled | Might miss real scenarios |
Use both for best results. Test environments catch issues early, while live profiling ensures real-world performance.
Reading Performance Graphs
Performance graphs are your secret weapon. Here's how to use them:
- Spot CPU, memory, or network usage spikes
- Compare patterns across time scales
- Link performance data to user activity or system events
"At Pyroscope, profiling our own servers has saved us tons of time and money by spotting performance issues", says Uchechukwu Obasi, Frontend Developer at Grafana.
When analyzing:
- Use the 95th percentile for problem bursts
- Look for metric correlations
- Track trends to predict future issues
sbb-itb-bfaad5b
Making Your App Faster
You've got your profiling data. Now what? Let's boost your cloud app's speed:
Fixing Code
Optimize based on what you've learned:
- Cut down database queries. Cache that data!
- Streamline those algorithms. Ditch inefficient loops.
- Batch API requests. Less network chatter = happy app.
"We zero in on indexing and query optimization. It's our database efficiency secret sauce." - Thomas Radosh, DevOps Engineer
Growing Your App
Time to scale up:
1. Load balancing
Spread that traffic across servers. It's like giving your app a breather. Bonus? Up to 30% less latency.
2. Auto-scaling
Let your app flex its muscles when needed. Here's a quick guide:
Metric | Threshold | Action |
---|---|---|
CPU usage | > 70% | Add 1 instance |
Memory usage | > 80% | Increase instance size |
Request count | > 1000/min | Add 2 instances |
3. CDNs
Bring content closer to users. It's like opening a corner store instead of making everyone drive to the supermarket.
Better App Design
Rethink your app's structure:
- Break it down into microservices. Smaller pieces, easier scaling.
- Go serverless. Less infrastructure headaches, more scalability.
- Embrace event-driven architecture. Your app will thank you for the responsiveness boost.
Tips for Ongoing Profiling
Here's how to make profiling a regular part of your cloud app development:
Adding to Your Build Process
Integrate profiling into your CI/CD pipeline:
- Use tools like Datadog APM for automatic performance data collection
- Set up performance gates to block deployments if metrics fall below thresholds
- Include profiling results in build reports
"Adding profiling to our build process caught a 15% CPU spike that would've hit 30% of our users", says Sarah Chen, Lead DevOps Engineer at CloudScale Solutions.
Setting Up Warnings
Create an alert system:
Metric | Threshold | Action |
---|---|---|
CPU usage | > 80% for 5 min | Notify on-call engineer |
Memory leak | > 10% growth/hour | Trigger auto-scaling |
Response time | > 500ms for 100 requests | Page dev team |
To avoid false alarms:
- Compare to baselines
- Retest immediately
- Increase observation windows
Keeping Track of Normal Performance
Establish performance baselines:
1. Set initial benchmarks
Run tests before cloud migration to set targets.
2. Monitor consistently
Use continuous profiling to collect key metrics.
3. Review regularly
Schedule monthly reviews to spot trends and adjust baselines.
"Continuous profiling helped us find a database query 30% slower than our baseline. Fixing it boosted app speed by 12%", says Alex Patel, CTO of DataStream Inc.
Fixing Profiling Problems
Cloud app profiling can be tricky. Here's how to tackle common issues:
Dealing with Missing Data
Missing data messes up your results. Here's what to do:
1. Check your setup
Make sure your tools are configured right. Turn on debug mode in your SDK to spot problems.
2. Fill in the gaps
When data's missing, try these:
Technique | What It Does | Best For |
---|---|---|
Mean/Median | Uses averages | Numbers |
KNN | Estimates from similar data | Complex sets |
Model-based | Predicts with stats | Big datasets |
3. Back it up
Set up auto-backups to save your profiling data.
When Tools Clash
Tool conflicts can mess up your analysis. Try this:
- Check if your tools work with your cloud platform
- Use one monitoring platform to avoid fights
- Look into middleware to connect incompatible tools
"We fixed our tool issues by switching to cloud-native profiling. It cut troubleshooting time by 40%." - Alex Chen, CTO, CloudTech Solutions
Keeping Data Safe
Protect sensitive info during profiling:
- Encrypt profiling data
- Control who sees the results
- Mask sensitive data before analysis
You AND your cloud provider are responsible for data security.
"After masking data in our profiling, 30% more clients joined our performance studies." - Sarah Lee, Data Security Lead, SecureCloud Inc.
Wrap-Up
Cloud app performance profiling keeps your apps running smoothly. Here's what you need to know:
1. Pick the right tools
Choose profilers that work with your cloud platform and track CPU, memory, network, and disk use.
2. Check smart, not hard
Don't wait for issues. Set up regular checks:
Environment | Frequency | Why |
---|---|---|
Production | Daily | Catch real issues |
Test | Pre-release | Prevent problems |
3. Act on insights
Use profiling data to:
- Fix code issues
- Scale your app
- Redesign inefficient parts
4. Make it automatic
Add profiling to your build process and set up alerts.
5. Be ready for challenges
Prepare for:
- Data gaps
- Tool conflicts
- Security concerns
Cloud environments change fast, so keep checking.
"Daily profiling cut our cloud costs by 22% in three months. We fixed inefficiencies we didn't know about." - Maria Rodriguez, CTO of CloudScale Solutions
Extra: Comparing Profiling Tools
Choosing the right profiling tool for your cloud app can be tricky. Let's break down some popular options:
Tool | Best For | Key Features | Pros | Cons |
---|---|---|---|---|
Datadog | Full-stack monitoring | Real-time dashboards, anomaly detection | Wide integrations, efficient detection | Complex setup |
Pyroscope | Continuous profiling | High-performance instrumentation, leak detection | Real-time insights, open-source | UI can be tricky |
Amazon CodeGuru | AWS applications | AI-powered performance baselining | Good for dev and production | AWS-only |
Google Cloud Profiler | Multi-cloud | Continuous profiling, flame graphs | Free for Google Cloud, cross-platform | Fewer features |
Here's the scoop on each tool:
Datadog is your go-to for broad application monitoring. It plays nice with lots of systems.
Pyroscope is all about continuous profiling. It's great at spotting memory leaks early on.
Amazon CodeGuru uses AI to set performance baselines. It's perfect if you're an AWS fan.
Google Cloud Profiler works across different cloud platforms, not just Google's.
When picking a tool, think about:
- Your cloud setup
- What programming languages you use
- The performance metrics you care about
- How much you can spend
"Pyroscope caught a memory leak that was burning $2,000 a month in cloud resources we didn't need", says Tom Chen, CTO of CloudStack Solutions.
Bottom line: Each tool has its strengths. Choose the one that fits your needs and budget best.
FAQs
What is a cloud profiler?
A cloud profiler is a tool that keeps an eye on your app's CPU and memory use without slowing it down much. It's like having a friendly robot watching your app 24/7, taking notes on what's using up resources.
Here's what cloud profilers do:
- Watch your app non-stop
- Use very little resources themselves
- Show you which parts of your code are resource-hungry
- Make data easy to understand with cool visuals
For instance, Google Cloud Profiler barely impacts your app - it only uses about 5% of CPU and memory while it's collecting data, and less than 0.5% on average.
How does cloud profiling boost app performance?
Cloud profiling is like a personal trainer for your app. It helps by:
- Spotting the code that's hogging resources
- Making your queries faster
- Cutting down on memory waste
Here's a real example: Someone set up an app that wasn't running well. They used Cloud Profiler to find the problems and fix them, making the app much faster.
What should I look for in a cloud profiler?
When picking a cloud profiler, think about:
What to consider | Why it matters |
---|---|
Works with your cloud | Make sure it plays nice with AWS, Google Cloud, etc. |
Supports your coding language | It should understand what you're writing |
Doesn't slow things down | You want a lightweight tool |
Fits your workflow | It should be easy to use with your current setup |
Keeps data long enough | Some tools store data for 7-15 days |
How do I start cloud profiling?
Getting started with cloud profiling is pretty straightforward:
1. Turn on the profiler API (like the Cloud Profiler API in Google Cloud)
2. Make sure your app meets the requirements (e.g., right version of .NET Framework)
3. Set up your app to work with the profiler
4. Keep an eye on the data and look for ways to make your app better