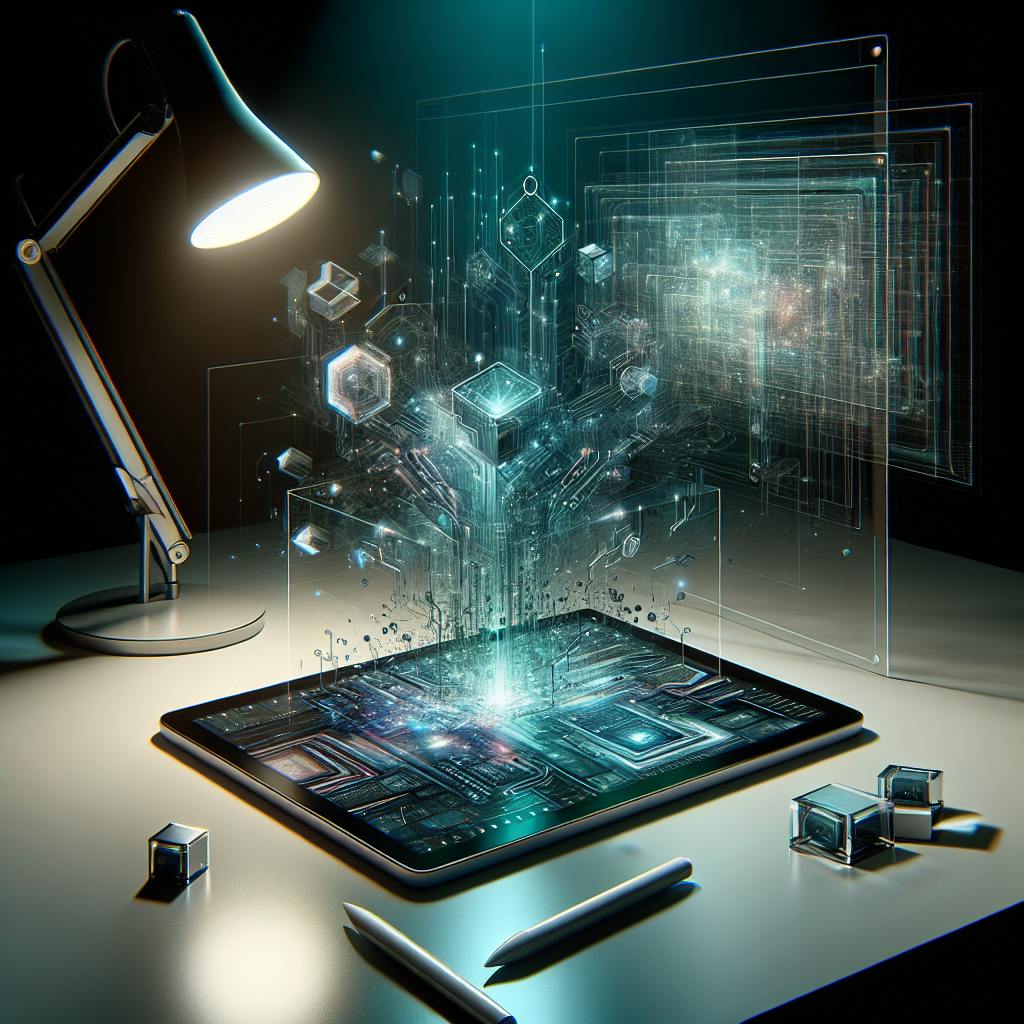
Discover advanced drawing techniques with Core Graphics on iOS, including complex methods, performance optimization, integration with Core Animation, and more.
Core Graphics is a powerful 2D graphics framework for iOS that lets you create high-quality visuals efficiently. This guide covers advanced techniques to improve your app's graphics:
- Complex drawing methods (paths, gradients, transformations)
- Performance optimization
- Integration with Core Animation
- Handling PDFs and vector graphics
- Using Metal for custom drawing
Key takeaways:
Feature | Benefit |
---|---|
C-based API | Fast and efficient |
Low-level drawing tools | Create custom UI elements |
GPU acceleration | Smoother animations |
Vector graphics support | Scalable, resolution-independent graphics |
To get started:
- Install latest Xcode
- Create a new iOS project
- Import Core Graphics framework
This guide assumes basic Swift and iOS development knowledge. By the end, you'll be able to create advanced graphics and optimize performance in your iOS apps.
Related video from YouTube
Prerequisites
Knowledge and Skills You Need
To work with advanced Core Graphics, you should know:
- Swift programming basics
- Simple graphics concepts
- How to use Xcode
Setting Up Your Development Environment
Here's what you need to do:
- Install the latest Xcode on your Mac
- Create a new iOS project in Xcode
This gives you a blank slate to try out different drawing methods.
Core Graphics Basics
If you're new to Core Graphics, review these key concepts:
Concept | Description |
---|---|
Coordinates | How to place items on the screen |
Paths | Ways to draw lines and shapes |
Colors | How to add and mix colors in your drawings |
Understanding these basics will help you learn more complex techniques.
Core Graphics Basics
Learn the main ideas and terms for understanding Core Graphics.
Key Concepts and Terms
To use Core Graphics, you need to know these main ideas:
Concept | Description |
---|---|
Graphics Context | The space where you draw |
Paths | Lines that make shapes |
Drawing Operations | Actions like drawing lines or filling shapes |
Knowing these ideas helps you make better graphics in your iOS app.
How the Coordinate System Works
Core Graphics uses a grid system:
- (0, 0) is at the top-left corner
- X goes right
- Y goes down
For example, (10, 20) is 10 points right and 20 points down from the top-left corner.
Understanding Graphics Contexts
Graphics contexts are where you draw in Core Graphics. Here's how to use them:
- Make a context with
UIGraphicsImageRenderer
- Draw shapes, lines, or text in the context
Here's an example of drawing a red square:
let renderer = UIGraphicsImageRenderer(size: CGSize(width: 100, height: 100))
let img = renderer.image { ctx in
ctx.cgContext.setFillColor(UIColor.red.cgColor)
ctx.cgContext.fill(CGRect(x: 0, y: 0, width: 100, height: 100))
}
This code makes a 100x100 context and fills it with a red square.
Advanced Drawing Methods
Core Graphics offers many ways to create complex graphics. Let's look at some of these methods.
Drawing with Paths
Paths help you make complex shapes. Here's how to create a triangle:
override func draw(_ rect: CGRect) {
guard let context = UIGraphicsGetCurrentContext() else { return }
let trianglePath = CGMutablePath()
let vertex1 = CGPoint(x: rect.midX, y: rect.minY)
let vertex2 = CGPoint(x: rect.minX, y: rect.maxY)
let vertex3 = CGPoint(x: rect.maxX, y: rect.maxY)
trianglePath.move(to: vertex1)
trianglePath.addLine(to: vertex2)
trianglePath.addLine(to: vertex3)
trianglePath.closeSubpath()
context.addPath(trianglePath)
UIColor.green.setFill()
context.fillPath()
}
This code makes a green triangle. You can add more points to create more complex shapes.
Using Gradients and Patterns
Gradients can make your graphics look better. Here's how to make a simple gradient:
override func draw(_ rect: CGRect) {
guard let context = UIGraphicsGetCurrentContext() else { return }
let colorSpace = CGColorSpaceCreateDeviceRGB()
let colors = [UIColor.red.cgColor, UIColor.yellow.cgColor]
let locations: [CGFloat] = [0, 1]
guard let gradient = CGGradient(colorsSpace: colorSpace, colors: colors as CFArray, locations: locations) else {
return
}
let startPoint = CGPoint(x: rect.minX, y: rect.midY)
let endPoint = CGPoint(x: rect.maxX, y: rect.midY)
context.drawLinearGradient(gradient, start: startPoint, end: endPoint, options: [])
}
This code creates a gradient from red to yellow. You can change the colors and points to make different gradients.
Applying Transformations
Transformations let you change your drawings. Here's how to rotate a shape:
override func draw(_ rect: CGRect) {
guard let context = UIGraphicsGetCurrentContext() else { return }
context.saveGState()
context.translateBy(x: rect.width / 2, y: rect.height / 2)
context.rotate(by: CGFloat.pi / 4)
let rectToDraw = CGRect(x: -50, y: -25, width: 100, height: 50)
context.addRect(rectToDraw)
UIColor.blue.setFill()
context.fillPath()
context.restoreGState()
}
This code rotates a blue rectangle by 45 degrees.
Clipping and Masking Techniques
Clipping lets you limit where you draw. Here's an example:
override func draw(_ rect: CGRect) {
guard let context = UIGraphicsGetCurrentContext() else { return }
context.saveGState()
let circlePath = UIBezierPath(ovalIn: rect.insetBy(dx: 20, dy: 20))
circlePath.addClip()
UIColor.purple.setFill()
context.fill(rect)
context.restoreGState()
}
This code makes a purple circle by clipping a rectangle.
Blending and Compositing
You can mix layers in different ways. Here's an example:
if let img = UIImage(named: "example"), let img2 = UIImage(named: "example2") {
let rect = CGRect(x: 0, y: 0, width: img.size.width, height: img.size.height)
let renderer = UIGraphicsImageRenderer(size: img.size)
let result = renderer.image { ctx in
UIColor.white.set()
ctx.fill(rect)
img.draw(in: rect, blendMode: .normal, alpha: 1)
img2.draw(in: rect, blendMode: .luminosity, alpha: 1)
}
}
This code mixes two images using a special blend mode.
Working with Text and Fonts
You can draw text with Core Graphics. Here's a basic example:
override func draw(_ rect: CGRect) {
guard let context = UIGraphicsGetCurrentContext() else { return }
let text = "Hello, Core Graphics!"
let font = UIFont.systemFont(ofSize: 24)
let textAttributes: [NSAttributedString.Key: Any] = [
.font: font,
.foregroundColor: UIColor.black
]
let textSize = text.size(withAttributes: textAttributes)
let textRect = CGRect(x: (rect.width - textSize.width) / 2,
y: (rect.height - textSize.height) / 2,
width: textSize.width,
height: textSize.height)
text.draw(in: textRect, withAttributes: textAttributes)
}
This code draws centered text. You can change the font, color, and other text settings.
Image Processing
Core Graphics lets you work with images. Here's how to mix two images:
override func draw(_ rect: CGRect) {
guard let context = UIGraphicsGetCurrentContext(),
let image = UIImage(named: "example"),
let overlayImage = UIImage(named: "overlay") else { return }
image.draw(in: rect)
context.setBlendMode(.overlay)
overlayImage.draw(in: rect)
}
This code puts one image on top of another using a blend mode.
Improving Performance
Making Core Graphics run faster is key for a good user experience. Here's how to speed up your graphics code.
Finding and Fixing Slow Points
To make your app faster:
- Use Xcode's Instruments tool to find slow parts
- Look for:
- Complex shapes or paths
- Too many setNeedsDisplay() calls
- Slow graphics tasks like image processing
To fix slow parts:
- Make shapes simpler
- Use fewer setNeedsDisplay() calls
- Save results of slow tasks for later use
Managing Memory Well
Good memory use helps avoid crashes. Here's what to do:
Do | Don't |
---|---|
Use autorelease pools for temporary objects | Keep unnecessary objects |
Use weak references to avoid loops | Forget to check for memory leaks |
Using Hardware Acceleration
Using the GPU can make graphics faster. Try these:
- Use CALayer and Core Animation for moving graphics
- Turn on GPU speed-up for some graphics tasks
- Use Metal for custom graphics if needed
This can make your app run smoother and look better.
sbb-itb-bfaad5b
Working with Core Animation
Core Graphics and Core Animation can work together to create moving drawings. This section shows how to combine these tools for better visuals.
Mixing Core Graphics and Core Animation
Core Graphics makes still pictures. Core Animation makes things move. When you use both, you can create lively, interactive screens.
To mix them:
- Draw with Core Graphics
- Put the drawing on a CALayer
- Move the layer with Core Animation
This method lets you use the best parts of both tools.
Making Drawings Move
To make a Core Graphics drawing move:
- Create a CABasicAnimation
- Set its properties
- Add it to a layer
Here's a simple example:
let animation = CABasicAnimation(keyPath: "position.x")
animation.fromValue = 0
animation.toValue = 200
animation.duration = 1.0
layer.add(animation, forKey: "positionAnimate")
This code moves a layer from left to right over 1 second.
Smooth Animation Tips
To make animations run smoothly:
Tip | Explanation |
---|---|
Use CALayer and Core Animation | Best for moving graphics |
Turn on GPU speed-up | Makes some tasks faster |
Use Metal for custom graphics | If you need more speed |
Avoid complex shapes | They can slow things down |
Use Instruments | Find and fix slow parts |
Good Practices and Common Errors
Writing Clear Code
When using Core Graphics, it's important to write easy-to-understand code. Here are some tips:
Tip | Example |
---|---|
Use clear names | drawCircle() instead of dc() |
Add helpful comments | // Draw the background |
Keep code simple | Avoid nested loops |
Use spaces wisely | Add blank lines between sections |
Following these tips will make your code easier to read and fix.
Debugging Core Graphics
Finding and fixing Core Graphics problems can be hard. Here are some tools to help:
Tool | What it does |
---|---|
Xcode debugger | Lets you step through code |
Core Graphics debugging | Shows drawing issues |
Instruments | Finds slow parts of your app |
Device testing | Checks how your app works on different iPhones |
Using these tools will help you find and fix issues faster.
Mistakes to Avoid
Here are some common Core Graphics mistakes and how to avoid them:
Mistake | How to avoid it |
---|---|
Blurry images | Draw on whole pixels |
Using the wrong tool | Use Core Animation for moving graphics |
Slow code | Check your code's speed often |
Unexpected behavior | Test your app thoroughly |
More Complex Topics
Handling PDFs and Vector Graphics
Core Graphics lets you work with PDFs and vector graphics in your iOS app. Here's what you can do:
Feature | Description |
---|---|
PDF rendering | Turn PDF pages into images |
Vector graphics | Create custom shapes and curves |
To use PDFs:
- Make a
CGPDFDocument
from a PDF file - Use
CGPDFPage
to get each page - Draw pages with
CGContextDrawPDFPage
For vector graphics, use CGPath
or UIBezierPath
to make shapes.
Custom Drawing with Metal
Metal is a tool for fast graphics on iOS. It works with the device's GPU. Here's how to use it:
- Make a
MTLDevice
for the GPU - Create a
MTLCommandQueue
to run GPU tasks - Use
CGContextCreateMetal
to mix Core Graphics and Metal
Metal helps with:
- 3D graphics
- Fast image editing
- Machine learning tasks
New Features in Recent iOS Versions
iOS updates have added new things to Core Graphics:
Feature | What it does |
---|---|
UIImage improvements |
Supports animated GIFs and WebP images |
CGImage updates |
Better alpha channels and color management |
Performance boost | Faster drawing and less memory use |
These changes make Core Graphics better for making good-looking graphics in your app.
Wrap-up
This guide has covered many ways to use Core Graphics for advanced drawing in iOS apps. We've looked at:
- Core Graphics basics
- Complex drawing methods
- Making drawings run faster
- Using Core Graphics with Core Animation
- Good coding practices
- Fixing common errors
We also touched on more complex topics:
Topic | Description |
---|---|
PDFs and vector graphics | How to work with these in your app |
Metal for custom drawing | Using the GPU for faster graphics |
New iOS features | Recent updates to Core Graphics |
As you keep working with Core Graphics, remember to:
- Keep learning about new iOS graphics tools
- Practice using different drawing techniques
- Test your app's graphics on various devices
FAQs
What is the difference between Core Animation and Core Graphics?
Core Animation and Core Graphics are two key tools for iOS graphics, but they work differently:
Feature | Core Animation | Core Graphics |
---|---|---|
Processing | Uses GPU | Uses CPU |
Best for | Moving graphics | Complex drawing |
Speed | Faster for animations | Slower for animations |
Use case | Smooth UI movements | Detailed custom graphics |
Core Animation is good for:
- Moving UI elements
- Smooth transitions
- Simple animations
Core Graphics is good for:
- Drawing custom shapes
- Making complex graphics
- Detailed image editing
When building your iOS app, pick the right tool for each task. Use Core Animation for moving parts and Core Graphics for detailed drawings. This helps your app run smoothly and look good.