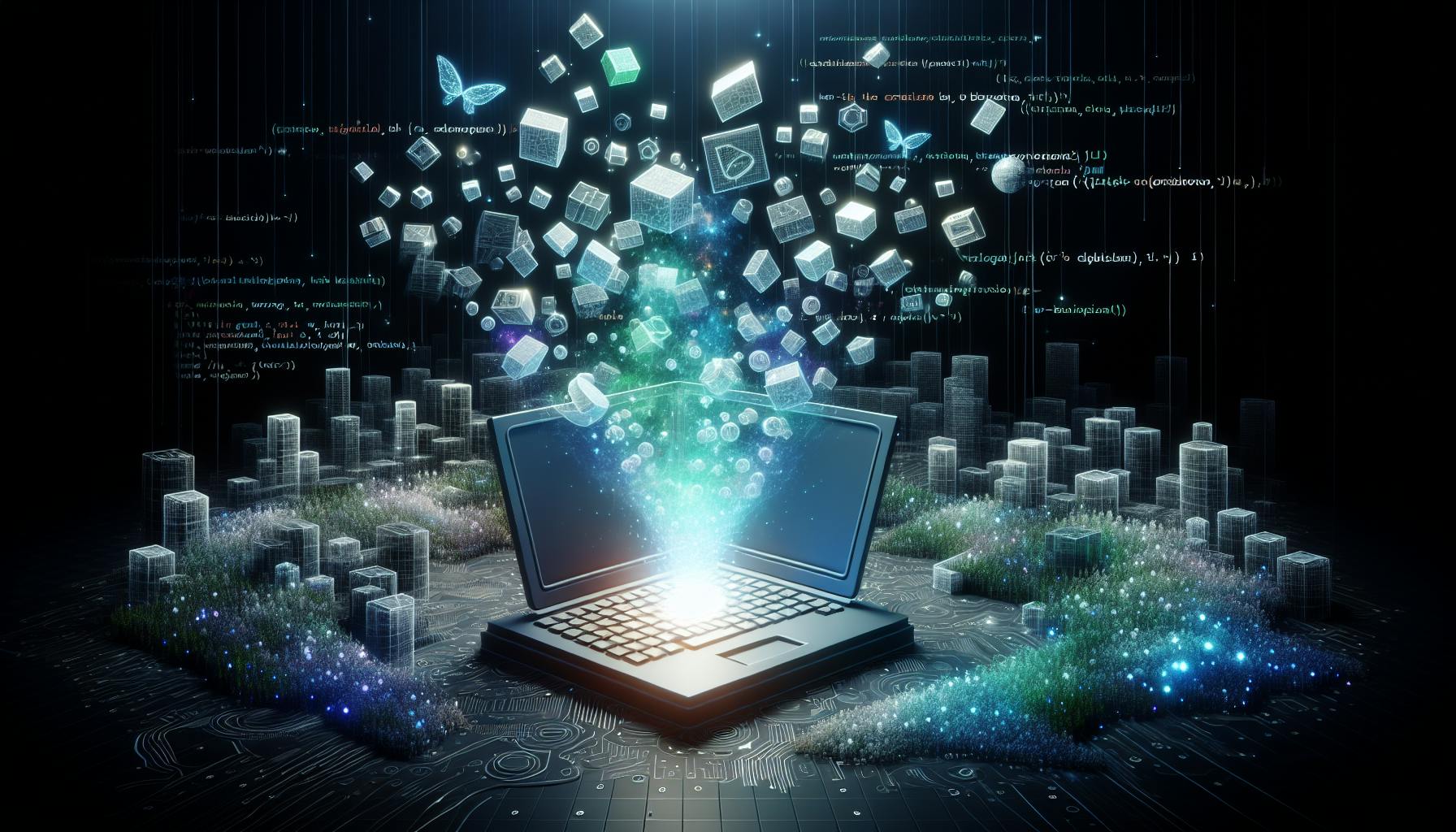
Learn the basics of Django, including models, views, URLs, and templates. Discover why Django is a great choice for web development and explore the advantages it offers. Get started with building a simple blog using Django.
Django is a powerful tool for creating websites, designed to help you build applications quickly and with less code. It's built with Python, making it a great choice for programmers familiar with the language. This guide will walk you through the basics of Django, covering everything from installation to building your first project, and diving into core components like models, views, URLs, and templates. Whether you're new to web development or just new to Django, here's what you need to know to get started:
- Why Django is useful: It's a full-stack framework that includes everything you need to build a website, from handling databases to user authentication, all with the goal of simplifying the web development process.
- Getting Started: Installation is straightforward, requiring Python 3, and then setting up Django in a virtual environment. The initial setup involves creating a project and understanding its structure.
- Building a Simple Application: Step-by-step guide to creating a basic blog, including setting up models for your data, creating views to control what users see, and using templates for the layout.
- Core Components: An overview of Django's models, views, URLs, and templates, and how they work together to build web applications.
- Advantages: Django is scalable, secure, and comes with a plethora of built-in features, making it a good choice for both beginners and experienced developers alike.
- Community and Resources: There's a vibrant community and a wealth of learning resources available to help you get the most out of Django.
This guide aims to provide a clear introduction to Django, showing you how to create web applications efficiently. With its 'batteries-included' approach, Django allows you to focus on writing your app without needing to reinvent the wheel.
Core Components of Django
In Django, building a website involves a few main parts: models, views, URLs, and templates. Let's break down what each of these does in simple terms.
Models
Think of models like blueprints for the information your website deals with. They help Django talk to the database in a way that doesn't require you to write complex database code. Here's what you need to know about models:
- They outline what kind of data your website works with (like a User or a Product).
- They manage all the database stuff for you.
- They make sure the data fits the rules you've set (like making sure an email address looks right).
- You can add special code to them for things you need your data to do.
Here's a simple example:
from django.db import models
class User(models.Model):
first_name = models.CharField(max_length=50)
last_name = models.CharField(max_length=50)
email = models.EmailField(unique=True)
def __str__(self):
return f"{self.first_name} {self.last_name}"
This code sets up a User with a first name, last name, and unique email, plus a way to display the user's name.
Views
Views are where Django decides what to show someone when they visit a page on your site. There are a few kinds of views:
- Function-based views: These are simple functions that get a request, do something, and then send back a webpage or data.
- Class-based views: These let you reuse code for common page patterns.
- Generic class-based views: Django provides these to make typical web page tasks easier, like showing or editing a list of items.
Example of a view:
from django.http import HttpResponse
from django.shortcuts import render
def home(request):
return HttpResponse("Welcome!")
def user_page(request, username):
context = {'username': username}
return render(request, 'user.html', context)
This shows how views can send back simple messages or use templates to show more complex pages.
URLs and Templates
URLs connect web addresses to the views. This means when someone goes to a certain address on your website, Django knows which view to use.
from django.urls import path
from . import views
urlpatterns = [
path('', views.home, name='home'),
path('user/<str:username>/', views.user_page, name='user_page'),
]
Templates are like patterns for the final page. They use placeholders for data that views send to them.
<!-- user.html template -->
<h1>Hello {{ username }}!</h1>
This template shows a greeting message using the username passed from a view.
Building a Simple Application
Let's create a basic blog using Django to see how it all fits together. This will help you understand how to make your own website with Django.
Set Up the Project
First, you need to create a new project. In your computer's command line, type:
django-admin startproject myblog
This command makes a new folder called myblog
that has everything you need to start your project.
Now, let's add a part to your project for the blog posts. Type this:
python manage.py startapp posts
This creates a section in your project where you'll work on the blog.
Build the Post Model
Go to posts/models.py
and make a model for your blog posts like this:
from django.db import models
class Post(models.Model):
title = models.CharField(max_length=100)
content = models.TextField()
date_posted = models.DateTimeField(auto_now_add=True)
This code sets up a way to keep track of your blog posts with a title, the post content, and the date it was posted.
To make this work with your database, type:
python manage.py makemigrations
python manage.py migrate
Create Views and URLs
Next, in posts/views.py
, write a view to show all the posts:
from django.shortcuts import render
from .models import Post
def home(request):
context = {
'posts': Post.objects.all()
}
return render(request, 'posts/home.html', context)
This takes the posts from your database and gets them ready to show on a webpage.
Now, tell Django how to find this page. In myblog/urls.py
, add:
from django.urls import path, include
urlpatterns = [
path('', include('posts.urls')),
]
And in posts/urls.py
, set up a path to your home view:
from django.urls import path
from . import views
urlpatterns = [
path('', views.home, name='blog-home'),
]
Create Templates
Make a file called home.html
in posts/templates/posts
and add:
{% for post in posts %}
<h2>{{ post.title }}</h2>
<p>{{ post.content }}</p>
{% endfor %}
This code will loop through each post and show its title and content on the page.
Run Development Server
Finally, to see your blog in action, start the server with:
python manage.py runserver
Then, go to http://127.0.0.1:8000
in your web browser. You should see your blog with the posts displayed.
This is just the beginning! You can add more features, like pages and forms, to make your blog do more things. This example shows you the basic steps of putting together a Django app.
Advantages of Using Django
Django is great for making websites because it comes with a lot of features that help you get the job done without having to start from scratch. Let's talk about why Django is a good choice for web development:
All-in-One Framework
Django has everything you need built in, so you don't have to piece together different parts:
- It has a system for users to log in, manage passwords, and set permissions.
- There's an admin area to help you manage your site's content.
- You can work with databases easily with its ORM (Object-Relational Mapping).
- Handling forms, checking data, and doing stuff with that data is simpler.
- For the look of your site, Django has templates.
- It makes your site faster by storing frequently used data.
- It keeps your site safe from common attacks.
This means you can build full websites faster and easier.
Well-Organized Code
Django keeps things tidy using an MTV (model-template-view) setup:
- Models: These are about your app's data and rules.
- Templates: They deal with how your site looks.
- Views: This is where the action happens, deciding what the user sees.
This setup makes your code easy to handle, even as your site grows.
High Scalability
Django can handle more users and more data without breaking a sweat. Big names like Instagram and National Geographic use Django because it can grow with them.
Enterprise-Ready
Django is ready for big projects right out of the box. It's got:
- User management and permissions
- Support for big databases
- Tools for background tasks
- An admin interface for managing content
- Support for APIs with XML/JSON
Here's a quick look at how Django stacks up against other web frameworks:
Feature | Django | Flask | Node/Express | Laravel |
---|---|---|---|---|
Admin Interface | โ | โ | โ | โ |
Database ORM | โ | โ | โ | โ |
User Authentication | โ | โ | โ | โ |
Template Engine | โ | โ | โ | โ |
Form Validation | โ | โ | โ | โ |
Caching | โ | โ | โ | โ |
Background Tasks | โ | โ | โ | โ |
In short, Django is perfect if you're looking for a framework that has everything you need to start building your site. It's designed to help you keep your code clean and easy to manage, no matter how big your site gets.
sbb-itb-bfaad5b
Django Community and Resources
Django has a really active and friendly community that's great for support and learning, no matter if you're just starting or have been coding for a while. Getting involved can really help you get better at using Django.
Learning Resources
Here are some good places to learn more about Django:
- Official Django Tutorials - The Django website has detailed guides that show you how to build different things with Django, like a polling app and how to set it up for people to use.
- Django for Everybody - This is a free online book by Dr. Charles Severance that covers the basics of Django, including how to work with databases.
- Django for Beginners - A book you can buy if you're new to Django. It talks about the basics like models, views, templates, and forms.
- SimpleIsBetterThanComplex Blog - A blog with lots of tips and tutorials for those who already know a bit about Django and want to learn more.
Community
- Django Forum - The official discussion forum for Django has over 93,000 members talking about Django, sharing advice, and helping each other out.
- Django Chat - For quick help with Django, you can chat in real-time on Gitter channels or the official Slack workspace.
- DjangoCon - Every year, DjangoCon conferences are held where experts give talks and you can meet other Django developers.
- Meetups - There are meetups all over the world for Django users to meet, learn from each other, and work on projects together. These often include talks and workshops.
Joining the Django community is a great way to improve your skills and help others learn too.
Conclusion
Django is a super handy tool for making all sorts of websites and apps. Whether you want to create a simple blog or a complex website that handles lots of data, Django has you covered with its many built-in features. It helps you build things quickly and keeps your code neat and easy to manage.
In this guide, we went over the basics of Django, including:
- Models - These help organize your app's data.
- Views - They decide what to show on your website.
- Templates - These control the look of your website.
- URLs - They connect web addresses to different parts of your site.
We also showed you how to put together a simple blog using Django to give you an idea of how everything works together.
Some great things about Django are:
- It comes with a lot of tools you'll need, so you don't have to build everything from scratch.
- It has an easy way to manage your site's content.
- You can work with databases easily.
- It has built-in ways to deal with user accounts and forms.
- You can make your site look nice with its front-end design options.
Plus, Django has a big community of people who use it and lots of resources to help you learn more.
To wrap up, Django is a great choice if you're looking to make web apps fast and efficiently. It has everything you need to get started and more, saving you from doing a lot of repetitive work. This way, you can focus on making your project unique.
If you're interested in diving deeper into web development with Django, here are some things you can do next:
- Try out the official Django tutorials.
- Make a basic CRUD app.
- Learn how to put your Django site online.
- Explore how to add user login to your site.
- Look into extra tools you can use to add more features to your site.
The Django community and online resources are great for learning more and getting help when you need it.
Appendix: Recommended Resources
Learning Django can be fun and rewarding. Here are some easy-to-follow resources that will help you get better:
Books
- Two Scoops of Django - A book that talks about how to do things the right way in Django. It covers a lot, like how to set up your project, how to make your website work with data, and how to show data on pages.
- Django for APIs - This book is all about making Django work with APIs (ways for different software to talk to each other). It's full of examples that make the ideas clear.
- Django for Professionals - This one is for when you're ready to take your Django skills to the next level. It goes into things like making your site ready for the public, keeping it safe, and other advanced topics.
Video Courses
-
Django 4 Course by Dennis Ivy on FreeCodeCamp - Perfect for beginners. It's a set of videos that show you how to start with Django, with lots of examples from the real world.
-
Complete Django Series by CodingEntrepreneurs - This is a paid course, but it's very detailed. It covers a lot of projects and shows you how to use Django with other tools.
Tutorials
-
Official Django Tutorials - The best place to start if you're new. These guides are made by the people who made Django, and they're really good at explaining things step by step.
-
SimpleIsBetterThanComplex Blog - A great blog for when you're ready to dig deeper into Django. It has articles on more complex topics and does a great job explaining them.
Community
- Django Forum - A place with over 93,000 members where you can ask questions and get answers about Django.
- Django Chat - Real-time chat rooms on Gitter and Slack where people talk about Django.
Mixing different types of learning resources, like reading books, watching videos, following tutorials, and joining community discussions, can really help you understand Django better. Keep practicing, and soon you'll be making your own advanced Django projects!
Related Questions
Can a beginner learn Django?
Yes, Django is great for beginners. It's made to help you build websites quickly and without too much trouble. If you know a bit of Python and understand the basics of how websites work, you can start with Django. There's a lot of help out there, like guides and tutorials, that make learning Django easier.
Can I learn Django in 3 days?
Learning Django really well in just 3 days is tough. To get a good handle on it, you'd usually need about 1-2 months if you work on it every day. But, in 3 days, you can learn some basics like:
- How to set up Django
- What a Django project looks like
- How to make simple web pages
- Connecting web addresses to your pages
- Adding HTML templates to make things look nice
This gives you a start, but there's a lot more to learn to get really good at it.
What is the introduction of Django?
Django is a tool for making websites with Python, a programming language. It was made in 2005 and is designed to help you build websites fast. Django does a lot of the heavy lifting for you, like:
- Working with databases
- Handling web requests and responses
- Making web pages with templates
- Managing users and their data
It's like a big box of tools for web development that saves you from having to build everything from scratch.
Is it worth learning Django in 2024?
Yes, learning Django is still a good idea in 2024. Big companies and lots of websites use it because it's reliable for handling lots of users and data. Django has a supportive community and lots of resources to help you learn. Plus, it teaches you good practices for building websites that are easy to maintain and update. With more businesses going online, knowing how to build web apps with Django can open up many job opportunities.