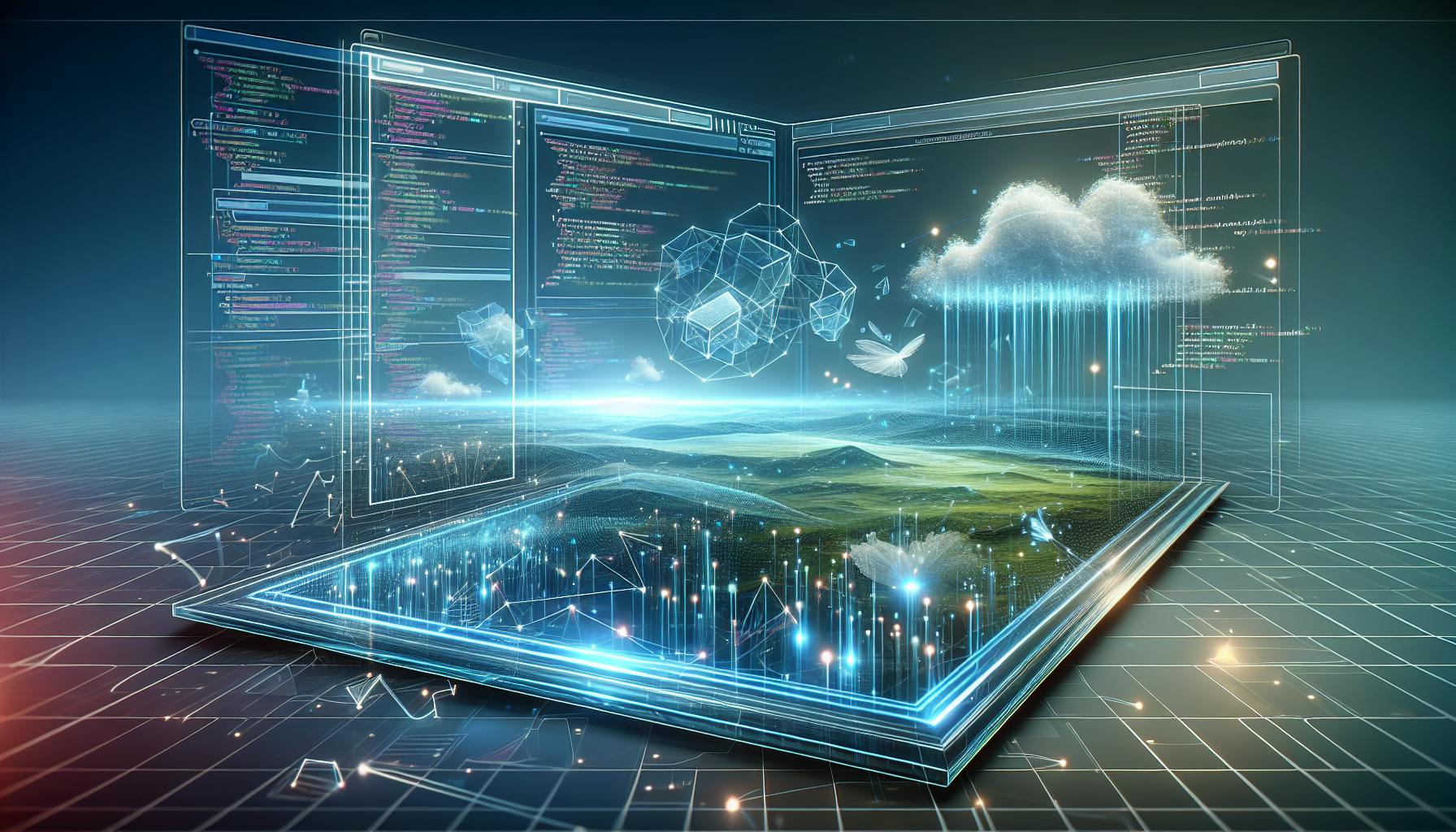
Learn the basics of React Hook Form for efficient and simplified form handling in React apps. Explore prerequisites, benefits, setting up forms, validations, handling different inputs, advanced form handling, and integration with UI libraries.
React Hook Form simplifies form handling in React apps, making it efficient and less complex. It leverages React Hooks for state management, validation, and form submission, offering key benefits like minimal re-renders, smooth performance, and easy integration with UI libraries. Here's what you need to know to get started:
- Prerequisites: Familiarity with React basics and having React v16.8 or newer.
- Benefits: Efficient updates, lightweight (around 2kB), and straightforward usage.
- Getting Started: Install with
npm install react-hook-form
and useuseForm
to access core functions. - Creating Forms: Leverage
register
andhandleSubmit
for easy setup and submission. - Validation: Easily validate inputs using rules for each field.
- Handling Different Inputs: Manage checkboxes, radio buttons, and custom select components effortlessly.
- Advanced Handling: Learn about resetting forms post-submission and simplifying validation error messages.
- Integration: Use with UI libraries like Material UI or Chakra UI using the
Controller
component.
React Hook Form stands out for its simplicity, performance, and flexibility, making it a go-to choice for React developers.
Prerequisites
Before diving into React Hook Form, you should know:
- The basics of how React works, including components, state, and props
- Have React v16.8 or newer (that's when Hooks were added)
- Your computer set up for React development, with Node.js and something to bundle your code, like Parcel or Webpack
Why Use React Hook Form?
Here's why React Hook Form is a good choice:
- Less refreshing - It only updates the parts that need to be updated, not everything every time you type something
- Runs smoother - It uses refs instead of state, which means it doesn't slow down your app
- Takes up less space - The whole thing is really small, only about 2kB when compressed
- Simple to use - It has easy-to-understand functions like register, handleSubmit, errors
- You write less code - It cuts down on extra stuff like context or reducers
- Quick to start - It's made to load fast when you first run your app
React Hook Form stands out because it makes dealing with forms simpler and more efficient right from the start, unlike other options like Formik or React Final Form.
Setting Up Your First Form
Installing react-hook-form
To start using React Hook Form in your project, you need Node.js on your computer. Open your terminal, go to your project's folder by typing cd
followed by the folder's name.
Then type:
npm install react-hook-form
This command adds React Hook Form to your project and lists it as a dependency in your package.json file.
Next, you need to include useForm in your file where you're making the form:
import { useForm } from "react-hook-form";
And that's it! You're now set to use React Hook Form.
Creating a Basic Form
Let's make a simple login form with an email and password field plus a submit button using React Hook Form and the useState hook.
Start by setting up useForm and useState:
import { useForm } from "react-hook-form";
import { useState } from "react";
function Login() {
const { register, handleSubmit } = useForm();
const [submittedData, setSubmittedData] = useState();
}
- useForm provides you with register and handleSubmit functions
- useState helps keep track of the form's submitted data
Now, let's add the email and password fields:
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input {...register("email")} placeholder="Email" />
<input {...register("password")} placeholder="Password" />
<button>Login</button>
</form>
)
- Using register connects your inputs to React Hook Form
- Placeholders help users understand what to type in each field
Lastly, let's handle what happens when the form is submitted:
function onSubmit(data) {
setSubmittedData(data);
}
This action saves the submitted information.
And there you have it! React Hook Form takes care of the form's state for you, allowing you to focus on other parts of your app.
Adding Validations to Your Form
Adding Simple Validations
Making sure your form is filled out correctly is key. React Hook Form helps you do this by letting you set rules for each part of your form.
Here's how to make sure the email and password someone enters in our login form follow certain rules.
First, we need to bring in some tools from react-hook-form:
import { useForm } from "react-hook-form";
import { required, minLength } from "react-hook-form/dist/validators";
Next, we tell our form what rules to check for:
<input
{...register("email", {
required: true,
pattern: {
value: /^[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,}$/i,
message: "Invalid email address"
}
})}
/>
<input
{...register("password", {
required: true,
minLength: {
value: 8,
message: "Password must be at least 8 characters"
}
})}
/>
- Now, the email must be a real email address and the password can't be too short.
React Hook Form will check these things when the form is sent. If something's wrong, it won't let the form go through.
To show messages when there's an error, we get the errors from useForm:
const {
register,
handleSubmit,
formState: { errors }
} = useForm();
And we add a little bit of code to show the error messages:
{errors.email && <p>{errors.email.message}</p>}
{errors.password && <p>{errors.password.message}</p>}
Now, if there's a mistake, your form will tell the user what they need to fix.
This way, you can make sure all kinds of inputs, like text, email, and password, are filled out right. And if you have more complex needs, React Hook Form has even more tools and options to help you manage your form's rules.
Working with Different Input Types
React Hook Form helps you easily manage different kinds of inputs in your forms, including checkboxes, radio buttons, and even custom select components from third-party libraries.
Checkboxes
For a checkbox, just register it like you do with other inputs. If you want it to be checked from the start, you can set its defaultValue
to true
:
<input
type="checkbox"
{...register("terms")}
/>
If you have a group of checkboxes and want to gather all the checked ones into a list, use the same name
attribute for them:
<input
type="checkbox"
value="html"
{...register("skills")}
/>
<input
type="checkbox"
value="css"
{...register("skills")}
/>
This way, if someone checks both, you'll get a list like ["html", "css"]
.
Radio Buttons
Radio buttons are for picking just one option. Register each button, and if you want one to be selected by default, use defaultValue
:
<input
type="radio"
value="html"
{...register("experience")}
/>
<input
type="radio"
value="css"
{...register("experience")}
/>
Custom Select Components
If you're using a custom select component, like something from a third-party library, you can still make it work with React Hook Form. Here's how:
- Register the component just like a regular input.
- Instead of using
register
, use the component's ownvalue
prop. - Make sure to update the value when it changes.
Here's a simple example with a custom select component:
// 1. Register the select component
const { register } = useForm()
// 2. Use the component's own value prop
<Select
value={selected}
onChange={setSelected}
/>
// 3. Update the value when it changes
function setSelected(value) {
setSelected(value)
}
This way, you can use all sorts of inputs in your forms, making them easy for people to fill out. React Hook Form handles the tricky parts, so you can focus on building a great form experience.
sbb-itb-bfaad5b
Advanced Form Handling
Resetting Form Values
After you submit a form, you might want to clear all the fields so it's ready for the next person. React Hook Form has a reset()
function that makes this super easy.
Just use reset()
when you're done submitting the form:
function onSubmit(data) {
// Here's where you handle submitting the form
reset();
}
This clears all your form fields back to how they started.
If you want to set the fields to specific values instead, you can do that by giving reset
some info:
reset({
name: "",
email: "",
terms: false
});
This way, your form is clean and ready for the next use.
Simplifying Validation Error Messages
When you're showing error messages from form validations, you can make it simpler. Instead of making a separate step, just include the error message right when you're setting up register()
:
register("email", {
required: "Email is required"
})
And in your form, show it like this:
{errors.email && <p>{errors.email.message}</p>}
This makes showing error messages a lot less work.
Integrating with UI Libraries
If you're using React Hook Form with UI components from libraries like Material UI or Chakra UI, it's pretty straightforward.
The trick is to use React Hook Form's Controller
component. It wraps around any UI component that uses a value
prop, making it compatible.
Here's how you might do it with Material UI's TextField
:
<Controller
name="email"
control={control}
defaultValue=""
render={({ field }) => (
<TextField
{...field}
/>
)}
/>
Controller
takes care of managing the value, checking for errors, and handling validation. All your component needs to do is accept the field
prop.
This way, you can use all kinds of UI components in your forms, making them not only functional but also nice to look at.
Conclusion
React Hook Form is a tool for managing forms in React that helps keep things simple, even when the forms get complicated. It has a few standout features:
- Makes form logic simple: It takes care of the hard parts of managing form states and updates only what needs to be updated. This makes your app run smoother. Plus, it has tools to check if forms are filled out correctly.
- Fits with any design library: It can be used with popular design libraries like Material UI and Chakra UI through something called the
Controller
component. - You can make it your own: It gives you a lot of hooks, which are like tools, to customize your forms exactly how you want, from checking forms to handling what happens when a form is submitted.
- Support for TypeScript: This means it can help catch problems early if you're using TypeScript for your project.
- Doesn't slow down your app: It's really small, only about 2kB, so it won't make your app heavy.
- A lot of people use it: It's a popular choice among React developers and gets regular updates from the community.
As you start to work with more complex forms in React, diving into React Hook Form's more advanced features can make your life easier. This includes managing tricky validations, adding or removing fields dynamically, and handling form submissions.
Check out the API reference for a detailed look at all the tools you have at your disposal. Also, the examples gallery has lots of examples of how to use it with different libraries.
Happy form building!
Related Questions
How do you use a form hook in React?
To start using form hooks in React, first bring in useForm
from react-hook-form
. Then, use it in your component to get tools like register
for connecting inputs and handleSubmit
for managing form submissions. Here's a quick example:
import { useForm } from "react-hook-form"
function App() {
const { register, handleSubmit } = useForm()
const onSubmit = (data) => {
console.log(data)
}
return (
<form onSubmit={handleSubmit(onSubmit)}>
{/* place for your form inputs */}
</form>
)
}
Here, register
links up your form inputs, and handleSubmit
looks after sending off the form.
What is React with hooks for beginners?
React hooks let you use state and other React stuff in function components. Here are some basics:
- Only use hooks like
useState
anduseEffect
at the top of your component - Hooks help you reuse logic across components
- Only call hooks in React function components, not regular functions
- They make handling state and lifecycle features easier in function components
For newbies, hooks make working with React a bit simpler compared to using class components.
What is register in React hook form?
In React Hook Form, register()
is a way to connect your input fields like <input>
and <select>
to the form, and set up rules for checking them. It gives back a bunch of helpers to attach to your inputs. Here's a peek:
const { register } = useForm()
<input {...register("username", {required: true})} />
This code links the input for "username" to the form and says it must be filled out.
How do you create a basic form in React?
Here's a simple form in React:
function App() {
return (
<form>
<label>
Username:
<input type="text" name="username" />
</label>
<label>
Password:
<input type="password" name="password" />
</label>
<input type="submit" value="Submit" />
</form>
)
}
This form has inputs for a username and password, and a button to send the form. To actually submit the form data, you need to add a function to handle the submission.