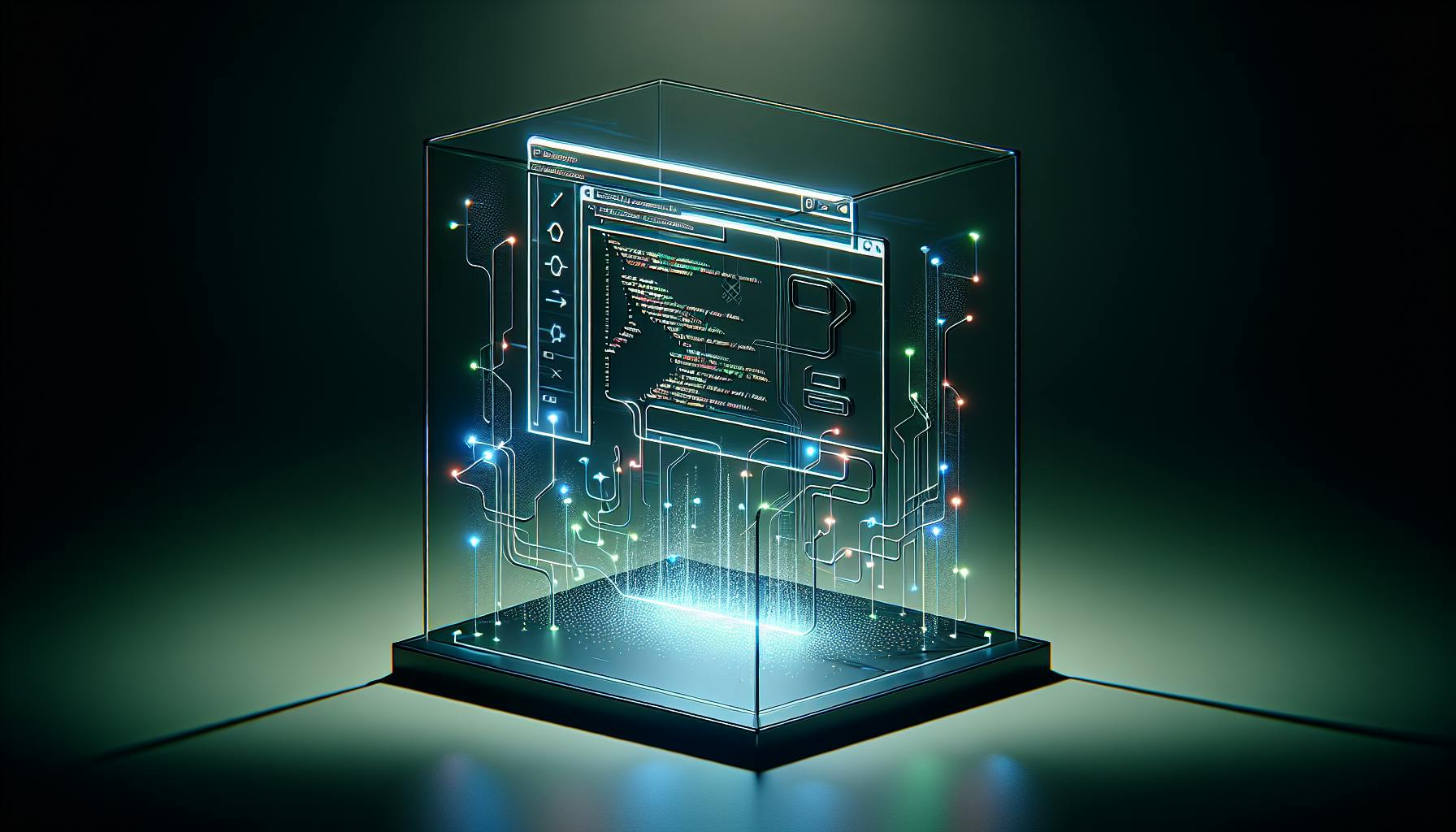
Learn how to troubleshoot React Hook Form errors and ensure robust and user-friendly form validation. Discover common issues, quick fixes, and advanced debugging techniques.
Developers often struggle with getting validation errors to properly display in React forms built with React Hook Form.
This post shares pro tips for troubleshooting missing or non-functioning errors to ensure robust and user-friendly form validation in React.
You'll discover common issues causing validation to fail, quick fixes to get errors displaying, and advanced techniques to deeply debug React Hook Form errors.
Introduction to React Hook Form Error Troubleshooting
This article provides troubleshooting tips for dealing with React Hook Form validation errors not displaying properly. We'll explore common issues that prevent errors from rendering and simple solutions to ensure robust, user-friendly form validation.
Understanding React Hook Form Validation
react-hook-form handles form validation by allowing you to define a validation schema and attach it to inputs via the Controller component. The useForm hook manages form state and surfaces any validation errors.
Some key points:
- Validation rules are defined using validator functions like required() or match()
- The Controller wraps inputs and connects them to form state
- Errors are passed back to inputs via the render prop
- Forgotten name attributes or not rendering errors are common mistakes
Overall, react-hook-form makes validating forms simple once set up correctly.
Common Causes of Missing Validation Errors
Some common reasons you may not see validation errors:
- Forgetting to pass errors to inputs via Controller render prop
- Name attribute missing/incorrect on wrapped input elements
- Async validation not displaying due to race condition
- Not triggering validation onSubmit for onBlur/onChange errors
These are easy mistakes but important to understand to diagnose issues.
Diagnosing React Hook Form Validation Not Triggering
If validation isn't activating properly:
- Check name attributes match schema definition
- Ensure you call trigger() if validating on blur/change
- Handle race conditions with async validation correctly
- Pass errors to inputs to display to user
Following react-hook-form patterns and the above tips will resolve most missing error cases.
Quick Fixes for Displaying Errors in React Forms
Outlines actionable solutions for getting errors to correctly appear, including checking render methods, re-binding inputs, and simplifying schema.
Ensure Errors are Passed to Inputs
A common reason React Hook Form validation errors fail to display is that the errors are not being passed to the input components correctly. Here are some tips:
- Make sure your
<input>
elements have anerror
prop defined that renders the error message when present. For example:
<input
{...register("email")}
error={errors.email?.message}
/>
-
Double check that the input
name
attributes match the key names returned in theerrors
object. Mismatched names will prevent errors from rendering. -
If using React Hook Form's
Controller
component, ensure you are spreading thefield
prop into the input to bind them together.
Properly associating inputs with matching errors ensures the end user sees validation messages when expected.
Check Mode and Trigger Settings in React-Hook-Form
React Hook Form's mode
and trigger
options can impact when validation occurs and errors show.
Some things to check:
-
If
mode
is set to"onSubmit"
, errors will only display after a form submit event. For real-time validation, use"onChange"
mode instead. -
The
trigger
setting determines what actions trigger validation. For example,"onBlur"
will only validate after an input loses focus. Compare different trigger options like"onChange"
to meet requirements. -
Use the
reValidateMode
option to control if validation should re-run on every change after initial failure. Can help ensure errors stay visible.
Adjusting these settings helps dial in desired validation behavior in React Hook Form.
Debugging react-hook-form Errors Empty Issue
Sometimes React Hook Form's errors
object returns empty despite inputs failing validation. Things to check:
-
Ensure the validation schema passed to
useForm
actually covers the inputs that should be validated. Any inputs lacking schema will not produce errors. -
Check that appropriate validation rules are defined in the schema for each input. For example,
required
for required fields. -
Log or debug the
errors
object after an invalid submit to inspect what is returned. The shape should match the schema. -
Try simplifying the schema first to eliminate any complex conditional logic that may be preventing errors.
An empty errors
object points to an issue with the validation schema configuration itself. Stripping down the rules can reveal where it falls short.
Implementing React Hook Form Scroll to Error
Having React Hook Form automatically scroll the page to the first invalid input makes for a better user experience.
-
Use the
formState
property and checkformState.isValid === false
to know validation failed. -
Access the first input ref from
formState.errors
when invalid. -
Use the DOM
scrollIntoView()
API on that input ref to scroll to it. -
Consider wrapping in
useEffect
to trigger smoothly after validation runs.
Scrolling to the first invalid input guides the user to fix errors faster in React Hook Form.
sbb-itb-bfaad5b
Advanced Debugging for React Hook Form Validation Bugs
For tricky cases, explores developer tools techniques and React Hook Form APIs to pinpoint where in the lifecycle errors fail to propagate.
Use React DevTools to Inspect Values
React DevTools allows inspecting component state and props to check if errors are present. Some tips:
- Check if
errors
are set in state after a form submit. If not, validation is not running. - Inspect props passed to inputs. Should see
error
prop populated. - Toggle DevTools after submit to see if errors briefly appear then disappear.
Adding console.log
statements in callbacks like onSubmit
also helps trace execution flow and values.
Log and Step Through Validation with React-Hook-Form Trigger
The trigger
API method manually runs validation. Adding logs here isolates issues:
const {trigger} = useForm();
const onSubmit = async (data) => {
console.log('Triggering validation');
const valid = await trigger();
console.log(valid ? 'Valid' : 'Invalid');
}
Stepping through this code in DevTools shows where in the lifecycle validation gets stuck.
Inspecting React Hook Form Controller for Error Handling
The Controller
component wraps inputs and handles errors under the hood. Inspect its render prop:
<Controller
render={({field, fieldState}) => {
console.log(fieldState.error); // Check for error
return <Input {...field} />
}}
/>
If errors aren't passed here, they won't reach inputs. May indicate an issue with Controller
usage.
Creating User-Friendly Error Messages with React Hook Form
React Hook Form provides basic validation error messaging out of the box. However, thoughtfully crafted error messages can greatly improve overall user experience. Here are some tips for making errors more meaningful and actionable for users.
Write Clear, Concise Error Text
- Keep messages short, simple, and easy to understand
- Avoid vague or technical jargon
- Specify exactly what needs to be fixed
- For example, "Please enter your email" instead of "Invalid input"
Strategically Place Error Display for User-Friendly Interaction
- Display errors inline next to the relevant form field
- Makes it easy for users to identify issues
- Reduces frustration from hunting around to see errors
Leveraging JavaScript and ReactJS for Dynamic Error Messaging
- Conditionally show different errors based on input validation
- For example, display "Invalid email format" only if email is incorrectly formatted
- Create custom hook to handle all validation and error display
- Keeps components clean and logic reusable
Taking a user-centered approach with thoughtful error handling ensures a smooth, frustration-free experience for visitors interacting with your forms. The effort pays dividends in higher conversion rates from improved usability.
Conclusion: Ensuring Robust Form Validation in React
In summary, common React Hook Form validation bugs can be addressed through steps like ensuring proper input binding, checking trigger configuration, logging execution flow, and crafting user-friendly errors to support data accuracy.
Key Takeaways from Troubleshooting React Hook Form Errors
- Double check all input names match the Controller names to ensure proper binding
- Validate trigger timing and configuration to match form requirements
- Log validation execution flow to debug issues
- Craft clear, actionable error messages to guide the user
- Set mode to "onChange" for real-time validation
- Leverage validation schema for complex logic
- Display errors visibly on submit for easy identification
Following these best practices will result in robust and user-friendly form validation in React.
Final Thoughts on Building Robust and User-Friendly Forms
Reliable form validation and error handling is critical for a smooth user experience. Taking the time to craft meaningful errors and debug issues sets applications up for success by ensuring accurate data capture. While debugging validation can be tricky, arming oneself with logging, visibility into form state, and an understanding of timing and triggers can unravel even the most complex issues. By putting users first and guiding them to correct submissions, applications build trust and encourage engagement.