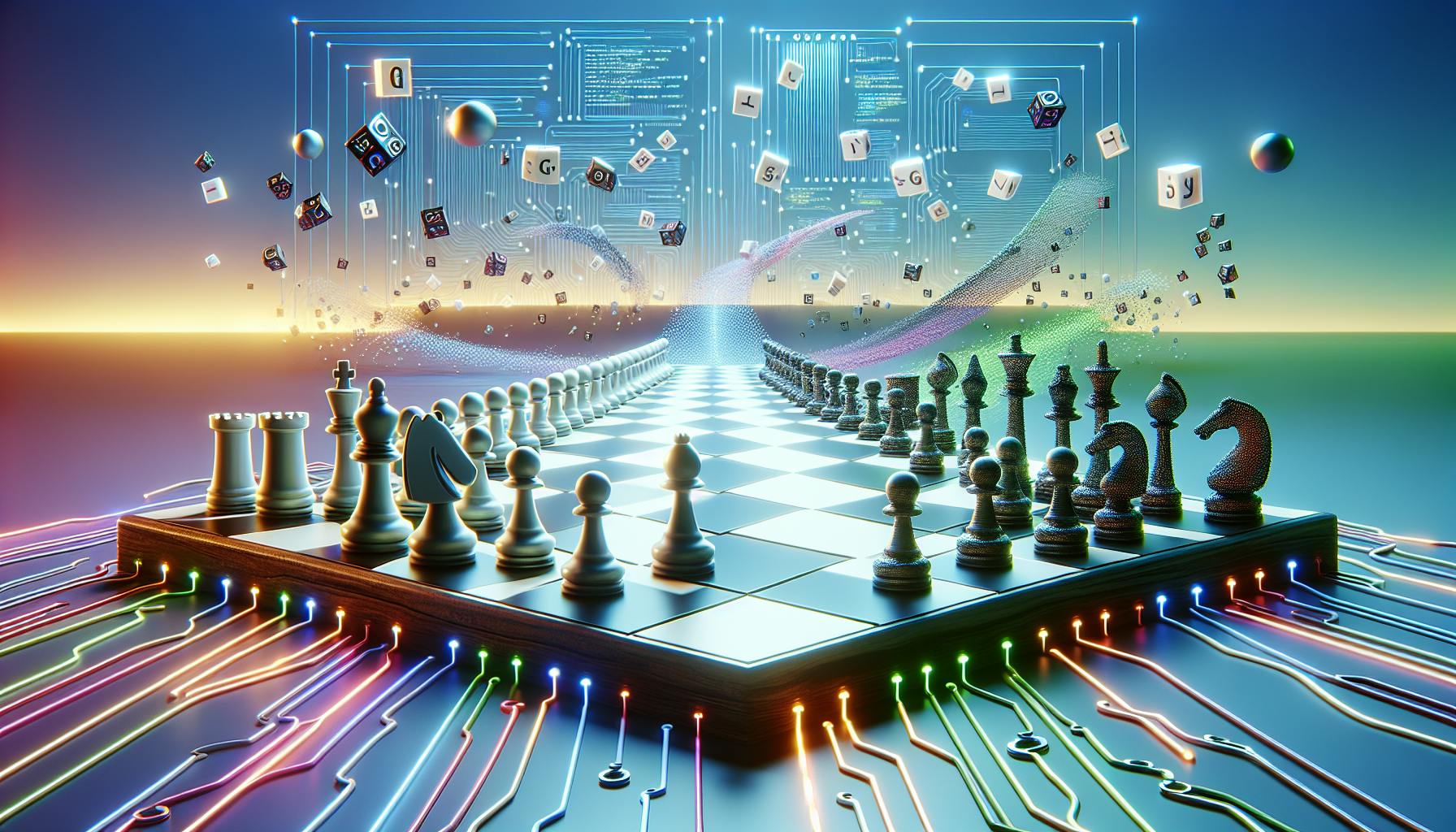
Explore key syntax differences between Go and JavaScript and learn how to adapt coding practices for a seamless transition. Understand control flow, data structures, and more.
Developers looking to transition from Go to JavaScript will likely agree that understanding key syntax differences is critical for effective code adaptation.
By analyzing structural and functional syntax variances between the two languages, developers can establish coding practices to bridge knowledge gaps during the transition process.
This guide examines Go and JavaScript syntax fundamentals, control flows, data structures, and more to illuminate transition challenges and solutions across projects.
Introduction to Syntax Differences in Go and JavaScript
Go and JavaScript are both popular programming languages used for web and application development. As developers gain experience with one language, some may consider expanding their skills by learning the other. However, the syntax between Go and JavaScript differs in key ways that can trip up coders during the transition.
Getting familiar with these core syntactical differences allows developers to adapt their coding practices more smoothly when moving between the languages. Understanding concepts like labels, control flow, and parameter default values in each language establishes stronger coding fundamentals. Developers can then write platform-specific code more intuitively in Go or JavaScript contexts.
The Rise of Golang to JavaScript Transitions
Go usage has grown rapidly since its launch in 2009. Its performance benefits, concurrency support, and simplicity have made it a preferred backend language. Meanwhile, JavaScript remains the most popular language overall thanks to its universal browser compatibility.
As more developers gain Go experience, interest has emerged in also using JavaScript for broader front-end development. Learning the key differences in syntax helps facilitate this transition.
For example, Go relies heavily on labels and goto
statements for control flow, an approach less common in JavaScript. Knowing how each language handles program flow reduces confusion when switching contexts.
Overall, understanding distinctions in coding style helps developers avoid frustrations when expanding their capabilities across languages.
Understanding JavaScript goto Label and Control Flow
Go allows liberal use of labels and goto
statements to control program flow. However, JavaScript does not have an equivalent goto
command and handles control flow differently.
Instead of goto
, JavaScript uses structures like if/else
, switch
, for
and while
loops, and try/catch
blocks to direct code execution. Functions also enable some control flow by accepting callback parameters.
Developers coming from Go may be inclined to use labels and goto
, especially for error handling. But in JavaScript, alternate approaches like try/catch
or callback functions achieve similar control flows.
Overall, Go's specific goto
method requires adaptation to JavaScript's alternate structures for controlling program flow. Understanding these core differences helps ease developer transitions.
Adapting Coding Practices for Syntax Differences
Beyond control flow, other syntax variations in areas like default parameters, asynchronous handling, and variable declarations can impact developer transitions.
For example, Go does not allow default parameter values, unlike JavaScript. Go also lacks native asynchronous support, managed differently than JavaScript's async/await.
Developers must remember to declare variables explicitly in Go, whereas JavaScript has loose implicit typing. And Go's strict compile-time checks differ from JavaScript's primarily runtime evaluations.
While adapting coding habits to account for these syntax differences takes concerted effort, over time developers can intuitively toggle contexts. Referencing compatibility documentation helps smooth the process of bridging knowledge between Go and JavaScript.
Understanding core language differences allows developers to adapt more easily when expanding skills across Go and JavaScript. With practice, developers can translate their coding instincts to match the syntax of each language.
Is there a goto in JavaScript?
JavaScript does not have a goto statement like some other programming languages. However, you can achieve similar behavior using labels with break and continue statements.
Here is an example of using a label with a loop in JavaScript:
outerLoop:
for (let i = 0; i < 10; i++) {
for (let j = 0; j < 10; j++) {
if (i === 5 && j === 5) {
break outerLoop;
}
}
}
In this example, when the inner loop reaches i=5 and j=5, it will break out of the outer loop completely. This allows you to break out from nested loops similar to a goto statement.
The key things to know about labels in JavaScript:
- Labels can be any valid JavaScript identifier followed by a colon
- Labels only apply to break and continue statements
- The labeled statement must contain the break/continue referencing it
So while there is no exact equivalent, labels allow some goto-like behavior in JavaScript loops. But it's considered better practice to structure your code to avoid the need for goto statements.
What is the alternative to goto in JavaScript?
Alternatives to goto statements in JavaScript provide more readable and maintainable code. Popular options include:
Break and Continue
These statements allow exiting or skipping iterations of loops without goto.
break
immediately exits the current loopcontinue
skips the rest of the current iteration
This improves readability by avoiding jumps.
Nested Conditionals
Complex conditional logic can be nested instead of using gotos:
if (condition1) {
if (condition2) {
// logic
}
}
Functions
Functions modularize code into logical blocks. Calling functions replaces gotos.
Promises and Async/Await
Managing async logic without gotos:
async function process() {
try {
const result = await doSomething()
return result
} catch(err) {
handleError(err)
}
}
Overall, JavaScript provides many goto alternatives for cleaner and more maintainable code. Carefully structuring logic avoids the need for labels and jumps.
Does go compile to JavaScript?
Go is a compiled language, meaning the Go compiler converts the human-readable source code into machine code that can be directly executed by the computer's processor.
However, there are some variant implementations of Go that can compile to other targets like JavaScript or WebAssembly instead of native machine code:
GopherJS
GopherJS compiles Go code to JavaScript code so it can run in the browser or on Node.js. This allows you to write front-end code in Go that interacts with the DOM and other JavaScript APIs. Some key things to know about GopherJS:
- It provides bindings to many JavaScript APIs and libraries like jQuery, AngularJS etc.
- The generated JavaScript code is readable but also heavily optimized.
- It supports gradual code migration from other languages to Go.
- GopherJS translates Goroutines to JavaScript promises and supports async/await.
So while standard Go compiles to native machine code, GopherJS opens up the possibility of compiling Go to JavaScript for front-end web development.
Other Implementations
There are other implementations like Golang to WebAssembly (WASM) compilers that can compile Go code to WebAssembly instead of machine code.
And experimental options like Godo that can compile a Go package to a Node.js module.
So in summary - pure Go compiles to native machine code, but variant implementations allow transpiling Go to other targets like JavaScript or WASM.
How to jump to a line in JavaScript?
JavaScript does not have a direct equivalent to Go's goto
statement to jump to a specific line number. However, there are a few ways to achieve similar behavior in JavaScript:
Using Labels and Break Statements
You can label loops in JavaScript and then use the break
statement to jump out of the loop. For example:
outerLoop:
for (let i = 0; i < 5; i++) {
for (let j = 0; j < 5; j++) {
if (i === 3) {
break outerLoop;
}
console.log(i, j);
}
}
This will stop executing the outer loop when i
reaches 3.
Using Functions
You can wrap sections of code in functions and then call those functions whenever needed to execute that code. For example:
function printMessage() {
console.log('Jumped to this function');
}
// Lots of code
printMessage(); // Jumps to function
This allows you to reuse and call code from anywhere.
Using Asynchronous Callbacks
With asynchronous JavaScript, you can specify callback functions that execute after asynchronous events complete. This allows "jumping" to callback functions based on external events.
In summary, while not a direct equivalent, JavaScript does provide ways to conditionally redirect code execution flow. Understanding these differences from Go can help adapt coding patterns effectively.
sbb-itb-bfaad5b
Fundamentals of Go and JavaScript Syntax
Go and JavaScript differ significantly in their overall design paradigms and syntax, which developers must understand when transitioning between the two languages.
From GopherJS to Native JavaScript Functions
GopherJS transpiles Go code to JavaScript, enabling Go packages to be used on the web. However, when calling native JavaScript functions from Go, developers may encounter issues around asynchronous code and binding. For example, JavaScript's fetch
API returns a Promise whereas Go expects synchronous code. Developers should reference GopherJS compatibility documentation to ensure correct interoperability.
When needing tighter control over generated JavaScript, developers can use bindings to JavaScript APIs and libraries like jQuery. However, this requires writing platform-specific code which reduces code reuse across platforms.
The Role of Default Parameter Values in JavaScript
Unlike Go, JavaScript supports default parameter values for functions. This provides more flexibility, allowing developers to omit arguments when calling functions. However, it can also introduce bugs when adding new parameters to existing functions. Go's strict function parameters avoid such issues.
When porting Go functions to JavaScript, developers should carefully consider if default parameters help or hinder their API design rather than blindly mirroring Go's parameter handling.
Labelled While Loop: A Substitute for Goto
Go supports goto
statements for control flow between labels. JavaScript lacks goto, but labelled while loops can be used instead for similar control flow. However, goto is considered harmful by many due to code complexity and lack of structure.
Developers should aim to write structured code avoiding goto usage even when programming in Go. Rethinking logic flow around function calls, returns, and loops leads to more readable code. Labelled while loops in JavaScript should be used sparingly in special cases, not as a goto replacement.
Structural Syntax Differences Between Go and JavaScript
Comparing Variable Declarations and Scope
Go uses statically typed variable declarations with explicit types like var name string
, whereas JavaScript employs dynamic typing with declarations like var name = 'value'
. Go variables also have block scope limited to the enclosing curly braces {}
, but JavaScript scopes variables to the enclosing function or global scope.
This means JavaScript developers must adjust to Go's stricter and more limited variable scoping rules. Declaring variables with explicit static types in Go versus implicit dynamic types in JavaScript also requires adapting coding habits.
Data Structures and Typing Systems
Go provides built-in data structures like arrays, slices, maps, and structs to organize data, while JavaScript relies more on objects and arrays. Go also enforces strict static typing for safety and performance, whereas JavaScript uses loose dynamic typing for flexibility.
For example, Go arrays have fixed lengths, but JavaScript arrays dynamically resize. Go maps behave like JavaScript objects in some ways, but do not inherit from other maps. These differences mean JavaScript developers must understand the right Go idioms for working with built-in data structures efficiently.
Control Structures and Asynchronous Patterns
Go uses for
loops, if/else
conditionals, switch
statements much like other languages. But JavaScript employs asynchronous constructs like promises and callbacks more often for non-blocking I/O.
So JavaScript developers should understand Go's synchronous control flows before attempting similar asynchronous patterns. Go's goroutines
and channels can accomplish async behavior, but differ syntactically from JavaScript's promise chains and event callbacks.
Interoperability: Binding Go with JavaScript APIs and Libraries
Go and JavaScript have some key differences in their syntax and code structure that developers should understand when building applications using both languages. This section explores strategies for interoperability through DOM bindings, integrating popular JavaScript libraries, and writing platform-specific code.
Integrating Go with DOM Bindings
The Document Object Model (DOM) enables programs to dynamically access and update a website's content, structure, and styles. While Go does not have built-in DOM manipulation capabilities, developers can leverage JavaScript DOM bindings to enable Go code to interact with the DOM. Some key approaches include:
- GopherJS - Compiles Go code to JavaScript so it can run in browsers and manipulate the DOM directly. This allows you to write entire front-end apps in Go.
- Bindings - Go offers DOM bindings to directly call JavaScript functions that interact with the DOM from Go code. Popular bindings include godom, go-js-dom, and go-dom.
For example, to add a <div>
using go-dom
:
div := dom.GetWindow().Document().CreateElement("div")
dom.GetWindow().Document().GetElementsByTagName("body")[0].AppendChild(div)
These bindings enable seamless interoperability between Go code and the DOM.
Leveraging JavaScript Libraries: jQuery and AngularJS Bindings
To leverage the power of popular JavaScript libraries like jQuery and AngularJS in Go code, developers can use binding packages:
- jQuery - GoJQ provides Go bindings for jQuery, enabling DOM manipulation, AJAX, events, etc.
- AngularJS - go-angular contains AngularJS bindings for Go, allowing integration of functionality like dependency injection, two-way data binding, and more.
For example, to use jQuery's fadeOut method in Go:
import . "github.com/google/gojq"
func main() {
FadeOut("#element", 300)
}
These bindings prevent developers from having to context switch between languages.
Writing Platform-Specific Code in Go and JavaScript
When building apps for multiple platforms like web, mobile, and desktop, developers can leverage Go and JavaScript's strengths:
- Use Go for performance-critical backend/networking logic.
- Use JavaScript for UI/frontend logic where DOM manipulation is needed.
Follow these guidelines when writing platform-specific code:
- Abstract logic into reusable modules/functions that can be shared between platforms.
- Use Go to handle intensive tasks like number-crunching, file I/O, etc.
- Use JavaScript for visualizations, animations, and interactive UIs.
This separation optimizes apps by having each language handle what it does best. Shared modules enable code reuse while allowing tailored implementations.
Development Tools and Compatibility Documentation
Navigating Compatibility Documentation for GopherJS
When using GopherJS to compile Go code for JavaScript environments, developers should carefully consult the compatibility documentation to avoid issues. The GopherJS documentation outlines supported Go language features, standard library packages, and provides a full compatibility table. This assists developers in determining if a particular Go package or function has JavaScript bindings available. If compatibility is limited, contributors can help improve GopherJS by adding support. Overall, consulting compatibility documentation early when evaluating GopherJS will simplify development.
Developer Guidelines for Cross-Platform Tools
Several key developer guidelines enable smoother cross-platform development between Go and JavaScript:
- Abstract platform-specific code into separable modules to isolate compatibility issues
- Use interface definitions to enable swapping implementations across platforms
- Leverage tools like GopherJS and Node-Go to ease interoperability
- Favor language features supported across both platforms
- Validate all inputs/outputs across language boundaries
- Provide comprehensive testing for cross-platform modules
Following these best practices will streamline building apps using both Go and JavaScript.
Automating Bindings to JavaScript APIs and Libraries
Generating bindings from JavaScript to Go can be automated using tools like GopherJS DOM, Go jQuery, and GopherJS React. These packages use code generation to wrap the JavaScript APIs in a Go interface. This allows easily calling the JS API from Go code. For other JS libraries, GopherJS Binding can automate bindings using Node.js. The tool parses JS files to produce a Go file defining wrappers. Using these automated binding generators simplifies accessing JavaScript from Go code.
Establishing Effective Coding Practices Across Languages
Providing guidelines to streamline transitioning between languages can help developers adopt consistent and effective coding practices.
Adopting Linters and Formatters for Consistent Syntax
Using tools like gofmt
and Prettier to auto-enforce coding styles can assist with maintaining consistent syntax across Go and JavaScript projects.
Here are some recommendations:
- Set up
gofmt
to run on save in Go projects to adhere to the standard Go formatting style guide. This avoids messy diffs due to inconsistent spacing or formatting. - Use Prettier in JavaScript projects to automatically format code on save based on opinionated rules. Configure EditorConfig to share rules between team members.
- Consistent syntax enforced by linters and formatters helps developers context switch between Go and JavaScript more easily without having to manually reformat code.
- Automated formatting frees up developers to focus on programming rather than code style, improving productivity.
Project Layouts and Modularization Techniques
Having consistent project organization between Go and JavaScript code bases makes it easier to navigate projects in different languages.
Some proposed conventions:
- Structure code into modules/packages based on features rather than file types. Group together functionality that belongs together.
- Use a common src/ directory to store application code separate from tests and vendor dependencies.
- Standardize naming schemes for directories, files, functions, variables, etc across projects.
- Adhere to established language idioms and project layout guidelines like golang-standards/project-layout and Airbnb's JavaScript Style Guide.
Inline Documentation: From Go Docstrings to JSDoc
Inline documentation makes code more readable and maintainable over time. Compare approaches:
- Go: Use docstring comments prior to package, constant, variable, function declarations per the godoc convention.
- JavaScript: Adopt JSDoc syntax for function/class/method comments to describe parameters, return values, etc.
- Go docstrings focus on packages and declarations while JSDoc annotates specific program constructs.
- Consistent use of inline docs improves understanding across Go and JS code.
In summary, establishing cross-language conventions through automated formatting, project structure, and documentation helps ease context switching for developers working across Go and JavaScript. This improves efficiency, collaboration, and code quality over time.
Conclusion: Synthesizing Go and JavaScript Syntax Mastery
Recap essential learnings for adapting between the languages, emphasizing cross-polination of coding styles.
Summarizing Syntax Differences and Developer Takeaways
As we have seen, while Go and JavaScript share some syntactical similarities, they have key differences developers should be aware of.
The main takeaways include:
- Go uses statically typed variables while JavaScript employs dynamic typing. Understanding the implications of this on variable usage is important.
- Go allows named return values which can improve readability versus JavaScript's single anonymous return.
- The
goto
statement in Go provides greater control flow flexibility compared to JavaScript's label syntax. - JavaScript prototype-based inheritance differs from Go's interface implementation. Developers should adapt their OOP approach accordingly.
- Asynchronous programming models diverge between the callback and promise patterns of JavaScript versus the goroutines and channels of Go.
Being cognizant of these distinctions will help developers avoid common pitfalls when switching between the languages. Identifying areas of alignment also allows knowledge to transfer beneficially between coding contexts.
Final Recommendations for Seamless Language Transition
To enable fluid transitions between Go and JavaScript, developers should:
- Maintain awareness of typing, return values, and inheritance model differences
- Standardize control flow patterns where possible using conditionals and loops compatibly
- Absorb idiomatic practices unique to each language through continued usage
- Consider utilities like GopherJS for JavaScript compilation or DOM bindings for access to web APIs
- Consult compatibility documentation when integrating external libraries or frameworks
Internalizing these recommendations will lead to syntax mastery adaptable between projects regardless of language. Developers will write code benefiting from the strengths of both Go and JavaScript.