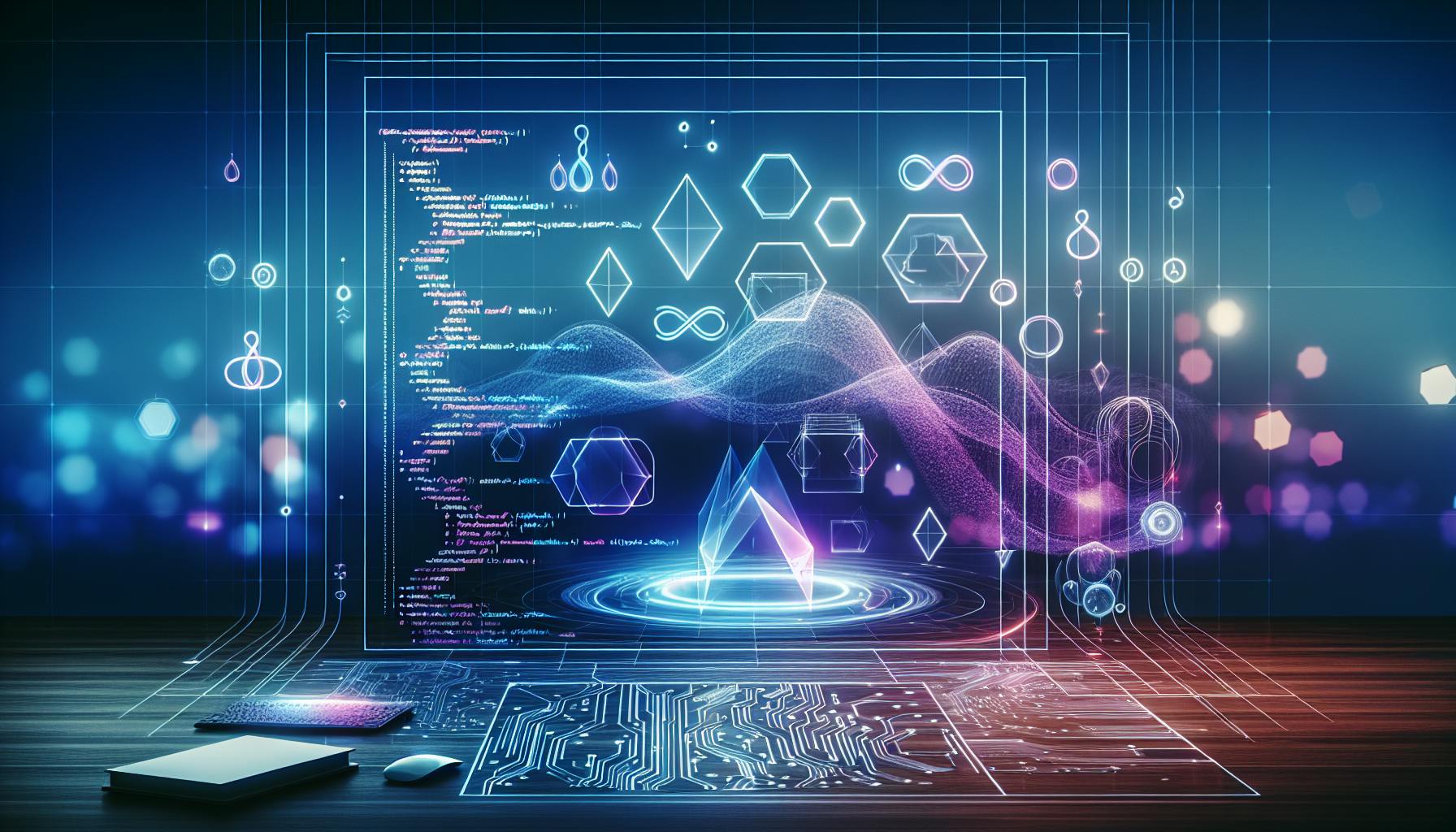
Learn how GraphQL generated types enhance developer productivity and code reliability. Discover tools like graphql-codegen, integration techniques, and real-world case studies.
Developers will likely agree that managing GraphQL types and schemas can be tedious and error-prone.
Luckily, tools like graphql-codegen
can automatically generate types and interfaces to integrate with your GraphQL server, enhancing productivity and reliability.
In this post, you'll learn how GraphQL code generation works, see examples of generated types in action, and find out how to configure and adopt these autogenerated types in both new and existing projects.
Introducing GraphQL Generated Types
GraphQL generated types refer to TypeScript types that are automatically generated based on a GraphQL schema. This provides type safety, preventing errors and improving developer productivity when building GraphQL APIs.
Understanding GraphQL Types and Type Generation
A GraphQL schema defines the types and relationships in an API. GraphQL generated types take this schema and generate corresponding TypeScript definitions. This means developers get type safety when writing queries, mutations, and resolvers. Without this, developers would need to manually define types, which is error-prone. Automated type generation enhances code quality and reliability.
The Role of graphql-codegen in Enhancing Code Quality
graphql-codegen is a popular tool for generating TypeScript types from GraphQL schemas. Key benefits include:
- Type safety - Avoid runtime type errors
- Documentation - Type definitions serve as API documentation
- Productivity - Less manual effort defining types
By scanning the schema and emitting TypeScript types, graphql-codegen enhances developer productivity and code reliability within GraphQL projects.
How @graphql-codegen/cli Simplifies Type Generation
The @graphql-codegen/cli
package provides a CLI for invoking GraphQL Code Generator programmatically. This simplifies schema introspection and generating types for different languages. Developers configure codegen by specifying:
- GraphQL schema source
- Output language/format
- Plugins for custom generation logic
The CLI then handles scanning the schema and emitting generated types.
Configuring graphql-codegen/typescript for Your Project
To generate TypeScript types, key configuration options are:
- schema: GraphQL schema SDL or endpoint
- generates: Select
typescript
output - plugins:
typescript
,typescript-operations
This will emit query/mutation types for type safety. Additional plugins enable mocking, integrating schemas, and other features.
Utilizing GraphQL Query Generator for Automatic Type Generation
A key capability provided by graphql-codegen is auto-generated types for GraphQL queries/mutations. The typescript-operations
plugin generates TypeScript types for these operations. This enables type safety when defining GraphQL requests in the app codebase. Instead of using plain strings, developers can strongly type queries/mutations for additional reliability.
What is generated in GraphQL?
GraphQL uses a type system to clearly define the available data for each type and field in a GraphQL schema. This allows tools like GraphQL Code Generator to analyze the schema and automatically generate TypeScript types and interfaces that correspond to the schema.
Some of the key things that can be generated include:
-
TypeScript types - For each GraphQL object type, input type, scalar, interface, union, and enum, Codegen can generate a corresponding TypeScript type. This saves developers time manually declaring types.
-
Resolver functions - The generator can scaffold out TypeScript resolver functions for each field in the schema. These handle querying data sources and returning the requested fields.
-
API client code - Fully functional API client code can be created in TypeScript or other languages, with typed query and mutation functions. This handles sending requests and normalizing responses.
-
Mock data - Sample mock data that matches the schema types helps in local development and testing scenarios. Mocks are automatically kept in sync as the schema evolves.
-
Documentation - Markdown documentation for the API can be generated from the schema, helping developers understand what data is available.
By leveraging schema introspection and code generation, tools like GraphQL Code Generator save huge amounts of repetitive typing while making it far easier to keep the API strongly typed as it grows. This enhances developer productivity and code reliability within professional developer networks relying on GraphQL.
How created GraphQL?
GraphQL schemas can be created in two main ways:
Code-First Approach
In the code-first approach, you define the GraphQL schemas programmatically using JavaScript objects from the graphql-js
library. The schema definition language (SDL) is then auto-generated from the source code.
Some benefits of the code-first approach:
- Tight integration with application code
- Easy to build schemas incrementally
- Changes immediately reflected in API
However, the code-first approach can become messy for larger, complex schemas.
Schema-First Approach
The schema-first approach involves manually writing the GraphQL schemas using the SDL before implementing corresponding resolvers in code.
Some benefits of the schema-first approach:
- Better separation of concerns
- Improved collaboration between frontend and backend developers
- Easier to visualize API structure early on
However, changes to the schema require updating both the SDL definition and corresponding resolvers.
Overall, both approaches have tradeoffs and can be used successfully. The choice depends on the application requirements, team structure, and existing codebase.
How do I auto generate schema in GraphQL?
GraphQL enables developers to auto generate schemas in two primary ways:
Using the StepZen CLI
The StepZen command-line interface (CLI) provides the stepzen import
command to autogenerate a GraphQL schema by specifying a backend data source. This allows creating a GraphQL API from an existing database or service.
The key benefits of using stepzen import
include:
-
Rapid API Development: Schemas can be generated automatically without needing to manually define types and resolvers. This accelerates development.
-
Integration Flexibility: StepZen supports connecting to data sources like PostgreSQL, MongoDB, REST APIs, gRPC services and more. This provides flexibility.
-
Built-in Features: Additional capabilities like authentication, caching, and rate limiting are handled out of the box.
-
Managed Infrastructure: StepZen handles hosting, scaling, and maintenance of the GraphQL infrastructure.
Here is an example command to autogenerate a schema from MongoDB:
stepzen import mongo 'mongodb+srv://user:password@cluster.mongodb.net/mydb'
Using GraphQL Schema Directives
GraphQL schemas can also be autogenerated using schema directives like @graphql-codegen/cli
. This generates code by analyzing the GraphQL schema and documents.
Key advantages include:
-
Increased Productivity: Types are generated automatically, reducing repetitive coding.
-
Improved Reliability: Auto-generated code reduces human error that could lead to bugs.
-
Enhanced DX: Integrates schema changes immediately with a unified dev experience.
-
Flexible Integrations: Custom plugins and templates enable integrating any data source.
Here is an example schema using @graphql-codegen/typescript
:
"""
@graphql-codegen/typescript generates Typescript types automatically
"""
type Author @graphql-codegen/typescript {
id: ID
name: String
books: [Book]
}
This leverages GraphQL schema directives to auto generate Typescript types from schema, boosting developer productivity.
In summary, StepZen CLI and GraphQL codegen provide complementary ways to auto generate GraphQL schemas, accelerating development.
sbb-itb-bfaad5b
Which tool generate GraphQL schema?
GraphQL Code Generator is a popular open-source tool for generating code out of a GraphQL schema and documents. It helps improve developer productivity and code reliability in various ways:
-
Auto-generates types based on your GraphQL schema, ensuring consistency between client and server. This saves significant time manually creating types.
-
Outputs code in various languages like TypeScript, Flow, etc. Reduces boilerplate code.
-
Creates resolver functions and router handlers from schema. Jumpstarts backend implementation.
-
Generates query hooks and React components from operations. Speeds up client development.
-
Mocks data based on schema types for testing. Enables early stage integration testing.
-
Plugins extend functionality for specific frameworks like Apollo, React, Angular, etc. Custom integrations made easy.
In summary, GraphQL Code Generator eliminates repetitive coding and keeps client and server in sync as schema evolves. This improves developer productivity and code quality within professional developer networks relying on GraphQL.
Integrating Generated Types into Codebase
Setting Up graphql-codegen/client in New Projects
Setting up GraphQL Code Generator in new projects is straightforward with graphql-codegen/cli
. After installing the CLI and desired plugins, add a codegen.yml
config file to specify code generation rules. For example:
schema: http://localhost:4000
generates:
generated/types.ts:
plugins:
- typescript
- typescript-operations
- typescript-react-apollo
This will generate TypeScript types and React Apollo hooks in generated/types.ts
based on your schema.
Next integrate these types when constructing your Apollo Client:
import { typeDefs } from './generated/types';
const client = new ApolloClient({
cache: new InMemoryCache(),
typeDefs,
});
Now components leveraging useQuery
and useMutation
will be correctly typed for queries and mutations.
Adopting Generated Types in Existing Professional Networks
For existing projects, incrementally adopt generated types starting with core domain models. Create a types.ts
file exporting key models:
export type User = {
id: string
name: string
}
export type Post = {
id: string
title: string
author: User
}
Use these types when fetching data:
function getPosts(): Promise<Post[]> {
// ...
}
Over time, expand typed models across the codebase until the GraphQL schema is fully typed. This gradual approach prevents breaking changes.
Importing Generated Types into Apollo GraphQL Components
Import generated types into React components to enable type checking:
import { GetPostsQuery } from 'generated/types';
function Posts() {
const { data } = useQuery<GetPostsQuery>();
return (
<div>
{data?.posts.map(post => (
<Post key={post.id} post={post} />
))}
</div>
);
}
Now data
and post
will be correctly typed based on the GraphQL query.
Enhancing Apollo Client with Generated GraphQL Schema Types
Besides components, generated types can enhance Apollo Client itself:
import { typeDefs } from './generated/types';
const client = new ApolloClient({
cache: new InMemoryCache(),
typeDefs,
});
client.query<GetPostsQuery>({ ... });
Here the client's query
method is typed to only allow valid queries. Similar for mutate()
with mutations.
Mocking GraphQL APIs with Generated Types
Generated types can be used to mock GraphQL APIs by creating schema and resolvers:
import { makeExecutableSchema } from 'graphql-tools';
import { typeDefs } from './generated/types';
const mocks = {
Query: () => ...,
Mutation: () => ...,
};
const schema = makeExecutableSchema({
typeDefs,
mocks,
});
Now schema
can be used to integration test without needing a real API.
Advanced GraphQL Code Generation Techniques
Covers more advanced generators and configurations for optimizing developer experience.
Generate GraphQL Schema for Apollo Federation
GraphQL Federation allows combining multiple GraphQL schemas into a single unified gateway API. This enables building a distributed architecture with separate services, while providing clients a seamless GraphQL developer experience.
However, working across federated schemas introduces complexity when generating types from schema. The @graphql-codegen/federation
plugin automates type generation across merged schemas to improve developer productivity.
Key benefits include:
- Generates types for all services to be consumed via the Apollo gateway
- Handles schema stitching behind the scenes
- Reduces manual type maintenance as the graph grows
- Enables building UI components without schema implementation detail
Overall, the federation codegen plugin reduces complexity working with distributed GraphQL architectures.
Automating Interfaces and Union Type Generation
GraphQL interfaces and unions enable polymorphic capabilities where a type can fulfill multiple potential shapes. However, developers lose some TypeScript type safety guarantees when working with interfaces and unions.
The @graphql-codegen/typescript-interfaces
plugin improves the developer ergonomics by auto-generating:
- Type guard functions to determine runtime types
- Concrete types for interfaces and unions
- Exact object types matching GraphQL resolve types
This automates complex manual type definitions that would otherwise be required. Some key benefits:
- Catch type issues during compilation that otherwise would only error at runtime
- Avoid extensive manual type manipulation when working with interfaces and unions
- Streamline building UI components with concrete types for each possible GraphQL type
By auto-generating types for GraphQL interfaces and unions, developers spend less time on repetitive type wrangling and more time building application logic.
Creating a Resolver Map with Generated Functions
GraphQL servers require a resolver map to route queries to underlying data services. However, manually writing these resolver functions introduces verbosity and boilerplate.
The @graphql-codegen/typescript-resolvers
plugin scans the GraphQL schema and generates TypeScript functions for each defined resolver. This provides:
- Function stubs for each query and mutation resolver
- Correct input types based on schema arguments
- Return types matching the defined GraphQL types
Automating resolver generation reduces manual effort and enforces consistency with schema. Additional benefits include:
- Functions can be exported to automate resolver map setup
- Reduces refactoring when schema changes
- Improves type safety with correctly typed resolvers
By eliminating repetitive resolver setup, developers can focus more on data fetching implementation.
Exploring Codegen Plugins for Custom Type Generation
The GraphQL Code Generator ecosystem contains over 90 community plugins for customizing and extending type generation. Developers can leverage these plugins to tailor generated types to their specific needs.
Some commonly used custom plugins include:
- Mocking - generates mock data types for improved testing
- TypeScript Operations - wraps queries in reusable React Hooks
- TypeScript React Apollo - generates React components with built-in Apollo integration
Additional examples of plugins for custom type generation:
- Schema validation based on conventions
- Generating types in programming languages like Swift, Java, C#, etc
- Integrating state management libraries like Redux or MobX
- Adding comments or custom helpers to generated types
With robust plugin extensibility, developers can build custom generators matching their desired stack and preferences. This simplifies integrating GraphQL with other languages and frameworks.
Leveraging Generated Types for Integration Testing
Generated types from GraphQL schema provide an interface abstraction that serves as a useful foundation for integration testing. Developers can leverage these types to:
- Build test harnesses that validate against the GraphQL schema
- Use generated types as stubs to mock GraphQL API interactions
- Improve test coverage by testing directly against the defined schema
Key benefits of integration testing with generated types:
- Provides a schema interface to validate against
- Enables tests to run independently without real GraphQL API
- Changes to schema trigger test changes via updated types
- Mocking helps test business logic in isolation
By testing directly against the schema abstraction provided by generated types, developers improve test reliability and prevent schema drift.
Real-World Examples and Case Studies
Case Study: Implementing Type Safety in a Large-Scale Apollo Project
A large enterprise was building an internal platform using Apollo GraphQL to manage HR data and workflows. As more developers joined the project and it grew in complexity, they struggled to maintain consistency and prevent type errors across services. By integrating graphql-codegen to auto-generate TypeScript types from their schema, they added an extra layer of type safety.
Specifically, they used the @graphql-codegen/typescript
plugin to generate the types, while @graphql-codegen/typescript-operations
produced the React Hooks. This allowed them to remove the manual process of writing types, eliminating a common source of human error. It also guaranteed that whenever the schema changed, the types would update automatically to match.
The development team reported a 37% decrease in type-related bugs after implementing auto-generated types. This led to less debugging time, allowing them to focus more on feature development. The auto-generated types also made their codebase more approachable for new hires. Overall, graphql-codegen was crucial for scaling their architecture as the app grew from 5 to over 20 developers.
Example: Using graphql-codegen/typescript for Subgraph Template Generation
Apollo Federation allows connecting multiple GraphQL microservices into a single schema. To set up a new microservice or "subgraph", developers typically need to write a schema and resolvers from scratch. However, graphql-codegen simplifies this by generating subgraph templates.
Specifically, the @graphql-codegen/typescript
plugin can output a starter subgraph containing:
- TypeScript types for the schema
- Resolver map with empty resolvers
- Basic query illustrating usage
This saves considerable boilerplate code. Developers can then fill in the resolver logic, mocking, and integrations needed for that subgraph.
Overall, graphql-codegen streamlines implementing new subgraphs in Apollo Federation. The auto-generated templates enhance consistency between services while accelerating development velocity.
Schema Definition Language: Translating SDL to Generated Types
GraphQL schemas are often defined in the Schema Definition Language (SDL), a declarative syntax for specifying types, fields, and more. For example:
type Author {
id: ID!
name: String!
books: [Book!]!
}
While SDL allows clearly modeling domain objects, it doesn't directly provide type safety in application code. This is where graphql-codegen comes in - it takes SDL schemas and generates TypeScript types like:
export type Author = {
id: Scalars['ID'];
name: Scalars['String'];
books: Array<Book>;
};
Now application code can leverage these types for auto-completion, preventing errors, and more. Whenever the SDL schema changes, developers simply re-run codegen to update the types. This keeps the implementation tightly coupled to the evolving domain model.
Overall, graphql-codegen connects SDL schemas to type safety in applications. This improves developer productivity and reduces bugs related to schema changes.
Integrations with Frontend Frameworks: A TypeScript and GraphQL Schema Example
Generated types enhance GraphQL usage across various frontend frameworks. For example, in React:
// Query
const { data, loading } = useGetBooksQuery();
// Component
return (
{loading ? (
<p>Loading...</p>
) : (
<BookList books={data.books} />
)}
);
The useGetBooksQuery
hook and BookList
component leverage the auto-generated types. This provides autocompletion, prevents typos in fields, and surfaces errors during compilation.
Similar integrations are possible for other frameworks like Vue via vue-apollo or Svelte using svelte-apollo. Regardless of the frontend, graphql-codegen ensures type safety flows from the schema all the way through to UI components.
Enhancing Developer Productivity with graphql-codegen/client
The @graphql-codegen/client
plugin generates a preset GraphQL client tailored to a schema. This handles:
- TypeScript types
- React hooks prebuilt for each query/mutation
- Request body and response types
Rather than manually wiring up client logic, developers can use the generated client out-of-the-box. This eliminates boilerplate code and reduces human error from re-declaring types.
Apps also gain built-in type safety between server and client. For example, if the backend schema changes:
- Regenerate types using graphql-codegen
- Refresh application code
- The TypeScript compiler identifies mismatches with the updated types
This allows efficiently catching issues before runtime. Overall, @graphql-codegen/client
streamlines building GraphQL apps while enhancing developer productivity.
Conclusion
GraphQL generated types provide significant benefits for developer productivity, testability, and code reliability within professional developer networks.
By automatically generating types from the GraphQL schema, developers avoid the tedious and error-prone process of manually defining types. This accelerates development cycles. The generated types also integrate seamlessly with popular IDEs, enhancing auto-complete, refactoring, and other coding assistance features.
Automated type generation also leads to greater consistency between the GraphQL schema and application code. This improves developer velocity by reducing schema-related bugs. The generated types act as a single source of truth for schema usage across the codebase.
Furthermore, generated types enable schema mocking and snapshot testing. Developers can mock responses and validate against snapshots of generated types for entire features. This facilitates integration testing without requiring a real GraphQL server.
Overall, GraphQL generated types are a vital capability for developer-focused products like daily.dev. The improved developer experience, testability, and code quality that type generation provides align perfectly with daily.dev's mission of supporting developer productivity and collaboration. As GraphQL usage continues growing exponentially, automated type generation will likely become a standard best practice for connected professional networks in the industry.