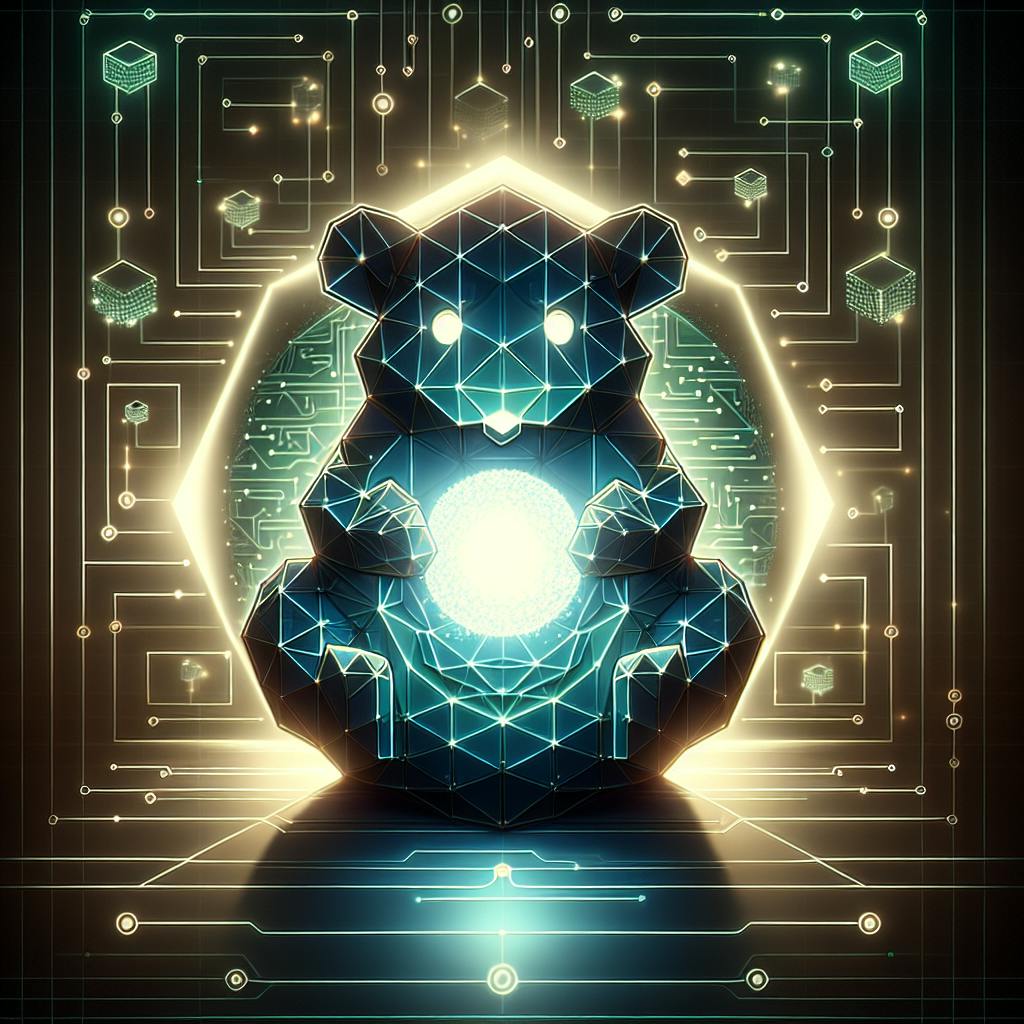
Learn essential tips for preventing Golang memory leaks to enhance application performance and stability with effective memory management practices.
Preventing memory leaks in Golang is crucial for efficient and stable application performance. Here are the key points:
- Avoid circular references between objects to allow proper garbage collection
- Properly manage goroutines and channels to prevent leaks from blocked or non-terminating goroutines
- Release unnecessary resources like files, network connections, and channels when no longer needed
- Optimize concurrency using worker pools and proper goroutine termination signals
- Monitor memory usage regularly with tools like pprof, Prometheus, and Pyroscope
- Avoid retaining large objects and implement bounded caches or expiration policies
- Use weak references for caches and global variables to allow garbage collection
- Regularly profile your application to identify and fix memory leaks early
- Review code for common leak patterns like circular references and unclosed resources
- Implement expiration policies with time-to-live (TTL) for caches and global variables
By following these tips, you can prevent memory leaks and ensure your Go applications run efficiently and reliably.
Quick Comparison: Memory Leak Prevention Strategies
Strategy | Description |
---|---|
Avoid Circular References | Break cyclic references between objects |
Manage Goroutines | Use channels, context cancellation, and sync.WaitGroup |
Release Resources | Close files, connections, and channels when done |
Optimize Concurrency | Use worker pools and terminate goroutines properly |
Monitor Memory Usage | Use pprof, Prometheus, Grafana, and other tools |
Avoid Retaining Large Objects | Release references and use bounded caches |
Use Weak References | Allow garbage collection of cached objects |
Regular Profiling | Identify and fix memory leaks early |
Review Code Patterns | Watch for circular references and unclosed resources |
Implement Expiration Policies | Set time-to-live (TTL) for cached objects |
1. Avoid Circular References
Circular references occur when objects reference each other in a loop, preventing the garbage collector from identifying them as unused and reclaiming their memory. This can lead to memory leaks in your Go application.
Causes of Circular References
- Data structures that form cyclic references
- Careless design of data relationships
Consequences
- Memory leaks
- Performance issues
- Crashes
- Security vulnerabilities
Prevention Strategies
- Refactor code to break the cycle
- Use weak references or expiration policies for caches and global variables that store large objects
By avoiding circular references, you can prevent memory leaks and ensure your Go application runs smoothly and efficiently.
2. Properly Manage Goroutines
Goroutines are lightweight threads that can improve the performance and concurrency of your Go application. However, if not managed properly, they can lead to memory leaks. A goroutine that never terminates or is blocked indefinitely can hold references to objects, preventing the garbage collector from reclaiming memory.
Causes of Goroutine Leaks
- Creating goroutines that never exit or are blocked indefinitely
- Not closing channels properly
- Not using context cancellation to signal goroutine termination
Consequences
Consequence | Description |
---|---|
Memory Leaks | Goroutines hold references to objects, preventing memory reclamation |
Performance Issues | Leaks lead to increased memory usage, slowing down the application |
Crashes | Unmanaged goroutines can cause the application to crash |
Security Vulnerabilities | Memory leaks can expose sensitive data, leading to security risks |
Prevention Strategies
1. Use channels to signal goroutine termination
Use channels to signal when a goroutine should exit, ensuring it releases its resources.
2. Implement context cancellation
Use context cancellation to propagate termination signals to goroutines, allowing them to exit cleanly.
3. Use sync.WaitGroup
Use sync.WaitGroup
to ensure all goroutines have completed before exiting the program.
4. Avoid creating unlimited goroutines
Consider using a worker pool to manage concurrency and prevent unlimited goroutine creation.
By properly managing goroutines, you can prevent memory leaks and ensure your Go application runs efficiently and reliably.
3. Release Unnecessary Resources
Releasing unnecessary resources is crucial to prevent memory leaks in Go. Failing to close resources like files, network connections, or channels can cause memory leaks.
Causes of Resource Leaks
Cause | Description |
---|---|
Unclosed files or network connections | Resources remain open, consuming memory |
Unused channels or goroutines | Resources hold references to objects, preventing memory reclamation |
Unreleased system resources | System resources remain allocated, causing memory leaks |
Consequences
Consequence | Description |
---|---|
Memory Leaks | Unclosed resources hold references to objects, preventing memory reclamation |
Performance Issues | Leaks lead to increased memory usage, slowing down the application |
Crashes | Unmanaged resources can cause the application to crash |
Prevention Strategies
1. Use defer statements: Ensure resources are closed or released when no longer needed.
2. Implement resource cleanup: Release resources when they are no longer required.
By releasing unnecessary resources, you can prevent memory leaks and ensure your Go application runs efficiently and reliably.
4. Optimize Concurrency
Optimizing concurrency is crucial to prevent memory leaks in Go. Concurrency allows a program to execute multiple tasks simultaneously, improving responsiveness and performance. However, if not managed properly, concurrency can lead to memory leaks.
Goroutine Leaks
Goroutines are lightweight threads of execution that can cause memory leaks if not terminated properly. A goroutine leak occurs when a goroutine is created and never terminated, holding references to objects in memory and preventing them from being garbage collected.
Prevention Strategies
Strategy | Description |
---|---|
Use channels to signal goroutine termination | Allows the goroutine to terminate cleanly, releasing any held resources. |
Use sync.WaitGroup |
Ensures that all goroutines have completed before exiting the program. |
Worker Pools
Using a worker pool can help prevent unlimited creation of goroutines, which can lead to poor performance and memory leaks. A worker pool is a group of goroutines that can be reused to perform tasks, reducing the overhead of creating new goroutines.
By optimizing concurrency, you can prevent memory leaks and ensure your Go application runs efficiently and reliably.
5. Monitor Memory Usage
Monitoring memory usage is crucial to prevent memory leaks in Go applications. Regular monitoring helps identify potential issues before they become critical.
Methods for Monitoring Memory Usage
Method | Description |
---|---|
Using pprof |
Analyze runtime profiling data to monitor memory usage and identify potential leaks. |
Logging and Monitoring | Regularly monitor memory usage in production to detect leaks. Tools like Prometheus and Grafana can alert developers to unexpected changes. |
Profiling Tools | Use tools like Pyroscope, Go Tool Pprof, and runtime/metrics package to monitor memory allocation and identify potential leaks. |
By regularly monitoring memory usage, you can prevent memory leaks and ensure your Go application runs efficiently and reliably.
6. Avoid Retaining Large Objects
When working with large objects in your Go application, it's crucial to avoid retaining them unnecessarily. This can lead to memory leaks, as the garbage collector (GC) cannot reclaim the memory occupied by these objects.
Causes of Memory Leaks
Cause | Description |
---|---|
Retaining large objects | Objects remain in memory, preventing the GC from reclaiming memory |
Cyclic references | Data structures form cyclic references, leading to memory leaks |
Prevention Strategies
1. Release references: Ensure you release references to large objects once they are no longer needed, allowing the GC to identify them as unused and reclaim their memory.
2. Implement bounded caches or expiration policies: Ensure large objects do not reside in memory indefinitely by implementing strategies like bounded caches or expiration policies.
By following these best practices, you can prevent memory leaks and ensure your Go application runs efficiently and reliably.
Remember, understanding memory allocation and following best practices is crucial to ensure efficient memory usage and prevent leaks in Go applications.
sbb-itb-bfaad5b
7. Use Weak References
Weak references are a useful strategy for preventing memory leaks in Go. They allow you to maintain a reference to an object without preventing the garbage collector (GC) from reclaiming its memory.
Benefits of Weak References
Benefit | Description |
---|---|
Prevents memory leaks | Allows the GC to reclaim memory when an object is no longer needed |
Efficient memory usage | Ensures that objects are garbage collected when they are no longer referenced |
Implementing Weak References
You can implement weak references in Go using the sync/atomic
package or by utilizing third-party libraries.
Example: Using Weak References in Caches
Weak references are particularly useful for caches and global variables that store large objects. By using a weak reference to store a large object in a cache, you can ensure that the GC reclaims its memory when the object is no longer referenced.
By using weak references, you can prevent memory leaks and ensure efficient memory usage in your Go application.
8. Regularly Profile Your Application
Regular profiling of your Go application is essential to identify memory leaks and optimize performance. Profiling helps you understand how your application uses resources like CPU, memory, and goroutines. This knowledge enables you to pinpoint areas of improvement, reducing the likelihood of memory leaks and improving overall application efficiency.
Profiling Tools
Go provides built-in profiling tools, including pprof
and net/http/pprof
. These tools allow you to collect and analyze profiling data, providing insights into your application's performance. Additionally, third-party libraries like Pyroscope offer more advanced profiling capabilities, making it easier to identify memory leaks and optimize performance.
Profiling Techniques
To effectively profile your application, follow these techniques:
Technique | Description |
---|---|
Collect CPU, memory, and goroutine profiles | Use pprof to collect profiling data. |
Analyze profiles | Use go tool pprof or visual tools like Pyroscope to analyze profiles. |
Identify resource-intensive functions and goroutines | Pinpoint areas of improvement to reduce resource usage and prevent memory leaks. |
Optimize code | Refactor code to reduce resource usage and prevent memory leaks. |
By regularly profiling your application, you can detect memory leaks early, optimize performance, and ensure your Go application runs efficiently and effectively.
9. Review Code for Common Leak Patterns
When preventing memory leaks in Golang applications, reviewing code for common leak patterns is essential. This involves identifying areas of your code that may be prone to memory leaks and taking steps to mitigate them.
Circular References
One common pattern to watch out for is circular references. These occur when two or more objects reference each other, preventing the garbage collector from reclaiming memory.
Example | Description |
---|---|
type A struct {B *B} type B struct {A *A} |
Two objects reference each other, creating a circular reference. |
In this case, if the references between A
and B
are not cleared when they are no longer needed, a circular reference will prevent the garbage collector from reclaiming the memory, resulting in a memory leak.
File Handles or Network Connections
Another common pattern to review is the proper closure of file handles or network connections. Failing to close these resources can lead to memory leaks.
Example | Description |
---|---|
file, err := os.Open("data.txt") // Oops! Forgot to close the file handle |
Failing to close a file handle can lead to a memory leak. |
To avoid this, ensure that you properly close file handles and release network connections when they are no longer needed.
By regularly reviewing your code for these common leak patterns, you can identify and fix potential memory leaks before they become a problem.
10. Implement Expiration Policies
To prevent memory leaks, it's essential to implement expiration policies for caches and global variables that store large objects. This involves setting a time-to-live (TTL) for each object, after which it is automatically removed from memory.
Why Expiration Policies Matter
Without expiration policies, objects can remain in memory indefinitely, leading to memory leaks. This is particularly problematic in applications with limited memory resources or those that handle large amounts of data.
Implementing Expiration Policies
To implement expiration policies, you can use the following techniques:
Technique | Description |
---|---|
Weak References | Use weak references to store objects in caches or global variables. Weak references allow the garbage collector to reclaim memory when the object is no longer referenced. |
TTL-Based Eviction | Implement a TTL-based eviction mechanism that removes objects from memory after a specified time period. |
Periodic Cleanup | Schedule periodic cleanup tasks to remove expired objects from memory. |
Example
Here's an example of implementing a TTL-based eviction mechanism using a cache:
var cache = map[string]struct {
data []byte
timestamp time.Time
}()
const ttl = 10 * time.Second
func cacheData(key string, data []byte) {
cache[key] = struct {
data []byte
timestamp time.Time
}{
data: data,
timestamp: time.Now(),
}
}
func evictOldEntries() {
for key, item := range cache {
if time.Since(item.timestamp) > ttl {
delete(cache, key)
}
}
}
func main() {
//... your application logic...
// Periodically clean up old cache entries
ticker := time.NewTicker(time.Second)
for range ticker.C {
evictOldEntries()
}
}
In this example, we use a TTL-based eviction mechanism to remove expired objects from the cache. This ensures that objects are properly garbage collected, preventing memory leaks and optimizing memory usage.
Conclusion
Preventing memory leaks in Golang applications is crucial for efficient and stable performance. By following the 10 tips outlined in this article, developers can ensure their applications are free from memory leaks.
Key Takeaways
- Go's garbage collector is not infallible and requires mindful coding practices.
- Regular profiling and monitoring are essential to detect memory leaks early.
- Following best practices and understanding potential pitfalls are key to writing memory-efficient Go applications.
Remember
The earlier you catch a memory leak, the easier it is to fix. Be proactive in monitoring and profiling your Go programs to ensure efficient and stable performance.
FAQs
Can Golang have a memory leak?
Yes, despite having a garbage collector, Go applications can still experience memory leaks.
Are memory leaks possible in Golang?
Memory leaks can occur in Go due to:
Reason | Description |
---|---|
Long-lived references | Objects remain in memory as long as there's a reference to them. |
Goroutines | Unused or blocked goroutines that never exit can cause memory leaks. |
By understanding these potential pitfalls, developers can take proactive measures to prevent memory leaks in their Go applications.