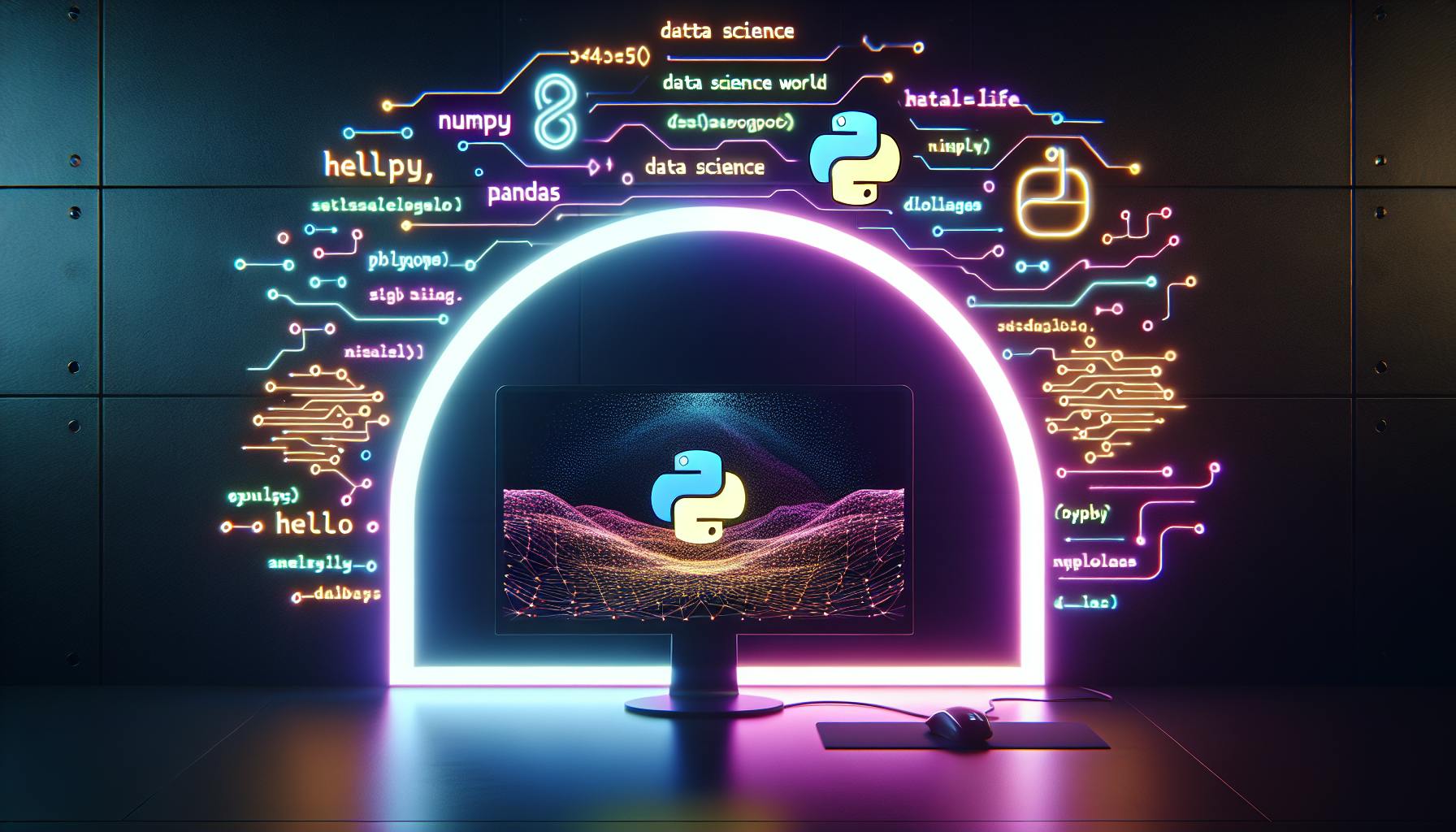
An introduction to Python for data science, covering setting up, Python basics, key libraries, data handling, visualization, machine learning, and further learning resources.
Python is a powerful and popular language for data science, offering a wide range of tools and libraries to help you handle, analyze, and visualize data. Here's a quick overview of what you'll learn in this guide:
- Setting Up: How to prepare your computer for Python data science projects with tools like Anaconda and Jupyter Notebooks.
- Python Basics: An introduction to Python's syntax and its data types.
- Key Libraries: A look at essential libraries including NumPy, Pandas, Matplotlib, Seaborn, and Scikit-learn.
- Data Handling: How to read, write, and wrangle data effectively.
- Visualization: Tips for creating insightful and attractive visualizations.
- Machine Learning: Steps to build predictive models using Python.
- Further Learning: Suggestions for books, courses, and communities to continue your Python data science journey.
Whether you're new to programming or looking to dive into data science, this guide will equip you with the knowledge to start analyzing data and building models with Python.
Why Use Python for Data Science?
Python is the top pick for people working on data science and AI, beating other languages like R, Java, C/C++. Here are the main reasons why Python is a favorite:
It's Easy to Learn
Python is straightforward and its code is easy to read, which is perfect for beginners. You usually need fewer lines of code compared to other languages, making it quicker to get your ideas up and running.
It Does Everything
You can use Python for all parts of working with data - from getting and cleaning data, to analyzing it, making graphs, building machine learning models, and putting those models to use. This makes Python really handy.
Lots of Helpful Tools
Python has tons of free tools and libraries for working with data, like NumPy for calculations, Pandas for data wrangling, and Matplotlib and Seaborn for making graphs. For machine learning, there's Scikit-learn and deep learning libraries like TensorFlow. These tools help you do more, faster.
Lots of People Use It
There's a big community of Python users. This means lots of guides, forums, and help available if you get stuck. Plus, there are many conferences and meetups about Python.
Fast Enough
Even though Python might not be as fast as C++ or Java, it has ways to speed things up with special frameworks like Numba and Cython. Usually, Python is fast enough for what you need, and it lets you work more quickly.
Here's a simple comparison of Python with other languages for data science and AI:
Language | Easy to Learn | Tools | Community | Speed |
---|---|---|---|---|
Python | Yes | Lots | Big | Good Enough |
R | Pretty Easy | Plenty | Big | Good Enough |
Java | So-So | Plenty | Big | Really Fast |
C/C++ | Hard | Plenty | Big | Really Fast |
In short, Python is great because it's easy to learn, has everything you need for data science, a big community, and it's fast enough for most projects. It's a solid choice for anyone getting into data science.
Setting Up a Python Data Science Environment
Installing Python & Package Manager
Getting started with Python for data science means you need to put Python on your computer first. Here's how you can do it easily:
Windows
- Visit python.org and grab the latest Python version for Windows. Pick the 64-bit installer.
- Open the installer file you downloaded and make sure to check Add Python to PATH. This makes using Python later much simpler.
- Open Command Prompt and type
python --version
to make sure Python is ready to go.
Mac
- Head to python.org and download the latest Python for Mac. Choose the Mac OS X 64-bit/Intel installer.
- Open the installer and follow the steps to put Python on your Mac.
- Open Terminal and type
python3 --version
to check if Python is installed.
Linux
Most of the time, Linux already has Python. To check:
- Open the terminal.
- Type
python3 --version
to see if Python is there.
If you need to install it, use your Linux package manager (like sudo apt install python3
for Debian/Ubuntu).
Next, we need pip, Python's package manager, to get data science libraries later:
curl https://bootstrap.pypa.io/get-pip.py -o get-pip.py
python get-pip.py
Now you're set with Python and pip!
Python IDEs and Notebooks
When writing Python code, you can choose from different tools:
- A web tool for running Python code piece by piece
- Great for trying out code and sharing results
PyCharm
- A full-featured tool for bigger projects
- Has lots of helpful features for writing and fixing code
- A lighter tool that can be customized
- Good for both simple and complex projects
Jupyter Notebook is a good starting point for beginners. For bigger projects, try PyCharm or VS Code.
To get Jupyter Notebook:
pip install jupyter
After installing, start Jupyter with jupyter notebook
, and it will open in your browser.
Essential Data Science Packages
Here are some key libraries for working with data:
NumPy: For handling large sets of numbers and doing math.
Pandas: Makes it easy to load, change, and look at data. Perfect for tables.
Matplotlib: Helps you make graphs and charts.
Seaborn: Builds on Matplotlib to make pretty and detailed graphs.
Scikit-Learn: A library for machine learning. It has everything you need to create models.
You can install these with pip:
pip install numpy pandas matplotlib seaborn scikit-learn
This gets your computer ready for analyzing data and building machine learning models with Python!
Python Syntax Basics
Numeric, String, and Other Data Types
Python works with a few main types of data that you'll use a lot when looking at data:
Numbers: These are for counting or measuring things. You can have whole numbers or numbers with decimals. Examples:
x = 10 # Integer
y = 3.14 # Float
Strings: These are for text. You wrap words in quotes. Examples:
text = "Introduction to Python"
name = 'Ada Lovelace'
You can also put strings together:
full = name + " " + text
print(full) # Ada Lovelace Introduction to Python
Lists: These are for keeping a bunch of items in order. You use square brackets. Examples:
nums = [1, 5, 2, 8]
fruits = ["Apple", "Banana", "Orange"]
Dictionaries: These are for storing info that matches up, like names to phone numbers. You use curly braces. Examples:
person = {
"name": "Maria",
"age": 32,
"job": "Developer"
}
Python is smart and figures out what type of data you're using as you go. This makes it easy to mix and match different kinds of data.
Conditional Statements and Loops
To make choices and do things over and over in Python, you use if/else and for/while statements.
If statements help you decide to do something if a condition is true:
num = 7
if num > 5:
print("Number exceeds 5") # This runs
You can add more choices with if/else:
num = 3
if num > 5:
print("Greater than 5")
else:
print("Less than or equal to 5") # This runs
For loops repeat actions for each item in a list:
fruits = ["apple", "banana", "orange"]
for fruit in fruits:
print("Fruit:" + fruit)
This goes through each fruit and prints it out.
While loops keep going as long as something is true:
count = 0
while count < 5:
print(count)
count = count + 1 # Adds 1 each time
It shows numbers from 0 to 4. The loop stops when count hits 5.
These tools help you control your program, making decisions and repeating actions as needed.
sbb-itb-bfaad5b
Working with Data in Python
Reading and Writing Data
When you're working with data in Python, you'll often need to read data from files and save your results. Here's what you should know:
-
Pandas is your go-to library for dealing with tables of data, like spreadsheets. It can read files like CSV and JSON with commands like
read_csv()
andread_json()
. -
To save your data back into files, Pandas uses
to_csv()
andto_json()
, letting you decide how you want your file to look. -
For simple text files, you can use Python's own
open()
function to read and write. This lets you work with files line by line or all at once. -
If you're dealing with numbers, NumPy has tools like
loadtxt()
andsavetxt()
for simple data files. -
Always take a quick look at your data after loading it to spot any errors early. Commands like
.head()
and.info()
, or making a quick histogram, can help with this.
Data Wrangling with Pandas
Pandas is great for cleaning up and organizing your data:
- Selecting Data: You can pick out specific parts of your data using indexing or conditions.
- Filtering: You can easily keep the data you want and remove the rest based on certain rules.
- Making Changes: Create new columns or change existing ones to get your data just right.
- Combining Data: You can stitch different datasets together to get all your information in one place.
- Summarizing: Break your data into groups, then summarize each group with totals or averages.
- Changing Shape: Rearrange your data to better suit your analysis needs.
These tools help you get your data ready for deeper analysis or modeling.
Handling Missing Data
Missing or incorrect data is common, but it can mess up your analysis:
-
Use Pandas to spot missing values with
isnull()
ornotnull()
. -
You can remove rows or columns with missing values using
.dropna()
, or fill them in using.fillna()
with a basic value like the average. - For more complex fixes, you might use interpolation or even machine learning to guess the missing values.
- It's important to think about why data might be missing to choose the best way to handle it.
Fixing missing data helps make sure your analysis is accurate and reliable.
Data Visualization with Python
Introduction to Matplotlib
Matplotlib is a go-to library for making charts and pictures out of data in Python. It's like a Swiss Army knife for plotting because it has lots of features:
- It loves working with NumPy, which is great for crunching numbers.
- You can make all sorts of charts - like lines, dots, bars, and even pies.
- You get to tweak a lot of things to make your chart look just right.
- If you've used MATLAB before, you'll find Matplotlib familiar.
- It plays nice with other data tools like Pandas and scikit-learn, making your data work smoother.
Matplotlib has two ways to make plots. The simple way is quick for checking out your data. The detailed way gives you more control to make things perfect.
Seaborn for Statistical Plots
Seaborn makes your data look good without much fuss. It's built on Matplotlib but focuses on making charts that tell you about your data's main points, like what's typical or how things relate.
Here's why Seaborn is cool:
- It's got special charts like heatmaps and violin plots that show a lot of info.
- The charts look nice out of the box, which can help your data stand out.
- It's great for looking at one thing (like ages) or two things together (like age and height).
- Seaborn works directly with Pandas, so you can get insights fast.
- It uses colors and shapes to help show what's going on in your data.
- It can even include lines or trends in your charts to make things clearer.
Seaborn makes it easier to get a feel for your data, showing you the big picture and the details.
Machine Learning Models in Python
Linear and Logistic Regression
Linear regression and logistic regression are two straightforward ways to predict numbers and categories. Here's how to use them with Python's Scikit-learn:
- First, bring in
LinearRegression
andLogisticRegression
fromsklearn.linear_model
. - Next, split your dataset into parts for training and testing using
train_test_split
. - Train (fit) the model with your training data.
- Use the model to make predictions on your test data.
- Check how accurate your predictions are with scores like
r2_score
for linear regression andaccuracy_score
for logistic regression.
For instance, if you're trying to guess house prices:
from sklearn.linear_model import LinearRegression
from sklearn.model_selection import train_test_split
X = df[['lotsize', 'bedrooms']]
y = df['price']
X_train, X_test, y_train, y_test = train_test_split(X, y)
model = LinearRegression()
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
r2_score(y_test, y_pred)
Logistic regression works similarly but for picking categories instead of numbers.
Model Evaluation Techniques
To make sure our models are doing a good job, we need to check them in different ways:
Train/Test Splits
- Divide your data into two parts: one for training and one for testing.
- Train your model with one part and test it with the other.
- This helps guess how well the model will do with new, unseen data.
Classification Reports & Confusion Matrices
- A classification report gives you details like precision and recall for each category.
- A confusion matrix shows what the model guessed against what's actually true.
- These help see if the model is favoring certain categories over others.
Cross-Validation
- Do the train/test split several times in different ways.
- This helps make sure the model works well across different sets of data.
- The KFold method is one way to do this.
Overfitting Checks
- Compare how the model does on both the training and test sets.
- A big difference might mean the model is overfitting, which means it's memorized the training data too well but won't perform well with new data.
- To fix this, you might need to make the model simpler.
These methods help us find and fix issues, making our machine learning models better.
Next Steps
Here are some extra resources to help you get even better at using Python for data science:
Books
- "Python for Data Analysis" by Wes McKinney - This book is all about how to use Pandas for working with data.
- "Introduction to Machine Learning with Python" by Andreas Mueller & Sarah Guido - This book is a good start to understand how machine learning works and how to use Scikit-Learn.
Courses
- "Machine Learning" by Andrew Ng (Coursera) - A well-liked course that teaches the basics of machine learning.
- "Applied Data Science with Python" Specialization (Coursera)- Covers a wide range of tools and ways to do data science with Python.
Communities
- Kaggle - A place where data scientists can compete and help each other out.
- r/learnmachinelearning - A Reddit community for learning and talking about machine learning.
- PyData - A worldwide group that supports using Python for data tasks through local meetups and events.
The best way to get better is by practicing and using what you've learned on actual projects. And when you're working on something new, don't hesitate to ask for help or share your work with these communities.