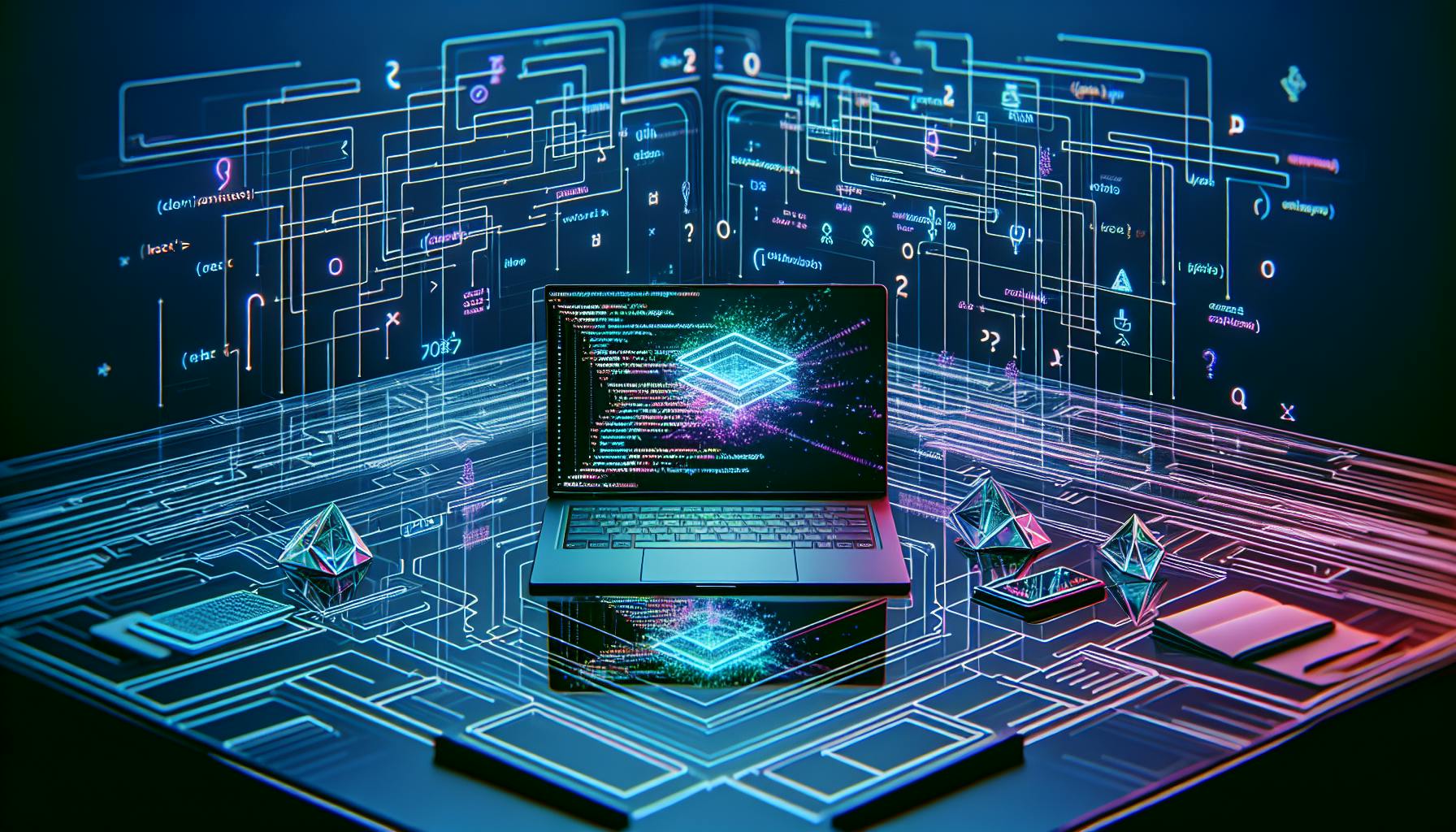
Get an overview of the latest version of JavaScript, ES2023, and learn about its key features, the TC39 proposal process, browser support, practical applications, and future directions.
JavaScript, a cornerstone of web development, has evolved significantly since its inception. The latest version, ES2023, introduces a suite of new features aimed at enhancing the language's flexibility and developer experience. Here's a quick overview of what ES2023 brings to the table:
- Array.prototype.findLast() and findLastIndex(): Methods for finding the last item in an array that meets a condition.
- Hashbang Grammar: Allows JavaScript files to specify the script interpreter directly, facilitating cross-platform scripts.
- Symbols as WeakMap Keys: Enables the use of symbols for more secure and organized key management in WeakMaps.
- Non-Mutating Array Operations: Methods like
toReversed()
andtoSorted()
that operate on arrays without altering the original data.
These updates, alongside the ongoing evolution of JavaScript through the TC39 proposal process and growing browser support, ensure JavaScript remains a robust, versatile language for web developers. Understanding these changes and how they compare to previous versions can help developers leverage JavaScript more effectively in their projects.
JavaScript Latest Version: ES2023 Overview
Key Features and Improvements
ES2023 brings in some cool new stuff that makes JavaScript even better:
Array Positions
- Array.prototype.findLast() - Helps you find the last item in a list that meets a certain condition.
const array = [1, 2, 3, 4];
array.findLast(n => n % 2 === 0); // 4
- Array.prototype.findLastIndex() - Tells you where the last item that meets a condition is in the list.
const array = [1, 2, 3, 4];
array.findLastIndex(n => n % 2 === 0); // 3
These tools make it easier to work with lists, especially when you're looking for something specific at the end.
Hashbang Grammar
The hashbang (#!
) lets you run a JavaScript file by telling the computer which program to use right at the start:
#!/usr/bin/env node
console.log('Hello world!');
This is great for making your code work well on different computers.
Symbols as WeakMap Keys
Now, you can use symbols as keys in WeakMaps, which is like giving your keys a secret name:
const key = Symbol('secret');
const weakMap = new WeakMap();
weakMap.set(key, 'password123');
This helps keep things organized and avoids mix-ups.
Non-Mutating Array Operations
New ways to change lists, like toReversed()
and toSorted()
, let you work with copies instead of the original list:
const array = [3, 1, 2];
const reversed = array.toReversed(); // [2, 1, 3]
const sorted = array.toSorted(); // [1, 2, 3]
array; // [3, 1, 2] (unchanged)
This is good because it means you won't accidentally change your original list.
Comparison with Previous Versions
Feature | ES2022 & Earlier | ES2023 |
---|---|---|
Array Positions | findLast() not available |
Added findLast() and findLastIndex() |
Hashbang | Not supported | Added hashbang grammar |
WeakMap Keys | Symbols not allowed as keys | Symbols now allowed as keys |
Array Operations | All methods mutate original array | New non-mutating variants introduced |
As you can see, ES2023 adds some pretty useful things that weren't there before. It's all about making your job as a developer easier and your code better.
Understanding ECMAScript Proposals
The group that sets the rules for JavaScript is called Ecma International, and they use something called ECMAScript (or ES for short) to do this. When JavaScript gets new features, it's because they've been added to ECMAScript.
TC39 Committee
A group of people called TC39, which includes folks from big companies that make web browsers and those who use JavaScript a lot, decides what new features should be added to JavaScript. They meet regularly to talk about and decide on these new ideas.
Stage 0 - Strawperson
This is just the beginning, where someone says, "Hey, I have an idea for JavaScript." It's a way to see if the committee is interested.
Stage 1 - Proposal
Now the idea is more serious. It has documents explaining how it works and why it's good. The TC39 group looks at it and gives their first thoughts.
Stage 2 - Draft
The idea has grown up a bit. It has a rough plan, and some people start trying to make it work in a basic way.
Stage 3 - Candidate
Everything about the idea is written down clearly, with tests and examples. Some web browsers might start to show how it could work in real life.
Stage 4 - Finished
The idea is now a full rule. Web browsers start using it for everyone.
This process makes sure that new features fit well into JavaScript and are supported properly.
Significance for Developers
For people who make websites or apps, understanding how new JavaScript features are decided on is helpful because:
- You can know what new things are coming and get ready for them.
- You can share your thoughts on new features, which might help shape the future of JavaScript.
- You can plan your projects better, knowing what JavaScript will be able to do.
- For features that are almost ready, you can start using tools that pretend they're already part of JavaScript.
As JavaScript gets new features, it becomes easier and more powerful to use. The TC39 group helps make sure that the language grows in a way that's good for everyone.
Browser Support for ES2023
ES2023, the latest version of JavaScript, is pretty new since it was just finished in 2023. This means not all web browsers can use its new features yet, but they're starting to:
Firefox
- Firefox version 108 and up can use:
- Array.prototype.findLast()
- Array.prototype.findLastIndex()
- It also kind of supports the hashbang feature, but not fully.
Chrome
- Chrome version 110 and above supports:
- Array.prototype.findLast()
- Array.prototype.findLastIndex()
- Chrome Canary, which is like a test version of Chrome, is trying out some ES2023 stuff too.
Safari
- Safari hasn't added any ES2023 features yet.
- It usually takes a bit longer for Safari to get new JavaScript stuff.
Edge
- Edge version 109 and newer supports:
- Array.prototype.findLast()
- Array.prototype.findLastIndex()
- But it doesn't have other ES2023 features just yet.
Node.js
- Node.js version 19.0 and up can use:
- Array.prototype.findLast()
- Array.prototype.findLastIndex()
- It's expected to add more ES2023 features in future updates.
Transpilers
To use the new ES2023 features now, developers can turn ES2023 code into an older version that more browsers understand, using tools called transpilers, like Babel. This way, you can start using the new stuff even if browsers aren't ready for it yet.
Right now, the support for ES2023 is still growing. Big browsers like Firefox and Chrome are beginning to include some of its features. For details on what's supported where, you can check out Can I Use. Using transpilers helps make these new features usable today, while we wait for browsers to catch up over the next year.
Practical Applications and Examples
ES2023 introduces helpful new JavaScript features that have practical uses for developers building web applications and sites. Here are some real-world examples of how these features can be leveraged:
Simplifying Search in Large Data Sets
The Array.prototype.findLast()
and findLastIndex()
methods make it easier to access the last matching element in large arrays, such as those used in databases and data science applications.
// Find last user who is 18 years old in large array
const users = [/* array with 1000 users */];
users.findLast(user => user.age === 18);
This simplifies search algorithms without needing to iterate through the entire array.
Creating Secret Game Codes
Using Symbols as WeakMap keys enables creating hidden game data that players can't easily access, such as secret unlock codes:
const secretCode = Symbol('unlock-code');
const gameData = new WeakMap();
gameData.set(secretCode, '828-555-0113');
The Symbol key keeps the data private for gameplay mechanics.
Safely Transforming Data Sets
The new non-mutating array methods like toReversed()
let you manipulate data without changing the original source array:
let data = [/* critical measurement data */];
let reversedData = data.toReversed(); // Safely reverse
This is useful when working with sensitive source data that cannot be corrupted.
Creating Browser-agnostic Scripts
Using the hashbang syntax at the top of scripts allows them to reliably execute across different runtimes:
#!/usr/bin/env node
// Script runs properly on Node.js and browsers
This simplifies writing cross-environment JavaScript code.
Other Examples
Other practical ES2023 use cases include:
- Adding secret authentication keys in WeakMaps
- Safely sorting datasets without altering raw data
- Making node scripts directly executable from CLI
The new features offer many options to optimize real-world code.
sbb-itb-bfaad5b
Future Directions of JavaScript
JavaScript is always getting better, with new ideas on how to improve it. If you're a developer, it's good to know what's coming up so you can use the newest features.
Stage 2 and 3 Proposals
Right now, there are a few updates almost ready to join JavaScript:
- Top-Level
await
: This makes it easier to useawait
without wrapping it in an async function, which is great for working with asynchronous code. - Class Fields: This update lets you write class fields in a simpler way.
- Static Class Features: This adds a straightforward way to include static fields and methods in classes.
- Ergonomic Brand Checks: This makes it easier to check if an object belongs to a certain type, like an Array or Promise.
- Decimal: This adds a new type of number that's better for decimal math.
These updates are pretty close to being part of JavaScript, maybe in the next year or two.
Earlier Stage Ideas
There are also some new thoughts that might take longer to become part of JavaScript:
- Pattern Matching: This would allow JavaScript to match patterns, making it easier to work with different types of data.
- Spaceship Operator: This is a new way to compare things, which could return -1, 0, or 1 based on the comparison.
- Pipeline Operator: This could make it easier to chain functions together in a clear way.
These ideas are still early in the discussion, but they show what could be next for JavaScript.
Staying in the Loop
Here's how you can keep up with all the new JavaScript updates:
- Regularly check the TC39 Proposals page.
- Read articles on sites like 2ality that follow new JavaScript updates.
- Use tools like Babel to try out new features before they're officially part of JavaScript.
- Follow people from the TC39 committee on social media.
The TC39 committee, which helps decide on new features, likes hearing from developers. Your feedback can help shape the future of JavaScript.
In the next few years, we'll see both small changes and big new ideas added to JavaScript. By keeping up with these updates, we can get ready for and even help shape the JavaScript of the future.
Conclusion
Since 1995, JavaScript has grown from a basic tool for making websites a bit more fun to a powerful language used for complex applications on the web and mobile devices.
The latest updates, like those in ES2023, bring new features that make coding easier and safer. For example, we now have better ways to work with lists, secure ways to use symbols in WeakMaps, and methods to change lists without messing up the original data.
Looking ahead, there are exciting updates on the way, such as making it easier to use 'await' outside of functions, simplifying how we write classes, and new checks for object types. And there are even more ideas being talked about, like pattern matching and a new way to link functions together.
- For developers: Keeping an eye on what's new in JavaScript can really help. Knowing about new features early gives you time to learn and use them in your work.
- For businesses: Staying updated with JavaScript means your tech stays modern and your team can work more efficiently. Updating your code and how your team works can help you deliver better projects faster.
- For the community: Getting involved in the development of JavaScript means you can help make sure it grows in a way that's good for everyone. Your input on new ideas can help shape the future of JavaScript.
JavaScript keeps getting better, with each new update building on the last to meet new challenges. For those of us who use JavaScript, staying curious and ready to learn about the latest developments lets us turn new ideas into real-world solutions. JavaScript's future looks bright, and it's an exciting time to be part of this community!
Additional Resources
Here are some helpful links and places to learn more about JavaScript's latest version, ES2023, and how to stay on top of new changes in the language:
ES2023 Specification
- Official ES2023 Specification: The full official details of what's new in ES2023.
ES2023 References
- ES2023 Features List: A quick look at the new stuff in ES2023.
- ES2023 Browser Compatibility: See which web browsers can use ES2023 features.
JavaScript Proposals & TC39 Process
- TC39 GitHub Repo: Check out the new ideas for JavaScript and their progress.
- TC39 Proposal Process: Learn how new features are added to JavaScript.
ES2023 Tutorials & Experiments
- ES2023 on MDN: Guides on how to use ES2023 features.
- ES2023 Experiments: A place to try ES2023 features yourself.
Keeping Up with JavaScript
- 2ality Blog: A blog that keeps track of JavaScript updates.
- Addy Osmani Blog: Articles by a Chrome engineer about JavaScript.
- /r/JavaScript Subreddit: A community forum for JavaScript topics.
Keeping up with JavaScript's latest changes can be a bit of work, but it's really helpful for using the newest features and tools in your projects. These resources are great for learning about ES2023 now and for keeping an eye on what might be added to JavaScript in the future.
Related Questions
What is the latest version of JavaScript?
The newest version of JavaScript is called ECMAScript 2023 or ES2023 for short. It came out in June 2023. This update adds some cool things like new ways to work with lists, a feature for running scripts in different environments, and better organization for certain types of data.
Is there JavaScript ES7?
Yes, there is an ES7, also known as ECMAScript 2016. It was a smaller update that mainly introduced the async/await
feature, making it easier to deal with code that runs at different times.
Is ES14 released?
Yes, ES14 is another name for ECMAScript 2023, which was released in June 2023. This version brings in a few updates, including new array methods, a way to start scripts in various environments, and improvements that help avoid changing data by accident.
What is the difference between ES6 and ES7?
ES6, or ECMAScript 2015, was a big update that introduced many new features like classes, arrow functions, and new ways to declare variables with let and const. ES7, or ECMAScript 2016, was smaller and mainly added the async/await
feature for easier asynchronous programming. So, ES6 changed a lot about JavaScript, while ES7 added a specific helpful feature.