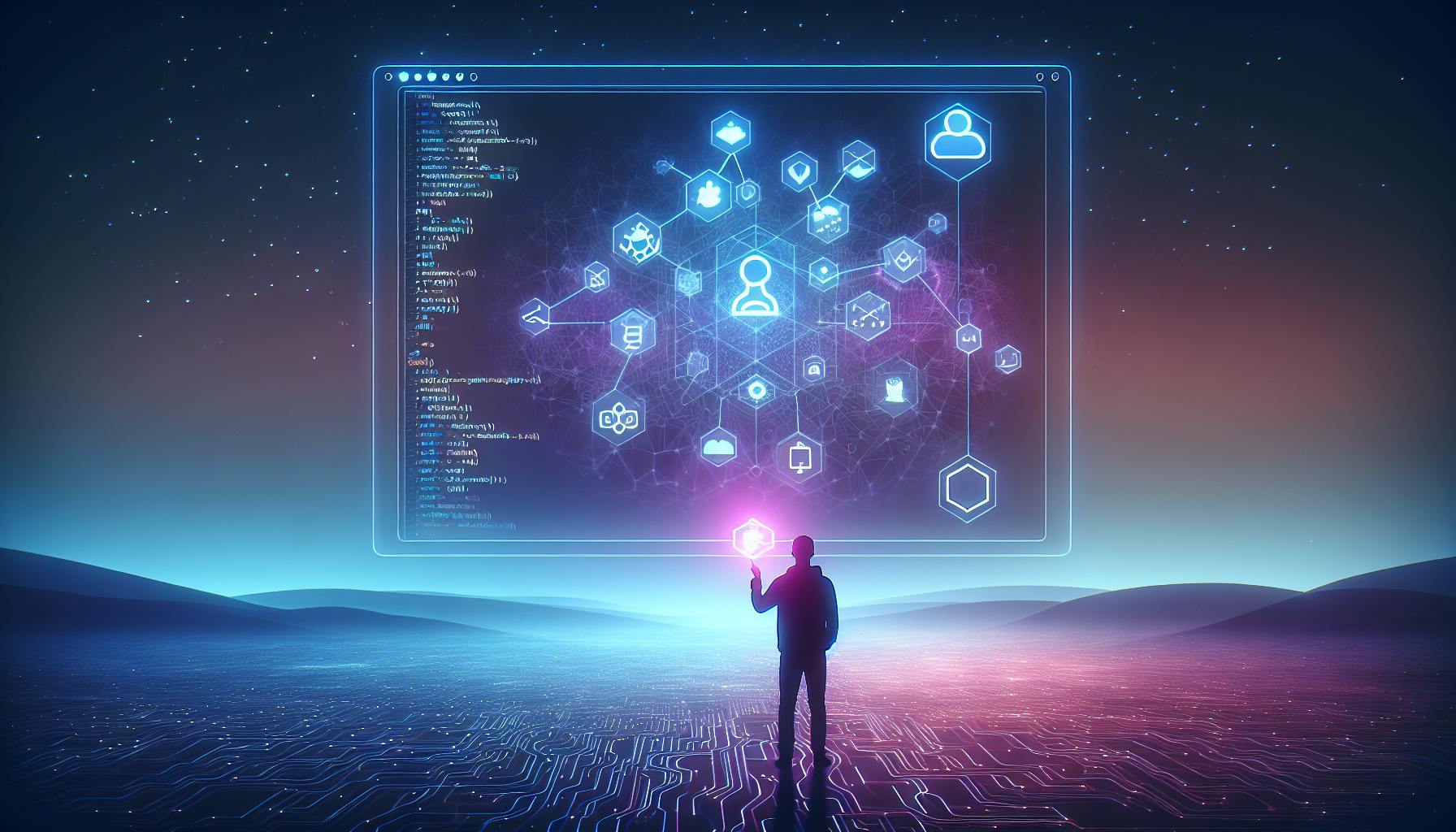
Learn the essentials of Node.js programming language, including asynchronous I/O, scalability, unified language use, rich ecosystem, and more.
Node.js is a powerful tool that lets developers use JavaScript for server-side programming, enabling the creation of efficient and scalable web applications. Here's what you need to know about Node.js in simple terms:
- Asynchronous I/O: Node.js handles tasks asynchronously, allowing for efficient multitasking without slowing down.
- Single-threaded but Scalable: It can manage thousands of simultaneous connections on a single thread.
- Unified Language: JavaScript is used on both the front and back end, simplifying development.
- Rich Ecosystem: Access to NPM, with over a million packages, aids in rapid project development.
Node.js excels in building applications that require handling numerous users or data simultaneously. It's favored for its efficiency, large community support, and the vast array of available tools and libraries. Whether you're setting up a simple web server or integrating with databases like MongoDB and MySQL, Node.js provides the foundation for building fast and scalable web applications. Plus, with a focus on best practices for performance, security, and scalability, developers can ensure their Node.js apps are robust and secure.
What is Node.js?
Node.js is a free tool that lets you run JavaScript on the server side, which means you can use JavaScript to make your website or app work. It started in 2009 by a guy named Ryan Dahl and works on the Chrome V8 engine. This engine lets you run JavaScript outside of your web browser.
Here are some quick points about Node.js:
- It lets you use JavaScript for both the stuff you see on a website (frontend) and the behind-the-scenes stuff (backend).
- Even though it does things one at a time (single-threaded), it's really good at handling lots of jobs at once without getting stuck. This is because it waits for nothing (non-blocking) and keeps things moving.
- Node.js has its own built-in stuff to let your app work like a web server. This means you don't need other software like Apache or Nginx to get your app online.
- It uses a system called CommonJS for organizing its code, and there are tons of extra tools and libraries you can get from the npm (Node Package Manager).
In simple words, Node.js lets you use JavaScript for more than just web pages. It's made for making websites and apps that can handle a lot of users at the same time.
Why Use Node.js?
Node.js is popular for a few good reasons:
- Fast and Can Handle Lots of Users - Its special way of dealing with jobs (event-driven, non-blocking) is great for serving a lot of users at the same time.
- Lots of Tools - There's a huge collection of extra tools and libraries in the npm, which can help you build things faster.
- One Language for Everything - Using JavaScript for both the front and back end makes things simpler and lets you reuse code.
- Easy to Learn - If you already know how to make websites with JavaScript, picking up Node.js is pretty straightforward.
- Big Community - A lot of people use Node.js, so it's easy to find help or hire someone who knows their stuff.
- Good for Asynchronous Programming - Its way of doing things at the same time without waiting around is perfect for making fast and efficient apps.
In short, Node.js is great for making fast, efficient websites and apps that can easily grow to serve more users. It's user-friendly, has a big community, and gives you access to a mountain of tools to help with your projects.
Setting Up Node.js
Installation Guide
Getting Node.js on your computer is simple. Just go to the official Node.js website and grab the Long Term Support (LTS) version that matches your computer's operating system.
On Windows:
- Pick the Windows installer (.msi file) for the most recent LTS version
- Open the installer and follow the steps it shows you, sticking with the standard options
- Restart your computer once the setup finishes
On macOS:
- Pick the macOS installer (.pkg file) for the most recent LTS version
- Double-click the file you downloaded and follow the on-screen instructions to install Node.js
- You might need to type in your admin password while installing
On Linux:
- For Linux users, it's best to use your distro's package manager to install the latest LTS version of Node.js
- For example,
sudo apt install nodejs
works for Debian/Ubuntu - And
sudo yum install nodejs
is for RHEL/CentOS
Verifying the Installation
To make sure Node.js is ready to go on your computer:
- Open a new terminal or command prompt
- Type
node -v
and hit enter to see the version of Node.js you installed - Type
npm -v
and hit enter to check the version of NPM you've got
If you see version numbers for both, you're all set. If not, you might need to check your system path or try installing Node.js again.
Node.js Basics
The Node.js Environment
Node.js works thanks to the V8 engine, which is the same thing Google uses to make Chrome run. This means Node.js can run JavaScript not just in your browser, but anywhere.
Here’s what you need to know about how Node.js works:
- Events and Callbacks - Instead of doing many things at once using different threads, Node.js waits for stuff to happen (events) and then knows what to do next (callbacks). This helps it manage lots of things happening at the same time without getting overwhelmed.
- Doesn’t Wait Around - Node.js can do other tasks while waiting for files to load or data to come through, thanks to its asynchronous, non-blocking nature.
- Keeps It Simple - Even though it uses just one thread to handle tasks, Node.js can still deal with a lot of requests. This is because it doesn’t get bogged down waiting for tasks to finish.
- Modules - Node.js lets you organize your code into chunks (modules) that you can reuse, making your code cleaner and easier to handle.
Node.js is really good at doing a lot of things quickly and efficiently, thanks to its unique way of handling tasks.
Key Parts of Node.js
These are some main pieces of Node.js:
- Event Loop - This is the heart of Node.js. It’s what allows Node.js to perform actions like reading files or responding to network requests one at a time, without stopping or waiting.
- Modules - These are like building blocks for your code. Node.js comes with some built-in ones, but you can also create your own or use others that people have made.
- npm Packages - Think of npm as a giant library of tools and code that you can borrow to build your Node.js projects faster and easier. With over 1.5 million options, it’s like having a toolbox that’s always growing.
- The Global Object - This is a special object in Node.js that has useful things built into it, like functions to delay actions (
setTimeout()
). - EventEmitter Class - This is used to make your own events happen and react to them. It’s a big part of making Node.js work the way it does.
Understanding these parts of Node.js will help you get how to make apps that are fast and can do a lot of things at once. It’s all about making your code work smarter, not harder.
Getting Started
First Node.js Program
To start with your first Node.js program, make a file named app.js
and open it in any text editor.
First, you need to bring in the http
module, which lets Node.js send and receive data over the web:
const http = require('http');
Then, create a web server using http.createServer()
. This needs a function that runs every time someone asks for something:
const server = http.createServer((req, res) => {
// handle the request
});
In this function, you can use request
and response
to deal with the web request.
For a simple start, let's send a "Hello World" message back:
res.write('Hello World!');
res.end();
To get the server going on port 3000:
server.listen(3000);
After saving the file, run node app.js
in your terminal. Your web server is now up on port 3000, saying "Hello World!" to anyone who asks.
For more complex apps, you might want to use more modules like fs
for working with files or get extra tools from npm.
Core Modules
Node.js comes with a bunch of built-in modules for things like networking, working with files, handling events, and more:
Http - Sets up a web server to handle web requests Fs - Lets you read and write files Path - Helps with managing file paths Os - Gives info about the operating system Events - Lets your code listen for and trigger events
To use one of these core modules:
const fs = require('fs');
These are just a few examples. You can find a complete list of built-in modules on the Node.js website.
You can also add third-party modules from npm in the same way.
Asynchronous Programming
Asynchronous Concepts
In Node.js, asynchronous programming is a big deal because it lets the program do many things at once without stopping or waiting around. Here's what you need to know:
- Event Loop - This is a smart system that lets Node.js do lots of tasks like reading files or getting data from the internet without getting stuck. It's like having a super-efficient assistant who can juggle many tasks at once.
- Non-Blocking - In Node.js, when you ask it to do something (like getting info from a database), it doesn't just sit there waiting for an answer. It moves on to other tasks and comes back to it once the answer is ready.
- Parallel Execution - Even though Node.js works on one thing at a time, it can handle many tasks in the background. This means your app can serve more people and do more things at the same time.
Understanding how to work with callbacks, promises, and async/await is key to making your Node.js app fast and responsive.
Callbacks, Promises and Async/Await
Here's how you can handle tasks that take some time in Node.js:
Callbacks
fs.readFile('/file.md', (err, data) => {
if (err) throw err;
console.log(data);
});
Callbacks are functions you give to Node.js to call back when it's done with a task. It's like saying, "Call me when you're done, and I'll decide what to do next."
Promises
readFilePromise('/file.md')
.then(data => {
console.log(data);
})
.catch(err => {
throw err;
});
Promises are like deals. You ask Node.js to do something, and it promises to let you know when it's done, either with your data or an error.
Async/Await
async function readFileAsync() {
try {
const data = await readFilePromise('/file.md');
console.log(data);
} catch (err) {
throw err;
}
}
readFileAsync();
Async/await is a way to write your code that makes it look like everything happens in order (synchronously), but it's still doing things in the background. It's like saying, "Wait here for this task to finish, and then move on."
Learning to use these three methods will help you write better, faster code in Node.js.
Building Web Applications
Introducing Express.js
Express.js is a tool that makes it easier to build web applications and APIs using Node.js. Here's what you should know about Express:
- It's free and open-source.
- It's built on top of Node.js, making it powerful.
- It's designed to be simple and flexible, so you can quickly build web apps and APIs.
- It supports middleware, which are like plugins or add-ons, to add more features like user login, setting up templates, and more.
- It makes setting up routes (like different pages or API endpoints) easy.
- It's great for all kinds of web projects, from small to big.
Basically, Express helps you build web apps quickly without having to start from scratch every time. It gives you tools and a structure to make strong web apps fast.
Routes, Middleware and Requests
Express deals with HTTP requests through:
Routes
Routes help match requests based on the path and the type of request (like GET or POST). For example:
app.get('/', (req, res) => {
// handle GET request for homepage
});
app.post('/contact', (req, res) => {
// handle POST request to contact page
})
Middleware
Middleware are functions that run during the request process. They can check or change the request and response. For example, to log requests:
app.use((req, res, next) => {
console.log(req.method, req.path);
next();
});
Request & Response
The request (req) and response (res) objects have all the details about the HTTP request and what should be sent back. For example, to use parameters:
app.get('/:id', (req, res) => {
let id = req.params.id;
})
Understanding how to use routes, middleware, and dealing with requests and responses is essential for making useful Express apps.
sbb-itb-bfaad5b
Working with Databases
MongoDB Integration
MongoDB is a type of database that stores data in a way that's easy to work with for applications. To use MongoDB in Node.js apps, we often use something called Mongoose, which helps organize and use our data.
Here's a simple guide to get started:
- First, add Mongoose to your project with
npm install mongoose
- Connect to your MongoDB database by giving it the address where your database lives
- Set up schemas, which are like blueprints for what data you want to keep track of
- Make models from those schemas, which let you work with the data easily
- Use methods like
.find()
,.create()
, and.update()
to work with the data - Add features like validation to make sure the data looks right
For example, to set up a user schema:
const mongoose = require('mongoose');
const userSchema = new mongoose.Schema({
name: String,
age: Number
});
const User = mongoose.model('User', userSchema);
With this setup, you can easily find, add, change, or remove users in your database. Mongoose makes it straightforward to include MongoDB into your app's logic.
MySQL Integration
To add a MySQL database to your Node.js app, you can use the mysql
package.
Here's how to do it:
- Install it with
npm install mysql
- Bring the
mysql
module into your app - Set up a connection with your database details
- Run SQL queries to get or change data
- Use special placeholders to keep your queries safe
- Close the connection when you're done
For example:
const mysql = require('mysql');
const conn = mysql.createConnection({
host: 'localhost',
user: 'root',
password: 'password',
database: 'mydb'
});
conn.query('SELECT * FROM users', (err, results) => {
// handle query results
});
conn.end();
The mysql
package also lets you:
- Manage multiple connections at once
- Use promises for easier coding
- Handle transactions for more complex operations
- Work with stored procedures
In short, the mysql
package is a simple way to work with SQL databases in your Node.js app, allowing you to easily manage your data.
Real-World Applications
Case Studies
Node.js is used by many big companies for their websites and apps that get a lot of visitors. Here are some examples:
PayPal uses Node.js for its website and handles a lot of money through it every year. They found that it made their developers work faster and made their website run better and handle more users.
Uber uses Node.js for important parts of its service, like tracking where drivers are in real-time. This helps them match drivers and riders quickly, even when there are a lot of them.
Netflix uses Node.js for parts of its website and streaming service. Things like searching for shows, controlling playback, and showing what you've watched use Node.js to work smoothly even when lots of people are using the service at the same time.
NASA uses Node.js for its website, nasas.gov. It helps them show updates about space exploration in a way that's interactive and works well even when lots of people visit the site.
LinkedIn uses Node.js for parts of its website and some tools they use inside the company. It helps with things like letting members know when they have new messages or updates, even with millions of users.
Best Practices
When making apps with Node.js, here are some good things to do:
- Performance - If you have tasks that need a lot of computing power, use worker threads so they don't slow everything else down. Use cluster mode to make the most of computers with more than one processor.
- Scalability - Use tools like Nginx to help your app handle more users. PM2 is a tool that can help run your app across several servers.
- Security - Protect your app from too many requests at once and make sure the data people send to your app is safe. Keep your app's code up to date to avoid security problems. Helmet is a tool that helps protect against common web attacks.
- Error Handling - Keep track of errors in one place. Make sure your app tells users what went wrong in a way they can understand. Use try/catch and promises to manage errors.
- Testing - Test your app to catch bugs and make sure it works well. Mocha and Jest are tools that can help with testing.
- Deployment - Use Docker to make it easier to set up and run your app on different computers. Use automated processes to update your app without manual work.
Following these tips will help make your Node.js app stable, safe, and able to handle lots of users.
Advanced Topics
Streams and Security
Streams are a way to handle big chunks of data in Node.js without having to load everything at once into your computer's memory. Here's a quick rundown:
Readable Streams
These are like a steady flow of data that you can read from whenever it's ready. Think of them like a stream of water you can dip into. Examples include getting data from a website (http request) or reading a file (fs read stream).
Writable Streams
These let you send out data bit by bit, sort of like putting letters into a mailbox. Examples include sending a reply to a website (http response) or writing to a file (fs write stream).
Pipelines
Pipelines connect readable streams to writable ones so data can flow smoothly from one to the other. It's like connecting hoses together to move water from a source to a destination. For instance, you can connect a stream that reads a file to a stream that compresses the file and then to a stream that writes the compressed file somewhere else.
Security
When building web apps with Node.js, keeping them safe is super important. Here are some tips:
- Use Helmet, a tool that helps set up protective barriers for your app
- Always check the data users send you to avoid harmful scripts (XSS attacks)
- When working with databases, use special placeholders to keep bad data out (prevent SQL injection)
- Limit how often someone can request data from your app to stop overload attacks (DDoS)
- Keep your app's code and tools up to date to protect against known problems
- Use secret codes (encryption) to keep sensitive information safe
In short, streams help you manage big data efficiently, and following good security practices keeps your app and its users safe.
Beyond the Basics
Resources for Further Learning
If you want to get even better at using Node.js, here are some great places to learn more:
- Books: Check out Node.js in Action and Node.js Design Patterns from O'Reilly for deep dives into building apps with Node. Another good one is Node.js in Practice by Manning, which talks about how to do things the right way.
- Online Courses: Websites like Coursera, Udemy, and LinkedIn Learning have lots of courses on Node.js. They cover everything from setting up web servers and working with databases to testing your programs and putting them online.
- Tutorials: Nodeschool.io has fun lessons you can do in your command line. Tutorialspoint and W3Schools have easy-to-follow guides on the basics of web development with Node.
- Documentation: The official Node.js website has all the details on how to use Node and its standard library. For more on tools like Express and Mongoose, their websites are super helpful.
Joining the Node.js Community
Being part of the Node.js community can help you learn a lot:
- The Node.js Foundation organizes events and groups for learning and working together.
- NodeSlackers is a cool place to chat about Node.js stuff.
- Discord Communities like the Node.js one are great for talking and getting help.
- The Node.js subreddit is a big community of Node developers sharing tips.
- Look for Node Meetup groups near you to meet other Node enthusiasts in person.
- Checking out GitHub issue boards for Node, Express, and other tools is a good way to see how people solve problems.
Getting involved with other Node users is a fantastic way to keep learning and to share what you know.
Conclusion
Node.js has really changed how we build websites by letting us use JavaScript for both the stuff you see and the behind-the-scenes parts. It's designed to handle lots of work at once without getting slow, which makes it great for websites that a lot of people visit at the same time.
In this guide, we talked about the basics of Node.js:
- Asynchronous programming - Node.js is really good at doing things at the same time, like talking to databases or updating things in real-time. Learning how to use callbacks, promises, and async/await is important for managing this.
- Building web apps - With tools like Express.js, you can quickly set up web servers and APIs. Knowing how to handle routes, middleware, and requests is key.
- Database integration - It's easy to connect to databases like MongoDB and MySQL with Node.js. This lets your app save and use data.
- Scalability - Node.js apps can grow to handle more visitors by spreading out the work across servers.
- Testing and deployment - Testing tools like Mocha help make sure your app works right. Using Docker makes it easier to get your app running on different computers.
- Security - Using tools like Helmet and following good practices helps protect your app from attacks and keeps user data safe.
Node.js has a big community and lots of resources to help you keep learning:
- Look at official documentation and guides for the main features and popular modules
- Take online courses to learn more about Node.js
- Join Node.js meetups or online groups to share ideas and get advice
With so many people using and improving Node.js, it's a strong choice for building fast, efficient websites using JavaScript from start to finish.
Related Questions
Is Node.js used for frontend or backend?
Node.js is super flexible and can be used for both making things look good on a website (frontend) and managing the behind-the-scenes stuff (backend). For the backend, it's great for creating quick and efficient server applications thanks to its ability to handle lots of tasks at once. Frameworks like Express.js help make it even easier to set up web services and APIs with Node.
For the frontend, Node.js comes in handy for managing the tools that help make websites. It works with tools like Gulp and webpack to make the process smoother. Plus, it can help run frontend frameworks like React by handling server-side templates. So, yes, Node.js can do a lot for both the frontend and backend.
Does node run on C++?
Indeed, Node.js uses the V8 JavaScript engine, which is written in C++. The V8 engine is what lets Node.js run JavaScript so well. While you write your apps in JavaScript, they're powered by this high-speed C++ engine.
Is Node.js required for VS code?
No, you don't need Node.js just to use Visual Studio Code. VS Code is ready to help you write JavaScript right out of the box. But, if you want to actually run Node.js apps, you'll need to have Node.js installed. VS Code is the place where you write your code, and Node.js is what runs it.
What should a Node.js developer know?
A Node.js developer should be good with:
- Basics of JavaScript and newer features
- How to handle tasks that run at the same time, like using promises or async/await
- Important Node.js parts like the fs and http modules
- Using Node.js frameworks, such as Express or Nest
- Making and working with web services
- Working with databases like MongoDB or MySQL
- Using caching with Redis
- Testing their code with tools like Mocha
- Figuring out problems in their apps
- Working with streams and buffers
- Basic skills for setting up and running apps on servers
- Making apps run faster and better
Knowing Node.js well, along with other web tech, makes a developer much more effective.