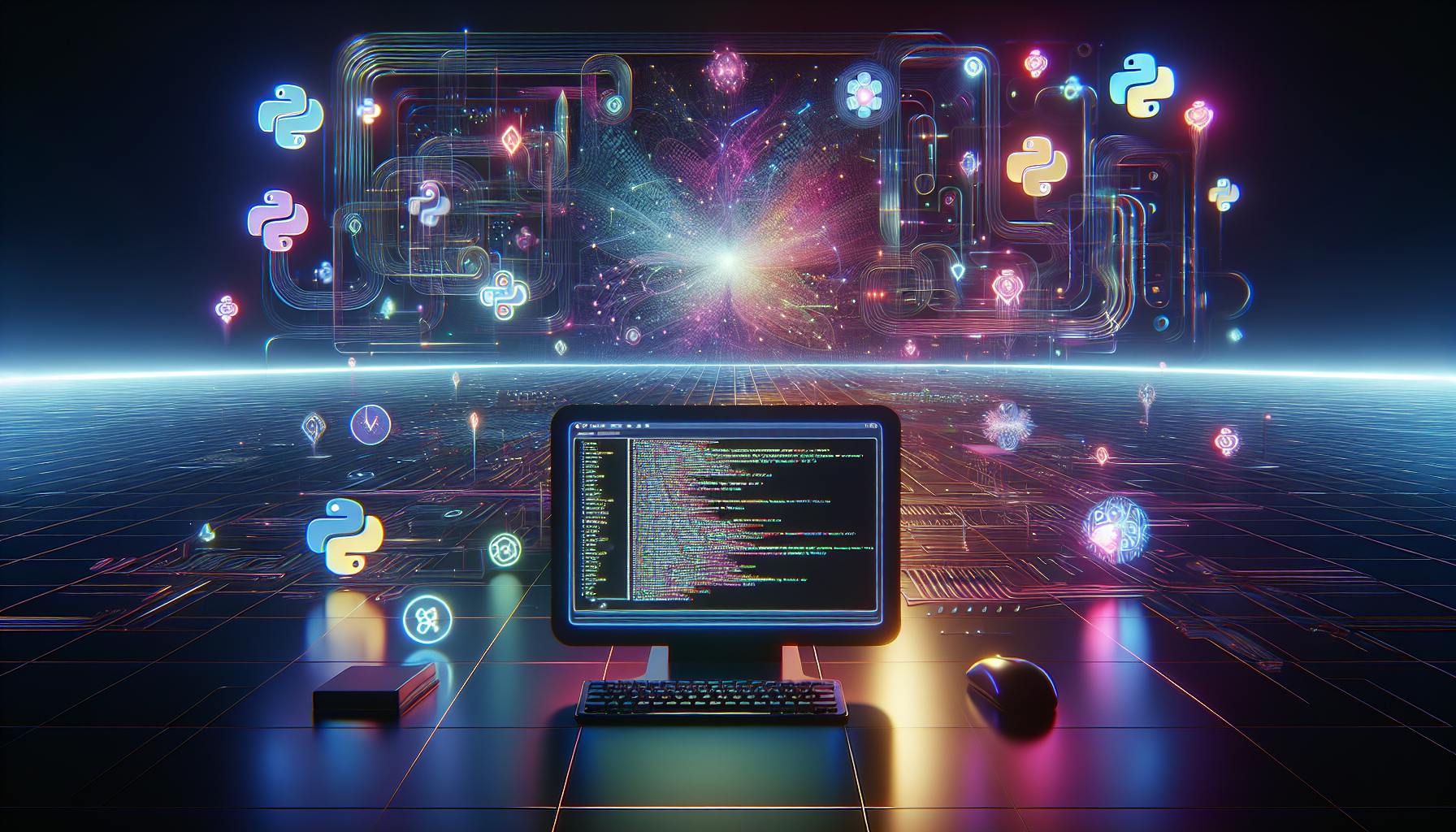
Learn about essential tips and tricks for using Pip, the package manager for Python developers. Discover how to manage dependencies, create virtual environments, and troubleshoot common issues.
Pip is a crucial tool for Python developers, enabling you to easily manage additional libraries or tools that aren't included in the default Python installation. Here's a quick overview of what you need to know about pip:
- Pre-installed with Python: If you're using Python version 3.4 or newer, pip is already included.
- Access to PyPI: Pip connects you to the Python Package Index (PyPI), offering over 200,000 packages.
- Simple Commands: Use commands like
pip install <package>
to add packages, and others to remove, update, or list them. - Requirements Management: Easily list all project dependencies in a single file for better management and sharing.
- Virtual Environments: Utilize virtual environments to keep packages for different projects separate and organized.
Whether you're working on large projects or handling multiple package dependencies, understanding and using pip effectively can significantly streamline your development process. This guide will walk you through pip essentials, from basic commands and managing dependencies to troubleshooting common issues.
Check pip Installation
First, let's see if pip is already on your computer. Open your command line or terminal and type:
pip --version
This command shows you the pip version you have, if it's installed. If you see an error like "pip command not found," then you need to install pip.
Install or Upgrade pip
If you don't have pip yet, here's how to get it:
- Go to https://bootstrap.pypa.io/get-pip.py and download the get-pip.py file.
- Open your command line, go to the folder where you saved get-pip.py
- Type and enter:
python get-pip.py
This installs pip and setuptools on your computer.
To update pip to the newest version:
pip install --upgrade pip
Basic pip Commands
Now, let's go over some simple pip commands to handle Python packages:
- Install Packages:
pip install <package-name>
e.g.pip install pandas
- Uninstall Packages:
pip uninstall <package-name>
- List Installed Packages:
pip list
- Show Package Details:
pip show <package-name>
- Upgrade Packages:
pip install --upgrade <package-name>
And that's the basic rundown on starting with pip! Next, we'll dive into more detailed ways to use it.
Working with Virtual Environments
Virtual Environment Necessity
Think of virtual environments like separate little boxes where you can keep the tools and libraries needed for different Python projects without mixing them up. Here’s why they’re super helpful:
- They stop different projects from stepping on each other's toes by using different versions of the same package.
- They keep your main Python area clean, so only the stuff you really want is there.
- You can pick a specific version of Python for each project.
- It’s easier to work with others on the same project because you can make sure everyone is using the same setup.
- They help make sure your project will work the same way on any computer.
Without these virtual boxes, managing all the extras for your Python projects can get really messy, especially if you’re juggling several at once.
Create and Activate a Virtual Environment
Here’s how to set up your own virtual box for a project:
- Open your terminal and go to your project’s folder.
- Type
python3 -m venv env
to make a new virtual environment calledenv
. - To start using this environment, on Linux or macOS type
source env/bin/activate
, or on Windows typeenv\Scripts\activate
. - You’ll know it’s working because you’ll see the environment name before your prompt.
Now, any Python packages you add with pip will stay in this environment.
Using pip in Virtual Environments
Inside your virtual environment, just use pip
to handle your packages. Here’s what to do:
- To add new packages, simply type
pip install <package>
. - To make a list of all the packages you’re using, type
pip freeze
and save it to a file named requirements.txt. - You can share this file with others, so they can set up their environment the same way by typing
pip install -r requirements.txt
.
Sticking to these steps makes sure that all the packages you use for a project are neatly stored in your virtual environment. This way, you won’t run into trouble with the wrong versions or mix-ups with other projects. It’s a smart move for any Python developer, especially when you’re working on bigger projects or anything involving data science, machine learning, or web development with tools like Flask, Django, or FastAPI.
Managing Dependencies
Details on how to use a special text file to keep track of all the extras your project needs.
Requirements Files Explained
Think of requirements files like a shopping list for your project. They are simple text files that list all the Python extras you need, including the specific versions. This way, you can make sure that anyone who works on the project uses the exact same stuff.
Here's why they're useful:
- You make one by running
pip freeze > requirements.txt
, which writes down everything you've installed. - The list looks like
numpy==1.21.5
, telling you the name and the exact version. - To set up the same environment, use
pip install -r requirements.txt
. - This helps keep everyone's setup the same, avoiding mix-ups.
- You decide which versions of which packages are used, keeping things stable.
- It's perfect for sharing what your project needs with others.
- You can keep this list with your project's code so it's easy to find.
Using a requirements file means you won't have trouble with missing or wrong versions of packages. It makes managing your project's needs straightforward.
Generating Requirements Files
Here's how to make a requirements file:
- Make sure you're working in the right virtual environment for your project.
- Install everything you need using
pip install
. - Run
pip freeze > requirements.txt
to write down what you've installed. - Now, your
requirements.txt
has a list of everything, ready for others to use.
Remember to update this file and share it, so everyone has the latest list.
Some tips:
- To focus on main packages, use
pip freeze --local > requirements.txt
. - Change the file yourself if you need to adjust or remove versions.
- For extra security, add hashes with
pip freeze --hash > requirements.txt
.
Update this file now and then to keep it current.
Installing from a Requirements File
To set up an environment using a requirements file:
- Activate the project's virtual environment.
- Use
pip install -r requirements.txt
to install everything listed.
This is a good step to follow whenever you need to get your project's setup just right.
Some extra steps you can take:
- To get the latest versions, add
--upgrade
when you install with the requirements file. - To install from a web link, just use
pip install -r https://example.com/requirements.txt
.
Using requirements files is a smart way to manage what your project needs, making sure everything works smoothly whether you're sharing your work, setting it up somewhere else, or just keeping your code organized.
Advanced pip Usage
Pip can do more than just install and manage packages. Some of its features are a bit more advanced but really handy to know.
Install from Source Control
Sometimes, you might want to install a package directly from where it's being developed, like GitHub. Here's how to do it:
- Find the GitHub page for the package, for example, for
pandas
it would be:
https://github.com/pandas-dev/pandas
- Then, use pip to install it like this:
pip install git+https://github.com/pandas-dev/pandas
This command tells pip to download the package from GitHub and install it. You can also specify a specific version or part of the code by adding more details to the URL:
pip install git+https://github.com/pandas-dev/pandas@v1.3.0
This is great when you need the very latest version of a package or a specific feature that's not in a release yet.
Using pip with Proxy Servers
If you're in a place that uses a proxy server (like many companies do), you'll need to tell pip about it to download packages. Here's how:
- Figure out your proxy server's details (URL and port).
- Set the
HTTP_PROXY
andHTTPS_PROXY
environment variables with those details. - Now, pip can use this proxy to reach the internet and download what you need.
If your proxy needs a username and password, you can set those up too with HTTP_PROXY_USER
and HTTP_PROXY_PASS
.
Version Pinning Dependencies
"Version pinning" means you decide exactly which versions of packages your project uses to avoid problems. Here are some tips:
- For important stuff, lock down the exact versions in
requirements.txt
. - When experimenting, you can be a bit more flexible with versions.
- Check your versions regularly to make sure they're still what you want.
- Consider using
Pipenv
. It's a tool that helps manage your project's package versions for you.
Sticking to specific versions can save you from headaches later by making sure your project doesn't break because of an unexpected package update.
Best Practices
Recommended steps for keeping your tools up to date and organizing your work.
Keep pip and Packages Updated
It's smart to regularly check that pip and any extra tools you've added to Python are the latest versions. Here's how:
-
Every so often, type
pip install --upgrade pip
to make sure pip itself is current. This helps you access the newest features and fixes. -
Use
pip list --outdated
now and then to see which of your added tools could be updated. This command shows you a list of updates you can make. -
To update everything at once, type
pip install --upgrade
. If you just want to update one thing, like pandas, you'd typepip install --upgrade pandas
. -
Think about setting up a system that checks and updates your tools automatically, like Dependabot.
Keeping everything current helps avoid problems and lets you use the latest improvements and security updates.
Separate Development and Production
It's a good idea to keep the tools you use for building and testing separate from what you use when your project is actually running for real users.
-
For developing, where you might want to try out new things, list these tools in a
dev-requirements.txt
file. -
For running your project (production), make sure to use specific versions of tools listed in a
prod-requirements.txt
file to avoid surprises.
This method helps keep things running smoothly by making sure changes in your development tools don't accidentally cause problems when your project is live.
Some tips:
- Keep both lists in your project files so everyone is on the same page.
- When building, install tools from both lists with:
pip install -r dev-requirements.txt -r prod-requirements.txt
. - For the live version, only use the
prod-requirements.txt
.
This keeps your project flexible during development and stable when it's live.
Manage Installed Packages
Pip lets you see what tools you've added and learn more about them with pip list
and pip show
.
Use pip list
to see everything you've added:
pandas 1.3.5
matplotlib 3.5.1
And pip show
gives you more info about a specific tool:
---
Name: pandas
Version: 1.3.5
Summary: Powerful data structures for data analysis
Home-page: https://pandas.pydata.org
Author: None
Author-email: pandas-dev@python.org
License: BSD-3
Location: /virtualenvs/myenv/lib/python3.8/site-packages
Requires: numpy, python-dateutil
Required-by:
This helps you:
- Know what you've installed and if there's a newer version.
- Understand how different tools depend on each other.
- Find out where to get more info or help.
Keeping track of your tools this way helps you stay organized and informed.
sbb-itb-bfaad5b
Alternatives to pip
While pip is great for adding packages to Python, there are other tools out there that can do more, especially for complex projects or when working with a team:
Poetry
Poetry is like a Swiss Army knife for Python projects. It helps you keep track of all your project's needs, from the packages it uses to how it's built and shared. Here's what it does:
- Uses a single file to manage dependencies, making things less cluttered
- Helps package and share your project with others
- Manages virtual environments for you, so you don't have to
- Lets you work with different versions of Python easily
Poetry is great for when your project starts to get a bit more complicated.
Pipenv
Pipenv combines the best parts of pip with an easier way to handle virtual environments:
- It sets up and manages a virtual environment automatically
- Uses a
Pipfile
for dependencies, which is clearer than arequirements.txt
file - Checks your packages for security issues
- Makes it easy to specify which version of Python and which packages you need
- Works well with pip for installing and managing packages
Pipenv is all about making your life easier by dealing with virtual environments and dependencies in a more straightforward way.
Conda
Conda is more focused on data science and comes with features that are really handy for data analysis, machine learning, and scientific computing:
- Has built-in support for data science packages like NumPy, pandas, and others
- Works with languages other than Python, like R and Java
- Makes it simple to manage complex packages and dependencies
- Helps you make sure your project works the same way on any computer
- Connects to a bigger ecosystem of data science tools
If you're doing a lot of work with data, Conda might be a better fit than pip.
So, pip is a good starting point for managing Python packages, but if you need more features or are working on specific types of projects, it's worth checking out Poetry, Pipenv, or Conda.
Troubleshooting Issues
Common pip problems and how to solve them.
Installation Errors
Fixes for pip install failures.
Sometimes pip doesn't work right:
- If Python or pip isn't found by your computer, make sure they're in your system's PATH. This is like telling your computer where to find Python and pip.
- On Windows, choosing "Add Python to PATH" during installation helps.
- If you can't install packages for everyone on your computer, try
pip install --user
to install just for you. - Sometimes, PyPI (where pip gets packages) might be down, or your internet might have trouble connecting. Check back later or try
--no-cache-dir
to avoid using old saved data. - If a package needs other packages that won't install, use
pip install --verbose
to see more about the problem. Try installing those needed packages by themselves first.
What you can do:
- Try installing Python again and make sure it's in your PATH
- Use Python's
ensurepip
to fix pip - Get
get-pip.py
from the internet to update pip
Dependency Conflicts
How to deal with packages that don't get along.
Sometimes, two packages need different versions of the same thing, causing errors.
To fix it:
- Use
pip check
to find the problem. - Look at
pip show pkgname
for more details on the packages. - Check your
requirements.txt
file.
Then, you can:
- Be more flexible with versions - Instead of saying you need one specific version, allow any version newer than a certain point like
numpy>=1.21.0
. - Choose which package is more important - Decide which one you need more and use the version it needs.
- Use different virtual environments - This keeps packages that don't get along separate.
- Update your packages - Sometimes newer versions work better together.
- Go back to an older version of one package that doesn't cause conflicts.
Tools like Poetry, Pipenv, or Conda might help with tricky situations.
The main idea is to figure out where the conflict is, decide which package you need more, and adjust the versions you're using. With some trial and error, you can usually find a solution.
Conclusion
Pip is a super handy tool for Python developers. It lets you easily add new features to your Python setup by downloading extra packages. Think of it as a way to quickly get new tools that make your coding projects better.
Here's what you should remember:
- Most people using Python can start using pip without installing anything extra. Just type something like
pip install
followed by the name of the package you want, and you're good to go. - Using virtual environments is a smart move. They keep your projects organized and prevent mix-ups between them. To set one up, use
python -m venv
and thensource env/bin/activate
to start it. - To make sure everyone can set up their project like yours, use
pip freeze > requirements.txt
. This creates a list of everything you've installed. - Keep an eye on what you've installed with
pip list
andpip show
. And don't forget to check for updates withpip list --outdated
. - If you're looking for more powerful tools, especially for big projects or data science and machine learning (like with pandas, NumPy, or PyTorch), you might want to check out Poetry, Pipenv, or Conda.
By using pip, you can easily add more power to your Python projects with just a few commands. Stick to good habits like managing your dependencies carefully, and you'll make your projects easy for others to pick up and work on, too.
Related Questions
What is the need of pip in Python?
Pip is like a handy tool that helps you add new features or libraries to Python, which aren't included from the start. It's like having an easy way to install new apps on your phone but for Python. With pip, you can quickly get and set up these extra pieces from a big online collection called the Python Package Index (PyPI).
Is pip bundled with Python?
Yes, pip comes with Python if you're using version 3.4 or newer. This means you probably already have pip if your Python version is relatively recent.
What packages do I need for Python?
For Python developers, especially those into Data Science, Machine Learning, or Data analysis, here are some must-have packages:
- NumPy: Helps with handling large data sets and doing complex math.
- Pandas: Makes it easier to clean and work with data.
- Matplotlib: Lets you create charts and graphs for data visualization.
- Scikit-Learn: Useful for applying machine learning algorithms like predicting trends or grouping data.
- TensorFlow: A toolkit for building and training neural networks, which are used in deep learning projects.
Should I use pip or pip3?
Use pip3
when you want to make sure you're installing packages for Python 3. While pip
and pip3
often do the same thing, pip3
specifically targets Python 3, ensuring you're managing packages for the right version of Python.