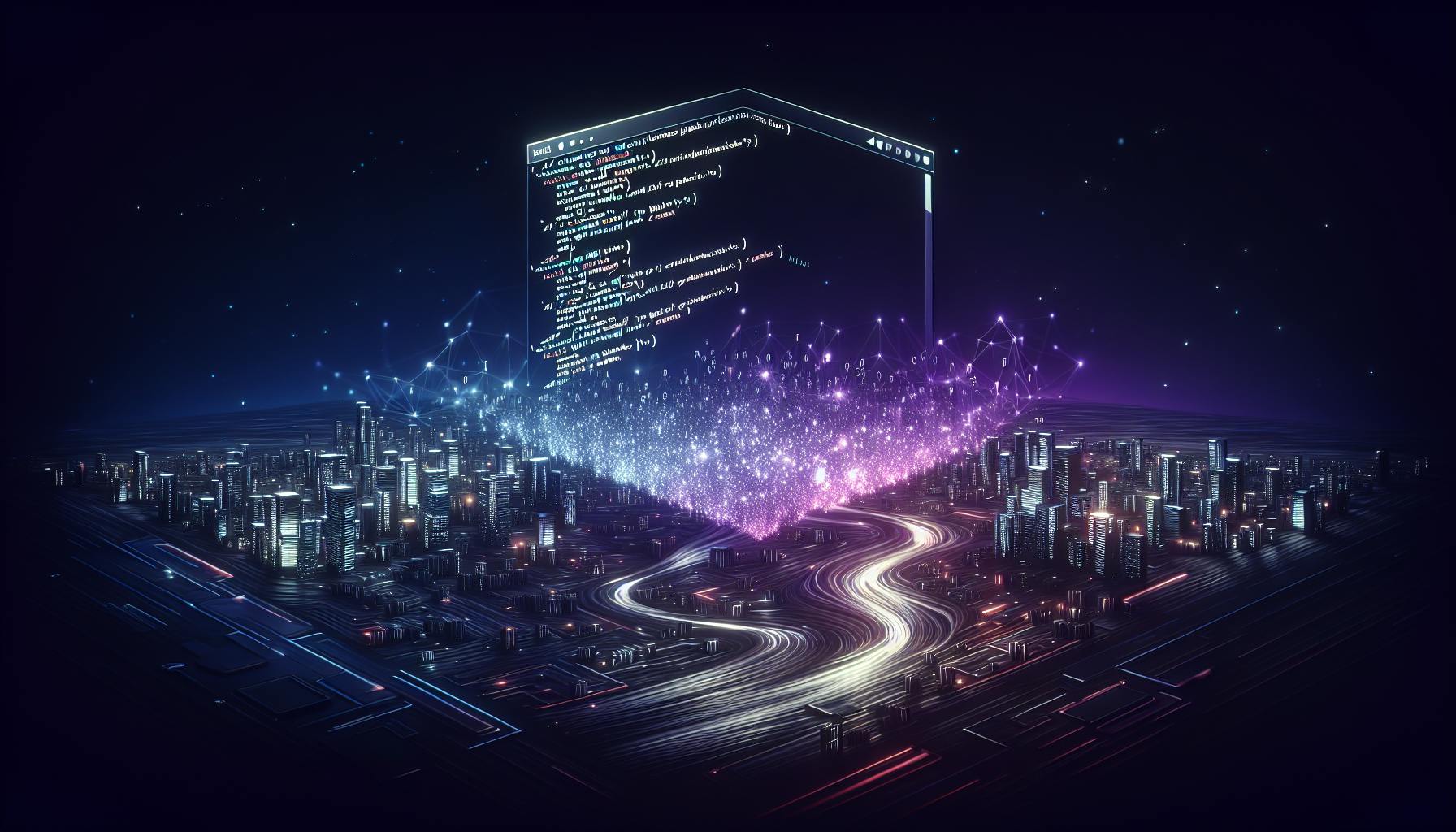
Learn the basics of npm, including package management, project setup, dependency management, and publishing packages. Get started with JavaScript development and Node Package Manager.
If you're new to web development or programming, understanding npm (Node Package Manager) is crucial. npm is a tool that comes with Node.js, allowing developers to share and use code from a vast library, automate tasks, and manage project dependencies efficiently. Here's a quick rundown of what you need to know:
- What npm is: It's a package manager for JavaScript, facilitating code sharing and reuse.
- Key Features: Simplifies project setup, manages dependencies, automates tasks, and connects you to a vast community of developers.
- Getting Started: Install Node.js (which includes npm), then use
npm init
to start a new project. - Managing Projects: Learn commands like
npm install
,npm update
, andnpm uninstall
to add, update, or remove packages. - Advanced Topics: Understand semantic versioning, the importance of the
package-lock.json
file, and how to audit your project for security vulnerabilities. - Publishing Packages: A step-by-step guide to sharing your code with the npm community.
This introduction aims to provide a clear and straightforward overview of npm, making it easier for you to begin your development projects with confidence.
What is npm?
npm is short for "Node Package Manager". It's a tool that lets JavaScript developers share their code and use code from others easily.
It has two big parts:
- The website (npmjs.com): This is where all the free JavaScript packages are stored. Think of it as a huge online library where you can borrow code for your projects.
- The CLI (command line interface): This is a tool that lets you install and manage these packages directly from your terminal. When you install Node.js, you get npm too.
With npm, you can grab code that does what you need, add it to your project, and save yourself a lot of time. It helps with things like:
- Keeping track of different versions of code
- Sharing your code with others, or using code they've shared
- Automating boring setup tasks
- Making sure your code works on different computers and versions of Node.js
- Finding useful tools among the vast amount of code others have written
In short, npm is a big toolbox and community for JavaScript projects, helping you to start, build, and share your work more efficiently.
The Importance of npm in Modern Web Development
JavaScript is super popular, and npm is a key tool for anyone working with it, whether on websites or servers. Here's why npm matters:
Simplified Project Setup: Starting a project with npm init
helps you organize all your project's details, like what code it needs and how it should run, in one file (package.json
). This makes it easier for others to understand your project.
Code Reuse: With npm, you can use pieces of code that others have made for common tasks, saving you from having to write everything yourself.
Managing Dependencies: npm takes care of keeping track of all the different pieces of code your project needs and makes sure everyone working on the project uses the same versions.
Scripting Builds: You can use npm to tell your project how to build itself automatically, using tools like Webpack.
Staying Updated: npm helps you keep your code up to date without breaking your project, thanks to a system called semantic versioning.
Collaboration: npm connects you to a huge community of developers. You can use what they've built and share your own work too.
Basically, npm makes working on JavaScript projects a lot easier and helps everyone stay on the same page.
Chapter 2: Setting Up npm
Installing Node.js and npm
First things first, to use npm, you need Node.js on your computer because npm comes with it. Here's what to do:
-
Visit nodejs.org and download the LTS version of Node.js for your computer.
-
Run the downloaded file and follow the steps it shows you.
-
To check if Node.js is installed, open your terminal or command prompt and type
node -v
. You should see something like this:node -v v16.14.0
-
Now, make sure npm is installed by typing
npm -v
:npm -v 8.3.1
If you see version numbers, you're all set with Node.js and npm!
Updating npm
Keeping npm up to date lets you use new features and packages. Here's how to update it:
- In your terminal or command prompt, type:
npm install -g npm
- This command updates npm to the latest version for all users on your computer.
- Check if the update worked by typing
npm -v
again:
npm -v
8.5.0
Now, you have the latest version of npm. Remember to update Node.js and npm now and then to keep things running smoothly.
Chapter 3: npm Basics
Initializing a New Project with npm init
Starting a new Node.js project is easy with the npm init
command. It sets up your project and creates a package.json
file, which keeps track of all the important details about your project. Here’s how to do it:
- Open your terminal and go to the folder where you want your project to be.
- Type
npm init
and press enter. - npm will ask you a bunch of questions to help set up your project, like what to call it, what version it is, and a short description. You can answer these or just press enter to skip.
- If you want to skip all the questions, you can type
npm init -y
instead. This fills in default answers for you, which you can change later by editing thepackage.json
file.
Once you’re done, you’ll have a package.json
file in your project folder with all your answers.
Understanding the package.json
File
The package.json
file is like the brain of your Node.js project. It’s a list in JSON format that keeps track of your project's details.
Here’s a quick look at what’s inside:
- name - Your project's name.
- version - How new your project is.
- description - A brief about what your project does.
- main - The first file that runs in your app.
- scripts - Shortcuts for tasks like running tests or starting your app.
- keywords - Words that help people find your project.
- author - Who made the project.
- license - The rules for using your project's code.
- dependencies - Other code your project needs to work.
- devDependencies - Extra tools for when you’re working on the project.
This file is super important because it tells others about your project and what it needs to run.
Managing Dependencies
Dependencies are bits of code from other people that your project needs to work. npm makes it easy to handle these.
There are two main kinds:
1. dependencies
These are essential for your app to run properly. For example:
"dependencies": {
"express": "^4.17.1"
}
2. devDependencies
These are only needed when you’re working on the project, not when it’s actually running. For example:
"devDependencies": {
"jest": "^26.6.3"
}
To add a dependency:
npm install express
This command downloads the package and adds it to your package.json
.
To upgrade a dependency:
npm update express
This updates the package to the latest version that’s allowed.
To remove a dependency:
npm uninstall express
This takes the package out of your project and updates the package.json
.
Keeping your dependencies up-to-date and well-managed helps your project run smoothly and makes it easier for others to work with.
Chapter 4: Working with npm
Essential npm Commands
Here are some basic npm commands you'll find yourself using a lot:
npm install
: This command adds a new package to your project. For instance, if you want to add a package called express, you'd type:
npm install express
npm update
: This one updates all your project's packages to their newest versions, as long as those versions match what you've said is okay in yourpackage.json
.
npm update
npm uninstall
: Use this command to get rid of a package you no longer need.
npm uninstall express
npm run
: This lets you run specific tasks set up in yourpackage.json
, like testing your code.
npm run test
Installing Packages
When it comes to adding packages, you have a few options:
- Locally: This installs packages in a folder called
node_modules
right in your project. It's the standard way to add something:
npm install lodash
- Globally: Adding
-g
installs packages so that your whole system can use them, not just one project:
npm install -g nodemon
- You can also pick a specific version of a package if you need to:
npm install lodash@4.17.21
npm Scripts
npm scripts are shortcuts you set up in your package.json
to do common tasks, like running your app or testing your code. Here's how to set them up:
"scripts": {
"start": "node index.js",
"test": "jest"
}
After you've added them, you can run these tasks easily from the terminal:
npm run test
npm start
This makes it quicker to do things without having to type out long commands.
sbb-itb-bfaad5b
Chapter 5: Advanced Topics
Semantic Versioning
Think of semantic versioning like a rule book for numbering software updates. It's set up like this:
major.minor.patch
- Major versions are big changes that might break things.
- Minor versions add new stuff but don't mess with what's already there.
- Patches are for fixing bugs without adding new features.
For instance, moving from version 1.2.3 to 1.3.0 could cause issues, but going from 1.2.3 to 1.2.4 is generally safe.
In your package.json
, you can set rules to automatically update to new versions:
"dependencies": {
"express": "^1.2.3"
}
The ^
symbol means your project can automatically update to new minor and patch versions. This keeps your project up-to-date with bug fixes without breaking your code.
Using semantic versioning means everyone can update their projects safely, without worrying about unexpected issues.
The package-lock.json File
When you install packages, npm creates a package-lock.json
file. This file lists the exact versions of packages your project is using. Why is this important?
- Consistency: It makes sure everyone working on the project uses the same versions of packages, which helps prevent bugs.
- Security: If there's a security issue in a package, you can quickly check if your project is affected.
- Speed: npm uses this file to install only what's missing, making things faster.
You should always keep package-lock.json
with your project files and share it with your team, but don't change it yourself. Let npm handle that.
Security and Auditing
Since npm packages come from various sources, it's possible to run into security issues. You can use the npm audit
command to check your project for known security problems. It:
- Looks for security issues in your packages
- Gives you a report of what it finds
- Offers ways to update packages to safer versions
Just type:
npm audit
It's a good idea to run this check now and then to make sure your project is as secure as possible.
Chapter 6: Publishing Your First Package
Step-by-Step Guide
Sharing your code with others through npm is a great way to contribute to the community and improve your skills. Here's a simple guide to get your npm package out there:
-
Create a Node.js module
- Start a new Node.js project and write your code in an
index.js
file. This file should include functions you want to share. - Ensure your code can be imported and used in other projects as a module.
- Start a new Node.js project and write your code in an
-
Package setup
- Use
npm init
to make apackage.json
file, which holds details about your project. - Make sure the
main
field points to the file that starts your module. - Fill in other important info like what your project does.
- Use
-
Version control
- Start a Git repository to keep track of your code changes.
- Stick to semantic versioning for your versions, starting with "1.0.0".
-
Testing
- Create tests to make sure your code does what it's supposed to.
- Test that your module behaves correctly when published locally.
-
Publish
- Sign up for an npm account if you don't already have one.
- From your module's folder, run
npm publish
. - Verify that your package can be installed in another project.
-
Maintenance
- Release new versions with semantic versioning.
- Keep your project details up to date.
- Help users by fixing issues they report.
Following these steps will help you put out a solid npm package.
npm Organizations
npm Organizations are tools for teams to work together on packages more easily:
Teaming and access control
- You can add team members and set who can do what.
- Give people specific access to certain packages.
Visibility
- Group your packages under one web address linked to your org.
- Show off your team's public packages in one spot.
Billing
- All your npm costs are in one bill.
- Makes it easier to keep track of what you're spending on npm.
In short, npm Orgs help teams work together better, let you show off your work, and simplify handling your npm expenses.
Conclusion
npm is super helpful for people who build websites or apps with JavaScript. It's like a big toolbox that lets you grab pieces of code made by others, saving you a ton of time and effort.
Here's what to remember:
- When you install Node.js, you also get npm. This means you're ready to use all the cool tools npm offers right from the start.
- Starting a project with
npm init
makes apackage.json
file. This file keeps track of all the bits and pieces your project needs to work. - With npm, you can easily add, update, or remove packages. This helps you keep your project fresh and working well with others.
- Commands like
install
,update
,uninstall
, andrun
are your best friends. They let you manage packages and run tasks without a headache. - npm scripts are handy shortcuts for doing regular stuff, like starting your app or checking your code.
- Semantic versioning is a smart way to know what changes come with new versions of packages.
- Sharing your code by publishing it to npm is a great way to help out other developers.
Knowing the basics of npm sets you up for success in building JavaScript stuff. There's a lot more to learn, so when you're ready, dive deeper into npm's extra features like NPX, managing the NPM cache, and using npm for team projects. The npm docs are a great place to start. Have fun coding!
Related Questions
How do I get started with npm?
To begin using npm, you need to create an account on the npm website. Pick a strong password and turn on two-factor authentication for extra security. You'll get a one-time password via email to confirm your account.
After setting up your account, download and install Node.js, which includes npm. You can then start new projects with npm init
, add packages with npm install <package>
, and dive into building. Consider setting up a .npmrc
file to make npm work better for you.
Why do developers use npm?
Developers turn to npm to share and manage code easily. It lets you use packages that other people have made instead of writing all the code yourself. npm takes care of which versions of packages your project needs, making it easier to work on big projects with lots of people.
How to create a new project using npm?
To start a new Node.js project with npm, make a new folder for your project and open it in your command line tool.
Run npm init
to get started. This creates a package.json
file where your project's settings are stored. You can also use npm init -y
to skip the questions and fill in default settings.
Next, install any packages you need with npm install <package>
. You can add them to your package.json
as either main or development-only packages.
You can also add scripts to your package.json
, like a start command, to make certain tasks easier to run.
Now, with your package.json
ready, your project is set up!
How to build code using npm?
To build a Node.js package with npm, follow these steps:
- Use Git to keep track of your code changes.
- Run
npm init
to create apackage.json
with your project's details. - Add your code files, like an
index.js
. - In
package.json
, set the"main"
field to point to your main file. - Add build scripts in
package.json
to compile your code. - Use semantic versioning to version your package.
- Write a README with instructions on how to use your package.
- Test your package, then publish it with
npm publish
.
Now, other developers can install your package with npm install <package>
and use it in their projects.