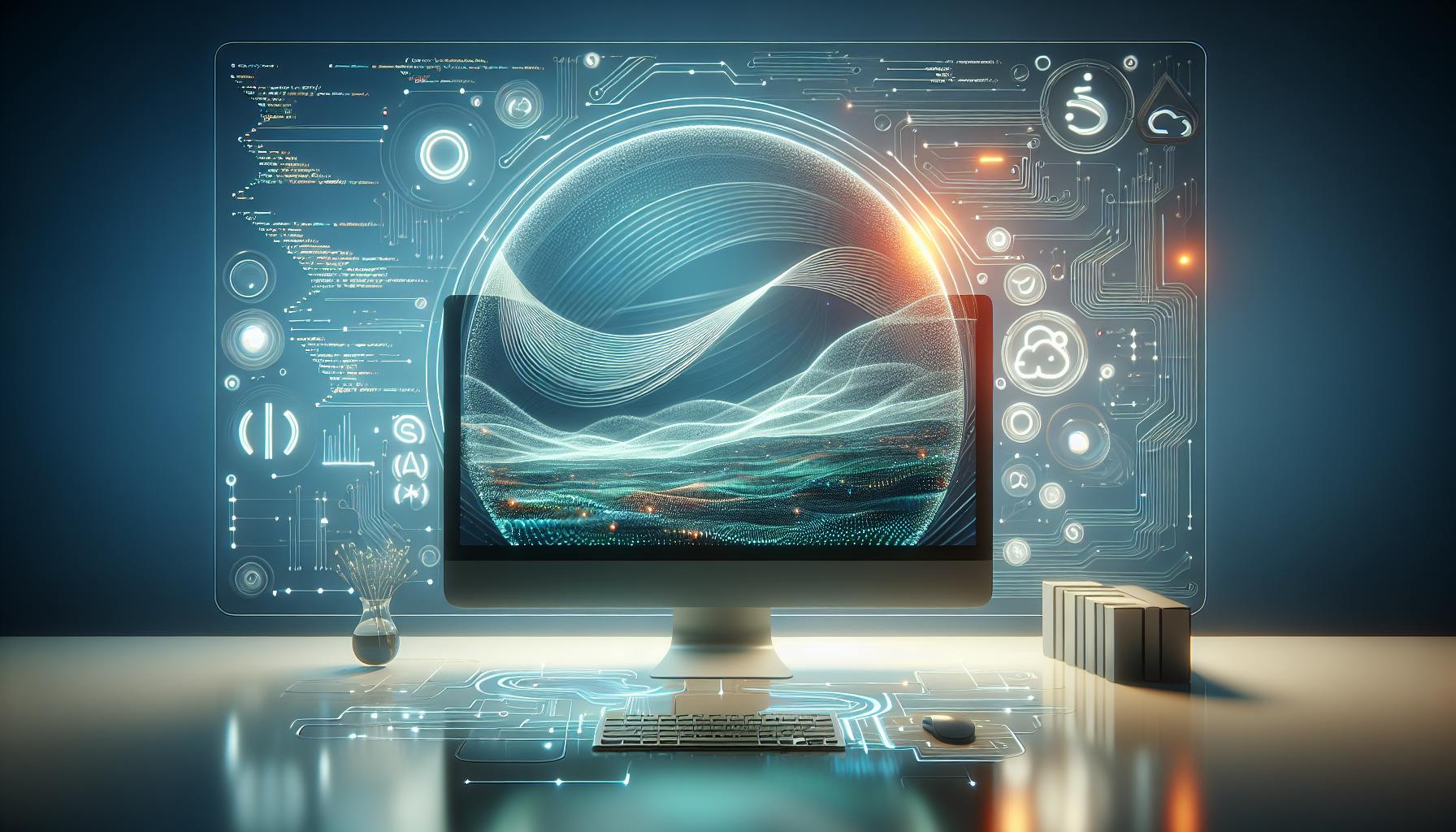
Learn the basics of Quarkus, a modern Java framework optimized for cloud environments. Get started with setup, creating your first app, and understanding its architecture and best practices.
Quarkus is a modern Java framework designed to optimize Java applications for cloud environments, making them faster and more efficient. It combines the best of Java technologies for a seamless development experience, especially in Kubernetes. Here's what you need to know about getting started with Quarkus:
- What Quarkus Is: A tool for making Java cloud-friendly, focusing on fast startup times and low memory usage.
- Setting Up: You'll need JDK 17+, an IDE, Apache Maven 3.9.6+, and optionally, the Quarkus CLI.
- Creating Your First App: Use the Quarkus CLI or Maven to initialize a new project.
- Live Coding: Quarkus allows for real-time coding changes without restarting your app.
- Architecture: Built on Vert.x and Eclipse MicroProfile, optimized at build time for efficiency.
- Extensions: Enhance your app with add-ons for databases, security, and more.
- Best Practices: Includes leveraging live coding and avoiding blocking calls.
Dive deeper into Quarkus with tutorials, documentation, and joining the community for support and discussions.
History of Quarkus
Quarkus was created by a group of engineers at Red Hat. They wanted to make Java better for the cloud by bringing together the best parts of several Java technologies, like Vert.x and Hibernate, into one package that's really good for working with Kubernetes.
Key Benefits of Using Quarkus
Quarkus helps Java applications start faster and use less memory, which is a big deal for running lots of applications efficiently. It also makes life easier for developers with features that let you see changes in real-time and work easily with containers.
Quarkus in Modern Software Development
Quarkus fits well with today's way of building software, which often involves breaking applications into smaller, independent pieces that can scale up or down easily. With its focus on cloud environments and support for both traditional and modern Java, Quarkus is a good choice for these kinds of projects.
Setting Up Your Quarkus Development Environment
Prerequisites
Before you dive into Quarkus, you'll need a few things:
- JDK 17+ - Quarkus works with Java 17 or newer. Make sure you have the latest JDK installed and set up the
JAVA_HOME
environment variable correctly. - IDE - You'll want an Integrated Development Environment like Visual Studio Code, Eclipse, or IntelliJ IDEA for writing and managing your code.
- Apache Maven 3.9.6+ - Maven helps build and manage your Quarkus projects. Check that you have the latest version.
- Quarkus CLI (optional) - This tool makes it easier to start new projects and do other tasks. We'll show you how to get it below.
Make sure Maven is using the right version of JDK by typing mvn --version
in your command line and checking the output.
Installing Quarkus
The Quarkus CLI is a handy tool for creating projects, adding stuff, and running your apps. Here’s how to get it:
- Linux/macOS - Open your terminal and type these commands to install the CLI using JBang:
curl -Ls https://sh.jbang.dev | bash -s - trust add https://repo1.maven.org/maven2/io/quarkus/quarkus-cli/
curl -Ls https://sh.jbang.dev | bash -s - app install --fresh --force quarkus@quarkusio
- Windows Powershell - Use these commands instead:
iex "& { $(iwr https://ps.jbang.dev) } trust add https://repo1.maven.org/maven2/io/quarkus/quarkus-cli/"
iex "& { $(iwr https://ps.jbang.dev) } app install --fresh --force quarkus@quarkusio"
To check if the CLI is ready, type quarkus --version
. You should see the version number. Now, you're all set to start creating Quarkus projects and exploring what it can do.
Your First Quarkus Application
Project Initialization
To start with Quarkus, you'll want to set up a new project. You can do this in two main ways:
- If you're using the Quarkus command-line tool, type this:
quarkus create app io.mygroup:my-first-app:1.0-SNAPSHOT
- Or, if you prefer Maven, use this command:
mvn io.quarkus.platform:quarkus-maven-plugin:2.16.3.Final:create -DprojectGroupId=io.mygroup -DprojectArtifactId=my-first-app -DprojectVersion=1.0-SNAPSHOT
This step creates a basic structure for your Quarkus app, including:
- pom.xml - This file helps manage your project's tools and libraries.
- src/main - Where your Java code lives.
- src/test - For testing your app.
- application.properties - For setting up your app's configurations.
- GreetingResource.java - A simple web page that says hello.
Now, you're ready to dive into coding with Quarkus!
Building and Running
To put your app together, just run:
./mvnw package
This creates a JAR file in target
that you can run. To start your app and see it in action, type:
./mvnw quarkus:dev
This special mode lets you make changes and see them right away.
Try out your web page by running:
curl http://localhost:8080/hello
You should see a friendly hello message!
Live Coding with Quarkus
One of the cool things about Quarkus is how you can change your code and see the results instantly, without restarting your app. This is great for when you're working on your app and want to see how your changes look right away.
In this mode, Quarkus:
- Quickly updates your code as you work.
- Restarts your app automatically if needed.
- Checks your changes and updates tests on the fly.
This means you can keep coding and testing without slowing down, making it easier to get your work done. It's especially handy for fixing bugs or adding new features like REST services.
sbb-itb-bfaad5b
Understanding Quarkus Architecture
Quarkus has a smart design that makes Java apps run better in places like Kubernetes. Let's dive into some important parts of how it's built.
Internal Architecture
At its heart, Quarkus uses some cool tools to manage the basics like putting pieces together and setting things up. It uses Vert.x to talk between parts of your app really quickly and adds in Eclipse MicroProfile to handle bigger, enterprise-level tasks.
When your Quarkus app starts, it only loads what it absolutely needs, connecting pieces that were figured out when the app was being built. This is different from older Java setups that took longer to start because they had to find and connect everything each time.
Extensions help Quarkus do more things, like working with databases or securing your app. They hook into Quarkus using special code markers, building steps, and other tricks. You'll find extensions for lots of needs, including SmallRye for MicroProfile stuff and Hibernate ORM for databases.
Build Time Optimizations
One cool thing about Quarkus is that it does a lot of prep work when you're building your app, not when you're running it. This means:
- It checks your app's code and figures out what parts it really needs.
- Connects everything together and sets up how your app will run.
- Gets your app ready to run as a super-fast native executable.
- Makes your app run faster and use less memory when it starts.
Doing this work early helps your app start up super quick and only use what it needs.
The Extension Model
Extensions are like add-ons that let Quarkus do even more stuff. They can add features for almost anything you need in your app, from talking to databases to sending messages.
Extensions work by:
- JVM services - They can add basic services like setting things up or securing your app.
- Code annotations - You mark your code, and Quarkus takes care of it.
- Build steps - They can add special steps when your app is being built.
- Native configurators - They help make your app ready to run as a native executable.
- Code starts - They can run setup code before your app even starts.
With extensions, you just pick what you need, and Quarkus makes them work together smoothly.
Best Practices for Quarkus Development
Leveraging Live Coding
Live coding lets you see changes to your code right away without stopping and restarting your app. Here are some tips to get the most out of it:
- When working on the look of your app, use live coding to see updates in your browser instantly.
- Keep coding while Quarkus updates your app in the background.
- Open a separate window to watch the logs while you code.
- Write tests that run automatically when you change the code to make sure everything still works.
- Turn off live coding when checking how fast your app runs to get a real sense of its speed.
Avoiding Blocking Calls
If your app waits around for things to happen when it starts, it can slow down. Here's how to avoid that:
- Wait to set things up until you really need them, so your app doesn't get stuck waiting.
- Move tasks that take a lot of time or need to wait for something (like getting data) to separate processes that can run in the background.
- Use Panache and Hibernate in a way that lets your database talk without stopping other things from happening.
- When checking if your app is running well, make sure it doesn't slow down because it's waiting for these checks.
Testing Considerations
- Quick tests check small parts, but testing the whole app together makes sure everything works well together. Use both kinds.
- Tests that check the whole app help you find problems with how your app talks to the internet or uses the database.
- Set up your app so it automatically tests new code as you write it.
- Use fake versions of outside services to test parts of your app by themselves.
- Use tools like testcontainers to create real conditions for testing how your app works with other services.
Next Steps
Expanding Knowledge
Now that you've got the basics down, it's time to dive deeper into what Quarkus can do. Here are some ways to build on what you've learned:
- Hands-on Tutorials - Check out the Quarkus website for tutorials that guide you through creating actual applications, showing you how to work with REST APIs, make your apps work well with Kubernetes, and more. It's a great way to learn by doing.
- Reference Documentation - For the nitty-gritty details on everything Quarkus, the user guide and API documentation have you covered with technical info and examples.
- Interactive Learning - Try out the Quarkus Workshop for hands-on labs that let you practice new skills in a controlled environment.
- Talks and Presentations - Watch talks and presentations by people who use Quarkus in real projects. They share useful tips, how they structure their apps, and lessons from using Quarkus in production.
Joining the Community
Getting involved with the Quarkus community can really speed up your learning:
- GitHub Discussions - The Quarkus Discussions forum is a place to chat with others using Quarkus. It's a good spot for asking questions and sharing what you know.
- Quarkus Chat - Join the Quarkus Zulip chat for live discussions on different topics with the community.
- Reporting Issues - If you find a bug or have an idea to make Quarkus better, you can tell the developers about it on GitHub.
- Contributing - Since Quarkus is open source, you can also help out by contributing code, fixing bugs, or improving the documentation. Check out how to contribute here.
Jumping into the Quarkus community is a great way to get better at using Quarkus and to keep up with the latest in app development.