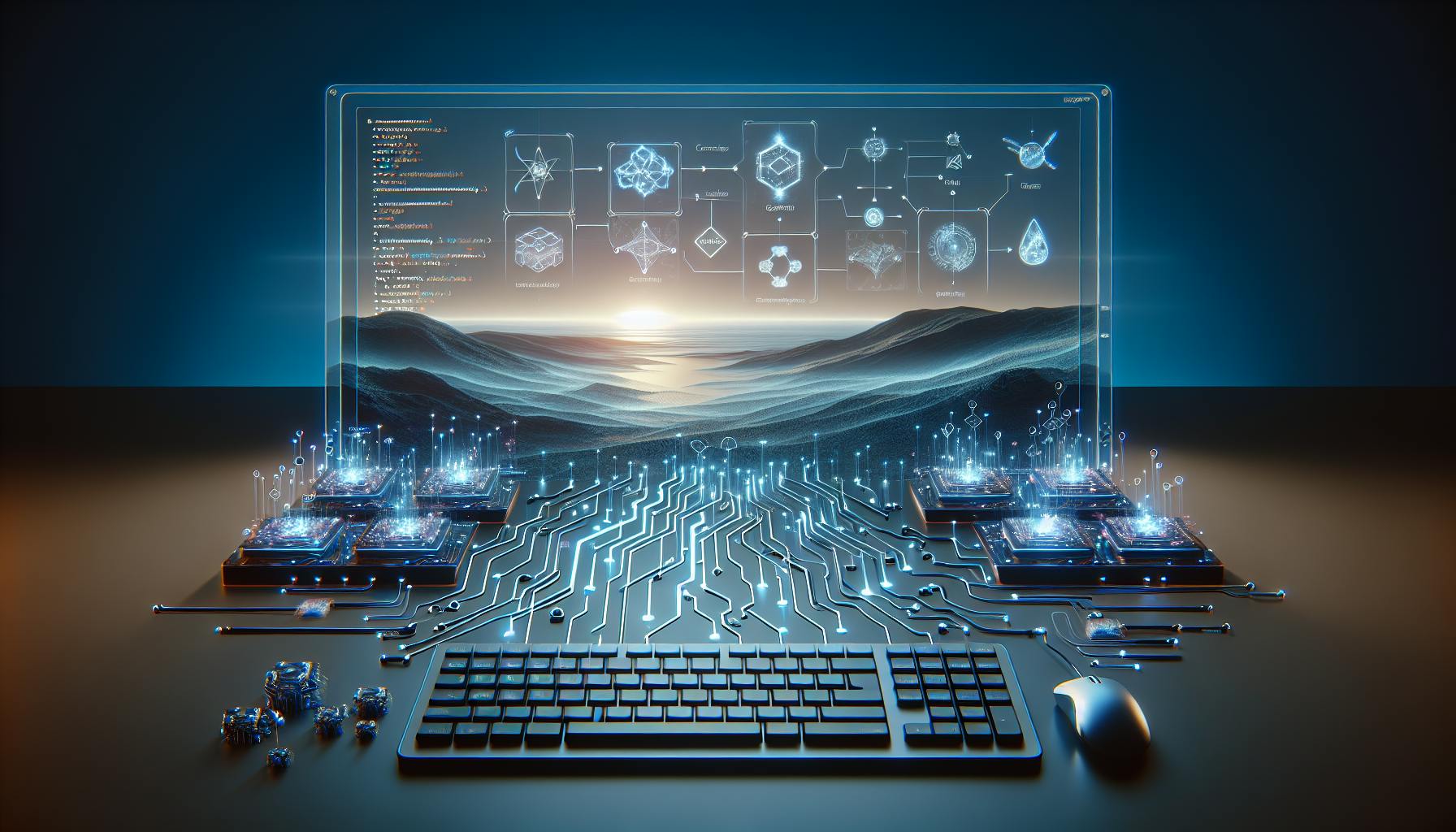
A guide to choosing the right React unit test framework, including Jest, React Testing Library, Enzyme, and Mocha. Learn why unit testing is important in React, how to test components, key tools for testing, and best practices.
Choosing the right React unit test framework is crucial for ensuring each component of your app works perfectly on its own. Here's a quick guide to help you decide:
- Jest: Easy setup, great for beginners, and comes with automatic features for React apps.
- React Testing Library: Focuses on testing components as users would interact with them, promoting more meaningful tests.
- Enzyme: Offers detailed ways to examine and interact with components, allowing for deeper testing.
- Mocha: Highly customizable, good for specific testing needs but requires more setup.
Quick Comparison
Framework | Ease of Setup | Focus | Customizability |
---|---|---|---|
Jest | Easy | Automatic features for React | Low |
React Testing Library | Easy | User-centric component testing | Medium |
Enzyme | Moderate | Detailed component interaction | High |
Mocha | Moderate to High | Custom testing setups | Very High |
Each framework has its pros and cons, but the best choice depends on your project's needs and your testing preferences. Whether you're just starting out with Jest or need the detailed control that Enzyme and Mocha offer, understanding these essentials can lead to better and more reliable apps.
Why Unit Test React Components
Here's why unit testing is a big deal in React:
- Prevent bugs - Testing parts one by one helps find and fix mistakes before they cause trouble in the full app.
- Facilitate changes - With tests in place, you can change stuff without fear. If something goes wrong, your tests will tell you.
- Simplify integration - When each part works well on its own, putting them all together is much smoother.
- Document functionality - Tests explain how parts should work, making it easier for others to understand your code.
How to Unit Test React Components
Here's a simple way to do unit testing with Jest:
- Render the component - Use tools like
render
from@testing-library/react
to show your component. - Query rendered DOM - Use tools like
getByText
orgetByTestId
to find elements. - Assert expectations - Check that elements are there and texts match what you expect.
- Simulate events - Use something like
fireEvent.click
to see how your component reacts to user actions. - Assert state changes - Make sure your component updates correctly when things change.
Following these steps helps you check if your React components act the way they should under different conditions.
Key Tools for Unit Testing React
Here are some go-to tools for React testing:
- Jest - A favorite for testing React apps. It's fast and easy to set up.
- react-testing-library - This tool helps test React parts in a way that focuses on how users will interact with them.
- Enzyme - Created by Airbnb, Enzyme makes it simpler to check and manage your React components.
Unit testing is a smart move in React. It means you can make sure every part of your app works well by itself, leading to better and more reliable apps.
Comparing React Unit Testing Frameworks
1. Jest
Ease of Setup
Jest is super easy to get going with React projects. If you're using create-react-app, Jest fits right in without a fuss. You don't need to do much to start testing your React components because Jest has everything ready for you.
Integration with React
Jest is a great match for React apps. It naturally understands JSX and offers special tools like .toContainReact()
that make checking your React components straightforward.
Testing Capabilities
Jest comes packed with features for testing, including taking snapshots, faking functions, and handling timers. Its watch mode is interactive, which means you can write and fix tests without headaches. Jest is also good with async code, which pops up a lot in React.
Community Support
Jest is popular, with millions of downloads every week. This means there's a lot of help out there if you get stuck, including detailed guides and a bunch of articles. The community is active, constantly making Jest better and quickly solving any problems.
Performance
Jest is quick and designed to make testing smooth. It runs multiple tests at the same time to speed things up. Jest is smart too, figuring out which tests are slow and doing those first to save time. All this makes for a hassle-free testing experience.
2. React Testing Library
Ease of Setup
Getting started with React Testing Library is pretty straightforward. It's made to fit right into React projects, like those made with create-react-app, without needing to tweak anything. Since it's built on DOM Testing Library, the way it works is simple and you can use it without hassle from the get-go.
Integration with React
React Testing Library is all about making sure your React components work in real life, just like how real people would use them. It fits perfectly with React, focusing on checking how things look and behave in the actual webpage. This means your tests will really match up with what users see and do.
Testing Capabilities
With React Testing Library, you test components by looking at them and interacting with them like a user would. This approach is great for checking whole components or even specific parts. It's more about what you see and do on the screen, rather than poking around inside the components themselves.
Community Support
Since the React team themselves suggest using React Testing Library, it's got a solid backing. You'll find plenty of guides and help for common stuff you might want to do. Plus, it's part of the bigger DOM Testing Library family, so there's a lot of shared advice and a community ready to help.
Performance
React Testing Library keeps tests quick and to the point by not getting into the nitty-gritty of how components are built or work on the inside. It only loads what's necessary for each test, which helps keep things running smoothly. The people behind it are always working to make sure it stays fast, even as React updates and changes.
3. Enzyme
Ease of Setup
Getting Enzyme ready for testing your React components is pretty straightforward. It plays well with popular tools like Jest or Mocha, so it fits into what you're already doing with React. Just install Enzyme and a matching adapter for your version of React, and you're good to start testing with Enzyme right away.
Integration with React
Enzyme is built to test React components. It gets JSX, allows you to move through the component tree easily, and offers tools made just for React. This means you can directly check and interact with the state of your components and see how they render and act.
Testing Capabilities
Enzyme lets you test components in different ways - you can do a shallow render to focus on just that component, a full DOM render to see how it fits with others, or a static render to just look at the output. This flexibility makes it easy to either test components by themselves or see how they work together. Plus, with Enzyme's detailed tools, you can check on things like state changes, props, and how the component behaves over time.
Community Support
Enzyme is well-liked in the React testing world, so there's a lot of help out there. You'll find detailed guides, lots of articles on how to do things, an active place on GitHub for Enzyme, and forums with experienced users ready to help. If you're stuck, there's likely someone who can help you figure it out.
Performance
Enzyme is designed to be quick and efficient at testing React components. Its shallow rendering means you don't have to deal with the whole DOM, and smart caching of components makes things run smoother. Plus, easy snapshot tests help you catch issues fast without slowing down. So, you can build a solid set of tests without making things slow.
4. Mocha
Ease of Setup
Mocha is pretty straightforward when it comes to setting it up for React apps. You can use it with other tools like Chai for checking your tests and JSDOM for showing your components. Installing Mocha is easy with npm, and you only need a few steps to get it ready. The guides are clear and helpful for getting started.
Integration with React
Mocha is flexible and works well for testing React code. It can handle async/await, which is great for testing React components that use promises. When you use Mocha with something like Enzyme or React Testing Library, you can really see how your components behave by mounting them, finding elements, and simulating events.
Testing Capabilities
Mocha lets you build detailed tests with features like setting time limits for tests, organizing tests in groups, and preparing your tests with before/after steps. Since itโs modular, you can add different libraries for assertions, mocking, and showing components. Itโs especially good for testing tricky async code and handling different cases. Mocha gives you a lot of freedom to set up your tests just how you need them.
Community Support
Mocha has a big community behind it. There are lots of guides and tools made by users that make Mocha even better. If you run into problems, there are plenty of people who might have answers. While the main focus is on JavaScript testing in general, you can find help for React-specific issues through the tools that integrate with Mocha.
Performance
Mocha is quick and can run tests at the same time to speed things up. It has a smart way of letting slower tests run a bit longer so they donโt just fail. Mocha itself doesnโt look for slow parts in your tests, but you can use other tools with it to find and fix them. By itself, Mocha is a solid choice for fast and reliable testing. How well it does with React apps depends on how you set it up.
Framework Integration into Developer Workflow
Making a unit testing framework part of your daily coding routine is key to creating reliable and easy-to-manage React code. The main frameworks are designed to fit into your workflow easily and can be customized to meet your specific needs.
Jest
Jest works really well with React projects. Starting with create-react-app, Jest is set up for you from the get-go, so you can begin testing right away. Its watch mode updates your tests as you write your code, helping you get into the habit of testing regularly. Jest also makes it easy to test parts of your app in isolation by letting you mock functions and modules.
To use Jest:
- Start with create-react-app to have Jest ready to go
- Keep your test files next to your component files to stay organized
- Turn on watch mode to have your tests run automatically as you code
- Use mocks to test components without needing everything else
React Testing Library
React Testing Library helps you focus on how users actually use your components. This approach makes you concentrate on testing the important stuff. It's easy to add to your project and works with any test runner. The library encourages you to test components as they appear to users, using simple commands like render
and fireEvent
.
To use React Testing Library:
- Add it to your project with npm
- Use
render
from React Testing Library instead of ReactDOM.render - Find elements by what they're called or what they do, not how they're made
- Use
fireEvent
to simulate how users interact with your app
Enzyme
Enzyme is great for getting into the finer details of your components. It lets you test components on their own by shallow rendering, which means you don't have to deal with the whole app. You can also check and control the state of components and see how they change over time. This is really helpful for testing more complex behaviors.
To use Enzyme:
- Get Enzyme and the right adapter for your React version
- Use
shallow
from Enzyme to focus on individual components - Access and control components with commands like
wrapper.setState()
andwrapper.find()
- Test what happens after updates with lifecycle methods
Each framework has its own strengths. Jest is all-in-one, while Testing Library and Enzyme let you pick and choose what you need. Try different combinations to see what works best for your coding style. The most important thing is to keep testing your components consistently!
Best Practices for React Unit Testing
When you're building apps with React, making sure each part works right on its own is super important. Here are some top tips to make sure your tests do their job well.
Test One Component at a Time
It's best to focus on just one piece at a time when testing. This makes things:
- Easier to write: You only have to pretend to be other parts of the app for one piece, not the whole thing.
- Faster to run: Since each test is its own thing, they can all go at the same time.
- More reliable: If something goes wrong, you know exactly where to look.
- Simpler to keep up: Changing one part won't mess up tests for other parts.
Use Mocks and Stubs
React parts often need info from the internet or other places. Instead of using real data in tests:
- Mocks act like these outside parts and how they behave.
- Stubs are like mocks but they already know what they're going to say back.
This way, your tests don't depend on things being up and running outside your test.
Write Tests Before Code
In test-driven development (TDD), you write tests that fail first based on what you want your app to do. Then, you write code to make those tests pass. Why this is good:
- Well-designed components: Thinking about how to use the parts first helps make them better.
- Higher test coverage: You end up writing tests as you go, not trying to add them later.
- Focused development: You only write what you need to pass the tests you've set.
Use a Testing Framework
Tools like Jest, React Testing Library, and Enzyme give you a bunch of helpful stuff for testing without having to make it all yourself. This can save you a lot of time.
The main idea is to keep making tests that are easy to handle, focus on one part at a time, use pretend data, and use tools that help with testing. This makes sure your React app works well from the start.
sbb-itb-bfaad5b
Demonstrations and Examples
When we test React components, we're making sure each part works right before we put them all together in our app. Let's look at some ways to do this with different testing tools.
Testing a Form Component with Jest
Imagine we have a form in our app. Here's how Jest can help us test it:
import { render, fireEvent } from '@testing-library/react';
import FormComponent from './FormComponent';
test('should submit form value on button click', () => {
const mockSubmit = jest.fn();
render(<FormComponent onSubmit={mockSubmit} />);
fireEvent.change(screen.getByRole('textbox'), {
target: { value: 'Test Input'}
});
fireEvent.click(screen.getByRole('button'));
expect(mockSubmit).toHaveBeenCalledWith('Test Input');
});
This test first shows the form, then types into it, clicks submit, and checks if the form sent the right text.
Testing Component Rendering with React Testing Library
Let's see how to check if a message shows up correctly with React Testing Library:
import { render } from '@testing-library/react';
import MessageComponent from './MessageComponent';
test('should render success message when prop is true', () => {
const { getByText } = render(<MessageComponent success />);
expect(getByText('Success!')).toBeInTheDocument();
});
test('should render error message when prop is false', () => {
const { getByText } = render(<MessageComponent success={false} />);
expect(getByText('Error!')).toBeInTheDocument();
});
This checks if the right message (Success or Error) shows up depending on a setting.
Testing Component State with Enzyme
Here's how Enzyme can help us see if a counter in our app goes up when we click a button:
import { shallow } from 'enzyme';
import Counter from './Counter';
test('should increment counter on button click', () => {
const wrapper = shallow(<Counter />);
expect(wrapper.state('count')).toEqual(0);
wrapper.find('button').simulate('click');
expect(wrapper.state('count')).toEqual(1);
});
This test checks that the counter number goes up by 1 when we click the button.
These examples show how we can use different tools to test parts of our React apps. Testing like this helps us find problems early and make our apps more reliable.
Pros and Cons
When we're choosing a React unit test framework, it's like picking the right tool for a job. Each one has its good points and not-so-good points. Let's look at them in a simple way.
Framework | Good Points | Not-So-Good Points |
---|---|---|
Jest | - Simple to set up and works well with React - Has a lot of helpful features for testing - Many people use it, so there's lots of help available - It's fast and keeps things moving smoothly |
- Sometimes it decides too much for you - You might need extra tools for some tasks |
React Testing Library | - Tests how users actually use your app - Easy to start using in your project - Pushes you to test the right way - Always getting better with updates |
- Doesn't have many built-in tools for checking details - Harder to look at the inside parts of your app |
Enzyme | - Lets you look closely at your app's parts - You can choose how to test things - Lots of ways to check if things work right - Easy to understand how to use - Works with different ways of testing |
- Can make you focus too much on small details - Setting it up can take extra steps |
Mocha | - You can make it work just how you want - Good at handling tasks that wait on something else - Lots of people use and improve it - You can add what you need to it - Tests can run at the same time to save time |
- Needs more work to set up - Doesn't come with built-in checks - You have to mix it with other tools for React - Doesn't give much advice on how to organize tests |
Jest is really handy because it's easy to use and fits right in with React apps, plus it's quick. But, it might not let you do everything your way.
React Testing Library is great for making sure your app works like real people would use it, but it might not give you all the tools you need.
Enzyme gives you a close look at how your app's parts work, but it can make you pay too much attention to small things.
Mocha lets you set things up just how you like, but you'll need to do more work to get it there.
The best choice depends on what your app needs. If you're using create-react-app, Jest might be the best fit. If you need to set things up in a special way, Mocha could be better. Enzyme is good for when you need to really dig into how your app works, and Testing Library is best for focusing on how users see your app. Mixing these tools can help you get the best of each.
Conclusion
When it comes to making sure the parts of your React app work right, picking the best tool to help you test them is key. Each testing tool has its own strengths and things it's not so good at, so you need to think about what's most important for your project.
Jest is super easy to use, especially if you're just starting out. It works really well with React right from the start, thanks to create-react-app. It also has cool features like watching your code and running tests automatically as you make changes. Plus, lots of people use Jest, so finding help when you need it is easy.
React Testing Library is all about making sure your app works like real users would use it. It doesn't get too caught up in the technical bits, which helps you focus on what matters. It might not do everything by itself, but it works well with other tools.
Enzyme is great when you need to look closely at what your app is doing and control how it behaves in tests. It lets you do things like check parts of your app without running everything, which can be really handy. But, you have to be careful not to get too focused on small details.
Mocha gives you a lot of freedom to set up your tests exactly how you want. It's good for when you have specific needs that other tools don't meet. But, getting started with Mocha can take a bit more work.
Choosing the right tool depends on what you're working on:
- If you're new, Jest is a good place to start because it's easy and works well with React.
- If making sure your app feels right to users is important, go with Testing Library.
- When you need to really dig into how your app works, Enzyme can help.
- For special setups, Mocha might be the way to go.
Sometimes, you might find that using more than one tool is the best way to cover all your bases. Testing is super important for making sure your React app is solid, so pick the tool that lets you and your team do your best work.
Related Questions
What is the best test framework for React?
Jest is really popular for testing React apps. Facebook made it, and it's designed to make testing easier for React developers. It's simple to set up, works well with React, and has cool features like automatically rerunning tests when you make changes, letting you pretend to be parts of your app, and checking if your UI stays the same over time. People like Jest because it's straightforward and has everything you need to test React apps.
What are the three essential as related to unit testing?
The three key steps in a unit test are:
- Set up the component you're testing
- Do something like click a button or enter text
- Check that what you expected to happen actually did
For instance, you might show a component on the screen, click a button on it, and then make sure it updated the way you thought it would.
What should be unit tested in React?
When you're testing React components, focus on one thing at a time. Start by making sure a component shows things correctly. Then, check that it responds to clicks or other actions the right way. Testing one thing at a time keeps your tests simple and quick.
What is React testing framework?
React Testing Library is a tool that helps you test your React components in a way that's similar to how real people use them. It's not about the nitty-gritty details of the code but more about making sure your app works as expected. It offers simple ways to show your components and see how they behave when you interact with them, like clicking or typing.