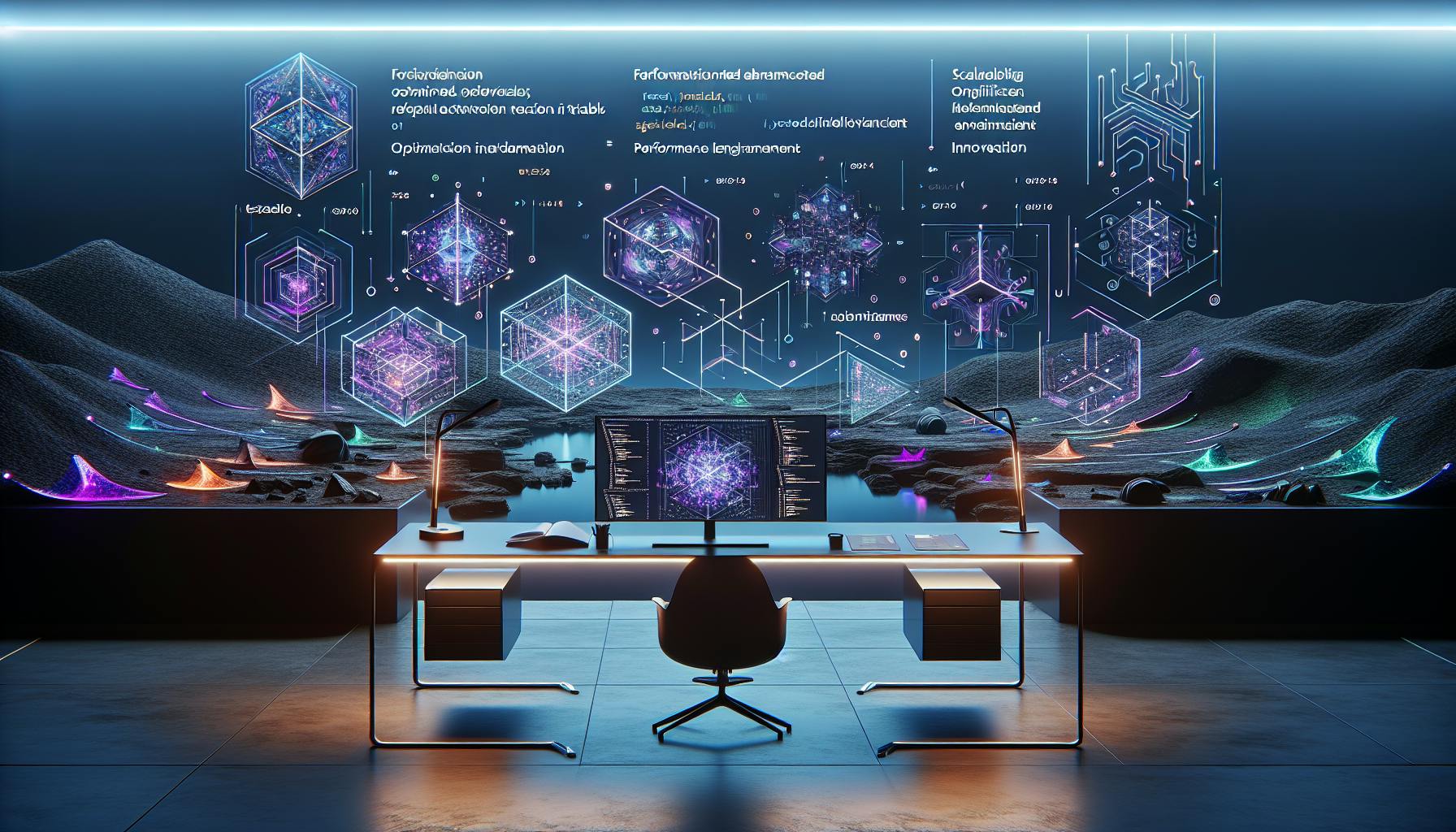
Learn React functional testing best practices, from React Testing Library to Jest matchers. Discover advanced techniques for scalable test suites and optimizing performance.
Most developers would agree that comprehensive testing is critical for building high-quality React applications.
By following industry best practices for React functional testing, you can create maintainable, scalable test suites that give you confidence in your code.
In this post, you'll learn key techniques like:
- Adopting React Testing Library for clear, isolated UI tests
- Writing expressive assertions with Jest matchers
- Simulating user events for interaction testing
- Optimizing test performance for speed and reliability
Equipped with these best practices, you'll be set up for success in professional React development.
Introduction to React Functional Testing
Understanding React Functional Testing
React functional testing refers to testing React components to verify that they render and behave as expected. It focuses on testing components from the user's perspective by interacting with them similarly to how an end user would. This involves rendering components, finding elements, interacting with those elements (clicking, typing text, etc.), and asserting that the component responds appropriately to those interactions.
Some key aspects of React functional testing include:
- Testing components in isolation to pinpoint issues
- Avoiding testing implementation details
- Using realistic component props and state
- Interacting with components from the user's perspective
Overall, React functional testing provides confidence that components will work properly under real-world use.
The Importance of Testing in Professional Development Environments
Testing is critical for professional React codebases. It:
- Catches bugs early, reducing cost
- Gives confidence when refactoring code
- Provides documentation on how components are intended to work
- Enables safe collaboration between developers
Without testing, projects risk becoming buggy and fragile over time. This makes continued development difficult and expensive.
Comparing React Testing Tools: React Testing Library vs Jest
The React Testing Library and Jest play complementary roles:
- Jest - Test runner with built-in assertions, mocks, test organization, and coverage reporting
- React Testing Library - Lightweight library with utilities to test components from the user's perspective
Together they provide a robust framework for testing React components functionally. React Testing Library handles rendering and interacting with components, while Jest provides infrastructure to run tests and make assertions.
Setting the Stage for Scalable Test Suites
To keep test suites maintainable as the app grows:
- Test small units - Target specific components rather than large sections of UI
- Avoid duplication - Reuse test logic between components
- Isolate tests - Components should not rely on other parts of the app to render properly
This keeps the test coverage focused, avoids brittleness when code changes, and prevents tests from becoming overwhelming.
Ensuring Maintainable Test Suites
To keep tests readable and updatable over time:
- Name tests clearly - Describe the specific behavior being tested
- Avoid implementation details - Target component outputs rather than internal logic
- Limit mock data - Mock only what's needed to render components independently
Well-structured and intention-revealing tests are much easier to update when requirements change. This reduces maintenance costs down the line.
What is functional testing in React?
Functional testing is the process of verifying that an application works as expected, meeting a set of functional requirements. This type of testing focuses on the business logic and UI components rather than testing implementation details.
Some key aspects of functional testing in React include:
- Validating UI components render correctly: Functional tests can verify that React components render without errors and display the expected UI elements on the page.
- Testing component interactions: Functional tests can simulate user interactions like clicking buttons, entering text into forms, and navigating between routes to validate that components function and interact as expected.
- Asserting proper state changes: Tests can assert that the React component state updates correctly in response to user interactions. For example, submitting a form should update state, triggering a re-render.
- Verifying correct API calls: If components make API calls to backends, functional tests can mock the API layer to assert that the React code makes the correct API requests when certain actions occur.
- Testing responsive behavior: Functional tests can validate that components display properly on various screen sizes and device types.
Overall, the goal of functional testing is to treat components as black boxes and validate that they function correctly from the user's perspective, rather than testing implementation details like state changes or lifecycle methods. This leads to faster, more maintainable test suites focused on verifying key user flows.
How do you test a function in React js?
Testing functions in React involves using a JavaScript testing framework like Jest to write and run unit tests. Here are the key steps:
Set up a React app with Jest
Create a new React app using Create React App, which comes with Jest already configured. Or manually set up Jest in an existing React project.
npx create-react-app my-app --template typescript
Create a component with a function to test
For example, create a Greeting
component that displays a greeting message based on a name
prop.
// Greeting.tsx
interface Props {
name: string;
}
export default function Greeting({ name }: Props) {
return <h1>Hello {name}!</h1>;
}
Write a test case using Jest
Import the component and assert that it displays the expected output for given props.
// Greeting.test.js
import Greeting from './Greeting';
test('displays greeting with name', () => {
render(<Greeting name="Dan" />);
expect(screen.getByText('Hello Dan!')).toBeInTheDocument();
});
Run the test
npm test
Jest will execute the test case and display the results. As you add more tests, Jest provides fast feedback on any regressions.
Overall, Jest integrates smoothly with React, making it straightforward to test components, functions, custom hooks, and more.
Can Jest be used for functional testing?
Yes, the Jest testing framework can absolutely be used for functional testing React applications.
Jest is a popular choice for testing React apps because:
- It comes built-in with Create React App, so it's easy to get started with Jest without any additional configuration.
- Jest provides useful features like mocking, test coverage reports, and snapshot testing out of the box. These make writing and maintaining tests easier.
- Jest works nicely with React Testing Library, which encourages testing behavior rather than implementation details. This leads to more maintainable tests over time.
- Jest is well-documented and has an active community behind it. There are lots of resources available for troubleshooting Jest tests.
Some key things to keep in mind when using Jest for functional testing:
- Structure tests using the AAA pattern - Arrange, Act, Assert. This keeps tests easy to read and understand.
- Try to test one behavior per test case. Don't overload a single test with too many assertions.
- Use React Testing Library utilities like
render
andscreen
to query DOM elements and simulate browser interactions. - Take advantage of Jest snapshot testing to prevent unexpected UI changes from being introduced.
So in summary - yes, Jest is a great option for functionally testing React components and applications. The combination of Jest and React Testing Library provides a robust testing framework that promotes writing maintainable, behavior-driven tests.
What testing framework does React use?
React does not prescribe a specific testing framework, but the most popular and commonly used framework is Jest.
Jest: The Preferred Testing Framework
Jest is an open-source JavaScript testing framework developed and maintained by Facebook. Here are some key reasons why it has become the preferred choice for testing React applications:
- Integrates seamlessly with React - Jest is designed to work specifically with React. It supports snapshot testing and other React features out of the box.
- Fast and interactive - Jest runs tests in parallel for improved performance. It also provides an interactive
watch
mode to rerun tests when files change. - Easy setup - Jest is simple to configure with Create React App projects. Just a few lines of configuration is enough to get started.
- Powerful mocking - Jest's mocking features make it easy to isolate component behavior and test specific parts of your app.
In summary, Jest's tight integration, speed, simplicity, and mocking capabilities make it a natural fit for testing React apps. Companies like Airbnb, Uber, and Amazon use it successfully for their React codebases. As React has continued to gain popularity, Jest has emerged as the de facto standard testing framework.
sbb-itb-bfaad5b
React Functional Testing Best Practices
Adopting React Functional Testing Library for UI Tests
The React Testing Library is a popular choice for writing functional tests that simulate user interactions in React applications. Here are some best practices when adopting React Testing Library:
- Import
@testing-library/react
and use itsrender
method instead of Enzyme or ReactTestUtils. This ensures your tests resemble how users interact with UI components. - Use
screen
to query elements rather than relying on component internals. This reduces fragility when refactoring UI code. - Leverage utilities like
userEvent
to simulate interactions like clicking buttons instead of directly calling props. This better matches real user behavior. - Follow the Guiding Principles from Testing Library docs to write maintainable tests. For example, avoid including implementation details in test code.
Designing React Functional Testing Examples for Clarity
Well-designed examples demonstrate how to properly test React components and make tests understandable for other developers:
- Use descriptive test names like
displays error message when submitting invalid form
. - Structure tests to follow AAA pattern - Arrange, Act, Assert. Helps reveal test intention.
- Limit each test to one assertion. Forces tests to be laser-focused.
- Leverage
data-testid
attributes for clear element selection. Avoids fragile DOM structure matching. - Illustrate both happy paths and edge cases in examples. Builds confidence in test suite.
Strategies for Isolated and Repeatable Tests
Effective testing requires each test case to be isolated and operate independently:
- Manage test side-effects with utilities like
react-testing-library/cleanup-after-each
. Ensures no shared state across tests. - Use
jest.spyOn
to mock functions instead of importing actual implementations. Allows for better control. - Introduce custom render with
wrapper
to contain global contexts like Redux store. Limits external dependencies. - Seed generator functions to remove randomness in test runs. Provides repeatable behavior.
Utilizing jest-dom Matchers for Expressive Assertions
The jest-dom library contains helpful custom matchers for DOM elements:
- Use
.toBeVisible()
and.toBeInTheDocument()
for clearer assertions compared to.toBeTruthy()
. - Leverage exact matchers like
.toHaveTextContent()
over.toContain()
to prevent false positives. - Introduce
.toBeDisabled()
and.toBeEnabled()
matchers to test element states. - Follow Testing Library's guidance to avoid overusing custom matchers.
Creating a Custom Render Function with @testing-library/react
Encapsulate common test logic and dependencies using a custom render function:
- Import
@testing-library/react
'srender
instead of React DOM'srender
. - Preload providers like
<MemoryRouter>
to handle routing in tests. - Initialize custom contexts like Redux store for state management.
- Attach reusable utilities like
userEvent
for simulated interactions. - Export wrapped render function for test files to easily import.
This promotes DRY principle in tests and creates reusable testing context.
Setting Up a React Testing Environment
Initializing a React Project with Create React App
Create React App is a useful tool for initializing a React project with zero configuration. It comes pre-configured with Jest, a popular JavaScript testing framework, making it a great starting point for React testing.
To set up a new React app with Create React App and Jest:
- Install Create React App globally:
npm install -g create-react-app
- Generate a new React project called
my-app
:
create-react-app my-app --use-npm
- Navigate into the project directory:
cd my-app
The project will now have Jest installed and configured out of the box. You can run existing test suites with:
npm test
Integrating React Testing Library with Jest
While Jest provides a test runner, React Testing Library is a helpful addition that encourages good testing practices focused on DOM interactions.
To set up React Testing Library:
- Install
@testing-library/react
and@testing-library/jest-dom
:
npm install --save-dev @testing-library/react @testing-library/jest-dom
- Import relevant utilities from both libraries in test files:
// example test.js
import '@testing-library/jest-dom'
import { render, screen } from '@testing-library/react'
- Use React Testing Library utilities like
render
andscreen
to write tests.
This combination enables you to leverage React Testing Library's API while keeping Jest's test runner and assertions.
Leveraging @testing-library/user-event for Simulating User Actions
@testing-library/user-event is a companion library that allows simulating realistic browser interactions like clicking, typing, hovering, etc. in tests.
To add it:
npm install --save-dev @testing-library/user-event
Then import userEvent
and simulate actions:
import userEvent from '@testing-library/user-event'
userEvent.click(screen.getByRole('button'))
This improves tests by modeling actual user events instead of directly invoking React handlers.
Incorporating MemoryRouter for Testing React Router
When testing components that use React Router, MemoryRouter can be used to simulate router behavior without requiring a full routing configuration.
Import it from react-router-dom
and wrap tested components:
import { MemoryRouter } from 'react-router-dom'
test('Navigation works', () => {
render(
<MemoryRouter>
<MyComponent/>
</MemoryRouter>
)
})
This enables proper testing of routing-dependent components.
Accessing Testing Docs and Dedicated Support
For detailed documentation on testing React apps, see the official React Testing Library docs.
The Jest website also contains excellent testing guides and API references.
For additional help, the React Testing Library and Jest GitHub repositories have dedicated support channels.
Writing Effective React Functional Tests
React Functional Testing Tutorial: From Setup to Assertion
Here is a step-by-step tutorial on setting up and writing a basic functional test for a React component:
- Set up the testing environment - Install React Testing Library and any other needed libraries with
npm install --save-dev @testing-library/react
. Configure Jest or other test runner. - Import libraries - Import
render
andscreen
from React Testing Library, as well as any other needed utilities likeuserEvent
:
import { render, screen } from '@testing-library/react';
import userEvent from '@testing-library/user-event';
- Render the component - Use the
render
method to render the target component:
render(<MyComponent />);
- Interact with component - Use
userEvent
to simulate browser interactions:
userEvent.click(screen.getByRole('button'));
- Make assertions - Use Jest's expect or React Testing Library utilities like
screen.getByText()
to make assertions:
expect(screen.getByText('Data loaded!')).toBeInTheDocument();
This covers the basics of setting up a test, rendering a component, interacting with it, and asserting expected outcomes.
Testing React Components with Screen and UserEvent
The screen
utility and userEvent
library provide ergonomic ways to test components.
screen
queries elements and makes them easy to assert on with methods like:
screen.getByText()
- get element by text contentscreen.getByLabelText()
- get by associated labelscreen.getByTestId()
- get by test ID attribute
For example:
screen.getByRole('button'); // Get button element
userEvent
simulates browser interactions:
userEvent.click(buttonElement); // Click button
Common actions like clicks, inputs, scrolls etc. are supported.
Together they facilitate straightforward interaction testing without needing DOM manipulation.
Validating Action Creators and Reducers in Functional Tests
Testing action creators and reducers with Jest:
Action creator
test('login action creator', () => {
const action = loginActionCreator('test_user');
expect(action).toEqual({
type: 'LOGIN',
payload: 'test_user'
});
});
Reducer
test('login reducer', () => {
const initialState = { user: null };
const state = loginReducer(initialState, {
type: 'LOGIN',
payload: 'test_user'
});
expect(state).toEqual({ user: 'test_user' });
});
Action creators and reducers can be tested in isolation without React components.
Crafting Snapshot Tests with Jest
Jest's snapshot testing feature lets you save component "snapshots" and detect changes on subsequent test runs.
test('MyComponent snapshot', () => {
const component = render(<MyComponent />);
expect(component).toMatchSnapshot();
});
On first run a snapshot file is generated. Jest compares future snapshots against it, surfacing unexpected differences.
Snapshots help prevent unintended changes but should be used sparingly.
React Integration Testing: Bridging Unit and Functional Tests
- Unit tests focus on specific functions/modules in isolation. Great for pure logic.
- Integration tests check interactions between coupled modules. Useful for imperative workflows.
- Functional tests validate components from a user perspective. Key for UX flows.
Aim for multiple levels for comprehensive coverage.
For example unit test action creators and reducers, integration test side effects/async logic, functional test component rendering and interactivity.
Each level complements the others. Together they help build robust, well-tested React applications.
Advanced React Functional Testing Techniques
Employing Cypress for End-to-End React Functional Testing
Cypress is a popular open-source end-to-end testing framework that can complement React functional testing. Here are some tips for integrating Cypress:
- Install Cypress as a dev dependency with
npm install --save-dev cypress
- Write test specs in
/cypress/integration
that navigate real user flows - Stub network requests and test edge cases not covered by unit tests
- Use
cy.intercept()
to mock API responses and test UI states - Ensure your app works across viewports with
cy.viewport()
- Leverage custom commands and utilities to reduce test code duplication
Overall, Cypress enables testing real user interactions from end-to-end, providing confidence your React app works as expected.
Optimizing Test Performance and Reliability
To keep test suites fast and reliable:
- Parallelize tests across processes with tools like
jest-runner-groups
- Profile slow tests with
jest --profile
and optimize bottlenecks - Set up CI to run tests on every commit to catch regressions
- Use
react-testing-library
guidelines to avoid fragile tests - Control test environment with custom render utils like
mockedProvider
- Freeze dependencies and test on locked commit SHAs to limit flakiness
Investing in performance and stability ensures tests remain useful over time.
Refactoring Tests with Custom Render and Utilities
Refactoring tests with custom utils like renderWithRouter
reduces duplication:
// test-utils.js
import { render } from "@testing-library/react";
import { MemoryRouter } from "react-router-dom";
export function renderWithRouter(
ui,
{
route = "/",
history = createMemoryHistory({ initialEntries: [route] })
} = {}
) {
return {
...render(<Router history={history}>{ui}</Router>),
history
};
}
// HomePage.test.js
import { renderWithRouter } from 'test-utils';
test('navigation', async () => {
const { history } = renderWithRouter(<HomePage />);
fireEvent.click(getByText('About'));
expect(history.location.pathname).toBe('/about');
});
Abstracting shared logic makes tests more maintainable.
Testing Overview: Guiding Principles and Recommended Tools
Effective testing starts with principles like:
- Confidence - Tests should inspire confidence the code works as intended
- Stability - Tests should provide consistent, reliable feedback
- Maintainability - Tests should be simple, clean, and reusable
Recommended tools include:
- React Testing Library - Lightweight and guidance-focused
- Jest - Fast and feature-rich test runner
- Mock Service Worker - Intercept and mock network requests
- Testing Playground - Visualize and debug tests
Choosing the right foundations sets your test suite up for success.
Building Custom Matchers for Enhanced Test Expressiveness
Custom matchers like toBeVisible()
make tests more expressive:
// test-utils.js
expect.extend({
toBeVisible(element) {
const style = getComputedStyle(element);
return style.visibility === 'visible';
}
});
// Display.test.js
test('shows the element', async () => {
render(<Display />);
const element = screen.getByRole('alert');
await waitFor(() => expect(element).toBeVisible());
});
Investing in custom matchers and utilities pays dividends in clarity.
Conclusion: Embracing React Functional Testing
Key Takeaways for React Functional Testing
React functional testing focuses on testing components in isolation to ensure they render and behave as expected. Key takeaways include:
- Use React Testing Library for simple, maintainable tests that encourage best practices
- Leverage utilities like
render
andscreen
to query components - Write tests from the user's perspective, interacting with components like a user would
- Avoid testing implementation details
- Mock dependencies to test components in isolation
- Start testing early, integrate into development workflow
The Role of Testing in Front End Development
As front end applications grow in complexity, testing is crucial to ensure quality and maintain velocity. Key roles testing plays:
- Prevents regressions - Tests safeguard against unintended changes and breakages
- Facilitates refactoring - Tests allow large scale changes with confidence
- Enables agility - Faster feedback lets developers iterate rapidly
- Reduces risk - Tests catch issues early, reducing cost of fixes
- Improves design - Testing incentives better componentization
For React specifically, unit and integration tests are invaluable for sustainable growth.
Next Steps for Enhancing Your Testing Skills
To further enhance React testing skills:
- Explore React Testing Library in depth through docs
- Learn effective test patterns like reducer testing
- Study integration testing tools like Cypress
- Contribute to open source React projects to learn from example test suites
- Attend testing focused talks and conferences
- Consider a testing role as a specialization
The React ecosystem offers rich resources to build impactful test suites. Continued learning pays dividends in application quality.