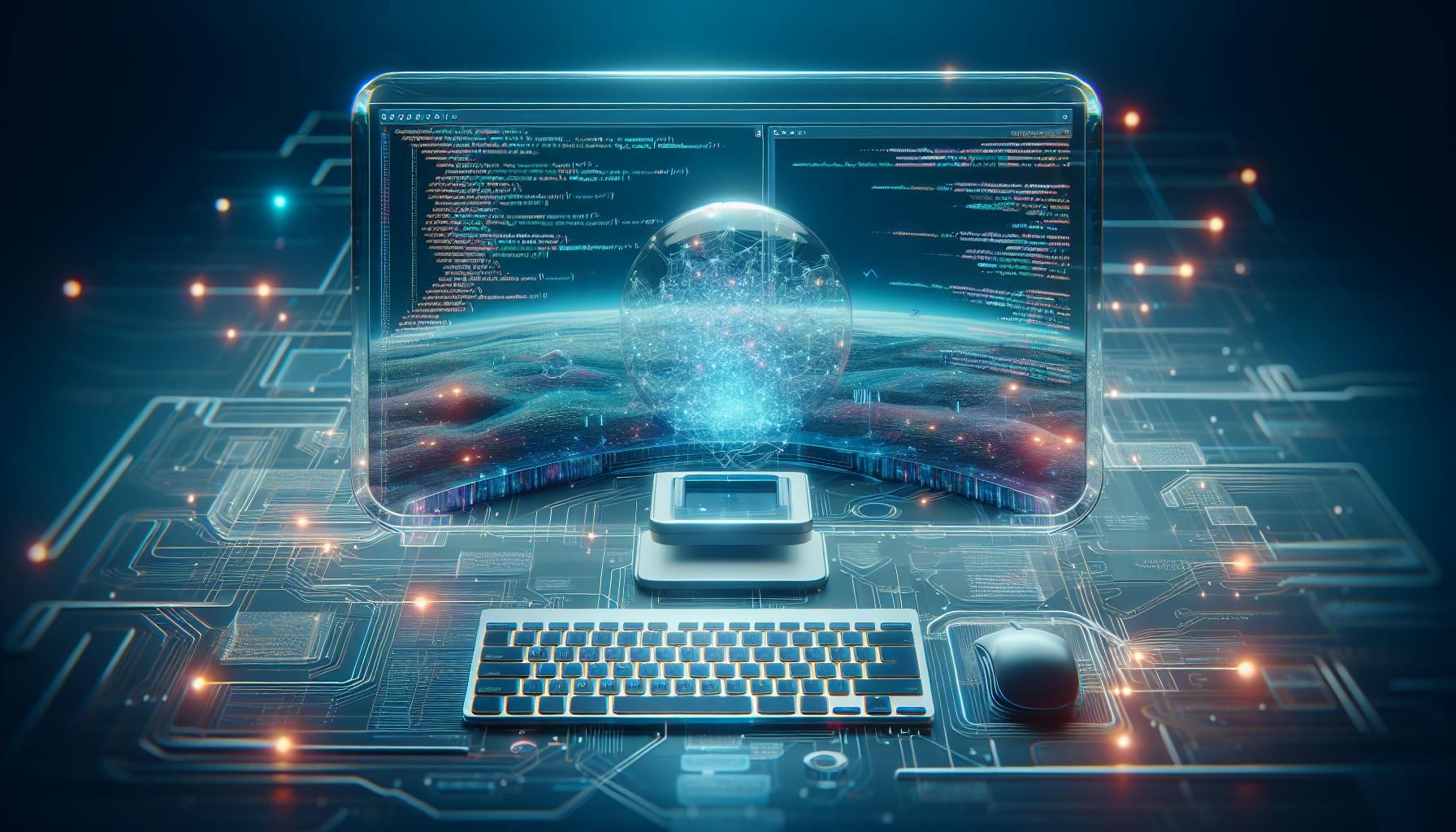
Learn how to use serverless functions on Netlify to build and deploy web applications. Explore the benefits, setup process, and examples to get started.
Starting with Serverless Functions on Netlify can revolutionize the way you build and deploy web applications, making it simpler, more cost-effective, and scalable. Hereโs what you need to know in a nutshell:
- Serverless functions are small, event-driven pieces of code that run without a dedicated server, saving you money and scaling automatically.
- Netlify provides an easy-to-use platform for deploying these functions, especially for Node.js projects.
- Getting started involves installing Node.js, the Netlify CLI, and possibly Git for version control.
- Deployment is straightforward with Netlify, automating many tasks and providing tools for local testing.
- Benefits include cost savings, automatic scaling, enhanced security, and the ability to focus on coding rather than infrastructure.
- Functions can be used for a wide range of tasks, such as processing data, handling forms, or integrating with other services.
This guide covers everything from the basics of serverless functions and why Netlify is a great platform for them, to setting up your project and writing your first function. Whether youโre a beginner or looking to expand your skills, this guide offers a comprehensive overview to get you started with serverless functions on Netlify.
What are Serverless Functions?
Serverless functions are like tiny programs that run only when they need to, based on certain triggers, like when someone visits your website. Here's what makes them special:
- They wait for a signal - These functions start working when something specific happens, like when a file is uploaded or someone fills out a form on your site. They're not running all the time, just when needed.
- Short-lived - They do their job quickly and don't hold onto any data. If they need information, they have to get it from somewhere else each time they run.
- They can handle the rush - If your website suddenly gets a lot of visitors, serverless functions can scale up automatically to keep everything running smoothly.
- You pay as you go - Costs are based on how much the functions run. If they're not being used, you're not paying for them. This can save you money compared to paying for a server all the time.
- Quick to update - You don't need to set up or manage servers, so you can focus on improving your code. Changes can be made fast and easily.
- Choose your language - You can write them in languages like JavaScript, Python, or others. This gives you the flexibility to use what you know best.
Serverless functions are great for doing things like processing data, working with files, connecting to other services, making your website interactive, automating tasks, or cleaning up data regularly.
With Netlify, these functions are super handy for adding features to websites without needing a separate server. You could use them for stuff like handling forms, making calls to other services, managing who gets to see what, and more.
Why Use Serverless Functions on Netlify?
Using Netlify for serverless functions makes things a lot easier. Here's why it's a good choice:
It Handles Busy Times Well
- Netlify can automatically handle more visitors by adding more power as needed. This means your site stays smooth without you having to do anything extra.
It's Safe
- Your code runs in its own safe space, and Netlify looks after security stuff like fighting off hackers and keeping your site secure.
You Can See How Things Are Going
- Netlify shows you how your functions are doing right now, so you can quickly fix any issues.
Keep Focused on Coding
- Spend more time writing code and less on setting up servers. With Netlify Dev, you can easily work on your functions on your own computer.
Great for Websites with Static and Dynamic Parts
- It's perfect for adding features like forms to websites that don't change much, making them more interactive.
Easy to Update
- When you're ready, just push your code, and Netlify takes care of the rest, like testing and making it live.
Flexible Power
- Netlify adjusts to your needs, whether you're getting a little or a lot of traffic. And you only pay for what you use.
In short, Netlify makes working with serverless functions simpler. It takes care of the heavy lifting so you can focus on creating.
Prerequisites
Before you dive into using Netlify functions, there are a couple of things you need to set up first:
Node.js
Netlify functions run on Node.js. This means you need Node.js installed on your computer. You can get it from the official website.
Go for the Long Term Support (LTS) version because it's more stable for ongoing projects.
Netlify CLI
The Netlify CLI is a tool that lets you test and deploy your functions right from your command line.
To install it, open your terminal and type:
npm install -g netlify-cli
After installing, connect it to your Netlify account with:
netlify login
This step links the tool to your account.
Git (optional)
Using Git isn't a must, but it's super helpful. It lets you keep track of changes and easily update your project. If you don't have Git, you can grab it here.
With Node.js and the Netlify CLI ready, you're all set to start playing with Netlify functions on your local machine. And if you decide to use Git, it'll make updating your project a breeze.
Initialize a Netlify Project
Setting up a new project with Netlify CLI is straightforward. It involves linking your project to a GitHub repository for easy updates and creating a configuration file.
1. Run netlify init
First, open your terminal, go to your project folder, and type:
netlify init
This starts a simple step-by-step process that helps you:
- Connect your project to a GitHub repository
- Set up automatic updates every time you change your code
- Create a netlify.toml file for your project settings
- Give you a website link to see your project live
Just follow the on-screen instructions to complete each step.
2. Link to a GitHub Repository
Linking your project to GitHub means every time you update your code on GitHub, Netlify will automatically update your website. This is known as continuous deployment.
To do this:
- Make a GitHub repository for your project
- During the
netlify init
process, choose this new repo - You might need to connect your Netlify and GitHub accounts
Now, whenever you push updates to GitHub, your Netlify site will automatically get updated too.
3. Generate a netlify.toml
During setup, a file named netlify.toml will be created in your project. This file tells Netlify how to handle your project, including:
- Build - Commands to build your site, where to find the built site
- Functions - Where your serverless functions are stored
- Redirects - How to direct website traffic
- Headers - Setting rules for your site, like security policies
Here's what the basic setup looks like:
[build]
command = "npm run build"
publish = "build"
[functions]
directory = "functions"
[[redirects]]
from = "/*"
to = "/index.html"
status = 200
You can change this file as needed to fit your project.
By following these steps, you've connected your project to both GitHub and Netlify, making it easier to manage updates and deploy your site.
sbb-itb-bfaad5b
Create a Simple Serverless Function
Let's get started with writing a basic serverless function on Netlify. Here's a step-by-step guide to creating a simple "Hello World" function.
1. Make a functions
Folder
First, you need a place for your serverless functions to live. Create a folder named /functions
at the root level of your Netlify project.
2. Write a Function File
Next, create a new file called hello.js
inside the /functions
folder. This file will contain your serverless function code. Here's a simple example that sends back a "Hello World" message:
exports.handler = async (event, context) => {
return {
statusCode: 200,
body: JSON.stringify({
message: "Hello World"
})
}
}
This code means that whenever the function is called, it will respond with a message saying "Hello World".
3. Test the Function Locally
To see if your function works, use the netlify dev
command in your project's main folder. This starts a local server.
You can find your function at http://localhost:8888/.netlify/functions/hello
.
Try going to this link in your web browser or use a tool like Postman to send a request. If everything's set up right, you'll see the "Hello World" message.
And that's it! You've just created and tested a simple serverless function on Netlify. To make it live, just push your updates to GitHub, and Netlify's Continuous Deployment will do the rest.
Deploy and Manage Functions
Once you make changes and update your code on GitHub, Netlify automatically takes care of getting your serverless functions out there and managing them, no matter how big or small the demand gets.
Easy Deploys
Push your code updates, and let Netlify do the heavy lifting.
- After you update your code on GitHub, Netlify automatically gets your functions ready and puts them online.
- You don't have to worry about setting up servers or dealing with complex setups - Netlify handles all that.
- This lets you focus more on coding and less on technical setup.
Function Logs
Netlify lets you see logs for your functions, so you can understand what's happening and fix issues fast.
- Access logs help you see when your functions are called and what's happening with them.
- Error logs are useful for spotting and fixing problems in your functions.
- You can check these logs on Netlify's website or by using the Netlify CLI.
- This is really handy for when you're trying out new functions or need to figure out why something didn't work as expected.
Function Metrics
Netlify gives you detailed info on how your functions are doing, like how often they're used and how well they perform.
- You get to see how many times your functions are run, how long they take, and how much memory they use.
- This information helps you understand which functions are used a lot and which ones might need a bit of tweaking for better performance.
- If a function uses too much memory or takes too long, Netlify lets you know.
- This helps you spot and fix any slow spots in your functions, making sure everything runs smoothly.
Conclusion
Using Netlify for serverless functions makes things super easy. It takes care of all the technical stuff, so you can just focus on writing your code.
Here's what you should remember:
- Serverless functions are little pieces of code that run only when they're needed. This is great because you don't have to pay for a server that's running all the time.
- Netlify can handle more visitors without you having to do anything. You can write functions in languages like JavaScript.
- The Netlify CLI tool lets you try out your functions on your own computer before you put them online. When your code is connected to GitHub, Netlify updates your site automatically whenever you make changes.
- You can use functions for lots of things, like when someone fills out a form on your site or uploads a file. They help make your site do more without needing a full server.
- Netlify also shows you how your functions are doing with logs and metrics. This means you can quickly fix any problems.
In short, Netlify makes it a lot easier to use serverless functions. You don't need to know about servers or infrastructure. The platform grows with your needs and helps keep costs down.
If you want to dive deeper into how Netlify can help you with serverless functions, have a look at these resources:
- Netlify Docs on Functions
- Serverless Functions Examples
- JAMstack Explorers Serverless Missions
Related Questions
How do I use Netlify serverless functions?
To start using serverless functions with Netlify, simply make a JavaScript file in your functions
folder. Name it and write your code. Netlify looks into this folder every time you build your project, setting up each file as its own function.
What is the limit of Netlify serverless?
- 1024 MB of memory
- 10 second execution limit for quick functions, including scheduled ones
- 15 minute execution limit for longer-running background functions
What is the difference between Netlify edge and serverless?
Edge functions run closer to your users, which can make them faster. Serverless functions run on Netlify's own servers and might be easier to work with because you have more control over the code.
How do you create a serverless function?
Here's a simple way to make a serverless function:
- Make a project folder
- Put a
functions
folder inside it - Create a
netlify.toml
file for settings - Write your function in a file (like
myFunction.js
) - Mention this function in your
netlify.toml
under[functions]
- Use
netlify dev
to try it out on your computer - Use
netlify deploy
to put it online
Check out Netlify's tutorial for more step-by-step guidance.