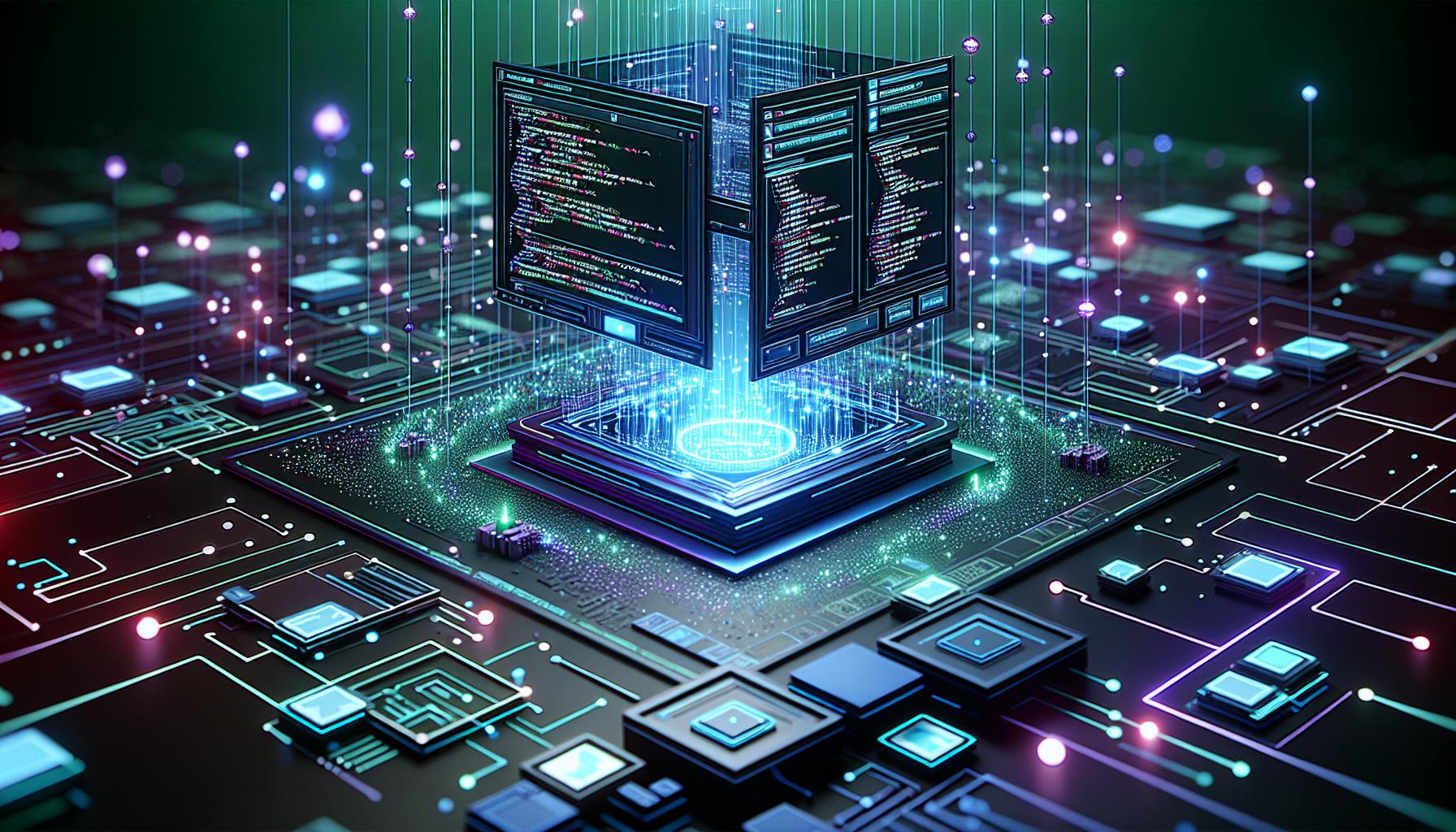
Get started with Vue 3 projects by following these initial steps. Learn how to install Node.js, set up your editor, create your project, understand the project structure, embrace the Composition API, add components and routing, and run your project.
Starting a Vue 3 project involves a few straightforward steps that get you up and running with the latest in web development. Here's what you need to know:
- Install Node.js and Vue CLI or Vite: Make sure you have Node.js (version 16 or higher) installed. Then, choose between Vue CLI and Vite for project scaffolding. Vue CLI offers more control, while Vite focuses on speed and simplicity.
- Set up your editor: VS Code with the Vetur extension is recommended for the best development experience.
- Create your project: Use Vue CLI (
vue create my-app
) or Vite (npm init vite@latest my-vue-app -- --template vue
) to create your project. - Understand the project structure: Familiarize yourself with the default structure and files created by Vue CLI or Vite.
- Embrace the Composition API: Learn about the Composition API for better code organization and flexibility, especially with TypeScript.
- Add components and routing: Start building your app with components and set up routing with Vue Router.
- Run your project: Use
npm run dev
to launch your development server and see your app in action.
These steps provide a solid foundation for developing modern web apps with Vue 3. Whether you're a beginner or an experienced developer, Vue 3 offers a blend of performance, flexibility, and ease of use that's hard to beat.
Prerequisites
Before you jump into starting your Vue 3 project, there are a few things you need to have ready:
Node.js
Node.js is a tool that lets you use JavaScript for server-side tasks. Since Vue 3 is built with JavaScript, you need Node.js to get your project environment ready and to run a server on your computer.
Go to the official website and download the latest LTS version of Node.js. It's important to get version 16 or higher because Vue 3 uses some new JavaScript features.
Once installed, you can check if Node.js is ready by typing this in your command line:
node -v
Vue CLI or Vite
To start your Vue 3 project, you can use tools like Vue CLI or Vite. These help set up your project quickly:
- Vue CLI: This has been around since Vue 2 and comes with options for different tools like Babel and ESLint.
- Vite: This is newer and faster, designed especially for Vue 3. It works more directly with the web's languages.
You can install either of these tools with npm by running one of these commands:
npm install -g @vue/cli
npm install -g vite
Editor
You'll also need a program to write and edit your Vue code. Some popular ones are VS Code, WebStorm, and Vim.
For the best experience with Vue, VS Code with the Vetur extension is highly recommended. It has helpful features like highlighting parts of the code, suggesting completions, and checking for errors.
With these things set up, you're all ready to start your first Vue 3 project!
Step 1: Install Node.js and Vue CLI
Installing Node.js
To get Node.js on your computer, here's what you do:
- Head over to nodejs.org and pick the LTS version to download. LTS stands for Long Term Support, which means it's more stable.
- Once you've downloaded Node.js, run the setup. Just keep clicking 'Next' until it's all done.
- To check if everything's okay, open up your terminal (or command prompt) and type
node -v
. You should see a version number, which means Node.js is ready to go.
Installing Vue CLI
Now that you have Node.js, let's get Vue CLI set up:
npm install -g @vue/cli
This command installs Vue CLI on your computer for everyone to use.
To make sure it's installed, type vue --version
in your terminal. You'll see the version of Vue CLI you have.
That's it for the basics of setting up your environment for starting a Vue 3 project. With Node.js and Vue CLI installed, you're ready to move on.
Step 2: Creating Your Vue 3 Project
When you're ready to start your Vue 3 project, you have two main ways to do it - using Vue CLI or Vite. Let's look at what each of these options offers.
Using Vue CLI
Vue CLI is a tool made by the Vue team to help set up new projects. Here's how to create a project with it:
- Type
vue create my-app
in your terminal. - Choose the "Vue 3 preset" option.
- Decide if you want to add extras like Babel, TypeScript, Router, Vuex, etc.
Good points:
- It's got lots of features, like testing and adding web app capabilities.
- It uses webpack, which is a popular way to put together your project files.
- There’s a big selection of plugins.
Not so good points:
- It can be a bit slow when you make changes and want to see them right away.
- Setting it up can get complicated.
Using Vite
Vite is another option that’s focused on making things fast and simple.
To start a project with Vite:
npm init vite@latest my-vue-app -- --template vue
Good points:
- It updates your app super quickly when you make changes.
- It starts up really fast.
- The setup is straightforward.
- It uses the latest web technology for importing your files.
Both Vue CLI and Vite are great starting points for a Vue 3 project. Vite is all about speed and simplicity, while Vue CLI gives you more control over your project's setup. Think about what you need for your project and choose the one that fits best.
Step 3: Project Structure and Configuration
After you start your Vue 3 project with Vue CLI or Vite, you'll notice it creates a basic setup for you. This includes some folders and files that are essential for your project. Let's break down what you get and why it matters.
Entry Point
The main.js
file is where your app begins. It's like the front door to your Vue app, setting everything in motion and connecting your app to the webpage.
App Component
The App.vue
file is the core of your Vue app. Think of it as the main room from which all other parts of your app branch out. It's the first component that gets loaded.
Assets Folder
The assets
folder is your storage space for things like pictures, fonts, and stylesheets. It's where you keep the stuff that makes your app look good.
Components Folder
The components
folder is for all your Vue components. Components are like building blocks for your app, letting you break up the interface into smaller, reusable pieces.
Router and Store
If you decided to include Vue Router for navigation or Vuex for state management during setup, you'll find they're already set up for you. This means less work to get started with these features.
Configuration Files
The package.json
file is like a list that tells your project what software it needs to run correctly.
For customizing how your project builds and runs, Vue CLI projects have a vue.config.js
file, and Vite projects use vite.config.js
. These files let you tweak your project settings, like how fast it loads or how it looks.
In short, when you start a Vue 3 project, the structure and files you get are there to make your life easier. They set the stage for your app, allowing you to focus on creating something awesome. As your project grows, you can add more components, play around with the router, manage your app's state, and adjust your build settings as needed.
Step 4: Understanding Composition API
Vue 3 introduced something called the Composition API, which is a new way to make your components. It's different from the old method used in Vue 2. Here's why it's pretty cool:
Better Code Organization
With the Composition API, you can split your code into smaller, reusable bits. This means you can keep parts of your code that do similar things together, making it easier to handle and look after.
Increased Flexibility
You can mix and match parts of your code as needed. If you have a chunk of code that does something neat, you can easily use it in other places.
Better TypeScript Support
This API works really well with TypeScript, which is a tool that helps make sure your code is error-free. It makes it easier to use TypeScript's features to manage your data.
Here's a simple example to show how it works:
import {ref} from 'vue'
export default {
setup() {
const count = ref(0)
function increment() {
count.value++
}
return {
count,
increment
}
}
}
ref
is a way to keep track of data like the count.- We also have a function called
increment
that adds to the count. setup
is the place where you write your code before your component shows up on the screen.
So, the Composition API helps you:
- Share logic between components
- Put together complex logic more easily
- Use TypeScript to make your code cleaner
This makes Vue 3 apps easier to manage and grow. Even though it might take a bit to learn, it's really helpful in the long run.
sbb-itb-bfaad5b
Step 5: Adding Components and Routing
Components are the parts you use to build your app's interface in Vue. Let's go through how to make and use components in your Vue 3 project.
Creating a Component
To make a new component:
- Create a file named
HelloWorld.vue
in thecomponents
folder. - Write your component code like this:
<template>
<h1>Hello World!</h1>
</template>
<script>
export default {
name: 'HelloWorld'
}
</script>
- Next, add it to App.vue by importing and registering it:
import HelloWorld from './components/HelloWorld.vue'
export default {
components: {
HelloWorld
}
}
Now, the <HelloWorld>
component can be used in your app!
Components help you split your app into smaller, reusable pieces.
Setting Up Routing
To move around in your Vue app, you'll need Vue Router, the official package for navigation.
To set up routing:
- First, install Vue Router:
npm install vue-router@4
- Then, make a router file:
import { createRouter, createWebHistory } from 'vue-router'
const router = createRouter({
history: createWebHistory(import.meta.env.BASE_URL),
routes: [
// your routes go here
]
})
export default router
- Finally, add it to main.js:
import { createApp } from 'vue'
import App from './App.vue'
import router from './router'
const app = createApp(App)
app.use(router)
app.mount('#app')
With that, you can set up routes and navigate your app!
Components and routing are essential for making Vue apps. With these basics, you're all set to build more complex interfaces.
Step 6: Running the Project
After setting up your Vue 3 project, the next step is to see your app working on your computer. Here's how to do it:
- Open a terminal window in the folder where your project is.
- Type this command and press enter:
npm run dev
This command starts a server on your computer, usually opening your app on http://localhost:3000
.
- The server will automatically update your app whenever you make changes to the files. This means you can see your changes right away.
- If you run into problems starting the app, here are some things you can try:
- Look for errors in the terminal and fix them.
- Check that you've installed all the needed npm packages.
- Try stopping and restarting the server.
- Clear your web browser's cache in case it's showing old information.
- Make sure you're visiting the correct web address. Sometimes the port number might change.
- Search online for any error messages you're getting for more help.
Getting your Vue 3 app to run smoothly on your computer is key to making your development process easier. While your project might get more complicated over time, having a working development server allows you to experiment and see your changes in real-time.
Best Practices
Here are some simple tips to help you organize your code, reuse parts of your app, make things run faster, and handle bigger Vue 3 projects.
Component Structure
- Break your app into tiny parts that each handle their own job.
- Put components into folders based on what they do.
- Use names for components that have two or more words, like UserInfoCard.vue.
- Move logic you use a lot into functions you can reuse.
- Try to keep components simple and avoid having lots of nested components.
Code Organization
- Use something like Pinia to manage shared data.
- Keep your imports and exports tidy.
- Organize your code into separate files based on what they do (like components, pages, API calls, etc.).
- Group common tools, like date converters, into folders you can use again.
Performance
- Only load parts of your app when they're needed to keep the initial download small.
- Use dynamic imports for features that aren't needed right away.
- Turn on production mode and use tools that remove unnecessary code.
- Choose images that are the right size and write efficient CSS.
- Get data ready in advance with Async components if you're going to use it again.
Testing
- Start testing components early.
- Use tools like Vitest for small tests and Cypress for bigger tests that cover more of the app.
- Stick to guidelines for how to fake parts of your app and check if things work as expected.
Scaling
- Plan how you'll organize files and manage data for bigger projects from the beginning.
- Write down how components should be used.
- Use tools like ESLint and Prettier to keep your code consistent.
- Keep an eye on how fast your app runs and its overall health.
- Make building, testing, and deploying your app automatic.
By following these straightforward tips, you'll be better prepared to handle larger and more complex Vue 3 projects while keeping your code clean and your app running smoothly.
Conclusion
Starting a Vue 3 project is the first step toward making modern websites and apps. This guide has shown you the basics to get started quickly, using the right tools and approaches.
Here's a quick summary of what we've covered:
- Prepare by installing Node.js and either Vue CLI or Vite, depending on your needs. These tools help you kick off your project.
- Pick Vue CLI if you want more control over your project's setup or Vite if you're after speed and an easy start. Both provide a ready-to-use project structure.
- Adopt the Composition API for a cleaner, more organized way to write your code, especially if you're using TypeScript.
- Break down your app into smaller parts called components, and use Vue Router for moving between pages.
- Start the development server to see your work in action and tackle any issues as they arise.
- Stick to good practices in how you structure components, organize your code, boost performance, test your app, and manage growth to ensure your Vue 3 projects are solid.
Now that you have the basics down, you're all set to build complex web apps. Dive deeper into Vue's features, like state management with Pinia, connecting to APIs, and more, by exploring the Vue documentation and community discussions. Vue 3 opens up lots of possibilities for your web projects!
Is there a particular Vue 3 feature you're eager to explore more? Let us know in the comments!
Related Questions
How do I start a vue3 project?
To get your Vue 3 project off the ground, follow these steps:
- Remove any old versions of vue-cli with
npm uninstall -g vue-cli
- Get the latest vue-cli by running
npm install -g @vue/cli
- Open vue-cli by typing
vue create my-project
and choose the Vue 3 option - Pick extra features you might want, like TypeScript, Router, Pinia, etc.
- Open the project folder with your code editor
- Start the dev server by running
npm run dev
- Add your changes to git with
git add .
- Save your changes with
git commit -m "initial commit"
How do I create my first project in Vue?
Here's how to start:
- Make sure Node.js is installed
- Get Vue CLI
- Create a new project with
vue create my-app
- Start the dev server with
npm run dev
- Adjust your project as needed
How do I launch a Vue project?
There are a few ways to launch your Vue project:
- Run
npm run serve
ornpm run dev
in your terminal - Add Vue with a CDN link and a
<script>
tag - Use this Vue CDN link:
<script src="https://cdn.jsdelivr.net/npm/vue@2.6.11"></script>
- Install Vue using npm
What is the command to start Vue?
To kick off a Vue 3 project, just type npm run dev
in your project's terminal. This starts the dev server so you can see your Vue app in action.