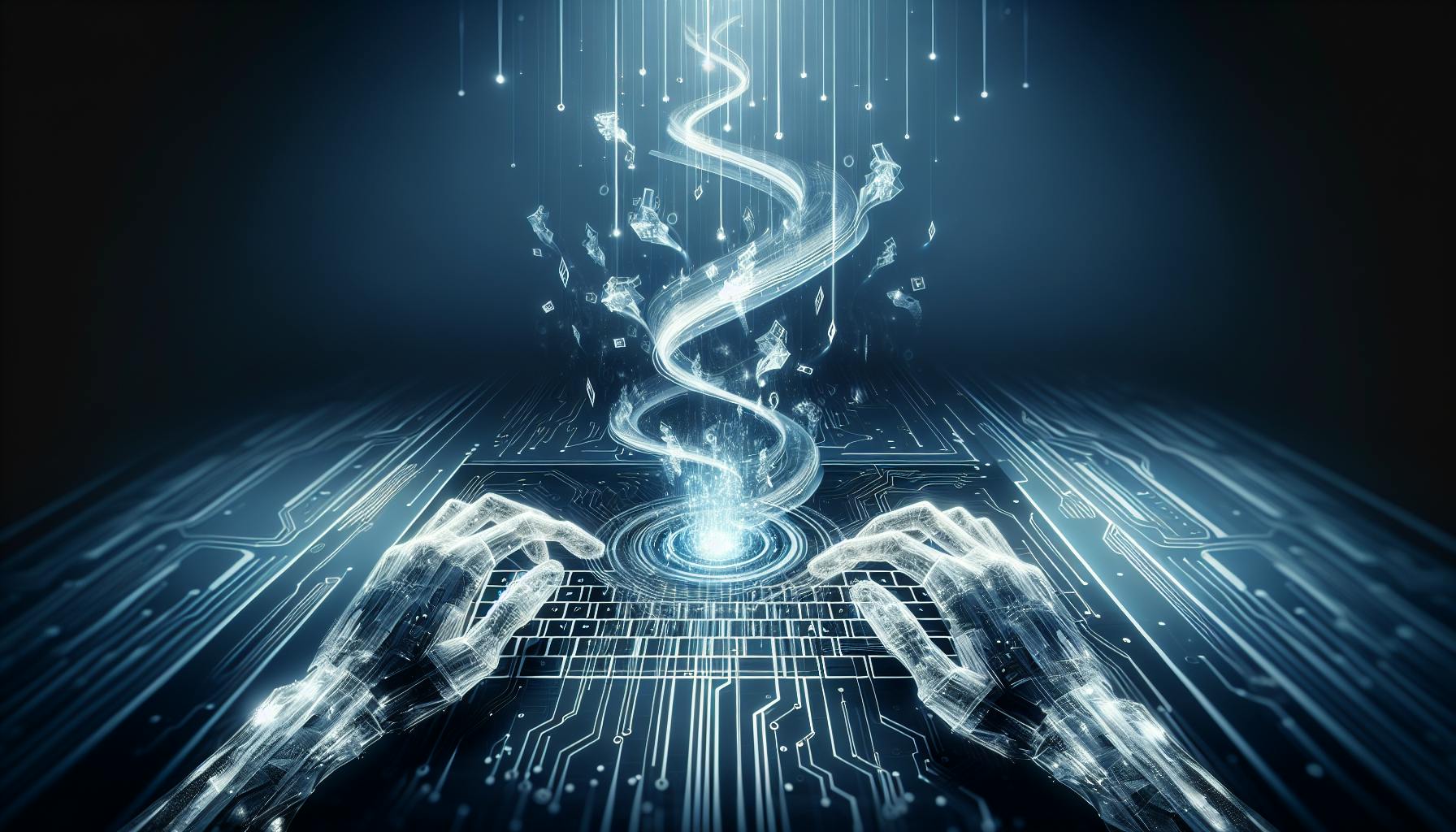
Transform code reviews into engines of clarity, consistency, and continuous improvement with key best practices. Learn actionable guidelines for structuring peer feedback, leveraging linting and metrics, and creating a culture focused on constructive collaboration.
Most software engineers would agree that code reviews are essential, but often frustrating and inefficient.
By following key best practices around psychological safety, automation, and metrics, you can transform code reviews into engines of clarity, consistency, and continuous improvement.
In this post, you'll get actionable guidelines for structuring peer feedback, leveraging linting and metrics, and creating a culture focused on constructive collaboration rather than criticism.
Introduction to Software Engineering Best Practices for Code Review
Peer code reviews are a critical practice in software engineering projects. They help teams build better quality software through collaboration, knowledge sharing, and early defect detection. This article will provide an overview of code reviews and outline essential best practices for both code authors and reviewers to enable effective reviews.
Understanding the Role of Code Review in Software Engineering
Code reviews involve a developer submitting code for their peers to review, provide feedback, and approve. Benefits include:
- Finding defects early, reducing cost of fixes
- Enforcing coding standards and best practices
- Knowledge transfer between team members
- Improving overall code quality and maintainability
Code reviews fit into the larger software development lifecycle by acting as a quality gate before changes are merged. They complement other practices like testing and pair programming.
Essential Best Practices for Effective Code Review
For reviewers, core best practices involve:
- Maintaining a collaborative, constructive mindset
- Understanding context around code changes
- Limiting scope of feedback to most critical issues
- Providing specific, actionable suggestions for improvement
- Double checking correctness of feedback given
For authors, key practices consist of:
- Adding overview comments explaining changes
- Keeping change size small and focused
- Following style guides and requirements
- Being open to feedback from reviewers
Following these practices leads to efficient, positive review experiences.
Preparing for Code Review: A Checklist for Authors
Before submitting code for review, authors should:
- Document reasons and context for changes
- Limit PR size to 300 LOC or less
- Confirm functionality with unit tests
- Check code against style guide requirements
- Annotate complex sections with comments
- Be responsive to reviewer comments
This checklist enables reviewers to provide rapid, helpful feedback.
What is an example of a software engineering best practice?
Here are 5 essential software engineering best practices to implement for high-quality code:
Clear Code Structure and Documentation
Maintaining a clear and organized code structure is essential for both you and your team. Properly structure your code into logical components, name variables intuitively, format code neatly with consistent indentation, and provide explanatory comments where needed. Create documentation that explains the architecture and intended functionality to smooth onboarding.
Version Control and Collaboration
Use Git version control to track code changes, enable collaboration, and allow rolling back when needed. Services like GitHub facilitate managing branches and pull requests so multiple developers can work together without risking the main code.
Testing and Test Automation
Comprehensive testing is crucial to ensure code works as expected before launching. Automated tests save significant time over manual testing, providing quick feedback on new code. Unit testing validates individual parts, integration testing checks combinations of components, and UI testing replicates user actions.
Performance Optimization
Profile your application to discover performance bottlenecks, then focus efforts on optimizing costly operations. Common techniques include caching repetitive computations, using efficient data structures, and avoiding unnecessary network calls.
Security and Data Protection
Apply industry standard protections like encryption for sensitive data, input validation and sanitization to prevent attacks, and access controls to limit data exposure. Keep dependencies up-to-date and integrate scanning tools to identify vulnerabilities early.
Following these software engineering best practices leads to higher quality, more reliable applications aligned with user needs. They enable catching issues early, collaborating smoothly, keeping complexity manageable, and focusing on what matters most - quickly delivering value.
What are the 4 principles of software engineering?
Software engineering relies on several key principles to ensure quality, maintainability, and efficiency in code and systems development. Here are four of the most widely adopted software engineering principles:
KISS (Keep It Simple, Stupid)
The KISS principle states that systems should be designed to be as simple as possible. Simple systems are easier to develop, test, use and maintain. Some ways to keep systems simple include:
- Avoiding unnecessary complexity in architecture and design
- Breaking large problems down into smaller, modular components
- Using simple language and minimizing jargon in code comments and documentation
DRY (Don't Repeat Yourself)
The DRY principle aims to reduce repetition in code and systems. This makes the software easier to change because there is only one place that needs editing. Ways to avoid duplication include:
- Creating reusable functions/modules/libraries
- Using variables instead of hard-coding values
- Implementing centralised storage for data/config
YAGNI (You Aren't Gonna Need It)
YAGNI recommends against implementing features or system elements until they are absolutely necessary. This avoids wasted effort and maintaining unused code. Some tips include:
- Resist planning for every possible future feature
- Wait for actual requirements before extending functionality
- Focus only on the current user needs
BDUF (Big Design Up Front)
While agile methods promote iterative design, upfront planning is still important. Taking the time to map architectures and data flows saves time down the road. Some key upfront tasks:
- Project scoping sessions
- High-level system architecture diagrams
- Data models and database design
- Defining coding standards to follow
Following principles like these leads to better quality software through reduced complexity, flexibility, easier maintenance and aligning features closely with actual user needs. They provide an excellent starting point for adopting software engineering best practices.
What are the 5 major techniques of good software engineering techniques?
Here are 5 of the most important software engineering best practices:
Iterative Development
Iterative development involves building software in small, rapid cycles rather than trying to deliver everything at once. Each iteration focuses on specific features or components that can be tested and improved. This allows for early feedback, flexibility, and continuous integration.
Incremental Development
Incremental development builds on iterative development by delivering software in small, incremental working versions rather than waiting until everything is complete. This allows features to be deployed faster and users to benefit from each upgrade.
Prototyping
Prototyping involves creating a basic mockup version of an application to visualize ideas and gather early user feedback. This is useful for validating concepts without investing heavily in development upfront.
Risk-Driven Development
Risk-driven development prioritizes high-risk elements first. By tackling complex or unclear components early, teams can identify issues sooner and have more time to resolve them.
Phase Planning and Retrospection
Detailed phase planning at the start combined with retrospection at the end of each phase helps teams evaluate progress, highlight successes and pain points, and continuously improve.
Following modern software engineering best practices leads to higher quality applications, faster delivery, better user experiences, and more productive teams. Core techniques like iterative development, incremental delivery, prototyping, and risk-driven prioritization set up projects for success.
What are some best practices for software development?
Here are some key software engineering best practices to follow for high-quality code:
Use version control
Version control systems like Git allow developers to track code changes, collaborate, and revert back if needed. Committing code frequently keeps the revision history clean.
Follow style guides
Consistency in coding styles improves readability and helps avoid errors. Style guides like Google's Python Style Guide provide standards to adhere to.
Use clear naming conventions
Well-named variables, functions, classes make code self-documenting. Avoid abbreviations and be descriptive.
Design first before coding
Creating a technical design doc allows discussion of approaches before implementation. This saves rework down the line.
Don't cram in features
Avoid scope creep. Prioritize must-have features first. Deliver iteratively instead of all at once.
Maintain staging environments
Have separate dev, test, staging environments before production to catch issues early.
Perform peer code reviews
Code reviews improve quality and spread knowledge. Use checklists to guide systematic reviews.
Following proven software engineering best practices leads to higher quality, maintainable code over the long term. What practices have you found most helpful? Let me know in the comments!
Cultivating a Culture of Collaborative Improvement through Code Review
Code review is essential for building high-quality software, enabling teams to share knowledge, find defects early, and align on best practices. However, implementing an effective review process requires nurturing a collaborative culture focused on continuous improvement.
Encouraging Consistent Participation in Peer Code Reviews
To promote active engagement in code reviews:
- Highlight benefits like learning, finding bugs sooner, and improving skills to motivate participation
- Gamify the process by tracking review metrics at team or individual levels
- Tie code review goals to OKRs to reinforce importance
- Automate reminders to review open pull requests
- Recognize top reviewers to inspire others
Establishing clear expectations around code review through team agreements and manager support is key for consistency.
Promoting Psychological Safety and Clarity in Code Review
Developing trust and comfort in giving/receiving feedback avoids unproductive debates:
- Encourage asking questions and making suggestions vs. demands
- Provide reviewer training on delivering constructive feedback
- Set norms, like assuming positive intent and avoiding harsh language
- Implement anonymous surveys to address sensitive issues
Structuring the Code Review Process for Consistency and Efficiency
Defining the workflow upfront optimizes for signal vs. noise:
- Set frequency expectations, like reviewing daily or 2x a week
- Limit change size to simplify analysis
- Require sign-off from 2 reviewers before merging
- Configure automated checks for tests, styles, security, etc.
- Use checklists outlining requirements reviewers should validate
Establishing a structured process makes code reviews more efficient while enabling collaborative learning on teams.
sbb-itb-bfaad5b
Leveraging Automation and Tools in Code Reviews
Automation and tooling can greatly enhance code reviews by ensuring consistency, simplifying repetitive tasks, and keeping all stakeholders informed. This frees up reviewers to focus on more meaningful feedback.
Implementing Linting and Static Analysis for Consistent Code Quality
- Linters analyze code to flag style issues, improving readability and consistency.
- Static analysis finds potential bugs before code review, increasing efficiency.
- By automating these checks before review, reviewers avoid wasting time on trivial issues.
Utilizing Pre-commit Hooks to Streamline Reviews
- Pre-commit hooks run tests/linters/checks on each commit.
- Blocking unfinished work from being committed saves reviewers' time.
- Reviewers can focus reviews on functionality rather than half-finished tasks.
Automating Review Reminders and Notifications for Timely Feedback
- Bots can track review progress and send reminders about pending reviews.
- Notifications upon review completion let authors know to resume work.
- This automation ensures issues don't get stalled and work moves forward.
Consistently applying linters, static analysis, pre-commit hooks and review bots/notifications helps optimize peer code reviews. By handling trivial, repetitive tasks automatically, these tools enable reviewers to focus their efforts on providing meaningful, actionable feedback to improve overall code quality.
Measuring Success with Key Metrics in Code Reviews
Tracking Code Review Coverage for Consistent Practice
Review coverage refers to the percentage of code changes that undergo peer review before being merged. This metric indicates whether teams are consistently applying code reviews across all projects.
To track review coverage:
- Enable code review workflows in version control systems like GitHub or GitLab
- Set policies requiring reviews before merging code
- Run periodic reports on review rates
High review coverage ensures code quality, knowledge sharing, and alignment with best practices. Teams should aim for at least 90-100% coverage for optimal collaboration. Lower rates may indicate inconsistent practices across teams or projects.
Analyzing the Number of Issues Found Through Reviews
The number of defects, bugs, security flaws and other issues identified during code review highlights the quality benefits. Quantifying these provides metrics like:
- Defects found per review
- Defect removal efficiency
- Number of critical defects prevented
Tracking defects found over time also shows whether:
- Review practices are improving and finding more issues
- Overall code quality is improving with fewer defects introduced
Effective code reviews should find at least 3-5 minor issues and 0.5 critical issues per 100 lines reviewed. Higher rates indicate opportunities to improve coding practices.
Optimizing Review Turnaround Time for Efficient Collaboration
Tracking the time from initiating a review to completion identifies inefficiencies. Potential metrics include:
- Average review cycle time
- Percentage of delayed reviews
- Review wait times
Optimizing cycle times ensures timely feedback to developers. Goals depend on team norms but 1-2 days is ideal for most.
Bottlenecks like overloaded reviewers, inactive requests or lack of reviewer specialization can cause delays. Analyzing turnaround times highlights these issues for process improvements.
Faster reviews enable faster development cycles for greater productivity. Tracking review velocity - issues reviewed per unit time - also quantifies productivity gains.
Navigating Common Pitfalls in Code Reviews
Code reviews are a critical practice for producing high quality software, but they can also create bottlenecks if not managed properly. Here are some tips for streamlining the review process while still ensuring code clarity and consistency:
Preventing Reviews from Becoming Bottlenecks
- Implement lightweight reviews for small changes: Quick 1-2 line code tweaks often don't need a full review. Use discretion for what truly needs an in-depth critique.
- Distinguish required and optional reviewers: Mark which reviewers must sign-off for approval versus those who can provide optional feedback.
- Automate parts of the workflow: Use integrations with project management and code review tools to automatically transition issues through review stages.
- Set expectations upfront: Establish an SLA per review type so authors know how quickly to expect a response. Track reviewer performance and revisit agreements if issues arise.
Ensuring Clarity and Context in Code Review Feedback
- Explain reasoning behind requests: Reviewers should share why they are suggesting a change, not just what to change. Understanding the why improves the author's skills.
- Provide reference material: Include links to style guides, best practice docs, or examples to support feedback requiring substantial changes.
- Prioritize actionable feedback: Focus reviews on fixes that can be reasonably accomplished within project scope and timeline. Don't let perfection become the enemy of progress.
Balancing Style and Substance in Code Review Critiques
- Lead with functional critiques: Focus first reviews on correctness - does the code work as intended? Only after meeting functional goals examine style preferences.
- Create consistency through automation: Use linters and formatters to programmatically enforce stylistic conventions rather than manual nitpicking.
- Separate subjective from objective guidelines: Clarify which standards are subjective preferences versus objectively critical. Focus reviews on the latter.
Following these best practices will help streamline review workflow while still improving code quality and consistency through meaningful, collaborative critiques. The end goal is to facilitate progress, not impede it.
Addressing Specialized Review Scenarios in Software Engineering
Best Practices for Reviewing Prototypes and Spike Solutions
When reviewing prototype or spike code, it's important to keep in mind that this code is meant to be experimental and test out concepts quickly. Here are some tips:
- Focus the review on the core concepts and approach rather than code quality. Prototype code often lacks tests, documentation, and polish.
- Validate that the prototype proves or disproves the original hypothesis. Make sure it fully explores the problem space.
- Assess whether the prototype sets up the project for future success. Will it be easy to iterate on and improve?
- Look for hardcoded data, shortcuts, and technical debt that should be cleaned up before moving to production.
- Determine if the prototype uses appropriate libraries/frameworks or if alternatives should be explored.
- Make sure the developer documents key learnings and next steps coming out of the prototype.
Managing Community and Open Source Contributions
Reviewing code from unknown external developers poses additional challenges:
- Thoroughly inspect for potential security vulnerabilities like SQL injection, XSS, etc.
- Validate adherence to project conventions and standards. Linting and static analysis can help.
- Assess whether contribution aligns with project roadmap and goals. Politely decline if not a fit.
- Check contributor's reputation on platforms like GitHub. Are they known/trusted?
- Start small with easier tickets to establish trust before tackling more complex tasks.
- Provide friendly, constructive feedback focused on improving code rather than criticizing.
Conducting Rigorous Security and Privacy Reviews
For sensitive code, specialized security reviews are essential:
- Utilize automated scanners (SAST, DAST) to surface vulnerabilities.
- Rigorously inspect authentication, access controls, encryption, data handling.
- Validate sanitization of inputs/outputs to prevent XSS, code injection etc.
- Adhere to OWASP Top 10 and platform-specific best practices.
- Conduct additional manual reviews focused solely on security.
- Consider independent audits from professional security consulting firms.
- Establish bug bounty program incentivizing external researchers to responsibly disclose vulnerabilities.
Regularly reviewing security-critical modules saves enormous headaches down the line. Prioritizing developer education on secure coding principles further strengthens protection.
Embracing Continuous Improvement in Code Review Practices
Soliciting Meta-feedback for Code Review Enhancement
Gathering feedback from both code authors and reviewers after each review can provide valuable insights into what's working well and what can be improved. This helps align practices with software engineering best practices over time.
Here are some tips:
-
Set up a lightweight feedback process - perhaps a quick survey or discussion at the end of each review. Don't let it become burdensome.
-
Ask questions like:
-
What did you appreciate about this review process?
-
What would have made this review more effective for you?
-
What changes would you suggest for future reviews?
-
Track feedback trends in a shared doc to inform ongoing enhancements.
Soliciting meta-feedback ties into principles of continuous improvement and keeping processes nimble. It enables teams to regularly tune reviews based on experience.
Fostering a Shared Approach to Lessons Learned
Encourage sharing key learnings, successful practices, and pitfalls between teams across the organization. This helps:
- Propagate software engineering best practices more broadly
- Increase clarity and consistency in reviews org-wide
- Build understanding of what works well for collaborative improvement
Consider venues like:
- A regular "code review community chat"
- An internal site highlighting team review practices
- Lightweight post-mortems on major initiatives
Fostering this shared dialogue helps align review approaches over time per core principles of software engineering practice.
Ongoing Tuning of Review Practices for Peak Performance
Regularly evaluate existing code review policies and processes against goals. Update them as needed to reflect evolving software engineering practices and principles.
Key aspects to revisit quarterly/annually:
- Review checklist/templates
- Automated analysis tools
- Training for reviewers
- Incentive structures
- Performance metrics
Updating policies prevents reviews from becoming rote. It helps incorporate software engineering best practices 2023 for clarity, consistency and impact.
Keeping reviews fresh and aligned with goals yields better code quality plus reviewer engagement over time.
Conclusion: Integrating Best Practices into Your Review Process
To nurture an effective and collaborative code review culture focused on quality and continuous improvement, integrate the following software development best practices into your process:
Establish Clear Guidelines
- Define code review objectives, scope, and processes upfront to set consistent expectations. Outline required checks, testing, documentation etc.
- Use checklists outlining best practices for what to look for in reviews. Include code quality, security, performance, accessibility etc.
- Standardize style guides to enable consistency across projects and codebases. Enforce linting and formatting.
Optimize Workflow
- Automate parts of review workflow for efficiency e.g. testing, linting. Allows focus on logic and architecture.
- Use specialized code review tools with features like inline comments, version control integration, analytics etc.
- Schedule regular review meetings for open discussion and to streamline volume.
Promote Constructive Feedback
- Frame feedback neutrally in terms of improvements, not personal judgement.
- Provide actionable suggestions on better approaches, not just what's "wrong". Offer mentoring.
- Recognize reviewer effort and constructive input. Reward finding issues early.
Integrating these practices provides clarity for all involved, while enabling collaborative improvement of code quality on an ongoing basis. The end result is higher performing software delivered faster.