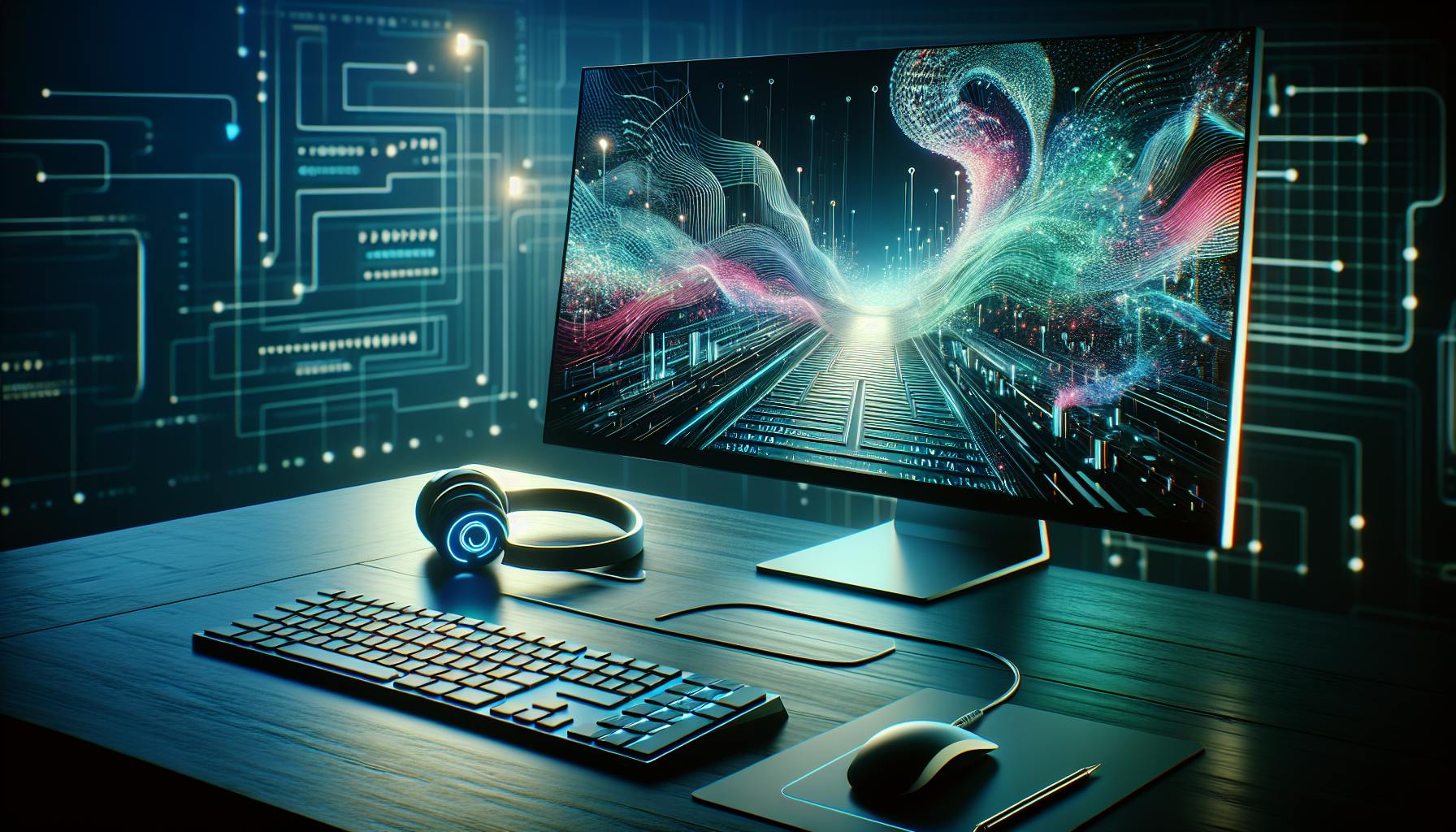
Learn how to translate programming languages effectively and efficiently, the importance of portability, reusability, and access to libraries, and the significance of syntax and semantics.
If you're curious about how to translate programming language from one to another, you've landed in the right place. This guide simplifies the process, whether you're moving code from Python to JavaScript for a web app, or from C++ to Java for cross-platform compatibility. Here's a quick rundown:
- Understanding Programming Languages: Discover what makes each programming language unique and why syntax and semantics matter.
- The Translation Process: Learn the difference between translation, compilation, and interpretation, and how tools like translators and compilers fit into the picture.
- Practical Guide to Translation: Step-by-step instructions using Python and the 'translate' library to translate text.
- Common Challenges: Tackling the syntax and semantics differences between languages and strategies to overcome them.
- Differentiating Translation and Porting: Clarifying the distinctions between making software work in a new place (porting) versus changing its programming language.
This introduction aims to give you a clear overview of what to expect and how to start translating programming languages effectively and efficiently.
Why is it Important?
Here are a few big reasons why changing programming languages is a big deal:
- Portability - It lets your code work on various platforms. Turning C++ into JavaScript, for example, lets you make web apps.
- Reusability - You can use existing code in a new language, saving lots of time and effort.
- Access to Libraries/Frameworks - Different languages have cool tools and helpers. Changing languages means you can use these tools across your projects.
- Performance - Some languages, like C++, are really fast. Changing to these languages can make your software run quicker.
- Productivity - Programmers can work in their favorite language, then change the code to run elsewhere. This makes things more efficient.
What You Will Learn
In this guide for beginners, you'll learn about:
- The basics of changing programming languages
- How translators, compilers, and interpreters help with this
- Steps to change code from one language to another
- Common problems and how to solve them
By the end, you'll know how to change code between languages to reuse software, make it work in more places, and use the best parts of different languages.
Understanding Programming Languages
Programming languages are special codes that let us tell computers what to do. They come with their own set of rules about how to write and structure that code, so the computer can understand it.
What Are Programming Languages?
Think of a programming language as a toolkit for chatting with computers. It has its own way of arranging words and symbols (syntax) and a set of rules (semantics) to make sure both you and the computer are on the same page. Here’s what’s important to remember:
- They use special words, symbols, and rules to create a set of instructions.
- They make it easier for us to write code that computers can run, instead of using complex binary code.
- They include tools for organizing and running programs, like loops and conditions.
- They work on different types of computers and devices, not just one.
Basically, programming languages help us give clear instructions to computers in a way we can easily understand.
How Do They Compare to Natural Languages?
Unlike English or other natural languages, programming languages have to be super precise. There’s no room for guesswork because computers need clear instructions. Here are the main differences:
- Syntax - Programming languages have strict rules about how to write code. Natural languages are more flexible.
- Ambiguity - Computers can get confused by unclear instructions, so programming languages need to be straightforward.
- Literality - Programming languages mean exactly what they say, without any hidden meanings or tones.
- Flexibility - You can’t play around with the structure of programming languages as much as you can with spoken or written languages.
Even though they’re different, both kinds of languages rely on clear rules and meanings to make sense.
The Significance of Syntax and Semantics
Syntax and semantics are the backbone of programming languages:
- Syntax is all about how code looks. It’s the rulebook for writing code correctly, making sure it’s organized and makes sense.
- Semantics is about what the code actually does. It’s the part that explains how different bits of code work together.
Getting both of these right is crucial. If you mess up the syntax or semantics, the computer might not understand what you’re trying to tell it to do.
The Translation Process
This part talks about what it means to translate programming languages. It also explains the difference between translation, compilation, and interpretation, and what roles translators, compilers, and interpreters have.
What is Translation in Programming?
Translation in programming is like changing a book from one language to another while keeping the story the same. It means taking code from one programming language (like Python) and turning it into another language (like Java), making sure it does the same thing.
Here are the main points:
- It changes code from languages like Python, Java, or C++ into another.
- The original code and the new code should work the same way.
- It focuses on what the code does, not just how it looks.
- Tools help change the code from one language to another.
How is it Different from Compilation and Interpretation?
Here's how they differ:
- Compilation: Changes your code into a language the computer (CPU) understands directly.
- Interpretation: Runs your code step-by-step without changing it first.
- Translation: Changes your code from one human-like language to another.
So, while compilation is about making code the computer can use directly, and interpretation is about running the code as is, translation is about changing code from one language to another.
Understanding Translators, Compilers, and Interpreters
These are the tools that help us work with code:
- Translators: Change code from one language to another.
- Compilers: Change your code into something the computer can run.
- Interpreters: Run your code one step at a time.
Translators help change code between languages like Python, Ruby, or Java. Compilers turn your code into a form that the computer's CPU can run. Interpreters let you run your code directly, making it easy to test and use.
Practical Guide to Translation
This part of the guide will walk you through how to use Python and a tool called 'translate' to change a simple sentence from English to French.
Installing Python and Setting Up an Editor
First, let's get Python and a place to write code ready:
- Head over to python.org and grab the latest Python version for your computer. Remember to tick the box to make Python available everywhere on your system.
- Pick a code editor like Visual Studio Code (VS Code) or Atom. They're free and great for coding in Python.
- After opening your chosen editor, add the Python extension. This gives you tools and support to make coding easier.
Now, you're all set with Python and a code editor!
Importing the 'translate' Library
To start translating, we need to bring in a special tool from the 'translate' library. Here's how:
from translate import Translator
This line of code lets us use the Translator
to do translations.
Writing and Running Sample Translation Code
Now, let's translate "Hello World!" from English to French:
from translate import Translator
translator = Translator(to_lang="fr")
text_to_translate = "Hello World!"
translation = translator.translate(text_to_translate)
print(translation)
Here's what happens:
- We get the Translator ready
- We tell it we want to translate to French
- We pick the English sentence we want to change
- We use
translate()
to get it translated - We show the French translation
Running this code will give you "Bonjour monde!" which is "Hello World!" in French.
Using the translate
library, we can easily switch between languages with just a few lines of code. Now you can try more translations and maybe even build bigger projects with it.
sbb-itb-bfaad5b
Common Challenges and Solutions
This part talks about the big problems you might face when changing code from one programming language to another, especially with syntax and semantics. We'll also look at ways to solve these problems.
Syntax and Semantics as Key Challenges
Sometimes, the rules for how to write code (syntax) or what code means (semantics) in the language you're changing to can be very different from your original language.
For instance, Python uses spaces to organize code, but languages like JavaScript use curly braces {}
. If you try to directly change Python code into JavaScript, the spacing won't work right.
Also, some things you can do in Python might not work the same way in another language. This means the translator has to make sure the code still does what it's supposed to do, even if it looks different.
The main problems come from these differences in rules and how concepts are used. The translator's job is to figure out how to connect these differences.
Strategies to Overcome Challenges
Here's how people usually deal with these problems:
- Carefully compare the two languages - Look at the differences in how they're written and what they can do. This helps find out where problems might pop up.
- Fix things by hand after translating - Sometimes, automatic tools can't catch everything, so you might need to go in and make some changes yourself.
- Know what the translator can and can't do - If you understand the tool's limits, you can step in and help only where it's really needed.
By looking closely at the differences between languages, making manual adjustments when necessary, and knowing what your tools can handle, you can keep the code working right even when changing it from one language to another.
Differentiating Translation and Porting
Code Porting in Software Development
Porting is about making your software work on a different system or device than it was originally made for. Here’s what it usually involves:
- Changing the code so it can run on various operating systems like Windows, Linux, or macOS. This might mean tweaking parts of the code that only work on one OS.
- Updating the software to be compatible with newer versions of programming languages or frameworks. For instance, moving a project from Python 2 to Python 3.
- Adjusting the software so it can work on different devices, like smartphones or special hardware.
Porting is mainly about making sure the software can run in a new place without changing what the software does. It’s a way to use the same code in more places.
Comparison to Programming Language Translation
Code Porting | Language Translation |
---|---|
Focus on making it work in a new place | Just changes the programming language |
Might tweak how the software is built | Keeps what the software does the same |
Results in software that works in a new environment | Results in software written in a new language |
Porting is about adjusting software to run on different systems or devices, while translation is about changing the code from one programming language to another, like turning Python code into Java.
Porting might mean making some big changes to how the software is set up, but translation tries to keep the software doing the same thing, just in a different language. In short, porting is about the where, and translation is about the how.
Conclusion
Summary of Key Points
- Translation is about changing code from one programming language to another. This makes it easier to use the same code in different ways.
- It's important to look at how each language is set up (syntax) and what the code means (semantics) because they can be quite different.
- Tools like translators and compilers help us do this job.
- For beginners, using Python's 'translate' library is a good way to start learning about translation.
Moving Forward
Now that you know the basics of translating between programming languages, here are some ideas to keep learning:
- Dive deeper into how to move software or tools to new systems, which is a bit like translation but more involved.
- Look into the latest tools and ways to automatically translate code from one language to another.
- Check out projects that use multiple programming languages to see how they tackle translation challenges.
With the basics under your belt, you're ready to explore more about how translation works and what you can do with it.