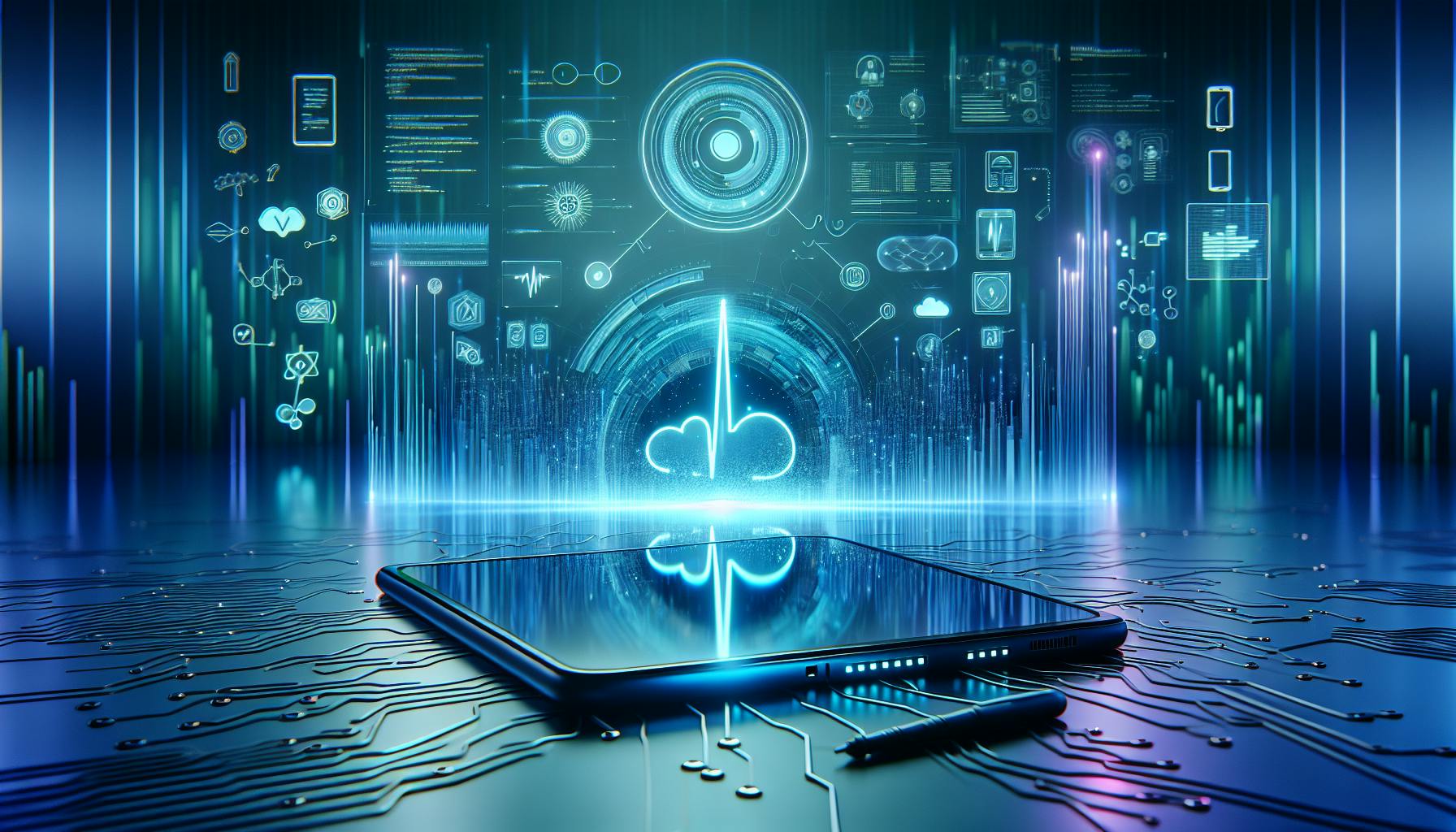
A comprehensive guide to WebRTC on Chrome, from setup to optimization. Learn about real-time communication, security measures, and applications in healthcare, business, and education.
WebRTC (Web Real-Time Communication) on Chrome allows developers to create applications for live audio, video, and data sharing directly in the browser, without needing extra software. Here's a straightforward guide to understanding, implementing, and optimizing WebRTC in Chrome:
- What is WebRTC?: A tool for real-time communication including video chats, voice calls, and file sharing within web browsers.
- Key Features of WebRTC on Chrome: Built-in support, automatic updates, developer tools, and compatibility with the latest WebRTC features.
- Setting Up Your Development Environment: Requirements include a computer with Windows, MacOS, or Linux, Git, a text editor like Visual Studio Code, Node.js, npm, and Google Chrome or Chromium browser.
- Implementing WebRTC: Basics involve accessing the camera and microphone, setting up peer connections, and handling data channels for direct communication.
- Debugging and Optimization: Chrome’s
chrome://webrtc-internals
tool helps identify and solve issues, with tips for enhancing performance. - Security Measures: Encryption, permissions, sandboxing, and code integrity ensure secure communication.
- Real-world Applications: WebRTC is used in healthcare, business communications, education, and more for efficient and flexible real-time interactions.
This guide aims to provide developers with the essential knowledge for integrating WebRTC into their Chrome applications effectively.
What is WebRTC?
WebRTC stands for Web Real-Time Communication. It's a tool that lets you chat, video call, or share files directly in your web browser without needing to download anything extra.
Here's what WebRTC can do:
- Let you video chat or talk in real-time
- Use your camera and microphone to capture video and audio
- Send files and messages quickly between people
- Work even when there are barriers like firewalls
WebRTC makes all this happen right in your web browser, using something called JavaScript APIs, so you don't have to install special software.
How WebRTC Works
WebRTC lets you communicate in real-time using three main tools:
- MediaStream API - This lets you use your camera and microphone.
- RTCPeerConnection API - This makes it possible to call or video chat by sending media and data between browsers.
- RTCDataChannel API - This is for sending files and messages directly to someone else.
These tools work together to let you capture video or audio, talk or share with someone else, and send files or messages.
WebRTC and Chrome
Chrome is really good at working with WebRTC because:
- It has built-in support for WebRTC, making it easier for developers.
- It keeps updating to make sure it has the latest WebRTC features.
- It has tools like chrome://webrtc-internals to help developers see how things are working.
- More and more Chrome extensions and Progressive Web Apps (PWAs) are using WebRTC.
- It can automatically switch to simpler features if someone's using an older browser.
Because Chrome updates on its own, developers can trust that they're always using the newest version of WebRTC for their websites and apps.
Setting Up Your Development Environment
Prerequisites
Before you start making apps that use WebRTC on Chrome, you'll need a few things:
- A computer that runs on Windows, MacOS, or Linux
- Git and a GitHub account to store your code online
- A text editor like Visual Studio Code for writing your code
- Node.js and npm (npm comes with Node) for running your code
- The Google Chrome or Chromium browser to test your apps
Some optional but useful tools include:
- Chrome Canary to try out new features before they're widely released
- ngrok for testing your app on your own computer with turn servers
- A WebRTC test service account for trying out WebRTC features
Installing Necessary Tools
Here's how to get ready for WebRTC development on Chrome:
1. Install Git
- First, download and set up the latest version of Git from git-scm.com
2. Sign Up for GitHub
- Make a free account on GitHub.com. This is where you'll keep your code.
3. Download a Text Editor
- Visual Studio Code is a good, free editor for WebRTC projects. Grab it from code.visualstudio.com.
4. Install Node.js
- Get the 'Recommended for Most Users' version of Node.js from nodejs.org. It comes with npm, which you'll need.
5. Install Google Chrome
- Download the regular version of Chrome from google.com/chrome.
6. (Optional) Install Canary Channel
- For early access to new Chrome stuff, download Canary from google.com/chrome.
7. (Optional) Set Up ngrok
- ngrok is handy for testing your app with TURN servers right on your computer. Get it from ngrok.com.
Now you're ready! With these tools, you can start creating WebRTC projects that work great on Google Chrome.
Basic WebRTC Implementation on Chrome
WebRTC lets you chat, share videos, and send data to others right in your web browser, without needing any extra software. Chrome supports WebRTC really well, keeping up with the latest changes and providing tools to help you check on your apps. This part talks about how to make a simple WebRTC app in Chrome and how to use Chrome's tools to fix any problems.
Creating Your First WebRTC App
To make a simple WebRTC video chat app, you'll:
- Use
getUserMedia()
to access the camera - Set up a connection between two users with
new RTCPeerConnection()
- Share ICE candidates with
onicecandidate
to help the connection - Make an offer with
createOffer()
and use it as the local description - Send this offer to the other user over a signaling channel
- Use the offer you got from the other user as the remote description
- Reply with
createAnswer()
and use it as your local description - Send this answer back over the signaling channel
- Add your video tracks to the connection with
addTrack()
- Show the other user's video when it comes in with
onaddstream
Check out this simple video chat guide for the complete code.
Debugging with Chrome’s WebRTC Internal Tool
Chrome v87+ has a special page called chrome://webrtc-internals
for checking on your WebRTC calls:
- Open a new tab and go to
chrome://webrtc-internals
while you're in a WebRTC call - You can see info about the video and audio, how the connection is set up, and more
- Find problems like when videos won't play, calls won't connect, or videos are slow
- Click "Download the PeerConnection updates and stats data" to save all the details for looking into later
- Look at things like
googTrackId
,googCodecName
, andpacketsLost
to figure out problems with video quality or connection issues
If you need more help, the WebRTC Troubleshooter is a good place to start.
Advanced WebRTC Features
Signaling and Peer Connections
Think of signaling like handing notes in class to set up a secret chat. It's how two devices agree to start talking using WebRTC. They use a special server to exchange these notes, which include details like 'Here's how you can reach me.' Common ways to send these notes include using WebSocket or XMPP.
For setting up the connection, you share ICE candidates, which are like addresses that help get through firewalls, and SDP descriptions that tell what kind of video or audio you can send.
Here are some simple steps to follow:
- Pick a signaling method like WebSocket to exchange messages.
- Make sure you can handle making and receiving offers to start the connection.
- Be ready for errors and have a plan to reconnect if needed.
- Use TURN servers to help with tough connections and try to avoid long waits.
Handling Media Streams
The MediaStream API lets you use your camera and microphone. You can ask for specific things like video quality or which camera to use.
Here are some easy tips:
- Choose the right settings for your video, like how clear it should be.
- You can turn the sound on or off as needed.
- Add or remove video or audio when you want.
- Keep an eye out for when new devices like cameras are connected.
- Check how it works on phones too.
Data Channels
The RTCDataChannel API is for sending messages or files directly between users. It's great for chat apps or sharing files.
Some advice for data channels:
- Decide if you need a super reliable connection or if it's okay if some messages don't make it.
- Think about how big your messages can be.
- Be ready for when the channel opens, closes, or has an error.
- Make sure it works on different browsers and phones.
- Remember, phones might have some limits.
WebRTC Security on Chrome
WebRTC takes several steps to protect your chats and data on Chrome. Here's a look at the main security features:
Encryption
Everything in WebRTC on Chrome is locked down with encryption. This includes:
- SRTP - Keeps your video and audio chats private from start to finish.
- DTLS - Makes sure only the right devices can unlock the conversation.
- HTTPS - A secure way to use WebRTC, making sure no one else can sneak a peek.
All these work together to make sure no one can listen in or watch your chats.
Permissions
Chrome asks you first before letting websites use your camera or microphone. You'll always see a clear signal when your devices are being used.
Sandboxing
WebRTC runs in a safe space in Chrome, keeping it away from other parts of your computer. This helps keep things secure. Chrome also updates itself to fix any security holes.
Code Integrity
Since WebRTC's code is open for anyone to check, security experts can spot and fix issues fast. Chrome's updates take care of these fixes quickly.
Signaling Flexibility
For setting up calls, developers need to pick secure ways to connect, like WebSocket or XMPP. This makes sure the setup chat is safe too.
Additional Controls
Chrome lets you turn off WebRTC or clear any WebRTC-related data. This means you have more control over your privacy.
With all these security steps, Chrome makes WebRTC safe to use. But, developers also need to do their part by using secure connections and handling permissions and data correctly.
sbb-itb-bfaad5b
Optimizing WebRTC Applications for Chrome
Performance Best Practices
To make your WebRTC apps work better and faster on Chrome, try these tips:
- When setting up connections between users, pick the best video formats that work smoothly on most devices, like VP8 or H.264.
- Make sure the video quality matches what the user's internet can handle. It's better to start with lower quality and then go higher if possible.
- Turn off any parts of the video or audio you're not using to help save on computer power and internet usage.
- When your app isn't being used much, slow down the connection to save resources.
- Choose settings that only look for nearby connections to make things run faster and smoother.
- It's smarter to use one connection for sending data instead of setting up many.
- Keep an eye on the Chrome tool
chrome://webrtc-internals
to see how your app is doing and make adjustments.
Troubleshooting Common Issues
If you run into problems with WebRTC on Chrome, here's how to fix them:
- Make sure the website has permission to use your camera and microphone. You can check your devices at test.webrtc.org.
- If you're having trouble connecting, look at the connection details in
chrome://webrtc-internals
. - Make sure the video formats you're using work well together by checking their details.
- If your app is slow or not working right, look at the performance info in Chrome's tool.
- Check if your internet setup and security settings are blocking the connection.
- Try turning off other browser add-ons or using private browsing to see if there's a conflict.
- If the app acts differently on various versions of Chrome, compare the browser details.
- For a deeper dive, look at the error messages and connection data in Chrome.
Sticking to these guidelines and keeping an eye on Chrome's diagnostic tools will help you avoid common issues with WebRTC.
Real-world Applications and Case Studies
WebRTC is being used in lots of different ways out in the world, showing us how useful it can be. Here are some examples of where you might see it:
Healthcare
-
Telehealth Platforms: Doctors and patients are meeting over video calls through WebRTC on sites like Doxy.me. This makes check-ups easy and safe from home.
-
Remote Patient Monitoring: WebRTC helps send health data, like blood pressure readings, straight to doctors. This is great for patients who need regular care but can stay at home.
Business Communications
- Video Conferencing: Tools like Whereby use WebRTC so teams can have video meetings right in their web browser. This includes sharing screens and chatting, making teamwork easier.
- Contact Centers: Customer service gets better with WebRTC. Companies use it to let you talk to support directly through their website, no phone needed.
Education
- Virtual Classrooms: With WebRTC, online classes feel more like the real thing. Teachers can use video, interactive whiteboards, and even split students into groups for projects.
- Peer Learning: Students can work together on projects or study with tutors online, thanks to WebRTC. It doesn’t matter where everyone is located.
IoT and Robotics
- Real-Time System Monitoring: WebRTC connects to sensors in devices, letting technicians check on things like appliance performance from anywhere.
- Telepresence Robots: These robots use WebRTC for video calls. They help people who work remotely feel like they’re right there with their team.
These examples show how WebRTC is changing the way we communicate across different areas. It’s making things more direct and flexible, whether for work, school, or healthcare.
Beyond the Basics
Integrating with Other Technologies
WebRTC works well with lots of other web tools, making it possible to build even cooler apps. Here are a few ways you can mix it up:
Web Audio API
- Add sound effects or reduce background noise in audio chats
- Show sound levels or patterns
- Connect audio from different sources
WebSocket API
- Help set up and manage calls by exchanging important info quickly
- Send messages fast while you're also sharing video or audio
MediaStream Recording API
- Lets you record videos, audio, or screen shares to a file
- Perfect for making tutorials or videos you can share
Media Stream Image Capture API
- Take pictures from a video
- Create your own video effects or filters
WebGL API
- Add cool visual effects to videos
- Make 3D spaces where people can chat with audio and video
By using WebRTC with these technologies, developers can create all sorts of new and exciting real-time apps.
Future of WebRTC on Chrome
WebRTC is always getting better, and Chrome is keeping up. Here's what's coming:
VP9 video codec
- A new way to compress videos so they look clear but don't take up much space
- It's an upgrade from VP8 and doesn't cost anything to use
Support for ORTC APIs
- Another way to control media in your apps
- Gives you more detailed control
Improved hardware access
- More ways to control your camera, like focus and zoom
- Support for more advanced video features
Simulcast and SVC support
- Send videos in different qualities
- Helps keep video smooth even if the internet connection changes
WebTransport protocol
- A new way to move data that's faster and more secure
- Might replace WebSockets for some things
Continued spec alignment
- Keeping up with the latest WebRTC standards
- Making sure Chrome works well with other browsers
Google is helping shape the future of WebRTC by working with groups that set these standards. This means Chrome will keep being a great place for WebRTC apps.
Conclusion
Using WebRTC with Chrome lets developers make web apps where people can chat, share videos, or send files to each other directly in the browser, without needing extra software. In this guide, we went over the basics, some tools, and tips on how to do this well.
In short, WebRTC lets people connect directly for live audio, video, and data sharing, using important tools like MediaStream, RTCPeerConnection, and RTCDataChannel.
Chrome is a great browser for WebRTC because it updates often and has tools to help developers check their work. Once you're set up, you can make simple video chat apps. You can use features like getUserMedia to access cameras and microphones, and RTCPeerConnection to share media between people.
For bigger projects, WebRTC lets you manage how devices connect, send data reliably, and keep everything secure. We saw how WebRTC is used in different areas like health care, business, and education, showing its versatility.
Looking ahead, Chrome will keep improving, adding support for new video types, better ways to connect, and more control over devices.
By using WebRTC and Chrome's features, developers can create new and exciting ways for people to communicate online. This guide covered the basics, but there's a lot more to learn and try out.
Related Questions
How do I use WebRTC in Chrome?
To use WebRTC in Chrome, simply follow these steps:
- Type
chrome://flags/#enable-webrtc
into the address bar and hit Enter. - Look for the 'WebRTC' section to turn WebRTC features on or off.
- Hit 'Relaunch' to apply any changes you've made.
You can also test WebRTC by visiting test.webrtc.org or trying out web apps that use WebRTC.
Does Google support WebRTC?
Yes, Google helped create WebRTC and included it in Chrome back in 2013. Google made WebRTC open-source and works on making it better. Now, many browsers use WebRTC for things like video calls right in your web browser.
Is WebRTC still used?
Absolutely, WebRTC is becoming more popular. It's behind the video, voice, screen sharing, and file sharing in tools like Google Meet, Microsoft Teams, and Facebook Messenger. It's also used in healthcare, live streaming, and connecting devices for real-time communication.
How do I debug Chrome WebRTC?
To find and fix problems with WebRTC in Chrome, go to chrome://webrtc-internals
. This page shows detailed info about your WebRTC connections, like the video and audio data being sent. For more help, the WebRTC Troubleshooter site has tools for debugging. Remember to turn on full logging to get detailed information on any issues.