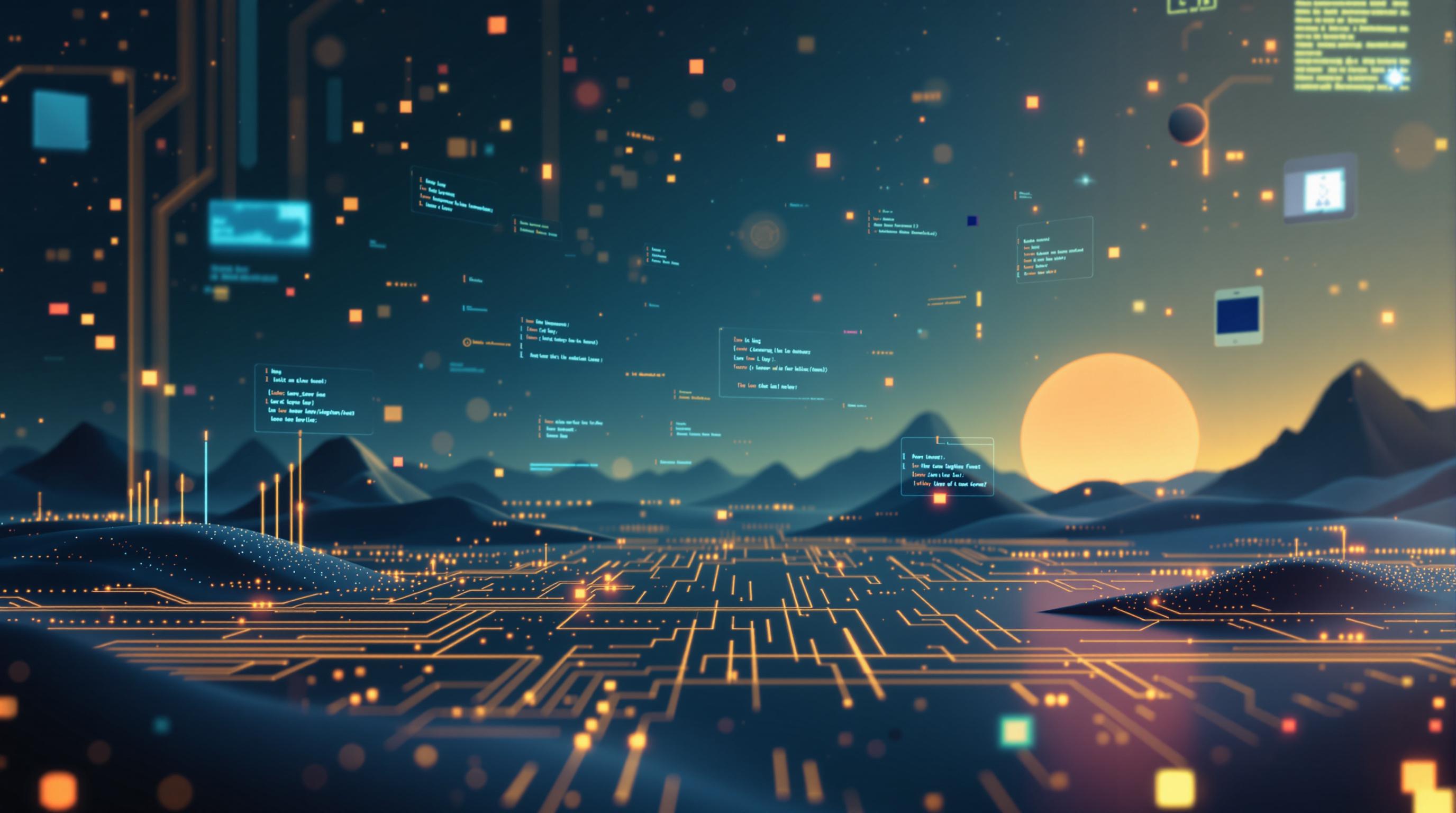
Zig 0.14.0 introduces significant upgrades like Incremental Compilation, improved memory tools, and enhanced platform support, driving developer efficiency.
Zig 0.14.0 is here! This release introduces major upgrades like Incremental Compilation, a faster x86 backend, and improved memory management tools. Over nine months, 251 contributors made 3,467 commits to deliver these changes.
Key Highlights:
-
Faster Development:
- Incremental Compilation reduces reanalysis time (e.g., 500K lines: 14s โ 63ms).
- Multithreaded backend cuts compiler build times (12.8s โ 8.57s).
-
Build System Updates:
- File System Watching for automatic rebuilds.
- New, readable package hash format.
-
Programming Enhancements:
- Labeled switch statements for state machines (+13% tokenizer performance).
- New
@branchHint
for finer performance control.
-
Standard Library Improvements:
- Memory tools: DebugAllocator (leak detection) & SmpAllocator (multi-threaded).
- ZON (Zig Object Notation) support for data serialization/deserialization.
-
Platform Support:
- Updates for Linux, macOS, Windows, and WebAssembly.
Quick Comparison:
Feature | Zig 0.14.0 Updates | Impact |
---|---|---|
Compilation Speed | Incremental Compilation, x86 backend | Faster edit/compile/debug cycles |
Memory Management | DebugAllocator, SmpAllocator | Leak detection, efficient resizing |
Build System | File Watching, new hash format | Easier, faster project builds |
Cross-Platform | Linux, macOS, Windows improvements | Broader compatibility |
Zig 0.14.0 emphasizes performance, control, and efficiency, making it a powerful choice for modern developers. Ready to try it? Download now!
Zig Incremental Compilation with Jetzig
Main Features in 0.14.0
Build System Updates
Zig 0.14.0 introduces updates to its build system that make development smoother and more efficient. A key addition is File System Watching, which automatically rebuilds projects whenever source files are modified. This feature monitors only the directories that matter, keeping system resource usage low.
The package management system has also been revamped with a new hash format that includes detailed metadata. Unlike the old, hard-to-decipher strings (e.g., "1220115ff095a3c3..."), the new format is easier to read and understand:
Hash Component | Example Value | Description |
---|---|---|
Name | mime | Identifies the package |
Version | 3.0.0 | Semantic version number |
Fingerprint | zwmL-6wgAADuFwn7 | Unique package identifier |
Size | gr-_DAQDGJdIim94 | Reference for unpacked size |
These upgrades to the build system align well with the programming improvements outlined below.
Programming Updates
Zig 0.14.0 brings several updates aimed at boosting code readability and performance. One notable addition is labeled switch statements, which simplify the design of state machines. For instance, using this feature in Zig's tokenizer resulted in a 13% performance boost.
Another improvement is the new @branchHint builtin, which replaces the older @setCold function. This change offers developers finer control over performance tuning.
Together, these updates make coding in Zig more intuitive and efficient.
Performance Improvements
This release also focuses on cutting down development time with two major advancements:
-
Incremental Compilation: By using the
-fincremental
flag along with--watch
, developers can significantly reduce reanalysis time. For example, a 500,000-line codebase saw a drop from 14 seconds to just 63 milliseconds. - x86 Backend Enhancement: The native x86 backend now achieves 98% compatibility with LLVM, passing 1,884 out of 1,923 behavior tests.
Additionally, the improved multithreaded backend allows code generation to run alongside frontend operations, speeding up the overall process.
sbb-itb-bfaad5b
Standard Library Updates
Memory Management Tools
Zig 0.14.0 brings updates to memory management with two revamped allocators. DebugAllocator now features built-in stack tracing and leak detection, cutting the zig ast-check
wall time by 10.1%. Meanwhile, SmpAllocator, designed for multi-threaded use in ReleaseFast mode, matches the performance of glibc malloc while being implemented in just 200 lines. It also reduces instructions by 3.9% when building the Zig compiler. To make memory resizing more efficient, the Allocator API now includes a remap
function in std.mem.Allocator.VTable
.
Data Processing
The standard library introduces support for ZON (Zig Object Notation), providing tools for streamlined data handling:
Component | Function | Use Case |
---|---|---|
std.zon.parse |
Parses ZON into Zig structs | Data deserialization |
std.zon.stringify |
Serializes data into ZON format | Data serialization |
std.zig.ZonGen |
Generates tree structures | Complex schema mapping |
Enhancements to binary search functions and the addition of a rehash method in std.hash_map
further improve performance, especially for cross-platform environments.
Platform Support
Version 0.14.0 boosts compatibility across major platforms with several updates:
- Linux: Kernel headers updated to 6.13.4
- macOS: Integrated Darwin libSystem 15.1
- Windows: Improved MinGW-w64 support
- WebAssembly: Enhanced wasi-libc implementation
Static std.mem.page_size
has been replaced by dynamic options: std.heap.page_size_min
, std.heap.page_size_max
, and the new std.heap.pageSize()
function.
Additionally, secure communication gets a boost with the addition of Transport Layer Security support via std.crypto.tls
.
Developer and Community Effects
Current Usage
The latest release prioritizes cutting down development latency, and early feedback from users highlights noticeable performance boosts in real-world projects. These new features and optimizations are showing clear improvements in critical development workflows.
Migration Guide
Upgrading to version 0.14.0 requires developers to make adjustments for several breaking changes. Here's a quick guide to the key updates:
Area | Required Change | Migration Action |
---|---|---|
Build System | New package hash format | Update build.zig files |
Export Syntax | @export now needs a pointer operand |
Add & operator where applicable |
Memory Operations | Removal of @fence |
Use stronger memory orderings instead |
Branch Hints | @setCold replaced |
Switch to @branchHint(.cold) |
Container Types | Field/declaration naming restrictions | Rename any conflicting identifiers |
One major update involves the build system API. Functions like Compile.installHeader
and b.addInstallHeaderFile
now require LazyPath
parameters. Additionally, the old WriteFile
step has been replaced by the new UpdateSourceFiles
step. Here's an example:
// Previous approach
const write = b.addWriteFiles();
// Updated approach
const update = b.addUpdateSourceFiles();
By addressing these changes, developers can ensure a smooth upgrade and be ready to leverage the new capabilities.
Future Development
The Zig community's involvement in version 0.14.0 has been impressive, with 251 contributors making 3,467 commits over a nine-month period. This collaborative effort is driving the project forward and laying the groundwork for exciting advancements.
"It takes a lot of time investment to make compilation fast, and we have gone all in on this investment." - AndyKelley
While incremental compilation is still in beta, developers can experiment with it using the -Dno-bin
option. This feature promises much faster recompilation for specific functions.
Another noteworthy update is the standard library's move to "Unmanaged"-Style Containers. This approach emphasizes explicit memory management, requiring developers to pass allocators directly to methods that need them. This aligns with Zig's philosophy of promoting transparency and control in resource management.
Next Steps
Download and Documentation
Zig 0.14.0 is ready for download, along with its Language Reference and Standard Library Documentation. Hereโs a quick look at the available files:
Operating System | Architecture | Download File |
---|---|---|
Windows | x86_64 | zig-windows-x86_64-0.14.0.zip |
Windows | aarch64 | zig-windows-aarch64-0.14.0.zip |
macOS | x86_64 | zig-macos-x86_64-0.14.0.tar.xz |
macOS | aarch64 | zig-macos-aarch64-0.14.0.tar.xz |
Linux | x86_64 | zig-linux-x86_64-0.14.0.tar.xz |
Linux | aarch64 | zig-linux-aarch64-0.14.0.tar.xz |
Once you've downloaded the files and explored the documentation, consider supporting Zig's development. Your contributions help fund its growth and improvement.
Contributing to Zig
The Zig Software Foundation (ZSF) oversees Zig's development, relying on community contributions. You can donate through various channels, such as:
- GitHub Sponsors
- Every
- Benevity
- Bank transfers
- Checks
- Wise
"It takes a lot of investment to maintain robust, optimal, and reusable software. Your recurring donations help turn unpaid volunteers into paid maintainers, accelerating our progress toward version 1.0." - Zig Software Foundation
For questions about donations, reach out to donations@ziglang.org. The foundation is dedicated to advancing the Zig programming language and supporting its growing community through education and resources.