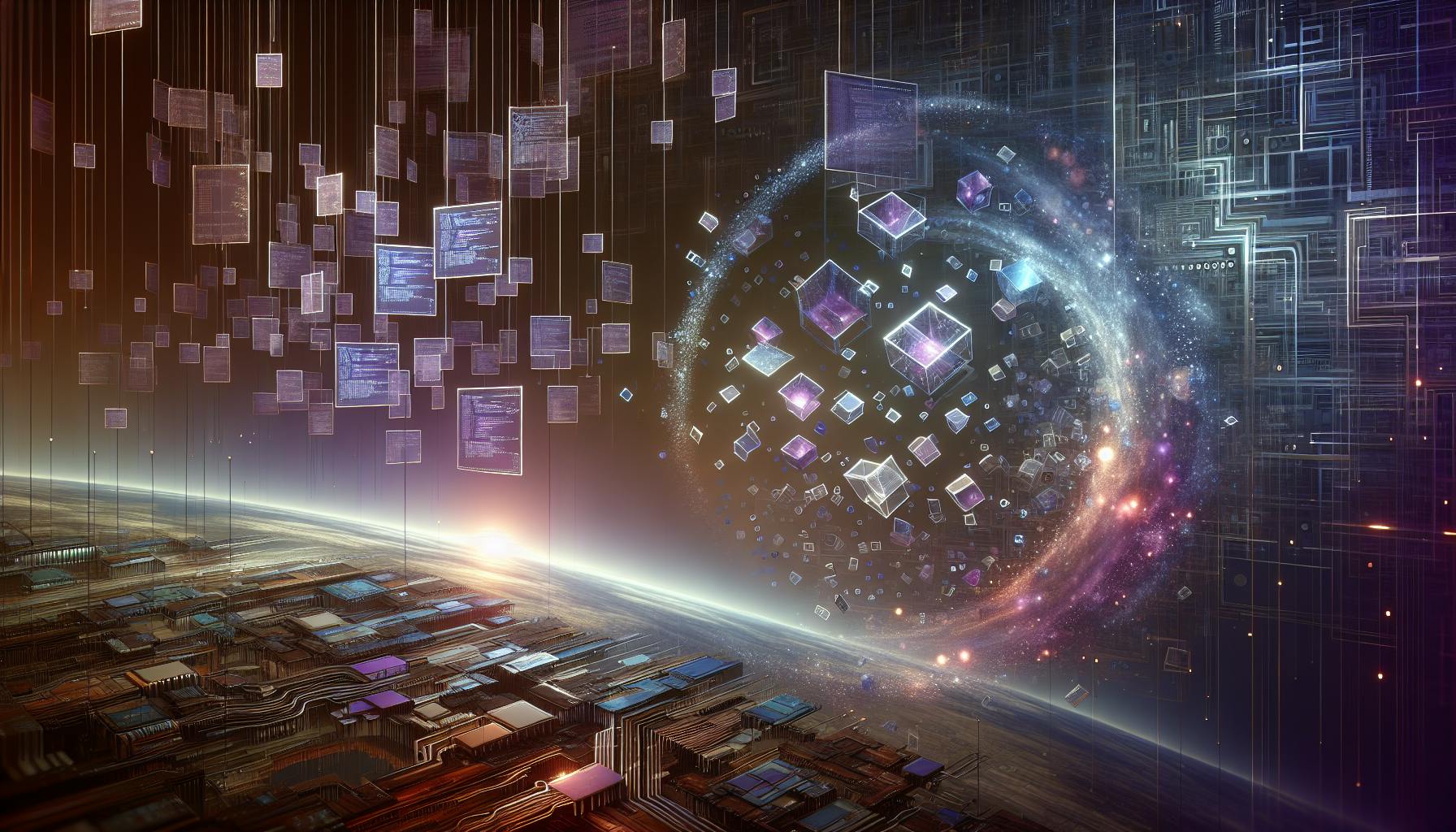
Learn the basics of Haskell, an advanced functional programming language, from setting up the environment to diving into syntax and advanced topics. Explore the community resources and real-world applications of Haskell.
If you're curious about Haskell and eager to embark on a coding journey, this guide is your starting point. Haskell, a purely functional programming language, offers a unique approach to coding with features like static typing, lazy evaluation, and pattern matching. Here's a quick rundown of what you'll learn:
- What Haskell Is: A precise language designed for complex data handling and concurrency.
- Setting Up: How to install the Glasgow Haskell Compiler (GHC) and set up your environment.
- Basics: Dive into syntax, data types, and creating functions in Haskell.
- Functional Programming: Understand the core ideas behind Haskell's approach to coding.
- Common Traps: Tips on avoiding beginner mistakes.
- Advanced Topics: An introduction to higher-order functions, type systems, and real-world applications.
- Community Resources: Where to find help and continue your learning journey.
This guide aims to make learning Haskell approachable, breaking down complex concepts into understandable parts. Whether you're completely new to programming or looking to expand your skills, Haskell offers a challenging yet rewarding path.
What is Haskell?
Haskell is a special kind of computer language that follows specific rules. It's known for being very precise, which helps spot mistakes early. Here are some things that make Haskell unique:
- Statically typed - Haskell knows what type of data (like numbers or text) you're using when you write your code. This helps catch errors before your program runs.
- Purely functional - In Haskell, functions work a bit like math equations. They always give the same result for the same input and don't cause unexpected changes elsewhere.
- Lazy evaluation - Haskell waits to do calculations until it absolutely has to. This can make programs more efficient and even lets you work with lists of items that go on forever.
- Pattern matching - This allows Haskell to check the structure of data and work with it more easily. It's like having a smart way to go through your data and pick out what you need.
- Type inference - Haskell is smart enough to figure out what data type you're using most of the time, so you don't have to keep saying it.
All these features make Haskell a powerful tool for certain jobs, like handling data in complex ways or doing many things at once.
The Evolution of Haskell
Haskell didn't just appear out of nowhere. It was created by a group of smart people in 1987 who wanted to make a new kind of programming language. They named it after a famous mathematician, Haskell Curry.
Here's how Haskell grew over time:
-
1990 - The first version of Haskell came out. It introduced some of its key features.
-
1997 - A big update called Haskell 98 made it ready for more serious work.
-
1999 - The first textbook on Haskell was published, making it easier for people to learn.
-
2010 - Another update, Haskell 2010, added new features for handling tasks at the same time more easily.
-
2020 - Haskell keeps getting better, with new tools and libraries for people to use.
Haskell was inspired by other languages but has grown into something used in many different fields, from finance to science and even art.
Chapter 2: Setting Up Your Haskell Environment
Choosing a Haskell Compiler
The main tool you'll use to work with Haskell is called the Glasgow Haskell Compiler (GHC). It's like the brain that understands and runs your Haskell code. Here's why GHC is a good choice:
- It's always being improved and can do the latest Haskell tricks.
- It has a special mode (GHCi) that lets you try out code on the fly.
- It's included in a package called the Haskell Platform, which makes it easy to get started.
- It's very good at making your code run fast.
- Lots of Haskell learners and experts use it, so it's easier to find help or resources.
GHC is great for beginners because it has lots of helpful guides, a big library of extra code you can use, and it's built to handle big projects as you get better.
Installation Guide
Here's how to get GHC and other tools set up on your computer.
Windows
- Download the Haskell Platform from Haskell.org.
- Run the installer and follow the steps.
- Check if it worked by opening a command prompt and typing
ghci
.
Linux
- Use your package manager to install Haskell. For Ubuntu or Debian, type
sudo apt install ghc cabal-install
in the terminal.
MacOS
- You can use Homebrew with
brew install ghc cabal-install
, MacPorts withsudo port install ghc cabal-install
, or download the installer from Haskell.org.
After installing, type ghci
in your terminal to see if it works.
Interactive Haskell
GHCi lets you experiment with Haskell code easily. It's like a playground:
- Open it by typing
ghci
in your terminal. - You can type Haskell code right there and see what happens.
- It's perfect for when you're learning and want to test out small bits of code.
- You can even load whole Haskell files to work on bigger things.
Some fun things to try in GHCi:
- Basic math operations like
+
,-
,*
,/
. - Try some built-in functions such as
sqrt
for square roots, orsin
andcos
for trigonometry. - Use
let x = 1
to define a variable. - Make a simple function, like
square x = x*x
, to see how functions work.
GHCi is a great tool for moving from learning Haskell in books or online to doing real projects.
Chapter 3: Haskell Basics
Syntax Overview
When you start with Haskell, you'll notice a few things are different from other programming languages:
-
Semicolons - You don't need semicolons (
;
) to end lines or separate parts in Haskell. -
Parentheses - These are used for calling functions and also to group parts of your code together. It helps Haskell understand what you want to do first.
-
Curly braces - Haskell doesn't use curly braces (
{ }
) to group code. Instead, how you indent or space out your code is important. -
Indentation - The way you line up your code matters a lot. If lines are aligned, Haskell sees them as related. This means you can control the flow of your program by how you arrange it.
-
Comments - To add comments, start with
--
for short notes, or use{--
and--}
to comment out bigger sections.
These differences might seem small, but they're key to getting comfortable with Haskell.
Basic Data Types and Operations
Haskell has several simple types of data you can work with:
-
Numerics - Use
Int
for whole numbers andDouble
for decimal numbers. Haskell supports usual math operations like+
,-
,*
,/
. -
Boolean -
Bool
is for true/false values, with operations like&&
(and),||
(or),not
(not). -
Characters -
Char
is for single letters or symbols, wrapped in single quotes like'a'
. -
Tuples - Tuples like
(Int, String)
let you group different types of data together. -
Lists - Lists
[1, 2, 3]
hold items of the same type. Haskell has many functions to work with lists.
This is a quick look at how you can handle basic data in Haskell.
Functions
Creating your own functions is a big part of using Haskell:
double x = x + x -- A simple function to double a number
factorial n = product [1..n] -- Factorial using a built-in function
-
To define a function, you write its name and parameters (like
x
andn
) on one side and what it does on the other. -
You can use multiple parameters just by putting them next to each other.
Haskell also has some cool features for functions:
-
Guards are like if/else checks that let your function do different things based on certain conditions.
-
Pattern matching lets your function react to different kinds of input by matching its shape or pattern.
Learning to define and use functions is key to doing more with Haskell.
Chapter 4: Diving Deeper
Higher-order Functions
Higher-order functions are like super helpers in Haskell. They can take functions as inputs or even give back functions as results. This feature makes Haskell very flexible and powerful.
Here are some examples:
map
is a function that takes another function and a list, then applies that function to every item in the list, giving back a new list. For instance:
map (+1) [1, 2, 3] = [2, 3, 4]
filter
is used to pick out certain items from a list. It takes a function that checks each item (true or false), and keeps only the ones that pass the test:
filter odd [1, 2, 3, 4] = [1, 3]
foldl
andfoldr
combine items in a list into a single result by repeatedly applying a function. It's like folding a piece of paper step by step until it's all stacked up:
foldl (+) 0 [1, 2, 3] = ((0 + 1) + 2) + 3 = 6
Using higher-order functions helps keep your code neat and allows for clever tricks in Haskell.
Type Systems and Type Classes
Haskell's type system is like a smart assistant that helps avoid mistakes by checking your code before it even runs. And the best part? Haskell can often guess the types for you, so you don't have to spell everything out.
Here's what you need to know:
-
Polymorphism means that functions can work with many types. Haskell uses type classes like
Eq
,Ord
,Show
to make this happen. -
Type variables are placeholders for any type, making functions flexible.
-
Algebraic data types let you create your own types by combining existing ones in new ways.
For example, you can define a shape as either a circle with a radius or a rectangle with width and height, and calculate the area differently based on the shape.
data Shape = Circle Float | Rectangle Float Float
area :: Shape -> Float
area (Circle r) = pi * r^2
area (Rectangle x y) = x * y
Haskell's type system makes your code safer and more reliable.
Pattern Matching and Guards
Pattern matching in Haskell lets you handle different situations in a clean and straightforward way, without getting lost in complicated if-else statements.
For example, calculating a factorial:
factorial 0 = 1
factorial n
| n > 0 = n * factorial (n-1)
| otherwise = error "Negative factorial undefined"
- Directly checking for 0 gives us the base case.
- The
|
syntax is used for guards, which are like traffic lights telling Haskell what to do under certain conditions.
Pattern matching and guards make your code cleaner and easier to read by handling different cases in a structured way.
Chapter 5: Real-world Haskell
Modules and Packages
In Haskell, we keep our code neat by organizing it into modules. Think of a module as a folder where you keep related stuff together:
module Math (factorial, fibonacci) where
factorial :: Integer -> Integer
factorial 0 = 1
factorial n = n * factorial (n-1)
fibonacci :: Integer -> Integer
fibonacci 0 = 0
fibonacci 1 = 1
fibonacci n = fibonacci (n-1) + fibonacci (n-2)
Here, we have a Math
module with two functions that can be used by other parts of our program.
Modules help us by:
- Keeping related code together
- Controlling what parts of our code can be used elsewhere
- Allowing us to reuse code easily
Packages are like bigger containers that hold several modules together. They save us time because we don't have to write everything from scratch.
To use a package, you add it to your project's settings, then download it with some commands (cabal update
and cabal install
). After that, you can use the modules inside it.
Input & Output
Haskell handles input and output (I/O) a bit differently. It keeps I/O separate, so it doesn't mess with the rest of the code.
Here's how you can read from and write to a file:
import System.IO
main = do
handle <- openFile "data.txt" ReadMode
contents <- hGetContents handle
putStr contents
hClose handle
- We use
do
to do several I/O things in order. openFile
opens a file.hGetContents
reads the file into a string.putStr
shows the string.hClose
closes the file.
This way, I/O doesn't interfere with the rest of your code.
Other I/O operations include reading user input and printing messages.
Building Your First Haskell Project
Let's make a simple tool that counts words in a file.
-
Start a new project with Stack or Cabal.
-
Update your project settings to include any modules or libraries you need.
-
Make a
Main.hs
module:module Main where import System.Environment import System.IO main = do [filename] <- getArgs contents <- readFile filename print (countWords contents)
-
Create a
Utils.hs
module for helper functions:module Utils where countWords :: String -> Int countWords = length . words
-
Build your project with
stack build
orcabal build
. -
Run it with
stack exec wordcount data.txt
.
And there you have it, your very own Haskell program!
sbb-itb-bfaad5b
Chapter 6: Advanced Topics
For those tackling more complex programs, this chapter introduces influential Haskell concepts like monads, functors, and applicatives.
Understanding Monads
Monads are a bit like magic boxes in Haskell that help you do things step by step, especially when you're not sure if everything will go as planned. Here's how to think about them in a simpler way:
-
Monads "wrap" values with some extra rules on how to use them.
-
Maybe
is a monad for when you might not have a value at all. -
IO
deals with input and output, like reading a file, in a way that doesn't mess up the rest of your code.
Monads work with two main ideas:
-
bind
helps you do one thing after another in order. -
return
takes a regular value and puts it into a monad.
You'll see monads like:
-
IO
for reading and writing stuff -
Maybe
for dealing with missing values -
List
for working with lists of possibilities -
State
for keeping track of changes
You can link monadic actions with do
notation, like this:
getLine >>= ame ->
putStrLn ("Hello " ++ name)
This connects getLine
and putStrLn
using the bind operator.
As you use them more, monads will start to make sense.
Functors and Applicatives
Other important concepts are:
- Functors, which let you apply functions to values inside contexts, like adding 1 to every item in a list:
fmap (+1) [1, 2, 3] = [2, 3, 4]
- Applicatives, which are like functors but can apply functions that are also wrapped up:
(+1) <*> Just 2 = Just 3
Functors and Applicatives are really useful for working with data in flexible ways.
Practical Applications
Haskell is great for big, complicated projects in many areas:
-
Web - Building websites with tools like Yesod and Scotty.
-
Data - It's really good at sorting and analyzing data. There's even a version of Pandas for Haskell.
-
DevOps - Managing cloud resources with code using Pulumi.
-
Finance - Haskell is used in trading for its speed and reliability.
-
AI - There are Haskell tools for machine learning, like HLearn.
In the real world, Haskell's strengths in managing types, keeping code clean, handling many tasks at once, and dealing with data efficiently make it a powerful choice.
Chapter 7: Resources and Community
Learning Resources
Here's a list of great places to learn more about Haskell:
- Haskell Programming from first principles - A detailed book for beginners. It explains Haskell concepts clearly with lots of examples.
- Haskell Wikibook - A free online book you can use to learn Haskell, whether you're just starting or already know a bit.
- Happy Learn Haskell Tutorial - A friendly guide for newbies with exercises to help you practice.
- Haskell 99 Problems - A set of 99 fun challenges to improve your Haskell skills.
- Project Euler - A collection of math problems that you can solve using Haskell.
There are also lots of blogs, YouTube channels, and other free resources online where you can learn more about Haskell.
Haskell Community
Haskell might not be the most common programming language, but it has a friendly and active community. Here's how you can join in:
- Check out the Haskell Reddit to talk about Haskell with others.
- Join Haskell Discord servers like Haskell Beginners and Haskell Cafe to chat in real-time.
- Go to local Haskell meetups to meet people who are also learning or using Haskell.
- Attend Haskell conferences and events like Haskell Exchange to meet other Haskell users face-to-face.
The Haskell community is very welcoming to newcomers, so don't hesitate to ask questions as you learn!
Chapter 8: Next Steps
Practice Makes Perfect
Getting better at Haskell, or anything new really, comes down to practicing a lot. Here are a few ways you can keep improving:
- Head over to websites with Haskell exercises and challenges. They have lots of problems to help you use what you've learned.
- Try making simple tools you use on your computer, but in Haskell this time. For example, you could make a simple version of
grep
orcurl
. - Help out with Haskell projects on GitHub. Starting with small fixes or helping with documentation is a great way to see real Haskell code in action.
- Look for Haskell monthly challenges and give them a try. They have problems for all skill levels.
- Think about a program you really want to make for yourself and try building it in Haskell. It's more fun to learn when you're making something you care about.
Using Haskell for small daily tasks is a good way to get comfortable with it.
Staying Updated
Haskell keeps changing and getting new features, so it's good to stay in the loop:
- Keep an eye on updates for GHC, the Haskell compiler. Big updates usually bring cool new stuff.
- Read Haskell newsletters and blogs, like Haskell Weekly and Well-Typed, to hear about new things in the Haskell world.
- Join Haskell groups on places like Reddit and Discord to chat about the latest news with others.
- Look at new research papers and articles about Haskell, for example at ICFP Conference, to see the newest ideas.
- Watch talks from recent Haskell events to learn from the experts.
Keeping up with the latest in Haskell makes sure you're writing your code in the best and most current way.
Conclusion
Summary
We've talked about a lot in this guide for beginners on Haskell. Here's what you should remember:
- Haskell is a special kind of programming language that's really good at dealing with complicated stuff in a smart way.
- It might look a bit odd at first, but things like pattern matching and functions that can take other functions as inputs make Haskell neat and useful.
- Haskell helps you catch mistakes early with its strong focus on types, but it's also flexible thanks to something called typeclasses.
- People use Haskell for all sorts of real jobs, like sorting data, managing computer systems, and even in finance. It's known for being reliable and fast.
- There's a friendly bunch of people out there using Haskell, sharing advice, making challenges, and keeping everyone updated on what's new.
Now that you know the basics, you're ready to dig into more detailed Haskell guides, start your own projects, and get involved with other Haskell users.
Encouragement
Learning Haskell can be tough at first. But if you keep trying things out, asking questions, and working on small projects, you'll get the hang of it.
The Haskell community is really welcoming, so don't hesitate to reach out if you need help!
Look for more learning materials, find a project that excites you, or join online groups. Staying engaged is key.
Soon enough, you'll find that moment where everything about Haskell makes sense. Just keep at it and enjoy the journey!
Related Questions
Is Haskell beginner friendly?
Yes, Haskell might look hard to start with because it's quite different from other programming languages. But don't worry, even if you're new to programming, you can learn Haskell. It's all about finding the right learning materials that are made for beginners, asking for help when you need it (like in online Haskell chatrooms), and taking your time to understand things. Remember, learning Haskell is a journey, and it's okay to move at your own pace.
Is it difficult to learn Haskell?
Compared to more common languages like Python, Haskell can be tougher to pick up. This is because it uses a lot of recursion (doing things over and over again in a specific way), has a very strict rule set for how data can be used, and introduces some complex ideas that might seem strange at first, like monads. But, once you get past these initial hurdles, Haskell allows you to solve complicated problems in a very neat and powerful way. It's worth the effort if you're up for the challenge.
How long will it take to learn Haskell?
If you're looking to get good at Haskell and start building things with it, plan on spending about 2-3 months diving deep. The first month is all about getting used to how Haskell looks and works. In the second month, you'll learn about its core ideas, like higher order functions (functions that work with other functions) and monads (a tricky concept that's very useful in Haskell). The last month is for putting everything into practice by working on bigger projects. Learning Haskell takes time, but it's a skill that really stands out.
What is the downside of Haskell?
The main challenges with Haskell are related to its design. Because it's 'lazy' (it waits as long as possible to do calculations), optimizing your code can be tricky. Also, because it's 'pure' (it doesn't allow for shortcuts or quick fixes when handling data), you have to be very clear about how data moves through your program. These aspects can be tough for beginners. However, they also lead to cleaner and more reliable code, which is a big plus. Like any language, Haskell has its trade-offs.