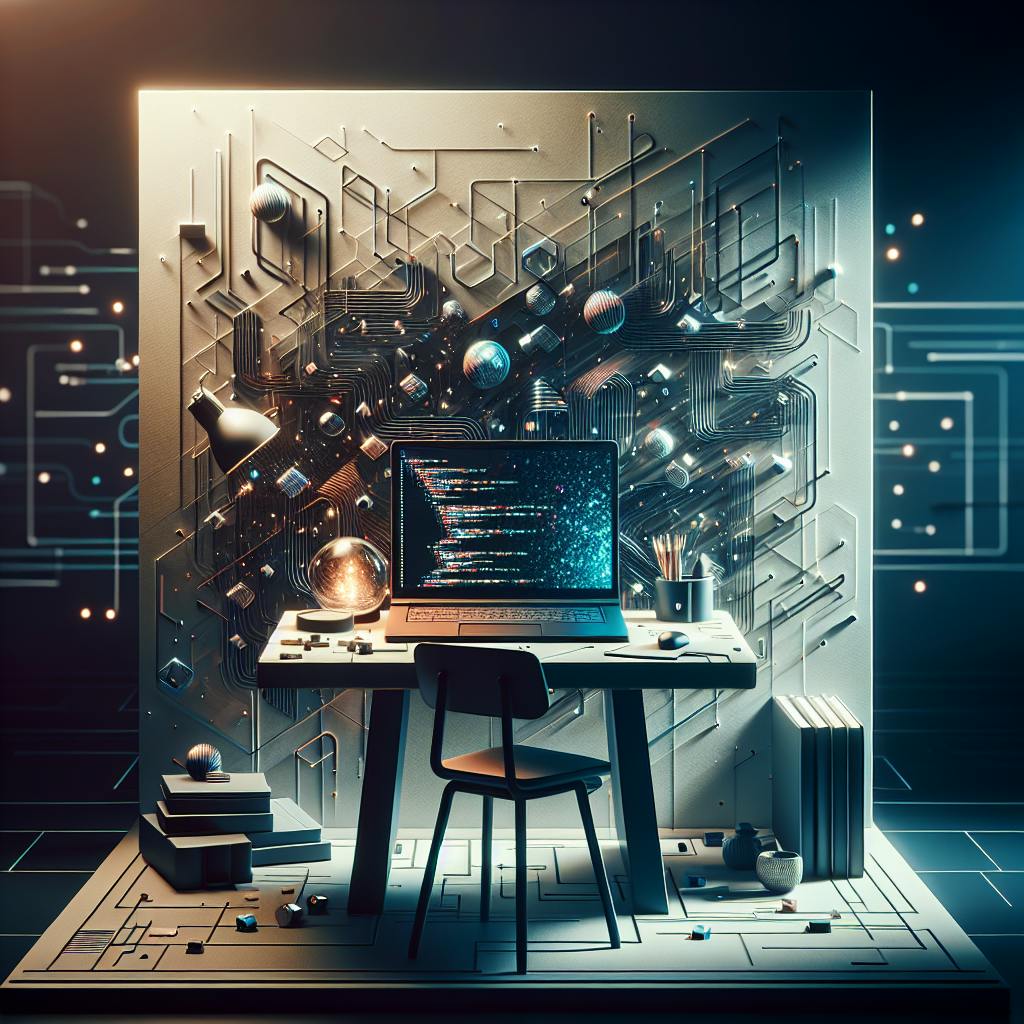
Learn about setting up, routing, sessions, and deployment in Sinatra, a lightweight web application framework for Ruby. Explore comparisons with Rails, installation steps, routing basics, session management, deployment options, and advanced tips.
Sinatra is a lightweight web application framework for Ruby, ideal for small projects and APIs. Here's what you need to know:
- Setup: Install Ruby, create a Gemfile, install Sinatra, and set up your development environment
- Routing: Define routes using HTTP methods (GET, POST, PUT, DELETE)
- Sessions: Enable sessions, store and retrieve data, and ensure security
- Deployment: Prepare your app, choose a hosting service, and deploy
Quick Comparison: Sinatra vs Rails
Feature | Sinatra | Rails |
---|---|---|
Project Size | Small | Medium to Large |
Learning Curve | Easy | Steeper |
Flexibility | High | Less |
Built-in Features | Few | Many |
Best For | Simple apps, APIs | Complex apps |
Sinatra offers a simple way to build web apps without the extra code often needed in bigger frameworks like Rails. It's perfect for small projects, quick prototypes, and microservices.
Related video from YouTube
Getting Started with Sinatra
Installing Ruby and Sinatra
To start using Sinatra, you need Ruby on your computer. If you don't have it, install Ruby first.
Next, install Sinatra:
-
Create a file named
Gemfile
-
Add this line to the file:
gem 'sinatra'
-
Run this command in your terminal:
bundle install
Creating Your First Sinatra App
Make a file called app.rb
with this code:
require 'sinatra'
get '/' do
'Hello, World!'
end
Run the app:
ruby app.rb
Open http://localhost:4567/
in your browser to see "Hello, World!".
Sinatra App Structure
Sinatra doesn't force a specific structure, but here's a common setup:
โโโ app.rb
โโโ lib
โ โโโ models
โ โโโ controllers
โ โโโ helpers
โโโ views
app.rb
: Main filelib
: Holds models, controllers, and helpersviews
: Stores templates
Setting Up Your Development Environment
To set up your workspace:
-
Choose a text editor (e.g., Atom, Sublime Text)
-
Install app dependencies:
bundle install
This installs everything in your Gemfile
.
Step | Action |
---|---|
1 | Install Ruby |
2 | Create Gemfile |
3 | Install Sinatra |
4 | Create app.rb |
5 | Set up file structure |
6 | Choose text editor |
7 | Install dependencies |
Now you're ready to build your Sinatra app. The next part will cover routing.
Routing in Sinatra
Basics of Routing
Routing in Sinatra is simple. It uses HTTP methods to define routes. Here's a basic example:
get '/' do
'Hello, World!'
end
This route responds to GET requests at the root URL with "Hello, World!".
Working with HTTP Methods
Sinatra supports main HTTP methods:
Method | Use |
---|---|
GET | Fetch data |
POST | Create data |
PUT | Update data |
DELETE | Remove data |
Example:
get '/users' do
# Get users
end
post '/users' do
# Add new user
end
put '/users/:id' do
# Update user
end
delete '/users/:id' do
# Remove user
end
Using Dynamic Routes and Parameters
Dynamic routes let you use URL parts as variables:
get '/users/:id' do
id = params[:id]
# Use id to find user
end
You can use multiple parameters:
get '/users/:id/:name' do
id = params[:id]
name = params[:name]
# Use id and name
end
Wildcard Routes
Wildcard routes catch any URL after a certain point:
get '/users/*' do
path = params[:splat]
# Use path
end
Organizing Routes in Larger Apps
For bigger apps, you can group routes:
group '/api' do
get '/users' do
# Get users
end
post '/users' do
# Add new user
end
end
This puts all routes under /api
, making them easier to manage.
sbb-itb-bfaad5b
Managing Sessions in Sinatra
Turning On Sessions
To use sessions in Sinatra:
- Add this line at the top of your app file:
enable :sessions
This turns on cookie-based sessions.
Saving and Reading Session Data
Use the session
hash to save and read data:
# Save data
session[:username] = 'john_doe'
# Read data
username = session[:username]
You can store complex data too:
session[:cart] = [
{ item: 'Book', price: 10.99 },
{ item: 'Pen', price: 1.99 }
]
Keeping Sessions Safe
Sinatra sessions are signed but not hidden. To make them safer:
- Set a secret key:
set :session_secret, 'your_secret_key'
- Use HTTPS to protect data in transit.
Other Ways to Handle Sessions
You can use a database for sessions:
- Add to your Gemfile:
gem 'sinatra-session'
- Set up in your app:
use Sinatra::Session
This stores session data in a database, not cookies.
Method | Storage | Pros | Cons |
---|---|---|---|
Cookies | Browser | Easy to set up | Less secure |
Database | Server | More secure | Needs extra setup |
Deploying Sinatra Apps
Getting Your App Ready for Production
Before you put your Sinatra app online, make sure it's ready:
- Test your app well
- Make it run faster
- Keep it safe
- Set up logging
Picking a Hosting Service
Choose where to put your app online:
Service | Features | Best For |
---|---|---|
Heroku | Free plan, easy to use | Beginners, small apps |
AWS | Many options, powerful | Big apps, custom needs |
DigitalOcean | Simple, good value | Medium apps, more control |
How to Deploy Your App
To put your app online:
- Make a Git repository for your code
- Set up a Heroku app
- Set your app's settings
- Send your app to Heroku
Keeping Your App Running Smoothly
After your app is online:
Task | Why It's Important |
---|---|
Check your logs | Find and fix problems |
Use a monitoring tool | See how well your app runs |
Update your app's parts | Keep your app safe and working well |
Save your data | Protect against losing information |
Advanced Sinatra Tips and Tricks
Building RESTful APIs with Sinatra
Sinatra works well for making RESTful APIs. It supports HTTP methods like GET, POST, PUT, and DELETE. Here's how to set up a basic RESTful API:
require 'sinatra'
get '/users' do
users = User.all
json users
end
post '/users' do
user = User.create(params[:user])
json user
end
put '/users/:id' do
user = User.find(params[:id])
user.update(params[:user])
json user
end
delete '/users/:id' do
user = User.find(params[:id])
user.destroy
json({ message: 'User deleted' })
end
Making Your App Run Faster
To speed up your Sinatra app:
Method | Description |
---|---|
Use caching | Store often-used data to reduce database load |
Improve database queries | Use indexes, limit data retrieval |
Choose a faster database | Try PostgreSQL or MySQL instead of SQLite |
Use a CDN | Serve static files from a Content Delivery Network |
Dealing with Errors and Logging
To handle errors and logging:
- Use Sinatra's built-in error handling
- Log errors with Logger or Log4r
- Try error tracking services like Airbrake or Rollbar
Testing Your Sinatra App
For testing your app:
- Use Rack::Test or other frameworks like RSpec
- Write unit tests for individual parts
- Create integration tests for overall function
- Try UI testing tools like Capybara or Selenium
Wrap-Up
Main Points to Remember
Sinatra is a useful tool for making web apps with Ruby. It's good for:
- Small to medium web apps
- APIs
- Microservices
Key things about Sinatra:
Feature | Description |
---|---|
Type | Web app framework for Ruby |
Size | Small and light |
Ease of use | Simple to learn and use |
Best for | Quick web app building |
What's Next for Sinatra
Sinatra will keep growing. Here's what might happen:
Area | Possible Changes |
---|---|
Web tech support | Better tools for new web tech |
More users | More people using Sinatra |
Bigger community | More people helping improve Sinatra |
Sinatra is good for many web tasks. It's easy to use and quick to start with. If you want to make web apps fast, Sinatra is a good choice.