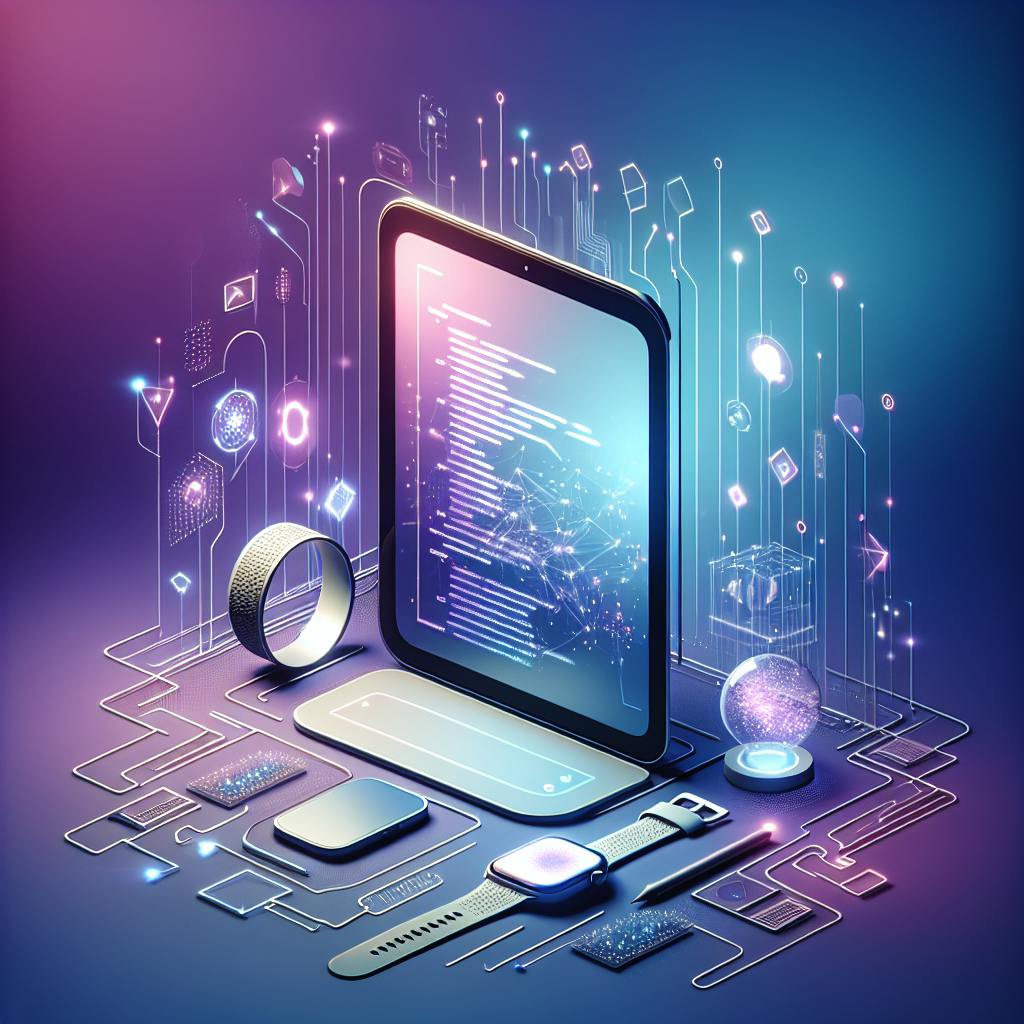
Enhance your iOS app's performance with 15 optimization techniques. From caching to Swift usage, learn how to improve speed and responsiveness for a better user experience.
Optimizing your iOS app's performance is crucial for delivering a smooth and engaging user experience. By implementing the following techniques, you can significantly enhance your app's speed, responsiveness, and overall performance:
- Reduce network requests with caching
- Asynchronous operations with Grand Central Dispatch (GCD)
- Optimize image and asset sizes
- Implement pagination for data display
- Use Core Data for local data storage
- Employ advanced compiler optimizations
- Reduce view hierarchy complexity
- Use Auto Layout wisely
- Profile and debug performance regularly
- Opt for Swift over Objective-C
- Background task management with background modes
- Implement smart prefetching
- Optimize battery consumption
- Utilize Content Delivery Networks (CDNs)
- Stay updated with the latest iOS SDKs and APIs
Technique | Benefits |
---|---|
Caching | Faster load times, reduced network requests |
GCD | Concurrent task execution, improved responsiveness |
Image Optimization | Lower memory usage, faster loading times |
Pagination | Faster loading, less memory usage, lazy loading |
Core Data | Efficient data retrieval, optimized storage |
Compiler Optimizations | Better code generation, faster runtime performance |
View Hierarchy Optimization | Decreased rendering time, lower memory usage |
Auto Layout | Reduced rendering time, lower memory usage |
Performance Profiling | Identify and fix slow areas, improve responsiveness |
Swift over Objective-C | Up to 2.6x faster, easier maintenance |
Background Modes | Smoother UI, continuous operation |
Prefetching | Faster loading, improved responsiveness |
Battery Optimization | Increased battery life, improved responsiveness |
Content Delivery Networks | Faster load times, reduced latency |
Latest SDKs and APIs | Improved performance, security, and features |
Prioritizing performance optimization can increase user engagement, retention, and drive business success. Stay updated with the latest iOS SDKs and APIs, and regularly profile and debug your app to identify areas for improvement.
Related video from YouTube
1. Reduce Network Requests with Caching
Caching helps make your iOS app faster and smoother. It cuts down on repeated network requests, saving data and speeding up load times. Having a smart caching plan is key for keeping info up-to-date and giving users a great experience.
How Hard Is It?
Setting up caching in your iOS app is pretty straightforward, thanks to Apple's built-in URLCache
. Just set up a custom URLCache
object and make it the shared cache instance. This lets you control how caching works for your app.
Speed Boost
Caching can seriously speed up your app by reducing network requests and cutting down on delays. The result? Faster load times, snappier responses, and an all-around better user experience.
Maintenance Effort
Aspect | Description |
---|---|
Complexity | Caching adds some complexity around invalidating the cache and keeping data fresh. |
Benefits | But the performance gains and improved user experience make it worthwhile. |
Strategy | Implement caching strategically to minimize ongoing maintenance work. |
2. Asynchronous Operations with Grand Central Dispatch (GCD)
Keeping your iOS app responsive and efficient is crucial. Grand Central Dispatch (GCD) is a powerful tool from Apple that helps manage concurrent tasks and parallelism. By using GCD, you can execute tasks asynchronously, taking advantage of multiple CPU cores and boosting your app's performance.
Easy to Implement
While GCD involves understanding concurrency and parallelism concepts, Apple provides a comprehensive guide. The initial setup may seem complex, but the benefits make it worthwhile.
Performance Boost
GCD can significantly improve your app's performance by:
- Executing tasks concurrently, reducing the load on the main thread
- Optimizing CPU utilization, resulting in faster task execution
- Improving responsiveness, as the app remains interactive during background tasks
Maintenance Considerations
Aspect | Description |
---|---|
Complexity | GCD requires understanding concurrency and parallelism. |
Benefits | Improved performance, responsiveness, and CPU utilization. |
Strategy | Implement GCD strategically for tasks that benefit from concurrency and parallelism. |
3. Optimize Image and Asset Sizes
Large images and assets can slow down your iOS app's performance and increase crashes due to high memory usage. Optimizing their sizes is crucial for a smooth user experience.
Easy to Implement
You can optimize image and asset sizes using tools like ImageOptim, Adobe Photoshop's Save for Web feature, or PNGCrush. These tools compress images without sacrificing quality. You can also use asset catalogs to organize and optimize your image assets.
Boosts Performance
Optimizing image and asset sizes can significantly improve your app's performance:
- Reduces memory usage, leading to faster performance and fewer crashes
- Improves loading times for a better user experience
- Decreases the overall app size, making it easier to download and update
Minimal Maintenance
Maintaining optimized image and asset sizes requires some ongoing effort. You'll need to ensure new images and assets are optimized as they're added to your app. However, this effort is minimal compared to the performance benefits.
To optimize image and asset sizes, consider these techniques:
- Use compressed image formats like WebP or HEIF
- Resize images to the appropriate dimensions for their intended use
- Compress images without significant quality loss
- Utilize image asset catalogs to generate optimized versions for different device resolutions
- Use image lazy loading techniques to load images on demand
Technique | Description |
---|---|
Compressed Formats | Use formats like WebP or HEIF for smaller file sizes. |
Resize Images | Ensure images are sized correctly for their intended use. |
Compress Without Quality Loss | Compress images without significantly reducing quality. |
Asset Catalogs | Generate optimized versions for different device resolutions. |
Lazy Loading | Load images on demand to reduce initial load times. |
4. Implement Pagination for Data Display
How Hard Is It?
Adding pagination to display data in an iOS app has a moderate level of complexity. You'll need to consider how to load and cache data, as well as handle user scrolling and UI updates. Using a UITableView
or UICollectionView
with pagination can simplify the process.
Speed Boost
Pagination can significantly speed up your app by:
- Faster Loading: Reducing the initial data load, resulting in quicker loading times.
- Less Memory Usage: Minimizing memory usage, preventing crashes and improving stability.
- Lazy Loading: Loading data only when needed, reducing bandwidth usage and improving user experience.
Maintenance Effort
Aspect | Description |
---|---|
Effort Required | Moderate effort is needed to maintain pagination. |
Data Loading | Ensure proper data loading and caching. |
UI Updates | Implement correct UI updates for a smooth experience. |
Optimization | Optimize for different devices and screen sizes. |
Implementation Tips
- Use a
UITableView
orUICollectionView
with pagination for simpler data loading and caching. - Implement lazy loading to load data only when needed.
- Optimize page size and data loading to minimize memory usage and improve performance.
- Use caching to reduce the need for repeated data retrieval.
- Handle user scrolling and UI updates correctly for a smooth user experience.
5. Use Core Data for Local Data Storage
How Difficult Is It?
Setting up Core Data for local data storage in an iOS app has a moderate to high level of difficulty. You'll need a solid understanding of Core Data's architecture, including entities, attributes, and relationships. You must design a data model, create a managed object context, and implement mechanisms for storing and retrieving data.
Performance Benefits
Using Core Data can significantly boost your iOS app's performance by:
- Faster Data Retrieval: Core Data provides an efficient way to retrieve data, reducing loading times and improving the overall user experience.
- Optimized Data Storage: Core Data optimizes data storage, decreasing memory usage and enhancing app stability.
- Automatic Data Validation: Core Data automatically validates data, ensuring consistency and reducing errors.
Maintenance Considerations
Aspect | Description |
---|---|
Effort Required | Moderate to high effort is needed to maintain Core Data. |
Data Model Updates | Update the data model to reflect changes in the app's data structure. |
Data Migration | Implement data migration strategies to ensure data consistency across app updates. |
Performance Optimization | Optimize Core Data performance by using efficient data retrieval and storage mechanisms. |
Implementation Tips
- Design a well-structured data model that accurately represents your app's data requirements.
- Utilize Core Data's built-in features for efficient data retrieval and storage.
- Implement data migration strategies to ensure seamless data transitions during app updates.
- Regularly monitor and optimize Core Data's performance to maintain a smooth user experience.
6. Employ Advanced Compiler Optimizations
How Difficult Is It?
Employing advanced compiler optimizations in your iOS app has a moderate level of complexity. You'll need a solid understanding of Swift's compiler optimizations and how to utilize them effectively. This involves enabling optimizations, using Swift-specific constructs, and profiling and optimizing performance-critical code.
Performance Boost
Swift's compiler optimizations can significantly boost your app's performance. By enabling and leveraging them, you can achieve better code generation and overall runtime performance. This results in faster app execution, improved responsiveness, and a better user experience.
Ongoing Effort
Maintaining advanced compiler optimizations requires moderate effort. You'll need to regularly profile and optimize your code to ensure the optimizations are effective and not causing performance regressions. Additionally, you may need to update your optimization strategies as new compiler optimizations become available.
Implementation Tips
Technique | Description |
---|---|
Enable Optimizations | Enable Whole Module Optimization and Link-Time Optimization (LTO) in your project settings. |
Use Swift Constructs | Utilize Swift-specific constructs like the "defer" statement and inline functions to help the compiler optimize better. |
Profile and Benchmark | Identify bottlenecks and areas that could benefit from compiler optimizations. |
Analyze Usage | Analyze CPU and memory usage, identify hotspots, and optimize frequently executed code paths. |
Cache Computed Values | Consider caching computed values or results to avoid redundant calculations that can impact performance. |
7. Reduce View Hierarchy Complexity
A complex view hierarchy in your iOS app can slow down rendering, increase memory usage, and degrade the user experience. Simplifying the view hierarchy is crucial for optimal performance.
Implementation Effort
Reducing view hierarchy complexity requires moderate effort. You'll need to analyze your app's view hierarchy, identify complex areas, and refactor your code to simplify the hierarchy. This may involve rearranging views, reducing transparency, and optimizing layout constraints.
Performance Impact
Simplifying your view hierarchy can significantly improve your app's performance:
- Decrease rendering time by reducing the number of views and layers
- Lower memory usage, preventing crashes and improving stability
- Improve overall responsiveness for a smoother user experience
Ongoing Maintenance
Maintaining a simplified view hierarchy requires moderate effort. As your app evolves, you'll need to regularly review and refine the view hierarchy, ensuring new features don't introduce unnecessary complexity. You may also need to optimize layout constraints and view configurations for optimal performance.
To reduce view hierarchy complexity, consider these techniques:
Technique | Description |
---|---|
Simplify Hierarchy | Reduce the number of views and layers to minimize rendering time and memory usage. |
Avoid Transparency | Minimize view transparency to reduce rendering complexity. |
Optimize Layout Constraints | Use efficient layout constraints to reduce calculation overhead and improve rendering performance. |
Lazy Loading | Load views and content dynamically to reduce initial rendering time and improve performance. |
sbb-itb-bfaad5b
8. Use Auto Layout Wisely
Auto Layout is a powerful tool for creating user interfaces in iOS apps. However, if not used properly, it can slow down your app's performance. To optimize performance, it's crucial to understand how Auto Layout works and how to use it efficiently.
Moderate Effort Required
Using Auto Layout wisely requires some effort. You'll need to understand the basics, like creating constraints, using size classes, and resolving layout issues. Additionally, you'll need to optimize your layout constraints to reduce calculation overhead and improve rendering performance.
Performance Impact
Optimizing Auto Layout can significantly improve your app's performance:
- Reduce rendering time by minimizing constraint calculations
- Lower memory usage by avoiding unnecessary views and layers
- Improve overall responsiveness for a smoother user experience
Techniques for Efficient Auto Layout
Technique | Description |
---|---|
Use Stack Views | Simplify layout and make it more flexible. |
Efficient Constraints | Implement constraints to reduce calculation overhead. |
Avoid Deep Hierarchies | Minimize transparency and deep view hierarchies. |
Size Classes | Use size classes to adapt to different screen sizes and orientations. |
Profile and Debug | Regularly profile and debug Auto Layout performance to identify bottlenecks. |
9. Profile and Debug Performance Regularly
Moderate Effort Required
Profiling and debugging performance regularly takes some work, but it's crucial to identify and fix slow areas in your app. You'll need to learn how to use Xcode's Instruments tool, choose the right template, and analyze the results.
Improves App Speed and Responsiveness
Regular profiling and debugging can significantly boost your app's performance:
- Reduce rendering time by minimizing calculations
- Lower memory usage by removing unnecessary views and layers
- Improve overall responsiveness for a smoother user experience
Techniques for Efficient Profiling and Debugging
To profile and debug performance regularly, follow these steps:
Technique | Description |
---|---|
Use Xcode's Instruments | Select the right template, like the Time Profiler, to analyze your app's performance. |
Analyze Results | Identify slow areas and opportunities for improvement in your app's performance. |
Optimize Code | Implement optimizations to reduce rendering time, memory usage, and improve responsiveness. |
Make it a Habit | Profile and debug regularly to ensure your app's performance stays optimal. |
10. Opt for Swift Over Objective-C
Simple to Implement
Choosing Swift over Objective-C can make your iOS app development process simpler. Swift's modern syntax and features like type inference and optionals make writing and maintaining code easier. With Swift, you can focus on your app's logic without worrying about memory management and performance optimization.
Faster Performance
Apps written in Swift are up to 2.6 times faster than those in Objective-C, according to Apple. Swift uses generics and higher-order functions, resulting in cleaner, more reusable code. Additionally, Swift's compiler optimizations and Automatic Reference Counting (ARC) ensure efficient and smooth app performance.
Easier Maintenance
Maintaining a Swift app is generally easier than an Objective-C app. Swift's syntax is more concise and expressive, making code easier to read and understand. You can also take advantage of modern development tools and frameworks like Swift Package Manager and Xcode, simplifying development and maintenance. Overall, choosing Swift over Objective-C can reduce the effort required to keep your app running smoothly and efficiently.
Aspect | Swift | Objective-C |
---|---|---|
Implementation | Simpler with modern syntax and features | More complex syntax and memory management |
Performance | Up to 2.6x faster | Slower compared to Swift |
Maintenance | Easier with concise syntax and modern tools | More effort required due to complex syntax |
11. Background Task Management with Background Modes
Managing background tasks is crucial for optimizing your iOS app's performance. Effective background task handling can significantly enhance the user experience and prevent battery drain. This section explores the importance of background task management using background modes.
How Difficult Is It?
Setting up background task management with background modes can be complex, especially for newcomers to iOS development. You need to declare the background modes your app supports in the Info.plist
file and handle state transitions by implementing specific methods in your app delegate. Additionally, you must understand how to transition between different states and respond to system notifications.
Performance Impact
Benefit | Description |
---|---|
Smoother UI | Moving resource-intensive tasks to the background prevents UI lag. |
Continuous Operation | Background modes allow your app to perform critical tasks even when not in the foreground. |
Overall Improvement | Effective background task management can significantly boost app performance. |
Ongoing Effort
Maintaining background task management with background modes requires continuous effort. You need to ensure your app is configured correctly and handles state transitions and system notifications properly. Additionally, you must monitor app performance and make adjustments as needed to prevent battery drain and ensure a seamless user experience.
12. Implement Smart Prefetching
Prefetching is a technique that loads data or content before the user requests it. This can significantly improve your iOS app's performance by reducing loading times and enhancing responsiveness.
How Difficult Is It?
Implementing prefetching requires careful planning:
- Identify critical data or content to prefetch
- Determine the optimal timing for prefetching
- Ensure prefetching doesn't interfere with normal app operation
- Consider factors like network connectivity, device storage, and battery life
Performance Benefits
Benefit | Description |
---|---|
Faster Loading | Prefetching reduces data loading times for a quicker user experience. |
Improved Responsiveness | By loading data in advance, prefetching minimizes lag and stuttering. |
Enhanced User Experience | Smart prefetching provides a seamless and intuitive app interaction. |
Ongoing Maintenance
Maintaining prefetching requires continuous effort:
- Monitor app performance and adjust prefetching settings as needed
- Ensure prefetching doesn't interfere with other app features
- Consider updates to app content or data and adjust prefetching strategy accordingly
13. Optimize Battery Consumption
Ensuring your app doesn't drain the device's battery is crucial for a smooth user experience. Here's how to optimize battery consumption:
Implementation Effort
Optimizing battery usage requires careful planning. You need to identify power-hungry areas like network requests, location services, and animations. Then, implement techniques like caching, batching, and optimizing algorithms to reduce power consumption.
Performance Impact
Optimizing battery consumption can significantly improve your app's performance:
- Increase battery life
- Improve app responsiveness
- Enhance overall user experience
Ongoing Maintenance
Maintaining optimized battery usage requires continuous effort:
- Monitor app performance and battery life
- Identify areas for improvement
- Update your app regularly for optimal performance
Tips for Battery Optimization
Technique | Description |
---|---|
Minimize Background Activity | Reduce unnecessary background tasks and processes. |
Use Energy-Efficient Coding | Implement coding practices that minimize power consumption. |
Optimize Animations and Graphics | Ensure animations and graphics are optimized for performance. |
Use Battery-Saving APIs | Utilize APIs like Android Battery Saver Mode and iOS Background Modes. |
14. Utilize Content Delivery Networks (CDNs)
Content Delivery Networks (CDNs) play a key role in boosting the performance of iOS apps. By distributing content across multiple servers worldwide, CDNs reduce latency, improve loading times, and provide a smooth user experience.
Setting Up a CDN
Integrating a CDN into your iOS app requires careful planning:
- Choose the right CDN provider
- Configure the CDN
- Optimize app assets
- Integrate the CDN with your app
- Test the app
While the process may seem complex initially, the benefits of improved performance and user experience make it worthwhile.
Performance Benefits
Utilizing a CDN can significantly enhance your app's performance:
- Faster Load Times: By caching content at edge locations closer to users, CDNs reduce the distance data has to travel, resulting in faster load times.
- Improved Responsiveness: With reduced latency, your app will feel more responsive and snappy.
- Seamless User Experience: Faster load times and improved responsiveness contribute to a better overall user experience.
Ongoing Maintenance
Maintaining a CDN requires continuous effort:
Task | Description |
---|---|
Monitor Performance | Keep an eye on CDN performance and optimize as needed. |
Update Assets | Update app assets and configurations regularly. |
Ensure Integration | Ensure seamless integration with your app. |
15. Stay Updated with the Latest iOS SDKs and APIs
Keeping your app up-to-date with the latest iOS SDKs and APIs is key for optimal performance. Apple regularly releases new SDKs and APIs that improve speed, security, and features. By updating, you can take advantage of these enhancements and keep your app competitive.
Easy to Implement
Updating to the latest iOS SDKs and APIs is generally straightforward, especially if you're familiar with the development environment. However, you may need to:
- Update your code
- Modify project settings
- Test for compatibility
Boosts Performance
New SDKs and APIs often provide:
- Improved Performance: Faster app speed and reduced latency.
- Enhanced Security: Better protection against threats.
- New Features: Access to the latest capabilities.
By implementing these updates, you can deliver a better user experience, leading to increased engagement and retention.
Ongoing Maintenance Required
Maintaining compatibility with the latest iOS SDKs and APIs requires continuous effort:
Task | Description |
---|---|
Check for Updates | Regularly review new releases. |
Test Your App | Ensure compatibility with updates. |
Make Changes | Modify code and settings as needed. |
While this requires work, it's worthwhile to keep your app competitive and provide the best user experience.
Conclusion
Optimizing your iOS app's performance is crucial for delivering a smooth and engaging user experience. By implementing the 15 techniques discussed, you can significantly enhance your app's speed, responsiveness, and overall performance.
Remember, app performance optimization is an ongoing process that requires continuous monitoring and adjustments. Stay updated with the latest iOS SDKs and APIs, and regularly profile and debug your app to identify areas for improvement.
Prioritizing performance optimization can increase user engagement, retention, and drive business success. Don't let poor performance hold your app back - take control of your app's performance today and provide a faster, more responsive, and enjoyable user experience.
For further learning, explore additional resources and tools that can help optimize your iOS app's performance, such as Apple's developer documentation, performance optimization guides, and third-party libraries and frameworks.