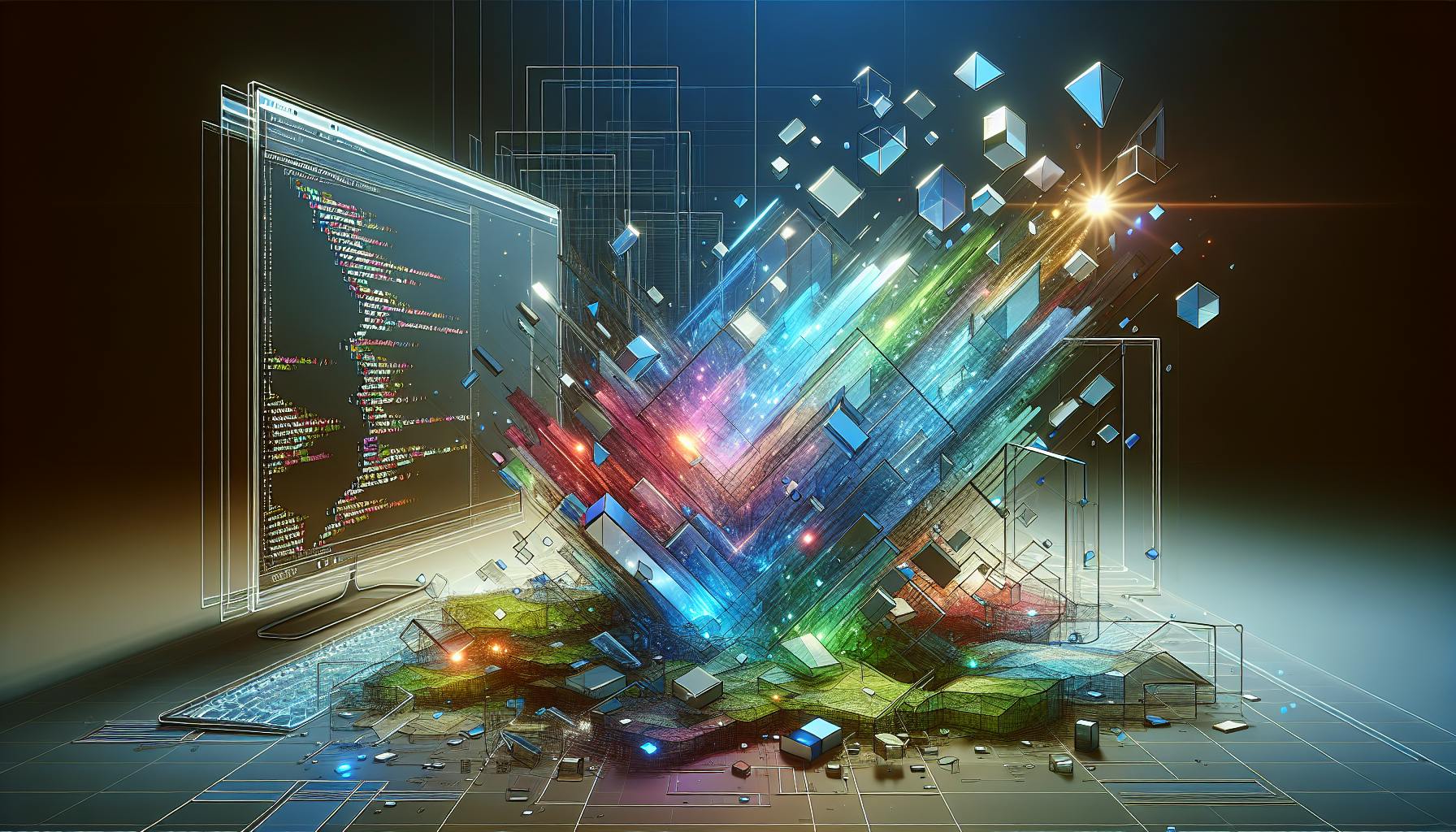
Discover the best CSS practices for clean, efficient, and scalable code. Learn about maintainability, scalability, performance, code styling, efficiency, and more.
Making your CSS code clean, efficient, and scalable is crucial for front-end development. Here's a quick guide to best practices:
- Maintainability: Use consistent spacing, meaningful names for classes/IDs, and comment on complex code sections.
- Scalability: Organize CSS into smaller, manageable files and use preprocessors like Sass for variables and mixins.
- Performance: Minimize file sizes by removing unused CSS and compressing files. Use CSS sprites to reduce image requests.
- Code Styling: Adopt a mindful file structure, validate your code, and use semantic HTML for better readability and SEO.
- Efficiency: Utilize modular CSS for reusability, leverage preprocessors for streamlined coding, and prioritize low specificity selectors to avoid conflicts.
- Optimization: Embrace shorthand properties and custom properties for cleaner code and easier maintenance.
- Responsive Design: Adopt a mobile-first approach with fluid layouts and consolidated breakpoints for a seamless cross-device experience.
- Naming Conventions: Use systems like BEM or SMACSS for clear, organized CSS that's easier to understand and maintain.
- Debugging and Testing: Utilize browser developer tools and automated testing to ensure your CSS works across all platforms and devices.
- Collaboration: Implement version control systems for better teamwork and conduct thorough code reviews for consistent quality.
By following these practices, you'll create websites that are not only visually appealing but also performant, maintainable, and accessible across all devices.
Improved Maintainability
- Keep your code looking neat with consistent use of spaces and lines. It makes it easier to read and understand at a glance.
-
Choose names for your classes and IDs that tell you what they do or what they're for. Avoid vague names like
.box
or.blue
which can be confusing. - If you have to do something tricky with your CSS, leave a note in your code to explain it. This helps anyone who looks at your code later on.
Enhanced Scalability
- Break your CSS into smaller pieces, like _variables.scss and _components.scss, to keep things organized. This makes your code easier to handle as your project grows.
- Try using tools like Sass that let you use variables, nest your code, and mix pieces together. This can make your CSS neater and more flexible.
Faster Page Loads
- Make your CSS files smaller by getting rid of extra spaces, lines, and comments. Smaller files mean your website loads faster.
- Turn on compression on your web server. This squishes your files down, making them quicker to download.
- Combine multiple images into one big image using CSS sprites. This means your website doesn't have to load lots of separate images.
In short, using good coding habits for your CSS can make a big difference in how easy your website is to keep up to date, how well it can grow, and how fast it loads. It's worth the effort to get these things right.
Code Styling Essentials
Mindful File Structure
When you start a new project, think about how you'll organize your files and folders. Group similar files together in folders named for their purpose, like "styles" for CSS files, or "images" for all your pictures. Use clear names for your files, such as _variables.scss
for Sass variables and components.css
for CSS parts you'll use more than once.
Getting your file structure set up right from the start helps keep everything easy to manage. This way, you won't waste time later trying to find files in a messy folder.
Code Validation
Before your website goes live, check your HTML and CSS to make sure there are no mistakes. Tools like the W3C CSS Validator and W3C Markup Validation Service can help you spot problems like:
- Tags you forgot to close
- Elements inside the wrong ones
- CSS properties with wrong values
Fixing these issues makes sure your site works well in different web browsers. It's a step that shows you care about making your site reliable and easy to use.
Semantic HTML
Using the right HTML tags, like <header>
, <nav>
, <main>
, etc., helps explain what each part of your page is for. This is not just good for people using your site, but also for search engines and tools that help people with disabilities. It also makes your CSS easier to work with. For example:
header {
/* Styles for the top part of your site */
}
nav ul {
/* Styles for your navigation list */
}
Choosing the right HTML tags makes your site easier to change and improve later. It helps keep your code clean and makes sure your website is set up in a logical way.
Organizing and Structuring CSS
Keeping your CSS code tidy and well-arranged makes it easier to read, maintain, and work with others. By sticking to a clear plan and breaking things down into smaller parts, you can handle bigger projects without getting lost in the code.
Modular CSS
Think of your CSS like building blocks:
-
Keep styles for each part of your website, like buttons or cards, in their own file. For instance:
button.scss card.scss modal.scss
- These parts should be like LEGO pieces that you can use and move around easily.
- Make sure the styles for one part don't mess up another. This keeps everything working smoothly, even if you make changes.
- Breaking your code into these blocks helps everything stay organized and makes updates easier.
CSS Preprocessors
Using tools like Sass can make writing CSS faster and simpler.
- Variables let you set a theme color or font once and use it everywhere, keeping your design consistent:
$brand-color: #108cf0;
.button {
background: $brand-color;
}
- Mixins help you avoid writing the same code over and over. They're like shortcuts:
@mixin flexCenter {
display: flex;
align-items: center;
justify-content: center;
}
.modal {
@include flexCenter;
}
- Nesting makes it easier to style parts of your page that are inside others, without making your code messy:
.card {
.title {
font-size: 1.2rem;
}
}
By using these tools and organizing your code into blocks, you can keep your CSS tidy and ready to grow with your projects.
Selector Efficiency
Low Specificity Selectors
When you style your web pages, it's better to use classes and IDs for targeting elements because they make your styles more flexible. This way, you avoid problems with too specific selectors.
For instance, rather than doing this:
h2 {
/* styles */
}
Try this:
.page-heading {
/* reusable styles */
}
This method lets you use the same style for different headings just by adding a class, making your code cleaner and easier to manage.
Limited Selector Nesting
Having too many layers of selectors can make your CSS complicated, leading to styles that clash or override each other when you didn't mean them to. Try to keep your selectors simple and not too deeply nested.
Instead of this:
.page .content .title {
/* styles */
}
Go for:
.page-title {
/* reusable styles */
}
By keeping nesting to a minimum, you avoid these problems and make your styles more versatile across your website.
Optimizing CSS Property Usage
Shorthand Properties
Shorthand properties in CSS let you write less code to do the same job. Instead of writing out each property one by one, you can combine them into a single line. This makes your code cleaner and saves time.
For example, you can set all four sides of margin
or padding
with just one line. Or, use background
to add an image, position it, and decide if it should repeat—all at once. The font
property can include style, weight, size, line height, and family in one go.
So, instead of writing:
margin-top: 10px;
margin-right: 20px;
margin-bottom: 10px;
margin-left: 20px;
You can simply write:
margin: 10px 20px;
This method helps keep your code short and sweet, making it easier to handle.
CSS Custom Properties
CSS variables, or custom properties, let you store values that you use a lot in one place. This way, if you need to change something like a color or font across your website, you can do it in one spot instead of everywhere.
For example, you might set a main color and font like this:
:root {
--primary-color: #3498db;
--font-family: \"Roboto\", sans-serif;
}
Then, you can use these variables in different places:
h1 {
color: var(--primary-color);
font-family: var(--font-family);
}
button {
background: var(--primary-color);
font-family: var(--font-family);
}
If you decide to change --primary-color
or --font-family
, the new value automatically updates everywhere those variables are used. This makes tweaking your site's look super easy.
Responsive Design
A mobile-first approach with fluid units like percentages and optimized, consolidated media queries streamlines responsive design.
Mobile-First Fluid Layouts
Starting with mobile designs and using flexible units such as percentages (width: 100%
) and viewport units (width: 100vw
) helps your website automatically adjust to fit any screen size. This approach makes your site work well on all devices, from phones to desktops.
Here's why this method is good:
- Your site fits the screen without making users scroll sideways or deal with content spilling over.
- Everything scales up or down smoothly as the screen size changes.
- You don’t have to design separate versions for each device size.
- It lets you focus on making the mobile version great first, then add more features for larger screens.
Using this flexible design from the start means your website is ready for any device right away.
Consolidated Breakpoints
Using fewer media queries for common screen sizes makes your CSS cleaner and easier to work with. Instead of having a bunch of separate rules for tablets and desktops, you can group them together:
/* Tablet & Desktop */
@media (min-width: 768px) {
/* Shared rules */
body {
font-size: 1.2rem;
}
/* Tablet rules */
/* Desktop rules */
}
This approach has several benefits:
- You avoid repeating the same code, making your CSS more organized.
- It’s easier to manage and update styles that target similar screen sizes.
- Grouping related styles together makes your code more efficient.
- It improves the experience for developers when they need to make changes.
By smartly grouping your media queries, you make your CSS more straightforward and your website easier to maintain.
CSS Naming Conventions
BEM
BEM stands for Block, Element, Modifier. It's a smart way to name your CSS stuff so everything stays organized.
- Blocks are big pieces like headers or buttons.
- Elements are parts of blocks, like a button icon.
- Modifiers change how blocks or elements look or act, like making a button a primary button.
Here’s how you might see it in code:
/* Block */
.button {
}
/* Element */
.button__icon {
}
/* Modifier */
.button--primary {
}
Using __
for elements and --
for modifiers helps show what belongs to what. It keeps your code clean and makes sure things don’t mix up.
SMACSS
SMACSS, or Scalable and Modular Architecture for CSS, sorts your CSS into clear groups:
- Base - Basic styles for things like headings and links.
- Layout - Big layout pieces, like grids or navigation bars.
- Modules - Parts you can use over and over, like cards.
- State - Changes in style for things like hidden or active items.
- Theme - Different looks, like a dark mode.
For instance:
/* Base */
body {
font-family: "Arial";
}
/* Layout */
.grid {
display: grid;
}
/* Module */
.card {
}
/* State */
.is-collapsed {
}
/* Theme */
.theme--dark {
}
This method helps keep your styles neat and makes it easier to know what each class is for.
sbb-itb-bfaad5b
Writing Readable CSS
Code Formatting
Making your CSS easy to read and manage starts with how you format it. Here are some simple tips:
- Indentation: Use spaces to create a visual hierarchy in your code. This means making nested selectors look like they are tucked under their parents by adding spaces at the beginning of the lines. Stick to either 2 or 4 spaces and avoid using the Tab key.
- Property Order: Keep your code organized by grouping similar properties together. For example, start with how you position something, then describe its size, and finally, its appearance.
- Quotes: Decide if you want to use single (' ') or double (\
Performance Optimization
Unused CSS Removal
Think of PurgeCSS as a cleanup crew for your CSS files. It looks through your website's HTML and JavaScript to find any CSS styles that you're not actually using. Then, it gets rid of those unused styles, making your CSS file smaller.
This step can make your website load faster because it shrinks the size of your CSS files. By removing what's not needed, your site can work quicker without losing any of its looks or functions.
Minification
Minification is about making your CSS files as small as possible. It does this by taking out all the spaces, comments, and anything else that's not needed for the code to work. Even though the code ends up looking squished together, it still does its job just the same.
You can use online tools or programs like Webpack to automatically make your CSS files smaller. Here's why it's a good idea:
- It reduces the size of your CSS files, so they load faster.
- It gets rid of extra spaces and comments.
- It makes some changes to your code (like shortening names) that don't affect how it works but make the file smaller.
When you combine getting rid of unused CSS with minification, you can make your website's CSS files much lighter. This means your site can load quicker on people's computers and phones, without changing how it looks or works.
Browser Compatibility
Autoprefixer handles adding special browser codes. Using smart tricks, we make sure websites work well on old and new browsers.
Vendor Prefixing
Autoprefixer is a tool that automatically adds special codes to your CSS so your website looks good in different browsers. It adds little bits like -webkit-
or -moz-
to make sure everything works as it should.
For example, if you're using fancy grid layouts, Autoprefixer will add the necessary bits to make sure it looks right in various browsers:
.grid {
display: -ms-grid;
display: grid;
}
This tool makes your life easier by handling these browser differences for you.
Supporting Legacy Browsers
It's important to make sure your website can be used by everyone, even people using older browsers. We do this using two main ideas:
Progressive enhancement means starting with the basics that everyone can access, like text and pictures, and then adding more fancy stuff for newer browsers. Think of it as building a house starting with the foundation and then adding extra floors.
Graceful degradation is the opposite. You start with all the fancy features, then tweak it so it still works on older browsers. It's like making sure a fancy smartphone app can still do the basic stuff on an older phone.
Testing your website on different browsers helps find any issues. Sometimes, you might use special scripts called polyfills to add new features to old browsers.
By planning and testing, you can make sure your website uses the latest features without leaving anyone behind.
Debugging and Testing
Browser Developer Tools
Every web browser comes with built-in tools like the Inspector and Console. These are super helpful for figuring out CSS problems. With these tools, you can:
- Look at the CSS rules for any part of a webpage and see where conflicts might be coming from.
- Turn CSS properties on and off to check what they do right away.
- Check out issues with how things are laid out, like if something's in the wrong spot.
- Find CSS rules you're not using, so you can remove them and make your files smaller.
- Quickly solve styling issues without having to change your original files.
Using these tools is key for fast problem-solving when you're working on the look of websites. They make fixing CSS issues a lot easier.
Automated Testing
Tools like Selenium and Cypress let developers set up tests that automatically check if the CSS is working right across different browsers and devices. These tests are great for:
- Spotting differences in how pages look or work on various browsers.
- Making sure that things like hover effects and animations are doing what they're supposed to.
- Checking that updates to CSS don't mess up something that was working fine before.
- Feeling sure that your CSS will look and work as expected before you show it to everyone.
Setting up automatic tests makes working with CSS safer because you can change things without worrying as much about breaking your site. Running these tests, especially to catch visual changes, is a smart move for websites that rely a lot on CSS.
Collaboration Workflows
Version Control Systems
Tools like Git help teams work together smoothly by letting them make changes separately without messing up the main project.
- Branching lets team members work on different parts at the same time. Changes in branches don't mix with the main project until they're ready.
- Merging is when you combine the changes from these separate branches back into the main project. If two changes clash, you'll need to sort that out.
- Pull requests on websites like GitHub and GitLab are a way to talk about changes before adding them to the main project. This makes sure everything fits the team's standards.
Using Git is key for teams to work together without stepping on each other's toes and keeping the project safe.
Code Reviews
Looking over each other's work is really important for catching mistakes and keeping things consistent.
- Checking CSS changes makes sure everyone is following the rules, like how to name things or write efficient code.
- Giving and getting feedback helps everyone learn and make better code.
- Spotting issues like clashing styles early stops bigger problems later.
- Tools can also check the code for common mistakes, security issues, and how fast it runs.
Having these reviews helps everyone get better, share what they know, and keep the project easy to manage. It's like having a safety net to catch problems before they happen.
Continuous Learning
Keep up with the latest in CSS by checking out new updates and trying out different tools and methods like CSS-in-JS.
Specifications Updates
It's important to regularly check places like MDN Web Docs to keep up with the latest changes in CSS and new features. Things like CSS Grid, Flexbox, and Custom Properties are getting better all the time, and they can make your work a lot easier.
Keep an eye on what the W3C is doing and sites like caniuse.com to see what new CSS features are coming. Looking into what's being developed can help you figure out if there's something new that could be useful for your projects.
Joining discussions on websites like CSS-Tricks and Smashing Magazine can also help you learn about what's new and what other developers are talking about.
New Libraries and Approaches
The world of CSS is always growing, with new tools and ways of doing things that can make coding easier. It's a good idea to explore these to see if they could make your work better.
CSS-in-JS tools like Styled Components and Emotion let you use CSS inside JavaScript. This means you can change styles based on what's happening in your app, use themes, and make sure styles are only applied to the parts they're meant for. It's worth checking these out to see if they fit your projects.
Other tools like CSS Modules help avoid mix-ups by giving styles unique names. And then there's Tailwind, which uses a different approach by letting you build up styles by adding classes in your HTML.
Even though regular CSS is still very useful, looking into these new tools and methods can help you stay up-to-date and maybe find easier ways to do your work. Trying new things is a great way to learn and keep improving.
Conclusion
Making sure you use CSS the right way is key for creating websites that are easy to keep up with and work well. Starting off with code that's neat and does its job efficiently means your website will be set for success in the long run.
Here's a quick summary:
- Be smart with your choices: It's better to use classes and IDs than tag names. Keep things simple and don't overcomplicate your CSS.
- Break it down: Organize your code into small, reusable bits.
- How you name things matters: Stick to clear, meaningful names like those suggested by BEM.
- Keep it neat: Use spaces and comments to make your code easy to read.
- Use shortcuts and variables: This helps avoid repeating yourself and keeps your design consistent.
- Think mobile-first: Using flexible sizes and smart breakpoints makes your site work on any device.
- Cut out the excess: Tools like PurgeCSS help by removing styles you don't use.
- Make it work everywhere: Adding prefixes and planning for older browsers means everyone can enjoy your site.
While it takes some effort to stick to these good habits, the benefits are huge. Your site will load faster and be more stable. Working with others on your project becomes easier. And, you'll be grateful later for a codebase that's easy to manage. Checking back on these tips now and then can help you see where you can do better. With a little attention and care, your CSS will be the best it can be.