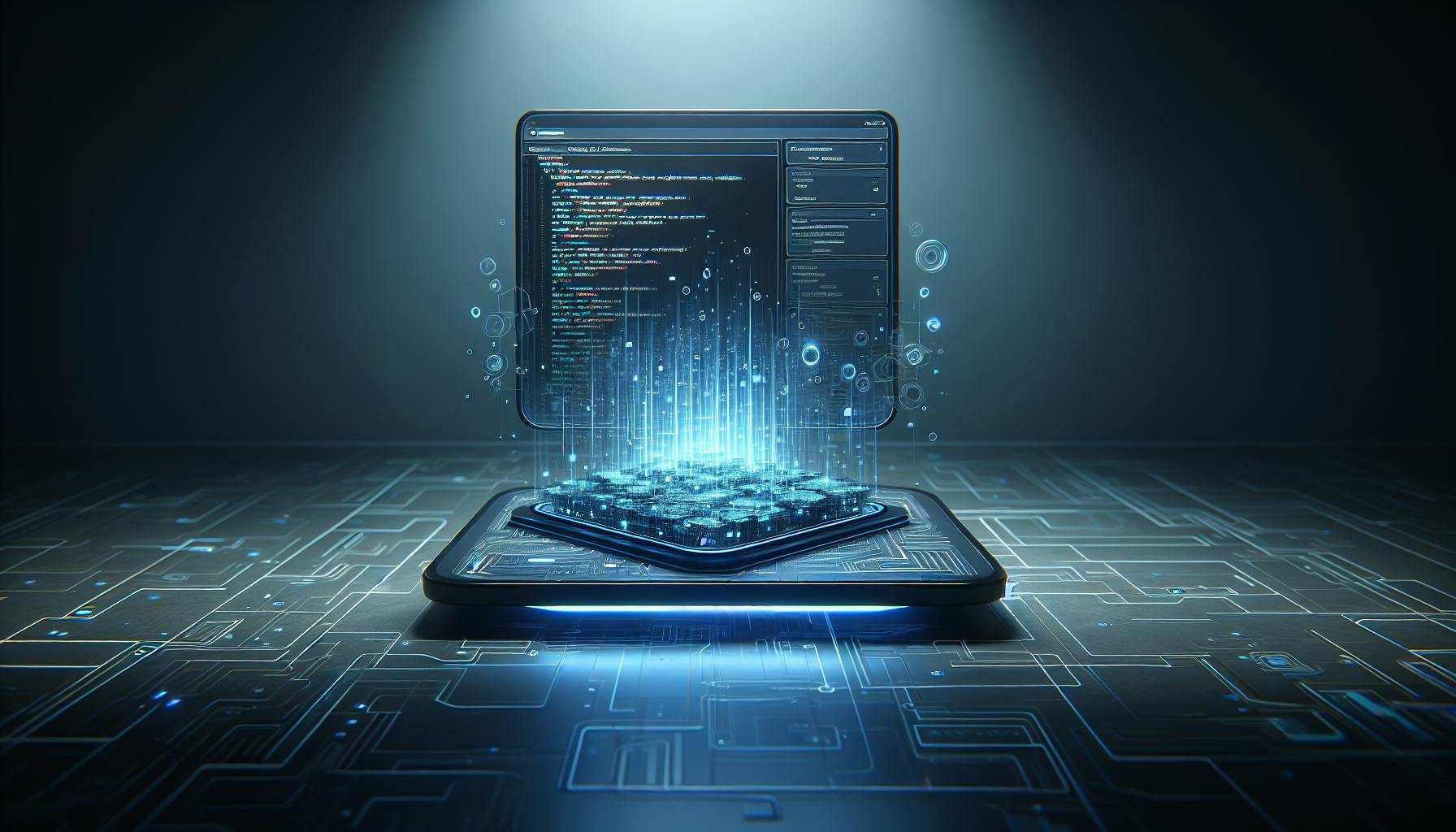
Improve your JavaScript skills with this concise guide to writing efficient, clean, and faster code. Learn about modern JavaScript features, DOM manipulation, error handling, code organization, performance optimization, and leveraging tools.
Looking to improve your JavaScript skills? Here's a concise guide to writing efficient, clean, and faster JavaScript code:
- Embrace Modern JavaScript: Utilize
let
,const
, arrow functions, async/await, and other ES6+ features for cleaner code. - Optimize Loops and Data Handling: Use array methods like
.map()
and.forEach()
for better performance. - Efficient DOM Manipulation: Make changes in batches and use frameworks to minimize direct DOM changes.
- Asynchronous Programming: Use
async/await
for better readability and handling of asynchronous operations. - Error Handling: Implement
try...catch
blocks to manage errors gracefully. - Code Organization: Keep your code modular with ES6 modules and avoid global variables to reduce conflicts.
- Performance Optimization: Minify and bundle your code, optimize network requests, and choose efficient data structures.
- Tooling: Utilize tools like Visual Studio Code or WebStorm and libraries thoughtfully, considering factors like size and performance.
This guide is designed to make your JavaScript projects more efficient and your code easier to maintain and understand.
What is JavaScript?
JavaScript is a programming language that makes websites interactive. It’s what allows you to have moving elements, forms that respond as you fill them out, and more on web pages. Here’s what you should know about it:
- JavaScript code works in your web browser, making pages react quickly to what you do without having to wait for the server.
- It’s one of the main tools for making websites along with HTML and CSS. They all work together to build the parts of a website you interact with.
- Although JavaScript looks a bit like the programming language C, it’s actually a scripting language. This means it runs right away in your web browser, without needing to be turned into another form first.
- JavaScript isn’t just for making websites look good. It’s also used on servers with something called Node.js, in mobile app development, and even in making games.
In short, JavaScript lets web pages do cool stuff, working right in the browser to make sites lively and interactive.
JavaScript Syntax Modernization
Since 2015, JavaScript got some updates that made writing code easier and cleaner:
- let and const - These are ways to name things in your code that are better than the old way (using var).
- Arrow functions - A shorter way to write functions, which are bits of code that do something.
- Template literals - A way to mix words and data together in a string using backticks (`).
- Destructuring - A shortcut for pulling specific items out of a list or object and giving them their own names.
- Promises - A way to deal with code that runs on its own time, like downloading a picture. It lets you say, "Do this, then do that when you’re done, or let me know if there’s a problem."
- Classes - A template for making objects that can do certain things and know certain information.
And there are more cool updates:
- Async/await - Helps you write code that waits on something, like data from the internet, in a way that’s easy to read.
- Import/export modules - Lets you organize your code into different files so it’s easier to handle.
- Enhanced object literals - A simpler way to make objects, which are collections of data and actions.
- Optional chaining - A safety net that prevents errors if you try to get information that isn’t there.
These updates help make JavaScript code easier to write, read, and manage, especially for big projects.
Writing Efficient JavaScript
JavaScript is a super handy language that lets you make web pages do fun and interactive stuff. But to keep everything running smoothly, it's important to write your code in a smart way. Let's dive into some key tips for making your JavaScript code work better.
Proper Variable Declarations
When you're naming things in your code, it's best to use let
and const
instead of var
:
let
is for things that might change.const
is for things that stay the same.var
is old-school and can lead to mistakes.
For example:
// Use const for things that don't change
const API_KEY = '123456789';
// Use let for things that might change
let count = 0;
count++;
// Try to avoid using var
Choosing let
and const
helps make your code clearer and keeps you from running into unexpected issues.
Optimizing Loops
When you're working with lists (arrays), it's a good idea to use special commands like .forEach()
, .map()
, and .filter()
instead of the old for
and while
loops. These commands are not only easier to read but also tend to be more efficient:
// This is a good way to do it
const numbers = [1, 2, 3];
numbers.forEach(n => {
// do something with n
});
// This way is not as good
for (let i = 0; i < numbers.length; i++) {
// do something with numbers[i]
}
Plus, try to figure out how big your list needs to be ahead of time, instead of making it bigger little by little.
Minimizing DOM Manipulation
Changing web page elements too often can make your site slow. To avoid this:
- Group changes together before making them all at once.
- Use tools like React that don't change the page directly.
- Wait a bit before making changes if lots of them are happening fast.
This helps your pages run faster and smoother.
Embracing Asynchronous JS
Using async/await
lets your code wait for things like data from the internet without stopping everything else. This makes your site feel quicker.
async function fetchData() {
const response = await fetch(url);
const data = await response.json();
return data;
}
It's like saying, "Go get this data, but keep everything else running while you wait."
Error Handling
Good error handling keeps small problems from turning into big crashes:
- Use
try/catch
to catch errors in risky spots. - Write down errors with details so you can fix them.
- Make sure your site keeps working, even if there's an error.
For example:
try {
riskyCode();
} catch (error) {
console.log(error, contextDetails);
showFallback();
}
This way, you're ready for errors and can handle them without stopping your site.
By following these tips, you'll make your JavaScript code work better and faster.
Code Structure and Organization
Modularization
It's smart to keep your JavaScript code in different files and sections, especially if you're working on a big project. Here's how:
-
Break up your code based on what it does. For example, keep all the login stuff in one file, and all the chat stuff in another.
-
Use ES6's import and export to let pieces of your code talk to each other. Like this:
// message.js
export function displayMessage() {
// function code
}
// app.js
import {displayMessage} from './message.js';
-
Only share code between files if you really need to. Not everything needs to be exported.
-
Make sure your code doesn't go in circles with imports (like file A needing something from file B, but file B also needs something from file A).
Planning your code like this makes it cleaner and easier to work with.
Avoiding Global Variables
Keeping your code to itself helps avoid mix-ups:
- Wrap your code in an IIFE (a function that runs right away) so it doesn't spill out:
(function() {
// code here
})();
-
Use
let
andconst
for your variables, notvar
, which can be seen everywhere. -
If you have data or utilities that need to be shared, put them in one big object instead of spreading them out:
const App = {
data: {},
utils: {}
};
This way, your data is neat and not all over the place.
Naming Conventions
Picking good names for things in your code makes it easier to understand:
-
Use camelCase for naming variables and functions.
-
Use PascalCase for naming classes.
-
Start global constants with GLOBAL_ so you know they're important and everywhere.
-
Give functions names that tell you what they do.
-
Choose meaningful names for your variables, not just a, b, c.
Sticking to these naming rules helps everyone get what your code is about.
Comments and Documentation
Leaving notes in your code helps others (and you later) understand it:
-
Give a quick overview of what sections of code do.
-
Use comments to explain tricky parts or why you made certain choices.
-
Document functions with JSDoc so tools can help explain them to you.
-
Keep a README file that talks about your project, how to set it up, and how it works.
-
Don't leave a bunch of commented-out code or notes on super obvious stuff.
Good notes make your code friendlier for everyone.
Performance Optimization
Minification and Bundling
Making your JavaScript files smaller and combining them helps your website load faster. Here's how you can do it:
-
Use tools like Webpack or Parcel to mix several JavaScript files into one. This means fewer pieces for the browser to load.
-
Turn on options in your tool to make files smaller by removing extra spaces and making names shorter without changing what the code does.
-
Split your code into smaller parts that load only when needed. This stops unnecessary code from loading.
-
Get rid of code you're not using with a trick called tree shaking.
-
Also, make images, CSS, and HTML smaller along with your JavaScript code.
Doing all this can really help your website load quicker.
Network Request Optimization
Making sure the browser doesn't ask for more than it needs is key:
-
Use cache settings so the browser doesn't keep asking for the same stuff.
-
Use CDNs to send your content from a place closer to your users. This makes things faster.
-
Load big things like images only when you need them, not all at once.
-
Avoid too many redirects that make extra trips.
-
Make things smaller with gzip to cut down on transfer size.
-
Don't send all your requests at once. Spread them out.
-
Have a plan for when requests don't go through, like setting timeouts and retries.
Planning your network requests can make your website more efficient.
Efficient Data Structures
Picking the right way to store and use data makes your code run better:
-
Use Maps for quick lookups and changes instead of objects.
-
Use Sets when you just need a list without key-value pairs. They're faster for checking if something is there.
-
Choose array methods like .filter and .map instead of slow loops.
-
When you need specific types of numbers, use typed arrays like Int32Array.
-
If you have complicated data, try to simplify it. This makes accessing data quicker.
Knowing which data structure to use can make your code more efficient.
sbb-itb-bfaad5b
Leveraging Tools and Libraries
Overview of helpful tools and tips for selecting libraries.
Comparison table in Markdown format with key criteria like supported languages, debugging, extensions, pricing etc.
Feature | Visual Studio Code | WebStorm |
---|---|---|
Supported Languages | JavaScript, TypeScript, Node.js, Python, Java, C++, C#, PHP and more with extensions | JavaScript, TypeScript, Node.js, HTML, CSS, SQL and more |
Debugging | Built-in debugger with breakpoints, call stacks and an interactive console | Robust debugger with breakpoints, watches and more |
Extensions | Huge extension marketplace with tools for linting, IntelliSense, themes and more | Wide array of plugins available |
Pricing | Free with open source | Starts at $199/year for personal license |
Both Visual Studio Code and WebStorm are great for writing JavaScript. VS Code is lighter and can be customized with add-ons, while WebStorm has lots of built-in features for web projects. Think about your budget, what you need for your project, and what you like to use when choosing.
Choosing JavaScript Libraries
When picking a JavaScript library, think about:
-
Size: Smaller ones are usually easier to handle. Make sure you really need all the features it offers.
-
Performance: See how fast it does things like changing webpage elements or handling data. Lighter libraries usually work faster.
-
Browser Support: Some libraries might not work in older web browsers. Make sure it fits what you need.
-
Modularity: Some libraries, like Lodash, let you only use the bits you need, which helps keep things light.
-
Activity Level: Make sure the library is still being updated and that people are fixing bugs.
-
Licensing: Check if there are any rules about how you can use it, especially for work stuff.
Start with well-known ones like React, Vue, jQuery, and Lodash. But also look at simpler options like Alpine.js or date-fns for certain jobs. Focus on what you really need and try not to add stuff you won't use much.
Conclusion
When it comes to writing JavaScript, it's all about making code that's quick, easy to handle, and less likely to mess up. By sticking to the smart tips we talked about, you can:
- Make things run faster by choosing the right way to name things, making loops smarter, organizing data better, putting code together, and more.
- Make your code easier to read by using names that make sense, breaking your code into parts, and explaining your code with comments.
- Make your code more trustworthy by using exact matches, handling errors well, and building things step by step.
- Make your life easier by using cool shortcuts like async/await for code that waits around.
- Work better with others by writing clear code and not mixing everything together.
Even trying just a few of these smart moves can make your JavaScript code cleaner, simpler, and easier to keep up with. Start with what helps your project the most. As you get the hang of it, adding more good habits will feel like a breeze.
Aim for code that's easy to get, don't rush to make things complicated, and only tweak working code if you really need to. Finding the sweet spot leads to JavaScript that works well, grows with you, and sets you up for winning as your projects get bigger. Keep using these coding tricks, and you'll get better and better at JavaScript.
FAQs
How can I measure the performance of my JavaScript code?
To check how fast your JavaScript code runs, you can use tools built into your web browser, like Chrome's DevTools. Look for the Performance tab to see how long your code takes to run and find parts that might be slowing things down. Tools like Lighthouse are also great for spotting ways to make your web app faster.
What tools can I use to minify JavaScript code?
For making your JavaScript code smaller (which helps it load faster), you can use:
- UglifyJS
- Terser
- Closure Compiler
These tools strip out stuff like extra spaces and notes that aren't needed for the code to work.
How do I optimize network requests in JavaScript?
To make sure your web page doesn't wait too long for things to load:
- Bundle and combine files to reduce requests
- Use caching so your browser remembers stuff it's already seen
- Compress data to make it smaller
- Use async requests with something like the Fetch API to keep the page responsive
- Add timeouts and retry strategies for when things go slow
What is the best way to handle errors in JavaScript?
Good error handling means:
- Catching mistakes with
try...catch
so they don't crash your app - Keeping track of errors to help fix them
- Making sure your app still works, even if something goes wrong
- Showing a backup plan on the screen if there's an error
This way, small problems don't turn into big ones.
Should I regularly update JavaScript libraries and frameworks?
Yes, it's a good idea to keep your tools up to date. This gives you:
- Fixes for bugs
- Better speed
- New features to use
Always read the update notes, follow safe update steps, and test your code to make sure everything still works right after an update.
Related Questions
How can I make my JavaScript code more efficient?
To make your JavaScript code run better, you can:
- Make your code smaller by getting rid of extra spaces and comments. Tools like UglifyJS can help.
- Combine several scripts into one file to cut down on how many times your page has to ask for files.
- Use Gzip to make your files smaller so they move faster.
- Put your files on a CDN so they get to users quicker.
- Load scripts without blocking other stuff by using async.
- Put off loading scripts that aren't urgent.
- Keep some data on the user's device to reduce network requests.
- Make sure loops and functions are as simple as possible, and pick the right way to store data.
How to improve code quality in JavaScript?
To make your JavaScript code better, you should:
- Stick to common naming and coding styles.
- Split your code into parts with specific jobs.
- Get rid of stuff you don't need like extra variables or functions.
- Keep your code clean with consistent spaces and lines.
- Avoid making things too complicated.
- Explain tricky parts with comments.
- Check your code with tools like ESLint.
- Test different parts of your code to catch mistakes.
- Tidy up your code to keep it simple.
Which JavaScript code is more efficient?
Usually, a for
loop runs a bit faster than a while
loop in JavaScript because it doesn't check conditions as much. But, the difference is small. What's more important is writing code that's easy to read. Use for
loops for straightforward tasks and while
loops when you need more control. Making your code clear is more important than making it a tiny bit faster.
What is the best way to write JavaScript code?
Here are some good habits for writing JavaScript:
- Use
const
andlet
instead ofvar
. - Set up your variables when you create them.
- Prefer arrow functions for a cleaner look.
- Use destructuring to avoid messy references.
- Name your functions clearly.
- Always use strict equality (
===
). - Use try/catch to deal with mistakes.
- Break your code into small, focused functions.
- Comment on your code to explain the tough parts.
Following these tips will make your JavaScript code easier to work with and understand.