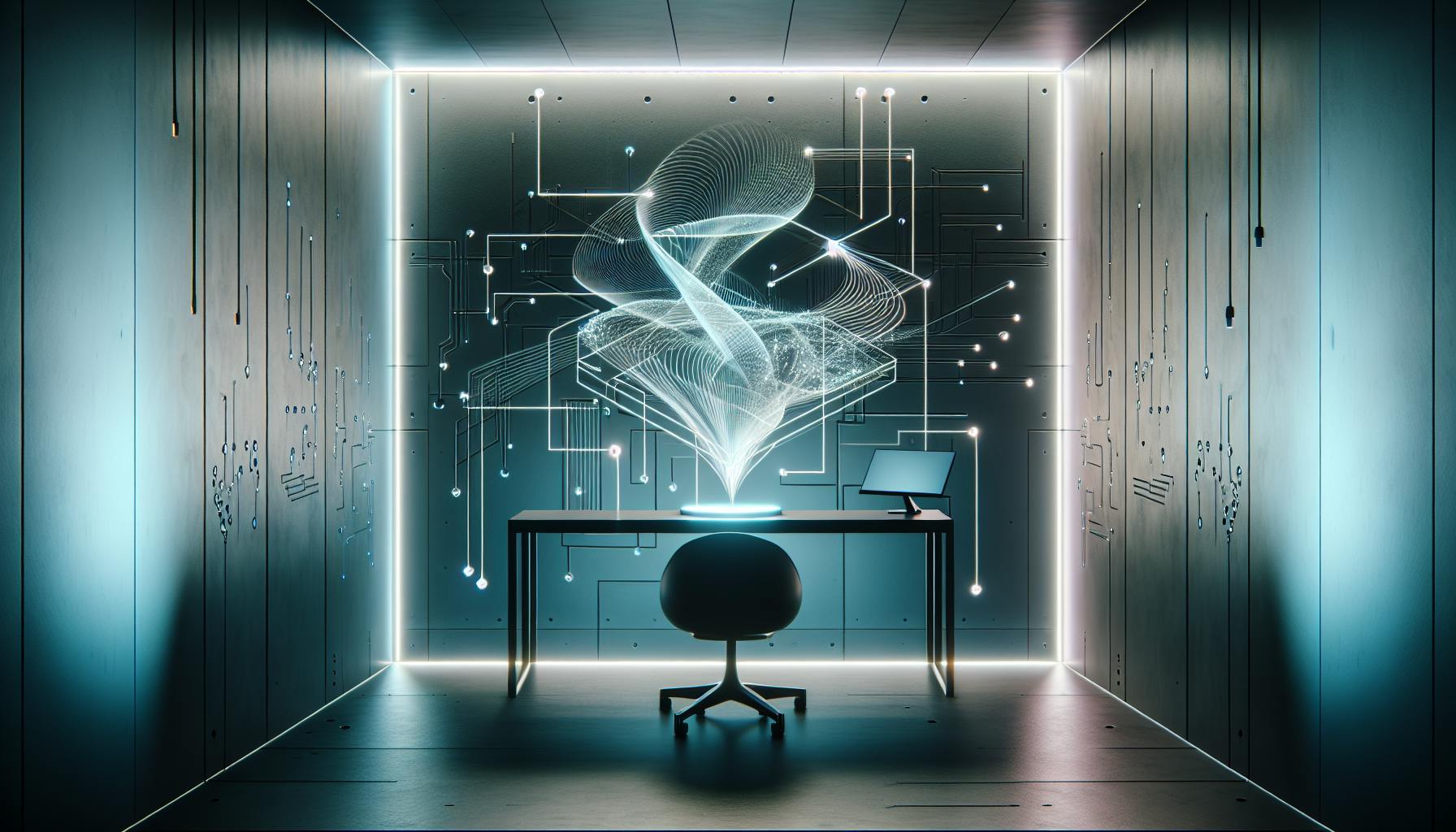
Learn the basics of Deno, a modern runtime for JavaScript and TypeScript that offers enhanced security and efficiency. Discover key features, installation steps, and how to write your first Deno program.
Deno is a modern runtime for JavaScript and TypeScript that aims to address some of Node.js's shortcomings, offering a more secure and efficient development experience. Here's what you need to know about Deno:
- Designed for Security: Deno runs your code in a sandbox ensuring scripts can't access your files or the network without permission.
- Supports TypeScript Out of the Box: You can run TypeScript code natively without additional tooling.
- Includes a Standard Library: Comes packed with a useful standard library for common tasks.
- Simplifies Module Management: Uses URLs for importing modules, eliminating the need for a package manager.
- Easy to Install and Use: You can quickly install Deno with a single command and start building applications.
Whether you're new to programming or an experienced developer, Deno offers an exciting platform for building web applications with advanced features and improved security.
Key Features and Benefits
Deno has some cool features that make coding better:
- TypeScript support - You can use TypeScript right away without setting anything up. This makes coding faster.
- Secure defaults - Your scripts can't access your files or the internet unless you allow it. This keeps things safe.
- Promise-based APIs - Deno uses promises, which are a modern way to handle tasks that take time, like downloading files.
- Standard library - Deno comes with useful tools and web features like
fetch
for getting data from the internet. - Single executable - Everything you need is in one file, making it easy to share and use your code.
These features help you write web apps more easily and safely, using the latest JavaScript tech without needing extra stuff.
Installation and Setup
Install Deno
To put Deno on your computer, follow these steps in your terminal:
MacOS/Linux:
curl -fsSL https://deno.land/x/install/install.sh | sh
Windows (PowerShell):
iwr https://deno.land/x/install/install.ps1 -useb | iex
This step grabs the latest Deno setup file and puts it in your home directory under ~/.deno
.
You can find other ways to install it, like using different folders or package managers, on the Deno website.
Verify Installation
To make sure Deno is ready to go, type this in your terminal:
deno --version
If you see a version number, you're all set.
For instance:
deno 1.29.1
Upgrade Deno
To switch to the newest version:
deno upgrade
If you want a specific version:
deno upgrade --version 1.29.1
Deno will get the new version and update your current one.
It's a good idea to update Deno now and then to get the latest tools, security improvements, and fixes.
Your First Deno Program
Hello World
Starting with Deno is usually done with the simple "Hello World" program. This basic program shows a message to check that Deno is working and can run code.
Here's how to do a Hello World program in Deno:
console.log("Hello World");
To run this, put the code in a file like main.js
.
Next, open your terminal, go to where the file is, and type:
deno run main.js
If Deno is working right, you'll see "Hello World" on your terminal screen.
The deno run
command lets you run the JavaScript file, just like how node
works in Node.js.
Granting File Access
Deno is built to be safe, so it doesn't let scripts touch your files or the internet unless you specifically say it's okay.
For instance, if you try to read a local file without permission like this:
const text = Deno.readTextFileSync("readme.md");
And run it with:
deno run main.js
You'll get an error saying permission is denied because Deno blocks access to the file system by default.
To let it read the file, add the --allow-read
flag like this:
deno run --allow-read main.js
With this flag, the script can read readme.md
and put its content into the text
variable without any trouble.
These permission flags help keep things secure by letting you control what each script can do. Always give only the permissions you really need.
Core Concepts
Security Model
Deno keeps your code safe by making sure it can't access things like your files or the internet unless you say it's okay. This is like giving apps permission on your phone.
You can let your scripts do more by using special commands when you run them:
--allow-read
- Lets the script read files--allow-write
- Lets the script write to files--allow-net
- Lets the script access the internet--allow-env
- Lets the script access environment info
For example:
deno run --allow-net main.js
This setup means you're in control of what your code can and can't do, making things safer.
TypeScript Integration
Deno lets you use TypeScript directly. This means you can write .ts
files and run them without extra steps.
For example:
function add(a: number, b: number) {
return a + b;
}
console.log(add(1, 2)); // Shows 3
Using TypeScript helps avoid mistakes by making sure your code gets the right kind of data.
Module System
Deno uses web links to manage code pieces (modules) instead of a package manager. This is a bit like bookmarking a webpage.
For example:
import { serve } from "https://deno.land/std@0.114.0/http/server.ts";
This way of handling code makes it simpler to manage and share, since you just use URLs.
sbb-itb-bfaad5b
Developing in Deno
An HTTP Web Server
Let's start by making a simple web server with Deno. You can do this using something called serve
that comes with Deno:
import { serve } from "https://deno.land/std@0.114.0/http/server.ts";
const server = serve({ port: 8000 });
console.log("HTTP webserver running. Access it at: http://localhost:8000/");
This code makes a web server that listens on port 8000. If you want to make it do something, like send a message back when someone visits, you can use this loop:
for await (const req of server) {
req.respond({ body: "Hello World" });
}
Now, anyone who visits your server will get a "Hello World" message. You can also make it send different messages based on the URL someone visits:
for await (const req of server) {
if (req.url === "/") {
req.respond({ body: "Home page" });
} else if (req.url === "/about") {
req.respond({ body: "About page" });
}
}
Using the Standard Library
Deno comes with a bunch of built-in tools that are really handy. Like, you can easily read and write files with these commands:
import { readFileStr, writeFileStr } from "https://deno.land/std@0.114.0/fs/mod.ts";
const data = await readFileStr("message.txt");
console.log(data);
await writeFileStr("hello.txt", "Hello World");
You could even make a simple database using files with Deno's file tools.
Deno has more built-in tools for things like working with dates, waiting on things to happen, and dealing with data in different formats.
Third-Party Modules
Besides the built-in stuff, you can also use code made by other people in your Deno projects. For example, there's a web framework called Oak that makes handling web requests easier:
import { Application } from "https://deno.land/x/oak/mod.ts";
const app = new Application();
app.use((ctx) => {
ctx.response.body = "Hello world!";
});
await app.listen({ port: 8000 });
With Oak, you get a lot of web server stuff done for you. You can find more third-party code like this on Deno's website.
Testing in Deno
Deno makes it easy to check if your code works right by letting you write and run tests.
Writing Tests
Deno has a simple way to help you test your code. Here's how you can write tests:
import { assertEquals } from "https://deno.land/std/testing/asserts.ts";
Deno.test("add function", () => {
function add(a, b) {
return a + b;
}
assertEquals(add(1, 2), 3);
});
Deno.test("capitalize", () => {
function capitalize(text) {
return text[0].toUpperCase() + text.slice(1).toLowerCase();
}
assertEquals(capitalize("hello"), "Hello");
});
You use Deno.test()
to tell Deno what to check. You give it a name and a function that does the checking. The assertEquals()
part is where you say what the right answer should be. This is great for making sure small parts of your code are doing what they're supposed to do.
Here are some tips:
- Name your tests clearly
- Use
assert
functions to check if things are right - Focus on one thing in each test
- If your code is supposed to wait for something, your test should too
Good tests help find problems early and make sure changes don't break things.
Running Tests
To run your tests, type this in the terminal:
deno test my_test.ts
Deno will run all the tests in that file and tell you what passed and what didn't.
Here are some options you can use:
--coverage
- Tells you how much of your code the tests checked--fail-fast
- Stops after the first test fails--filter <pattern>
- Only runs tests that match the name you give
For example, if you just want to run tests about adding numbers:
deno test --filter "add" my_test.ts
It's important to write tests that really check your code well. Try to cover all the main things your code should do. But remember, it's also about being smart with your tests, not just about having a lot of them.
Deno vs Node.js
Let's talk about how Deno and Node.js are alike and different. Both are tools for running JavaScript code, but they have some unique features. Here's a simple table to show you the main differences:
Feature | Deno | Node.js |
---|---|---|
Language | TypeScript + JavaScript | JavaScript |
Console Output | Colors | No Colors |
Package Manager | URL imports | npm |
File System API | Modern style | Callback-based |
Async Handling | Top level await | Callbacks/Promises |
Testing | Built-in | External libraries |
Security | Secure by default | Opt-in security |
Language
- Deno can use TypeScript and JavaScript right from the start. TypeScript is great because it helps find mistakes before your code runs.
- Node.js uses just JavaScript by itself. You can add TypeScript, but you need extra tools for that.
Console Output
- Deno makes your console output colorful. This makes it easier to see what's going on when you're debugging.
- Node.js keeps it simple with no colors, but you can add them with some extra work.
Package Manager
- Deno lets you import packages directly from the web using links. This method is a bit more hands-on but avoids some common headaches.
- Node.js uses a package manager called npm to handle all the packages. It's more straightforward but can sometimes lead to problems.
File System API
- Deno's file system access is modern and uses promises, which are easier to work with.
- Node.js uses an older style that relies on callbacks, making things a bit more complicated.
Async Handling
- Deno allows you to wait for things right at the top of your code, making it clearer and less cluttered.
- Node.js uses callbacks and promises, which means you often have to jump around in your code.
Testing
- Deno includes tools for testing your code right out of the box.
- For testing in Node.js, you need to get extra tools like Mocha or Chai.
Security
- Deno is very cautious and doesn't let your code do certain things unless you specifically allow it.
- Node.js gives your code more freedom by default, which can be risky unless you take steps to make it safer.
In short, Deno tries to improve on some things that people found tricky in Node.js, like security and making coding simpler. Node.js, on the other hand, has a big community and lots of packages ready to use.
Conclusion
Key Takeaways
Deno is a tool designed to make working with JavaScript and TypeScript safer and easier, especially when compared to Node.js. Here are the main points to remember:
- Deno has everything you need built-in, like support for TypeScript, a bunch of helpful tools, and a standard library. This means you can get started on projects faster.
- It's built to be safe. You have to give it permission to access your files or the internet, which helps keep your computer secure.
- Instead of using a package manager like npm, Deno uses web links for adding other people's code to your project. This can make things simpler.
- Deno makes dealing with asynchronous operations smoother with top-level await and promises, so you don't get stuck in callback hell.
- Deno is still new and not as widely used as Node.js, but it's growing and has a lot to offer.
Next Steps
If you're interested in learning more about Deno:
- Try making a small project with Deno, using its web APIs and the standard library.
- Look into what other people have created with Deno, like frameworks and tools, to see what's out there.
- See how Deno compares to Node.js by using both on different projects.
- Check out how to put your Deno apps online, using things like Docker or Deno Deploy.
- Stay updated with Deno by following its updates and new releases.
Deno has a lot of potential for web development alongside Node.js. It's worth keeping an eye on as it continues to grow and improve.