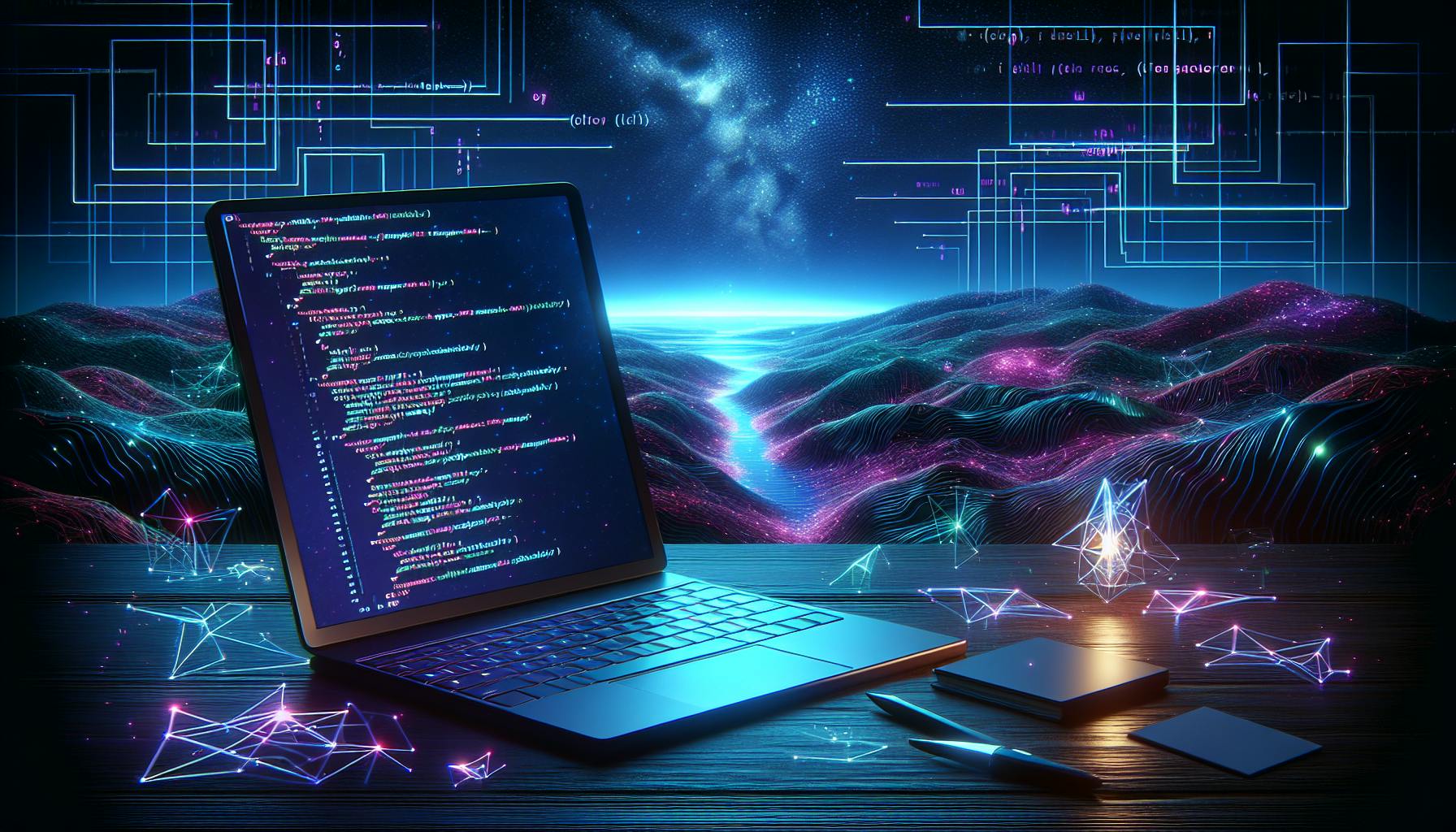
Discover the essentials of TypeScript (Type JS) for developers, from setting up a TypeScript environment to advanced features and using TypeScript with React. Learn how TypeScript enhances JavaScript development.
TypeScript, often referred to as Type JS, supercharges JavaScript development by adding type safety and other powerful features, making it an essential skill for developers. Here's what you need to know:
- TypeScript Basics: It's JavaScript with types, offering early error detection, better editor support, and features like enums and generics not present in plain JavaScript.
- Setting Up: You'll need Node.js, npm, a code editor (VS Code recommended), and the TypeScript compiler. Initialize your project with a specific
npm install
command and configure withtsconfig.json
. - Syntax Overview: Learn to declare variables, functions, and classes with types to reduce errors and manage code more effectively.
- Advanced Features: Dive into generics for reusable code, enums for clearer constants, and decorators for enhanced class functionality.
- Using TypeScript with React: TypeScript improves React development by providing type safety for props, state, and events, leading to fewer bugs and cleaner code.
This guide serves as a comprehensive introduction to TypeScript (Type JS), highlighting its importance and how to effectively incorporate it into your development workflow for cleaner, more maintainable code.
Setting Up a TypeScript Development Environment
To use TypeScript, you'll need:
- A project with Node.js and npm (a way to manage packages)
- A code editor that understands TypeScript (like VS Code)
- The TypeScript compiler (a program that turns your TypeScript into JavaScript)
To add TypeScript to your project, run:
npm install --save-dev typescript
Then set up your project with:
npx tsc --init --rootDir src --outDir build --esModuleInterop --resolveJsonModule --lib es6 --module commonjs --allowJs true --noImplicitAny true
This command creates a config file (tsconfig.json) with good default settings for a Node.js project.
Now, you can start writing TypeScript code in files like src/index.ts
, and they'll be turned into JavaScript files like build/index.js
.
The tsconfig.json file is important because it tells TypeScript how to turn your code into JavaScript. You might need to tweak it as you add more TypeScript to your project.
TypeScript Syntax Basics
TypeScript adds some extra rules to JavaScript, making it easier to catch mistakes by specifying the kind of data (like text or numbers) your variables should hold. Let's dive into the basics of TypeScript.
Variable Declarations
In TypeScript, you can tell exactly what type of data a variable should have:
let name: string = "John";
let age: number = 30;
let isActive: boolean = true;
This means the system will check to make sure the values fit those types. If you don't specify a type, TypeScript can guess based on the value you give it:
let hobbies = ["coding", "biking"]; // seen as an array of strings
Functions
When you write functions in TypeScript, you can be clear about what type of data they take in and what they give back:
function getName(id: number): string {
//...
return name;
}
And the same goes for arrow functions:
const log = (message: string): void => {
console.log(message);
}
Classes
TypeScript also has a special way to create classes:
class Person {
// Fields
name: string;
// Constructor
constructor(name: string) {
this.name = name;
}
// Methods
greet() {
console.log(`Hello, ${this.name}!")
}
}
const person = new Person("Maria");
person.greet();
This setup supports adding features like inheritance, which means a class can inherit properties from another. It also allows for private and public access modifiers, which control where and how properties of the class can be accessed.
So, these are the basics of TypeScript syntax - setting up variables, functions, and classes with specific types. This helps in writing code that's easier to manage and less prone to errors.
Advanced Features
Generics
Generics in TypeScript let you make components that can work with many types of data, not just one. This means you can use the same piece of code for different data types, keeping things simple and safe.
Here's how you can make a generic function that works with any type of data, using T
as a placeholder for the type:
function identity<T>(arg: T): T {
return arg;
}
let output = identity<string>("myString");
You can also make generic classes. Take a look at this Cache
class that can store any type of data:
class Cache<T> {
store: T;
constructor(value: T) {
this.store = value;
}
}
let stringCache = new Cache<string>("cached string");
Generics help you keep your code flexible and type-safe without having to write new functions or classes for each type you work with.
Enumerations
Enums let you set up a group of named numeric constants, making your code easier to read and understand. Here's an example:
enum Direction {
Up = 1,
Down = 2,
Left = 3,
Right = 4
}
let current = Direction.Up;
Enums can help make clear what your code is about, like using them for days of the week, status codes, or error codes. They're great for when you have a set of numbers that all relate to one another.
Decorators
Decorators are a cool way to add extra features to your classes and their parts. They let you add more behavior without changing the original code.
Here's a simple example where we log messages whenever a property is accessed or changed:
function Log(target: any, propertyKey: string) {
let value = target[propertyKey];
const getter = () => {
console.log(`Getting ${propertyKey}`);
return value;
};
const setter = (newVal) => {
console.log(`Setting ${propertyKey}`);
value = newVal;
}
Object.defineProperty(target, propertyKey, {
get: getter,
set: setter
});
}
class Person {
@Log
name: string;
constructor(name: string) {
this.name = name;
}
}
let person = new Person("John");
person.name = "Sara";
Decorators make it possible to easily track changes or add functionality, opening up new ways to use your code.
sbb-itb-bfaad5b
TypeScript with React
TypeScript can make building React apps a lot smoother by catching mistakes early and helping with code suggestions. Here’s a simple guide on using TypeScript for props, state, and handling events in React.
Typing Props
You can clearly define what types of data your props should be with interfaces:
interface Props {
message: string;
count: number;
disabled: boolean;
names: string[];
handler: (n: number) => void;
}
function MyComponent(props: Props) {
//...
}
This way, you'll know right away if the wrong type of prop is used.
For props that might not always be there, add ?
:
interface Props {
title?: string;
}
And if a prop can be more than one type, use unions:
interface Props {
value: number | string;
}
Typing State
Setting up state types works similarly, but with a State
interface:
interface State {
count: number;
isLoading: boolean;
}
class App extends React.Component<{}, State> {
state = {
count: 0,
isLoading: false
}
}
Typing Events
To avoid mistakes in event handlers, define the type of the event object:
interface Props {
onChange: (event: React.ChangeEvent<HTMLInputElement>) => void;
}
function MyComponent({onChange}: Props) {
return <input onChange={onChange}>
}
Or specify what the event handler should expect:
interface Props {
onChange: (id: number, value: string) => void;
}
This approach makes handling forms, clicks, and other events much clearer and helps find problems before they happen.
In short, TypeScript helps you be precise about what should go where in React components, making coding less prone to errors and more straightforward.
Conclusion
TypeScript is like a supercharged version of JavaScript that's great for building websites and apps. It adds some cool features to JavaScript, making it easier to catch mistakes and write better code.
Here are some reasons why TypeScript is helpful:
-
Catching Errors Early: TypeScript helps you find mistakes before your code runs. This means you spend less time fixing bugs.
-
Better Code Suggestions: Because TypeScript understands your code better, it can offer helpful tips and shortcuts as you write.
-
More Cool Tools: TypeScript adds neat things like enums, generics, and decorators to JavaScript. These make your code easier to understand and reuse.
-
Easy to Start: You can start using TypeScript in your current JavaScript projects bit by bit. It also works well with all web browsers.
-
Works with Popular Tools: TypeScript works smoothly with many tools developers love to use for building websites and apps.
Overall, TypeScript makes your JavaScript projects better, especially when they get big or when you're working with a team. If you're already familiar with JavaScript, learning TypeScript won't be too hard. More and more developers are using TypeScript for their projects.
To learn more about TypeScript, check out these resources:
- TypeScript Documentation - The official guide with all you need to know.
- TypeScript Deep Dive - A book that goes into detail about TypeScript.
- TypeScript Evolution - Find out what's new in TypeScript.
Try TypeScript for your next project and see how it makes coding easier and more fun!
Related Questions
What JavaScript developer must know?
If you're diving into JavaScript, here are some key things to get a handle on:
- Understanding the basics of JavaScript like variables, data types, loops, functions, and how variables work.
- Getting to know popular tools and libraries such as React, Angular, and Vue.
- Learning HTML and CSS basics to make web pages.
- Knowing how to use tools like Git for tracking changes in your code, and npm for managing project packages.
- Grasping web concepts like the Document Object Model (DOM) API, which lets you interact with web pages.
- Debugging code in web browsers to fix issues.
- Understanding how web apps work and how to connect with web services using REST APIs.
- Keeping up with the latest JavaScript features and syntax.
- Learning about asynchronous programming, which involves handling operations that take time, like fetching data from the internet.
- Testing your code with tools like Jest to catch bugs.
- Staying curious and continuously learning about new JavaScript developments and best practices.
Focusing first on core JavaScript skills before diving into more complex frameworks and tools is crucial.
What are JavaScript essentials?
Here are some must-knows for JavaScript developers:
- Data Types: Knowing the difference between simple data like numbers and strings, and more complex types like objects and arrays.
- Variables: How to declare and use variables with
var
,let
,const
. - Operators: Using basic math and logic operations in your code.
- Conditional Logic: Making decisions in code with
if/else
and switch. - Loops: Repeating tasks with for and while loops.
- Functions: Creating reusable code blocks.
- Scope: Understanding how variables are seen in your code.
- DOM Manipulation: Changing web page elements.
- Events: Setting up responses to user actions like clicks.
- OOP: Organizing code with objects and classes.
- Asynchronous Programming: Managing code that runs later, like after fetching data.
- Error Handling: Catching and dealing with errors.
Mastering these basics is key to becoming proficient in JavaScript.
What are the 8 types of JavaScript?
JavaScript has 8 basic data types:
- Number: For all kinds of numbers.
- String: For text.
- Boolean: For true/false.
- Null: Means 'no value'.
- Undefined: Used when a variable hasn't been given a value.
- Symbol: A unique and unchangeable value.
- BigInt: For really big numbers.
- Object: For collections of data.
Understanding these types is crucial for working with data in JavaScript.
What are the basic toolkit needed to develop JavaScript?
To start developing in JavaScript, you'll need:
- A text editor like Visual Studio Code to write your code.
- A web browser like Chrome or Firefox for testing and debugging.
- Node.js to run JavaScript outside a browser.
- npm to add libraries or tools to your project.
- Git for version control.
- Babel to make your JavaScript work in all browsers.
- ESLint to help write better code.
- Webpack to bundle your code and files together.
- Jest for testing your code.
With these tools, you're ready to start building JavaScript projects.